Just changed OUTPUT_SIZE and INPUT_SIZE in ros/node_handle.h
Dependents: WRS2020_mecanum_node
JointJog.h
00001 #ifndef _ROS_control_msgs_JointJog_h 00002 #define _ROS_control_msgs_JointJog_h 00003 00004 #include <stdint.h> 00005 #include <string.h> 00006 #include <stdlib.h> 00007 #include "ros/msg.h" 00008 #include "std_msgs/Header.h" 00009 00010 namespace control_msgs 00011 { 00012 00013 class JointJog : public ros::Msg 00014 { 00015 public: 00016 typedef std_msgs::Header _header_type; 00017 _header_type header; 00018 uint32_t joint_names_length; 00019 typedef char* _joint_names_type; 00020 _joint_names_type st_joint_names; 00021 _joint_names_type * joint_names; 00022 uint32_t displacements_length; 00023 typedef double _displacements_type; 00024 _displacements_type st_displacements; 00025 _displacements_type * displacements; 00026 uint32_t velocities_length; 00027 typedef double _velocities_type; 00028 _velocities_type st_velocities; 00029 _velocities_type * velocities; 00030 typedef double _duration_type; 00031 _duration_type duration; 00032 00033 JointJog(): 00034 header(), 00035 joint_names_length(0), joint_names(NULL), 00036 displacements_length(0), displacements(NULL), 00037 velocities_length(0), velocities(NULL), 00038 duration(0) 00039 { 00040 } 00041 00042 virtual int serialize(unsigned char *outbuffer) const 00043 { 00044 int offset = 0; 00045 offset += this->header.serialize(outbuffer + offset); 00046 *(outbuffer + offset + 0) = (this->joint_names_length >> (8 * 0)) & 0xFF; 00047 *(outbuffer + offset + 1) = (this->joint_names_length >> (8 * 1)) & 0xFF; 00048 *(outbuffer + offset + 2) = (this->joint_names_length >> (8 * 2)) & 0xFF; 00049 *(outbuffer + offset + 3) = (this->joint_names_length >> (8 * 3)) & 0xFF; 00050 offset += sizeof(this->joint_names_length); 00051 for( uint32_t i = 0; i < joint_names_length; i++){ 00052 uint32_t length_joint_namesi = strlen(this->joint_names[i]); 00053 varToArr(outbuffer + offset, length_joint_namesi); 00054 offset += 4; 00055 memcpy(outbuffer + offset, this->joint_names[i], length_joint_namesi); 00056 offset += length_joint_namesi; 00057 } 00058 *(outbuffer + offset + 0) = (this->displacements_length >> (8 * 0)) & 0xFF; 00059 *(outbuffer + offset + 1) = (this->displacements_length >> (8 * 1)) & 0xFF; 00060 *(outbuffer + offset + 2) = (this->displacements_length >> (8 * 2)) & 0xFF; 00061 *(outbuffer + offset + 3) = (this->displacements_length >> (8 * 3)) & 0xFF; 00062 offset += sizeof(this->displacements_length); 00063 for( uint32_t i = 0; i < displacements_length; i++){ 00064 union { 00065 double real; 00066 uint64_t base; 00067 } u_displacementsi; 00068 u_displacementsi.real = this->displacements[i]; 00069 *(outbuffer + offset + 0) = (u_displacementsi.base >> (8 * 0)) & 0xFF; 00070 *(outbuffer + offset + 1) = (u_displacementsi.base >> (8 * 1)) & 0xFF; 00071 *(outbuffer + offset + 2) = (u_displacementsi.base >> (8 * 2)) & 0xFF; 00072 *(outbuffer + offset + 3) = (u_displacementsi.base >> (8 * 3)) & 0xFF; 00073 *(outbuffer + offset + 4) = (u_displacementsi.base >> (8 * 4)) & 0xFF; 00074 *(outbuffer + offset + 5) = (u_displacementsi.base >> (8 * 5)) & 0xFF; 00075 *(outbuffer + offset + 6) = (u_displacementsi.base >> (8 * 6)) & 0xFF; 00076 *(outbuffer + offset + 7) = (u_displacementsi.base >> (8 * 7)) & 0xFF; 00077 offset += sizeof(this->displacements[i]); 00078 } 00079 *(outbuffer + offset + 0) = (this->velocities_length >> (8 * 0)) & 0xFF; 00080 *(outbuffer + offset + 1) = (this->velocities_length >> (8 * 1)) & 0xFF; 00081 *(outbuffer + offset + 2) = (this->velocities_length >> (8 * 2)) & 0xFF; 00082 *(outbuffer + offset + 3) = (this->velocities_length >> (8 * 3)) & 0xFF; 00083 offset += sizeof(this->velocities_length); 00084 for( uint32_t i = 0; i < velocities_length; i++){ 00085 union { 00086 double real; 00087 uint64_t base; 00088 } u_velocitiesi; 00089 u_velocitiesi.real = this->velocities[i]; 00090 *(outbuffer + offset + 0) = (u_velocitiesi.base >> (8 * 0)) & 0xFF; 00091 *(outbuffer + offset + 1) = (u_velocitiesi.base >> (8 * 1)) & 0xFF; 00092 *(outbuffer + offset + 2) = (u_velocitiesi.base >> (8 * 2)) & 0xFF; 00093 *(outbuffer + offset + 3) = (u_velocitiesi.base >> (8 * 3)) & 0xFF; 00094 *(outbuffer + offset + 4) = (u_velocitiesi.base >> (8 * 4)) & 0xFF; 00095 *(outbuffer + offset + 5) = (u_velocitiesi.base >> (8 * 5)) & 0xFF; 00096 *(outbuffer + offset + 6) = (u_velocitiesi.base >> (8 * 6)) & 0xFF; 00097 *(outbuffer + offset + 7) = (u_velocitiesi.base >> (8 * 7)) & 0xFF; 00098 offset += sizeof(this->velocities[i]); 00099 } 00100 union { 00101 double real; 00102 uint64_t base; 00103 } u_duration; 00104 u_duration.real = this->duration; 00105 *(outbuffer + offset + 0) = (u_duration.base >> (8 * 0)) & 0xFF; 00106 *(outbuffer + offset + 1) = (u_duration.base >> (8 * 1)) & 0xFF; 00107 *(outbuffer + offset + 2) = (u_duration.base >> (8 * 2)) & 0xFF; 00108 *(outbuffer + offset + 3) = (u_duration.base >> (8 * 3)) & 0xFF; 00109 *(outbuffer + offset + 4) = (u_duration.base >> (8 * 4)) & 0xFF; 00110 *(outbuffer + offset + 5) = (u_duration.base >> (8 * 5)) & 0xFF; 00111 *(outbuffer + offset + 6) = (u_duration.base >> (8 * 6)) & 0xFF; 00112 *(outbuffer + offset + 7) = (u_duration.base >> (8 * 7)) & 0xFF; 00113 offset += sizeof(this->duration); 00114 return offset; 00115 } 00116 00117 virtual int deserialize(unsigned char *inbuffer) 00118 { 00119 int offset = 0; 00120 offset += this->header.deserialize(inbuffer + offset); 00121 uint32_t joint_names_lengthT = ((uint32_t) (*(inbuffer + offset))); 00122 joint_names_lengthT |= ((uint32_t) (*(inbuffer + offset + 1))) << (8 * 1); 00123 joint_names_lengthT |= ((uint32_t) (*(inbuffer + offset + 2))) << (8 * 2); 00124 joint_names_lengthT |= ((uint32_t) (*(inbuffer + offset + 3))) << (8 * 3); 00125 offset += sizeof(this->joint_names_length); 00126 if(joint_names_lengthT > joint_names_length) 00127 this->joint_names = (char**)realloc(this->joint_names, joint_names_lengthT * sizeof(char*)); 00128 joint_names_length = joint_names_lengthT; 00129 for( uint32_t i = 0; i < joint_names_length; i++){ 00130 uint32_t length_st_joint_names; 00131 arrToVar(length_st_joint_names, (inbuffer + offset)); 00132 offset += 4; 00133 for(unsigned int k= offset; k< offset+length_st_joint_names; ++k){ 00134 inbuffer[k-1]=inbuffer[k]; 00135 } 00136 inbuffer[offset+length_st_joint_names-1]=0; 00137 this->st_joint_names = (char *)(inbuffer + offset-1); 00138 offset += length_st_joint_names; 00139 memcpy( &(this->joint_names[i]), &(this->st_joint_names), sizeof(char*)); 00140 } 00141 uint32_t displacements_lengthT = ((uint32_t) (*(inbuffer + offset))); 00142 displacements_lengthT |= ((uint32_t) (*(inbuffer + offset + 1))) << (8 * 1); 00143 displacements_lengthT |= ((uint32_t) (*(inbuffer + offset + 2))) << (8 * 2); 00144 displacements_lengthT |= ((uint32_t) (*(inbuffer + offset + 3))) << (8 * 3); 00145 offset += sizeof(this->displacements_length); 00146 if(displacements_lengthT > displacements_length) 00147 this->displacements = (double*)realloc(this->displacements, displacements_lengthT * sizeof(double)); 00148 displacements_length = displacements_lengthT; 00149 for( uint32_t i = 0; i < displacements_length; i++){ 00150 union { 00151 double real; 