
max4146x_comp
Embed:
(wiki syntax)
Show/hide line numbers
Max4146x.h
00001 /******************************************************************************* 00002 * Copyright (C) 2019 Maxim Integrated Products, Inc., All rights Reserved. 00003 * 00004 * This software is protected by copyright laws of the United States and 00005 * of foreign countries. This material may also be protected by patent laws 00006 * and technology transfer regulations of the United States and of foreign 00007 * countries. This software is furnished under a license agreement and/or a 00008 * nondisclosure agreement and may only be used or reproduced in accordance 00009 * with the terms of those agreements. Dissemination of this information to 00010 * any party or parties not specified in the license agreement and/or 00011 * nondisclosure agreement is expressly prohibited. 00012 * 00013 * The above copyright notice and this permission notice shall be included 00014 * in all copies or substantial portions of the Software. 00015 * 00016 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00017 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00018 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00019 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00020 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00021 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00022 * OTHER DEALINGS IN THE SOFTWARE. 00023 * 00024 * Except as contained in this notice, the name of Maxim Integrated 00025 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00026 * Products, Inc. Branding Policy. 00027 * 00028 * The mere transfer of this software does not imply any licenses 00029 * of trade secrets, proprietary technology, copyrights, patents, 00030 * trademarks, maskwork rights, or any other form of intellectual 00031 * property whatsoever. Maxim Integrated Products, Inc. retains all 00032 * ownership rights. 00033 ******************************************************************************* 00034 */ 00035 00036 #ifndef MAX4146x_H_ 00037 #define MAX4146x_H_ 00038 00039 #include "mbed.h" 00040 #include "Max41460_regs.h" 00041 #include "Max41461_2_regs.h" 00042 #include "Max41463_4_regs.h" 00043 00044 #define I2C_ADDRESS 0xD2 00045 00046 /** 00047 * @brief Base Class for All Maxim Max4146x RF Transmitters 00048 * 00049 * @details The MAX4146X is a UHF sub-GHz ISM/SRD transmitter 00050 */ 00051 template <class REG> 00052 class MAX4146X 00053 { 00054 private: 00055 REG *reg; 00056 I2C *i2c_handler; 00057 SPI *spi_handler; 00058 DigitalOut *ssel; 00059 00060 //manchester coding variables 00061 unsigned char *manchester_bit_array; 00062 unsigned char *bits_array; 00063 static const unsigned char mask = 1; // Bit mask 00064 char data_rate; 00065 DigitalOut *data_sent; // data sent pin 00066 00067 uint8_t preset_mode; 00068 float crystal_frequency ; 00069 float center_frequency; 00070 float baud_rate; 00071 00072 typedef enum { 00073 CFG1_ADDR = 0x00, 00074 CFG2_ADDR = 0x01, 00075 CFG3_ADDR = 0x02, 00076 CFG4_ADDR = 0x03, 00077 CFG5_ADDR = 0x04, 00078 SHDN_ADDR = 0x05, 00079 PA1_ADDR = 0x06, 00080 PA2_ADDR = 0x07, 00081 PLL1_ADDR = 0x08, 00082 PLL2_ADDR = 0x09, 00083 CFG6_ADDR = 0x0A, 00084 PLL3_ADDR = 0x0B, 00085 PLL4_ADDR = 0x0C, 00086 PLL5_ADDR = 0x0D, 00087 PLL6_ADDR = 0x0E, 00088 PLL7_ADDR = 0x0F, 00089 CFG7_ADDR = 0x10, 00090 I2C1_ADDR = 0x11, 00091 I2C2_ADDR = 0x12, 00092 I2C3_ADDR = 0x13, 00093 I2C4_ADDR = 0x14, 00094 I2C5_ADDR = 0x15, 00095 I2C6_ADDR = 0x16, 00096 CFG8_ADDR = 0x17, 00097 CFG9_ADDR = 0x18, 00098 ADDL1_ADDR = 0x19, 00099 ADDL2_ADDR = 0x1A, 00100 } register_address_t; 00101 00102 //Functions 00103 00104 protected: 00105 00106 //Functions 00107 int io_write(uint8_t *data, uint32_t length); 00108 00109 public: 00110 00111 //Constructors 00112 MAX4146X(REG *reg, SPI *spi, DigitalOut *cs); 00113 00114 MAX4146X(REG *reg, SPI *spi); 00115 00116 MAX4146X(REG *reg, I2C *i2c); 00117 00118 MAX4146X(DigitalOut *cs); 00119 00120 00121 /** 00122 * @brief Register Configuration 00123 * 00124 * @details 00125 * - Register : CFG1(0x00) 00126 * - Bit Fields : [7:6] 00127 * - Default : 0x2 00128 * - Description : Start delay before enabling XO clock to digital block 00129 */ 00130 typedef enum { 00131 XOCLKDELAY_0_CYCLE, /**< 0x0: No delay. XO clock is immediately enabled to rest of digital block */ 00132 XOCLKDELAY_16_CYCLE, /**< 0x1: XO clock is enabled after 16 cycles to rest of digital block */ 00133 XOCLKDELAY_32_CYCLE, /**< 0x2: XO clock is enabled after 32 cycles to rest of digital block */ 00134 XOCLKDELAY_64_CYCLE, /**< 0x3: XO clock is enabled after 64 cycles to rest of digital block */ 00135 } xoclkdelay_t; 00136 00137 /** 00138 * @brief Set start delay before enabling XO clock to digital block 00139 * 00140 * @param[in] delay delay cycle 00141 * 00142 * @returns 0 on success, negative error code on failure. 00143 */ 00144 int set_xoclkdelay(xoclkdelay_t delay); 00145 00146 /** 00147 * @brief Get start delay before enabling XO clock to digital block 00148 * 00149 * @param[in] delay delay cycle 00150 * 00151 * @returns 0 on success, negative error code on failure. 00152 */ 00153 int get_xoclkdelay(xoclkdelay_t *delay); 00154 00155 /** 00156 * @brief Register Configuration 00157 * 00158 * @details 00159 * -Register : CFG1(0x00) 00160 * - Bit Fields : [5:4] 00161 * - Default : 0x2 00162 * - Description : XO clock division ratio for digital block 00163 */ 00164 typedef enum { 00165 XOCLKDIV_BY_4, /**< 0x0: Divide XO clock by 4 for digital clock */ 00166 XOCLKDIV_BY_5, /**< 0x1: Divide XO clock by 5 for digital clock. High time is 2 cycles, low time is 3 cycles */ 00167 XOCLKDIV_BY_6, /**< 0x2: Divide XO clock by 6 for digital clock */ 00168 XOCLKDIV_BY_7, /**< 0x3: Divide XO clock by 7 for digital clock. High time is 3 cycles, 00169 and low time is 4 cycles */ 00170 } xoclkdiv_t; 00171 00172 /** 00173 * @brief Set XO clock division ratio for digital block 00174 * 00175 * @param[in] div division ratio 00176 * 00177 * @returns 0 on success, negative error code on failure. 00178 */ 00179 int set_xoclkdiv(xoclkdiv_t div); 00180 00181 /** 00182 * @brief Get XO clock division ratio for digital block 00183 * 00184 * @param[in] div division ratio 00185 * 00186 * @returns 0 on success, negative error code on failure. 00187 */ 00188 int get_xoclkdiv(xoclkdiv_t *div); 00189 00190 /** 00191 * @brief Register Configuration 00192 * 00193 * @details 00194 * - Register : CFG1(0x00) 00195 * - Bit Fields : [2] 00196 * - Default : 0b0 00197 * - Description : Sets the state of FSK Gaussian Shaping 00198 */ 00199 typedef enum { 00200 FSKSHAPE_DISABLE, /**< 0x0: FSK Shaping disabled */ 00201 FSKSHAPE_ENABLE, /**< 0x1: FSK Shaping enabled */ 00202 } fskshape_t; 00203 00204 /** 00205 * @brief Sets the state of FSK Gaussian Shaping 00206 * 00207 * @param[in] shape enable/disable fskshaping 00208 * 00209 * @returns 0 on success, negative error code on failure. 00210 */ 00211 int set_fskshape(fskshape_t shape); 00212 00213 /** 00214 * @brief Gets the state of FSK Gaussian Shaping 00215 * 00216 * @param[in] shape enable/disable fskshaping 00217 * 00218 * @returns 0 on success, negative error code on failure. 00219 */ 00220 int get_fskshape(fskshape_t *shape); 00221 00222 /** 00223 * @brief Register Configuration 00224 * 00225 * @details 00226 * - Register : CFG1(0x00) 00227 * - Bit Fields : [1] 00228 * - Default : 0b0 00229 * - Description : Controls if clock output acts as an input. When an input, 00230 * it will sample the DATA pin. 00231 */ 00232 typedef enum { 00233 SYNC_0, /**< 0x0: asynchronous transmission mode */ 00234 SYNC_1, /**< 0x1: synchronous transmission mode */ 00235 } sync_t; 00236 00237 /** 00238 * @brief Sets the state of clock pin 00239 * 00240 * @param[in] state pin state async/sync 00241 * 00242 * @returns 0 on success, negative error code on failure. 