00152 uint64_t base; 00153 } u_st_displacements; 00154 u_st_displacements.base = 0; 00155 u_st_displacements.base |= ((uint64_t) (*(inbuffer + offset + 0))) << (8 * 0); 00156 u_st_displacements.base |= ((uint64_t) (*(inbuffer + offset + 1))) << (8 * 1); 00157 u_st_displacements.base |= ((uint64_t) (*(inbuffer + offset + 2))) << (8 * 2); 00158 u_st_displacements.base |= ((uint64_t) (*(inbuffer + offset + 3))) << (8 * 3); 00159 u_st_displacements.base |= ((uint64_t) (*(inbuffer + offset + 4))) << (8 * 4); 00160 u_st_displacements.base |= ((uint64_t) (*(inbuffer + offset + 5))) << (8 * 5); 00161 u_st_displacements.base |= ((uint64_t) (*(inbuffer + offset + 6))) << (8 * 6); 00162 u_st_displacements.base |= ((uint64_t) (*(inbuffer + offset + 7))) << (8 * 7); 00163 this->st_displacements = u_st_displacements.real; 00164 offset += sizeof(this->st_displacements); 00165 memcpy( &(this->displacements[i]), &(this->st_displacements), sizeof(double)); 00166 } 00167 uint32_t velocities_lengthT = ((uint32_t) (*(inbuffer + offset))); 00168 velocities_lengthT |= ((uint32_t) (*(inbuffer + offset + 1))) << (8 * 1); 00169 velocities_lengthT |= ((uint32_t) (*(inbuffer + offset + 2))) << (8 * 2); 00170 velocities_lengthT |= ((uint32_t) (*(inbuffer + offset + 3))) << (8 * 3); 00171 offset += sizeof(this->velocities_length); 00172 if(velocities_lengthT > velocities_length) 00173 this->velocities = (double*)realloc(this->velocities, velocities_lengthT * sizeof(double)); 00174 velocities_length = velocities_lengthT; 00175 for( uint32_t i = 0; i < velocities_length; i++){ 00176 union { 00177 double real; 00178 uint64_t base; 00179 } u_st_velocities; 00180 u_st_velocities.base = 0; 00181 u_st_velocities.base |= ((uint64_t) (*(inbuffer + offset + 0))) << (8 * 0); 00182 u_st_velocities.base |= ((uint64_t) (*(inbuffer + offset + 1))) << (8 * 1); 00183 u_st_velocities.base |= ((uint64_t) (*(inbuffer + offset + 2))) << (8 * 2); 00184 u_st_velocities.base |= ((uint64_t) (*(inbuffer + offset + 3))) << (8 * 3); 00185 u_st_velocities.base |= ((uint64_t) (*(inbuffer + offset + 4))) << (8 * 4); 00186 u_st_velocities.base |= ((uint64_t) (*(inbuffer + offset + 5))) << (8 * 5); 00187 u_st_velocities.base |= ((uint64_t) (*(inbuffer + offset + 6))) << (8 * 6); 00188 u_st_velocities.base |= ((uint64_t) (*(inbuffer + offset + 7))) << (8 * 7); 00189 this->st_velocities = u_st_velocities.real; 00190 offset += sizeof(this->st_velocities); 00191 memcpy( &(this->velocities[i]), &(this->st_velocities), sizeof(double)); 00192 } 00193 union { 00194 double real; 00195 uint64_t base; 00196 } u_duration; 00197 u_duration.base = 0; 00198 u_duration.base |= ((uint64_t) (*(inbuffer + offset + 0))) << (8 * 0); 00199 u_duration.base |= ((uint64_t) (*(inbuffer + offset + 1))) << (8 * 1); 00200 u_duration.base |= ((uint64_t) (*(inbuffer + offset + 2))) << (8 * 2); 00201 u_duration.base |= ((uint64_t) (*(inbuffer + offset + 3))) << (8 * 3); 00202 u_duration.base |= ((uint64_t) (*(inbuffer + offset + 4))) << (8 * 4); 00203 u_duration.base |= ((uint64_t) (*(inbuffer + offset + 5))) << (8 * 5); 00204 u_duration.base |= ((uint64_t) (*(inbuffer + offset + 6))) << (8 * 6); 00205 u_duration.base |= ((uint64_t) (*(inbuffer + offset + 7))) << (8 * 7); 00206 this->duration = u_duration.real; 00207 offset += sizeof(this->duration); 00208 return offset; 00209 } 00210 00211 const char * getType(){ return "control_msgs/JointJog"; }; 00212 const char * getMD5(){ return "1685da700c8c2e1254afc92a5fb89c96"; }; 00213 00214 }; 00215 00216 } 00217 #endif
Generated on Tue Jul 12 2022 18:49:19 by
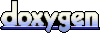