00243 */ 00244 int set_sync(sync_t state); 00245 00246 /** 00247 * @brief Gets the state of clock pin 00248 * 00249 * @param[in] state pin state async/sync 00250 * 00251 * @returns 0 on success, negative error code on failure. 00252 */ 00253 int get_sync(sync_t *state); 00254 00255 /** 00256 * @brief Register Configuration 00257 * 00258 * @details 00259 * - Register : CFG1(0x00) 00260 * - Bit Fields : [0] 00261 * - Default : 0b0 00262 * - Description : Configures modulator mode 00263 */ 00264 typedef enum { 00265 MODMODE_ASK, /**< 0x0: ASK Mode */ 00266 MODMODE_FSK, /**< 0x1: FSK Mode */ 00267 } modmode_t; 00268 00269 /** 00270 * @brief Sets modulator mode to ASK or FSK 00271 * 00272 * @param[in] mode ASK or FSK 00273 * 00274 * @returns 0 on success, negative error code on failure. 00275 */ 00276 int set_modmode(modmode_t mode); 00277 00278 /** 00279 * @brief Gets modulator mode 00280 * 00281 * @param[in] mode ASK or FSK 00282 * 00283 * @returns 0 on success, negative error code on failure. 00284 */ 00285 int get_modmode(modmode_t* mode); 00286 00287 /** 00288 * @brief Register Configuration 00289 * 00290 * @details 00291 * - Register : CFG2(0x01) 00292 * - Bit Fields : [7:6] 00293 * - Default : 0x2 00294 * - Description : Selects the delay when CLKOUT starts 00295 * toggling upon exiting SHUTDOWN mode, 00296 * in divided XO clock cycles 00297 */ 00298 typedef enum { 00299 CLKOUT_DELAY_64_CYCLE, /**< 0x0: CLKOUT will start toggling after 64 cycles 00300 whenever moving into normal mode from shutdown mode */ 00301 CLKOUT_DELAY_128_CYCLE, /**< 0x1: CLKOUT will start toggling after 128 cycles 00302 whenever moving into normal mode from shutdown mode */ 00303 CLKOUT_DELAY_256_CYCLE, /**< 0x2: CLKOUT will start toggling after 256 cycles 00304 whenever moving into normal mode from shutdown mode */ 00305 CLKOUT_DELAY_512_CYCLE, /**< 0x3: CLKOUT will start toggling after 512 cycles 00306 whenever moving into normal mode from shutdown mode */ 00307 } clkout_delay_t; 00308 00309 /** 00310 * @brief Sets clkout delay 00311 * 00312 * @param[in] delay delay cycles 00313 * 00314 * @returns 0 on success, negative error code on failure. 00315 */ 00316 int set_clkout_delay(clkout_delay_t delay); 00317 00318 /** 00319 * @brief Gets clkout delay 00320 * 00321 * @param[in] delay delay cycles 00322 * 00323 * @returns 0 on success, negative error code on failure. 00324 */ 00325 int get_clkout_delay(clkout_delay_t* delay); 00326 00327 /** 00328 * @brief Register Configuration 00329 * 00330 * @details 00331 * - Register : CFG2(0x01) 00332 * - Bit Fields : [2:0] 00333 * - Default : 0x1 00334 * - Description : Baud clock post-divider setting. 00335 */ 00336 typedef enum { 00337 BCLK_POSTDIV_RESERVED_0, /**< 0x0: RESERVED */ 00338 BCLK_POSTDIV_BY_1, /**< 0x1: Divide by 1 */ 00339 BCLK_POSTDIV_BY_2, /**< 0x2: Divide by 2 */ 00340 BCLK_POSTDIV_BY_3, /**< 0x3: Divide by 3 */ 00341 BCLK_POSTDIV_BY_4, /**< 0x4: Divide by 4 */ 00342 BCLK_POSTDIV_BY_5, /**< 0x5: Divide by 5 */ 00343 BCLK_POSTDIV_RESERVED_6, /**< 0x6: RESERVED */ 00344 BCLK_POSTDIV_RESERVED_7, /**< 0x7: RESERVED */ 00345 } bclk_postdiv_t; 00346 00347 /** 00348 * @brief Sets baud clock post-divider 00349 * 00350 * @param[in] div divider value 00351 * 00352 * @returns 0 on success, negative error code on failure. 00353 */ 00354 int set_bclk_postdiv(bclk_postdiv_t div); 00355 00356 /** 00357 * @brief Gets baud clock post-divider 00358 * 00359 * @param[in] div divider value 00360 * 00361 * @returns 0 on success, negative error code on failure. 00362 */ 00363 int get_bclk_postdiv(bclk_postdiv_t* div); 00364 00365 /** 00366 * @brief Register Configuration 00367 * 00368 * @details 00369 * - Register : CFG4(0x03) 00370 * - Bit Fields : [1:0] 00371 * - Default : 0x0 00372 * - Description : Power Down Mode Select. 00373 */ 00374 typedef enum { 00375 PWDN_MODE_SHUTDOWN, /**< 0x0: SHUTDOWN low power state is enabled. While entering 00376 low power state, XO, PLL, and PA are shutdown. */ 00377 PWDN_MODE_STANDBY, /**< 0x1: STANDBY low power state is enabled. While entering 00378 low power state, XO is enabled. PLL and PA are shutdown */ 00379 PWDN_MODE_FAST_WAKEUP, /**< 0x2: FAST WAKEUP low power state is enabled. While entering 00380 low power state, XO and PLL are enabled. PA is shutdown. */ 00381 PWDN_MODE_REVERT_TO_FAST_WAKEUP, /**< 0x3: Will revert to 0x2 */ 00382 } pwdn_mode_t; 00383 00384 /** 00385 * @brief Sets power down mode 00386 * 00387 * @param[in] pwdn_mode power down mode 00388 * 00389 * @returns 0 on success, negative error code on failure. 00390 */ 00391 int set_pwdn_mode(pwdn_mode_t pwdn_mode); 00392 00393 /** 00394 * @brief Gets power down mode 00395 * 00396 * @param[in] pwdn_mode power down mode 00397 * 00398 * @returns 0 on success, negative error code on failure. 00399 */ 00400 int get_pwdn_mode(pwdn_mode_t* pwdn_mode); 00401 00402 /** 00403 * @brief Register Configuration 00404 * 00405 * @details 00406 * - Register : SHDN(0x05) 00407 * - Bit Fields : [0] 00408 * - Default : 0x0 00409 * - Description : Enables a boost in PA output power for frequencies above 850MHz. 00410 * This requires a different PA match compared to normal operation. 00411 */ 00412 typedef enum { 00413 PA_BOOST_NORMAL_MODE, /**< 0x0: PA Output power in normal operation. */ 00414 PA_BOOST_BOOST_MODE, /**< 0x1: PA Output power in boost mode for more output power. */ 00415 } pa_boost_t; 00416 00417 /** 00418 * @brief enable/disable boost mode 00419 * 00420 * @param[in] pa_boost power amplifier output mode 00421 * 00422 * @returns 0 on success, negative error code on failure. 00423 */ 00424 int set_pa_boost(pa_boost_t pa_boost); 00425 00426 /** 00427 * @brief Gets boost mode 00428 * 00429 * @param[in] pa_boost power amplifier output mode 00430 * 00431 * @returns 0 on success, negative error code on failure. 00432 */ 00433 int get_pa_boost(pa_boost_t* pa_boost); 00434 00435 /** 00436 * @brief Register Configuration 00437 * 00438 * @details 00439 * - Register : PA1(0x06) 00440 * - Bit Fields : [2:0] 00441 * - Default : 0x0 00442 * - Description : Controls the PA output power by enabling parallel drivers. 00443 */ 00444 typedef enum { 00445 PAPWR_1_DRIVER, /**< 0x0: Minimum, 1 driver */ 00446 PAPWR_2_DRIVER, /**< 0x1: 2 Drivers */ 00447 PAPWR_3_DRIVER, /**< 0x2: 3 Drivers */ 00448 PAPWR_4_DRIVER, /**< 0x3: 4 Drivers */ 00449 PAPWR_5_DRIVER, /**< 0x4: 5 Drivers */ 00450 PAPWR_6_DRIVER, /**< 0x5: 6 Drivers */ 00451 PAPWR_7_DRIVER, /**< 0x6: 7 Drivers */ 00452 PAPWR_8_DRIVER, /**< 0x7: 8 Drivers */ 00453 } papwr_t; 00454 00455 /** 00456 * @brief set PA output power by enabling parallel drivers 00457 * 00458 * @param[in] papwr number of parallel drivers 00459 * 00460 * @returns 0 on success, negative error code on failure. 00461 */ 00462 int set_papwr(papwr_t papwr); 00463 00464 /** 00465 * @brief Gets PA output power 00466 * 00467 * @param[in] papwr number of parallel drivers 00468 * 00469 * @returns 0 on success, negative error code on failure. 00470 */ 00471 int get_papwr(papwr_t* papwr); 00472 00473 00474 /** 00475 * @brief Register Configuration 00476 * 00477 * @details 00478 * - Register : PA2(0x07) 00479 * - Bit Fields : [4:0] 00480 * - Default : 0x00 00481 * - Description : Controls shunt capacitance on PA output in fF. 00482 */ 00483 typedef enum { 00484 PACAP_0_fF, 00485 PACAP_175_fF, 00486 PACAP_350_fF, 00487 PACAP_525_fF, 00488 PACAP_700_fF, 00489 PACAP_875_fF, 00490 PACAP_1050_fF, 00491 PACAP_1225_fF, 00492 PACAP_1400_fF, 00493 PACAP_1575_fF, 00494 PACAP_1750_fF, 00495 PACAP_1925_fF, 00496 PACAP_2100_fF, 00497 PACAP_2275_fF, 00498 PACAP_2450_fF, 00499 PACAP_2625_fF, 00500 PACAP_2800_fF, 00501 PACAP_2975_fF, 00502 PACAP_3150_fF, 00503 PACAP_3325_fF, 00504 PACAP_3500_fF, 00505 PACAP_3675_fF, 00506 PACAP_3850_fF, 00507 PACAP_4025_fF, 00508 PACAP_4200_fF, 00509 PACAP_4375_fF, 00510 PACAP_4550_fF, 00511 PACAP_4725_fF, 00512 PACAP_4900_fF, 00513 PACAP_5075_fF, 00514 PACAP_5250_fF, 00515 PACAP_5425_fF, 00516 } pacap_t; 00517 00518 /** 00519 * @brief set shunt capacitance value 00520 * 00521 * @param[in] pacap shunt capacitance value 00522 * 00523 * @returns 0 on success, negative error code on failure. 00524 */ 00525 int set_pacap(pacap_t pacap); 00526 00527 /** 00528 * @brief Gets shunt capacitance value 00529 * 00530 * @param[in] pacap shunt capacitance value 00531 * 00532 * @returns 0 on success, negative error code on failure. 00533 */ 00534 int get_pacap(pacap_t* pacap); 00535 00536 /** 00537 * @brief Register Configuration 00538 * 00539 * @details 00540 * - Register : PLL1(0x08) 00541 * - Bit Fields : [7:6] 00542 * -Default : 0x1 00543 * - Description : Sets the level of charge pump offset current 00544 * for fractional N mode to improve close in 00545 * phase noise. Set to 'DISABLED' for integer N mode. 00546 */ 00547 typedef enum { 00548 CPLIN_NO_EXTRA_CURRENT, /**< 0x0: No extra current */ 00549 CPLIN_CHARGE_PUMP_CURRENT_5_PERCENT, /**< 0x1: 5% of charge pump current */ 00550 CPLIN_CHARGE_PUMP_CURRENT_10_PERCENT, /**< 0x2: 10% of charge pump current */ 00551 CPLIN_CHARGE_PUMP_CURRENT_15_PERCENT, /**< 0x3: 15% of charge pump current */ 00552 } cplin_t; 00553 00554 /** 00555 * @brief set level of charge pump offset current 00556 * 00557 * @param[in] cplin percentage of charge pump current 00558 * 00559 * @returns 0 on success, negative error code on failure. 00560 */ 00561 int set_cplin(cplin_t cplin); 00562 00563 /** 00564 * @brief Gets level of charge pump offset current 00565 * 00566 * @param[in] cplin percentage of charge pump current 00567 * 00568 * @returns 0 on success, negative error code on failure. 00569 */ 00570 int get_cplin(cplin_t* cplin); 00571 00572 00573 /** 00574 * @brief Register Configuration 00575 * 00576 * @details 00577 * - Register : PLL1(0x08) 00578 * - Bit Fields : [5] 00579 * - Default : 0b1 00580 * - Description : Sets PLL between fractional-N and integer-N mode. 00581 * 00582 */ 00583 typedef enum { 00584 FRACMODE_INTEGER_N, /**< 0x0: Integer N Mode */ 00585 RACMODE_FRACTIONAL_N, /**< 0x1: Fractional N Mode */ 00586 } fracmode_t; 00587 00588 /** 00589 * @brief set PLL mode 00590 * 00591 * @param[in] fracmode Integer N/Fractional N mode 00592 * 00593 * @returns 0 on success, negative error code on failure. 00594 */ 00595 int set_fracmode(fracmode_t fracmode); 00596 00597 /** 00598 * @brief Gets PLL mode 00599 * 00600 * @param[in] fracmode Integer N/Fractional N mode 00601 * 00602 * @returns 0 on success, negative error code on failure. 00603 */ 00604 int get_fracmode(fracmode_t* fracmode); 00605 00606 /* Register Configuration 00607 * 00608 * @Register : PLL1(0x08) 00609 * @Bit Fields : [2:1] 00610 * @Default : 0x0 00611 * @Description : 00612 */ 00613 typedef enum { 00614 LODIV_DISABLED, /**< 0x0: Disabled */ 00615 LODIV_DIVIDE_BY_4, /**< 0x1: LC VCO divided by 4 */ 00616 LODIV_DIVIDE_BY_8, /**< 0x2: LC VCO divided by 8 */ 00617 LODIV_DIVIDE_BY_12, /**< 0x3: LC VCO divided by 12 */ 00618 } lodiv_t ; 00619 00620 /** 00621 * @brief set divider of LC VCO 00622 * 00623 * @param[in] lodiv divider value 00624 * 00625 * @returns 0 on success, negative error code on failure. 00626 */ 00627 int set_lodiv(lodiv_t lodiv); 00628 00629 /** 00630 * @brief Gets divider of LC VCO 00631 * 00632 * @param[in] lodiv divider value 00633 * 00634 * @returns 0 on success, negative error code on failure. 00635 */ 00636 int get_lodiv(lodiv_t * lodiv); 00637 00638 /** 00639 * @brief Register Configuration 00640 * 00641 * @details 00642 * - Register : PLL1(0x08) 00643 * - Bit Fields : [0] 00644 * - Default : 0b0 00645 * - Description : Sets LO generation. For lower power, choose LOWCURRENT. 00646 * For higher performance, choose LOWNOISE 00647 */ 00648 typedef enum { 00649 LOMODE_RING_OSCILLATOR, /**< 0x0: Ring Oscillator Mode */ 00650 LOMODE_LC_VCO, /**< 0x1: LC VCO Mode */ 00651 } lomode_t; 00652 00653 /** 00654 * @brief set LO generation 00655 * 00656 * @param[in] lomode selection of mode 00657 * 00658 * @returns 0 on success, negative error code on failure. 00659 */ 00660 int set_lomode(lomode_t lomode); 00661 00662 /** 00663 * @brief Gets LO generation 00664 * 00665 * @param[in] lomode selection of mode 00666 * 00667 * @returns 0 on success, negative error code on failure. 00668 */ 00669 int get_lomode(lomode_t* lomode); 00670 00671 /** 00672 * @brief Register Configuration 00673 * 00674 * @details 00675 * - Register : PLL2(0x09) 00676 * - Bit Fields : [1:0] 00677 * - Default : 0x0 00678 * - Description : Sets Charge Pump Current in microAmpere 00679 * 00680 */ 00681 typedef enum { 00682 CPVAL_5_UA, /**< 0x0: 5�A */ 00683 CPVAL_10_UA, /**< 0x1: 10�A */ 00684 CPVAL_15_UA, /**< 0x2: 15�A */ 00685 CPVAL_20_UA, /**< 0x3: 20�A */ 00686 } cpval_t; 00687 00688 /** 00689 * @brief set charge pump current 00690 * 00691 * @param[in] cpval current value 00692 * 00693 * @returns 0 on success, negative error code on failure. 00694 */ 00695 int set_cpval(cpval_t cpval); 00696 00697 /** 00698 * @brief Gets charge pump current 00699 * 00700 * @param[in] cpval current value 00701 * 00702 * @returns 0 on success, negative error code on failure. 00703 */ 00704 int get_cpval(cpval_t* cpval); 00705 00706 /** 00707 * Register Configuration 00708 * 00709 * @details 00710 * - Register : CFG6(0x0A) 00711 * - Bit Fields : [2] 00712 * - Default : 0b0 00713 * - Description : Enables DATA transmission in I2C mode. Aliased address for I2C_TXEN1. 00714 */ 00715 typedef enum { 00716 I2C_TXEN1_DISABLE, /**< 0x0: Data transmission not enabled in I2C mode. */ 00717 I2C_TXEN1_ENABLE, /**< 0x1: Data transmission enabled in I2C mode. */ 00718 } i2c_txen1_t; 00719 00720 int set_i2c_txen1(i2c_txen1_t setting); //sdivarci 00721 00722 /** Register Configuration 00723 * 00724 * @details 00725 * - Register : CFG6(0x0A) 00726 * - Bit Fields : [1] 00727 * - Default : 0b0 00728 * - Description : Transmission enable. 00729 */ 00730 typedef enum { 00731 SPI_TXEN1_DISABLE, /**< 0x0: Transmission disabled. */ 00732 SPI_TXEN1_ENABLE, /**< 0x1: Transmission enabled. */ 00733 } spi_txen1_t; 00734 00735 /** 00736 * @brief Register Configuration 00737 * 00738 * @details 00739 * - Register : CFG6(0x0A) 00740 * - Bit Fields : [0] 00741 * - Default : 0b0 00742 * - Description : Four wire readback on CLKOUT pin mode. 00743 * 00744 */ 00745 typedef enum { 00746 FOURWIRE1_READBACK_DISABLE, /**< 0x0: Four wire readback disabled. */ 00747 FOURWIRE1_READBACK_ENABLE, /**< 0x1: Four wire readback enabled. */ 00748 } fourwire1_t; 00749 00750 00751 /** 00752 * @brief Register Configuration 00753 * 00754 * @details 00755 * - Register : CFG7(0x10) 00756 * - Bit Fields : [2] 00757 * - Default : 0b0 00758 * - Description : Enables DATA transmission in I2C mode. Aliased address for I2C_TXEN1 00759 */ 00760 typedef enum { 00761 I2C_TXEN2_DISABLE, /**< 0x0: Data transmission not enabled in I2C mode. */ 00762 I2C_TXEN2_ENABLE, /**< 0x1: Data transmission enabled in I2C mode. */ 00763 } i2c_txen2_t; 00764 00765 int set_i2c_txen2(i2c_txen2_t setting); //sdivarci 00766 /** 00767 * @brief Register Configuration 00768 * 00769 * @details 00770 * - Register : CFG7(0x10) 00771 * - Bit Fields : [1] 00772 * - Default : 0b0 00773 * - Description : Transmission enable. 00774 */ 00775 typedef enum { 00776 SPI_TXEN2_DISABLE, /**< 0x0: Transmission disabled. */ 00777 SPI_TXEN2_ENABLE, /**< 0x1: Transmission enabled. */ 00778 } spi_txen2_t; 00779 00780 00781 /** 00782 * @brief Register Configuration 00783 * 00784 * @details 00785 * - Register : CFG7(0x10) 00786 * - Bit Fields : [0] 00787 * - Default : 0b0 00788 * - Description : Four wire readback on CLKOUT pin mode. Aliased address for FOURWIRE1 00789 */ 00790 typedef enum { 00791 FOURWIRE2_READBACK_DISABLE, /**< 0x0: Four wire readback disabled. */ 00792 FOURWIRE2_READBACK_ENABLE, /**< 0x1: Four wire readback enabled. */ 00793 } fourwire2_t; 00794 00795 00796 00797 /** 00798 * @brief Register Configuration 00799 * 00800 * @details 00801 * - Register : I2C1(0x11) 00802 * - Bit Fields : [7] 00803 * - Default : 0b0 00804 * - Description : Packet Length Mode 00805 * 00806 */ 00807 typedef enum { 00808 PKTLEN_MODE_SET_LENGTH, /**< 0x0: PKTLEN[14:0] need not be programmed. FIFO underflow event will 00809 be treated as end of packet event. For cases where actual packet length 00810 is greater than 32767 bits, it is expected that the �C will pad 00811 such a packet to make it an integral multiple of 8-bits */ 00812 PKTLEN_MODE_NO_SET_LENGTH, /**< 0x1: PKTLEN[14:0] will provide the length of packet. Once FIFO is 00813 read for PKTLEN[14:0] bits, or if FIFO underflow, MAX4146x will consider 00814 that as an end of packet event. */ 00815 } pktlen_mode_t; 00816 00817 /** 00818 * @brief set packet length 00819 * 00820 * @param[in] pktlen_mode packet length mode 00821 * 00822 * @returns 0 on success, negative error code on failure. 00823 */ 00824 int set_pktlen_mode(pktlen_mode_t pktlen_mode); 00825 00826 /** 00827 * @brief Gets packet length 00828 * 00829 * @param[in] pktlen_mode packet length mode 00830 * 00831 * @returns 0 on success, negative error code on failure. 00832 */ 00833 int get_pktlen_mode(pktlen_mode_t* pktlen_mode); 00834 00835 00836 /** 00837 * @brief Register Configuration 00838 * 00839 * @details 00840 * - Register : CFG8(0x17) 00841 * - Bit Fields : [0] 00842 * - Default : 0b0 00843 * - Description : Places DUT into software reset. 00844 */ 00845 typedef enum { 00846 SOFTRESET_DEASSERT, /**< 0x0: Deassert the reset */ 00847 SOFTRESET_RESET, /**< 0x1: Resets the entire digital, until this bit is set to 0 */ 00848 } softreset_t; 00849 00850 /** 00851 * @brief set softreset bit 00852 * 00853 * @param[in] softreset enable/disable 00854 * 00855 * @returns 0 on success, negative error code on failure. 00856 */ 00857 int set_softreset(softreset_t softreset); 00858 00859 /** 00860 * @brief Operation state of the rf transmitter 00861 * 00862 * @details 00863 * - Default : 0b0 00864 * - Description : Places DUT into software reset. 00865 */ 00866 typedef enum { 00867 INITIALIZED = 0, 00868 UNINITIALIZED = 1, 00869 UNKNOWN = 2, 00870 } operation_mode_t; 00871 00872 00873 // Indicates whether initialization is successful 00874 operation_mode_t operation_mode; 00875 00876 /** 00877 * @brief get fifo flags (I2C6) Read only 00878 * 00879 * @param[in] fifo_flags 8-bit fifo flags 00880 * 00881 * @returns 0 on success, negative error code on failure. 00882 */ 00883 int get_fifo_flags(uint8_t *fifo_flags); 00884 00885 /** 00886 * @brief get pktcomplete bit field flags (I2C4) Read only 00887 * 00888 * @param[in] pktcomplete 0x0: Packet transmission is not completed 00889 * 0x1: Packet transmission is completed 00890 * 00891 * @returns 0 on success, negative error code on failure. 00892 */ 00893 int get_pktcomplete(uint8_t *pktcomplete); 00894 00895 /** 00896 * @brief get packet length of transmitted packet 00897 * 00898 * @param[in] pktlen Provides status information of bits transmitted for the current packet 00899 * 00900 * @returns 0 on success, negative error code on failure. 00901 */ 00902 int get_tx_pktlen(uint16_t *pktlen); 00903 00904 00905 /* PUBLIC FUNCTION DECLARATIONS */ 00906 00907 /** 00908 * @brief Read from a register. 00909 * 00910 * @param[in] reg Address of a register to be read. 00911 * @param[out] value Pointer to save result value. 00912 * @param[in] len Size of result to be read. 00913 * 00914 * @returns 0 on success, negative error code on failure. 00915 */ 00916 int read_register(uint8_t reg, uint8_t *value, uint8_t len); 00917 00918 /** 00919 * @brief Write to a register. 00920 * 00921 * @param[in] reg Address of a register to be written. 00922 * @param[out] value Pointer of value to be written to register. 00923 * @param[in] len Size of result to be written. 00924 * 00925 * @returns 0 on success, negative error code on failure. 00926 */ 00927 int write_register(uint8_t reg, const uint8_t *value, uint8_t len); 00928 00929 /** 00930 * @brief Initial programming steps after power on or soft reset. 00931 * 00932 * @returns 0 on success, negative error code on failure. 00933 */ 00934 int initial_programming(void); 00935 00936 /** 00937 * @brief Set Baud clock predivision ratio 00938 * 00939 * @param[in] prediv values between 3 and 255 00940 * 00941 * @returns 0 on success, negative error code on failure. 00942 */ 00943 int set_bclk_prediv(uint8_t prediv); 00944 00945 /** 00946 * @brief Gets Baud clock predivision ratio 00947 * 00948 * @param[in] prediv values between 3 and 255 00949 * 00950 * @returns 0 on success, negative error code on failure. 00951 */ 00952 int get_bclk_prediv(uint8_t* prediv); 00953 00954 /** 00955 * @brief Controls GFSK shaping 00956 * 00957 * @param[in] tstep values between 0 and 31 00958 * 00959 * @returns 0 on success, negative error code on failure. 00960 */ 00961 int set_tstep(uint8_t tstep); 00962 00963 /** 00964 * @brief Gets tstep 00965 * 00966 * @param[in] tstep values between 0 and 31 00967 * 00968 * @returns 0 on success, negative error code on failure. 00969 */ 00970 int get_tstep(uint8_t* tstep); 00971 00972 /** 00973 * @brief Set PLL frequency 00974 * 00975 * @param[in] freq FREQ value to PLL between 0x00 and 0xFFFFFF 00976 * 00977 * @returns 0 on success, negative error code on failure. 00978 * 00979 * @description LO frequency = FREQ<23:0>/2^16*fXTAL 00980 */ 00981 int set_frequency(uint32_t freq); 00982 00983 /** 00984 * @brief Gets PLL frequency 00985 * 00986 * @param[in] freq FREQ value to PLL between 0x00 and 0xFFFFFF 00987 * 00988 * @returns 0 on success, negative error code on failure. 00989 * 00990 * @description LO frequency = FREQ<23:0>/2^16*fXTAL 00991 */ 00992 int get_frequency(uint32_t* freq); 00993 00994 /** 00995 * @brief Set frequency deviation from the space frequency for the mark frequency 00996 * 00997 * @param[in] deltaf frequency deviation value 00998 * 00999 * @returns 0 on success, negative error code on failure. 01000 * 01001 * @description fDELTA = DELTAF[6:0]* fXTAL/8192 01002 */ 01003 int set_deltaf(uint8_t deltaf); 01004 01005 /** 01006 * @brief Get frequency deviation from the space frequency for the mark frequency 01007 * 01008 * @param[in] deltaf frequency deviation value 01009 * 01010 * @returns 0 on success, negative error code on failure. 01011 * 01012 * @description fDELTA = DELTAF[6:0]* fXTAL/8192 01013 */ 01014 int get_deltaf(uint8_t* deltaf); 01015 01016 /** 01017 * @brief Set frequency deviation from the space frequency for the mark frequency 01018 * 01019 * @param[in] deltaf_shape frequency deviation value 01020 * 01021 * @returns 0 on success, negative error code on failure. 01022 * 01023 * @description fDELTA = DELTAF_SHAPE[3:0]* fXTAL/81920 01024 */ 01025 int set_deltaf_shape(uint8_t deltaf_shape); 01026 01027 /** 01028 * @brief Gets frequency deviation from the space frequency for the mark frequency 01029 * 01030 * @param[in] deltaf_shape frequency deviation value 01031 * 01032 * @returns 0 on success, negative error code on failure. 01033 * 01034 * @description fDELTA = DELTAF_SHAPE[3:0]* fXTAL/81920 01035 */ 01036 int get_deltaf_shape(uint8_t* deltaf_shape); 01037 01038 /** 01039 * @brief Set Packet Length for I2C communication 01040 * 01041 * @param[in] pktlen values between 0x00 and 0x7FF 01042 * 01043 * @returns 0 on success, negative error code on failure. 01044 */ 01045 int set_i2c_pktlen(uint16_t pktlen); 01046 01047 /** 01048 * @brief Gets Packet Length for I2C communication 01049 * 01050 * @param[in] pktlen values between 0x00 and 0x7FF 01051 * 01052 * @returns 0 on success, negative error code on failure. 01053 */ 01054 int get_i2c_pktlen(uint16_t* pktlen); 01055 01056 /** 01057 * @brief Adjust baud rate 01058 * 01059 * @param[in] baud_rate preferred baud rate 01060 * 01061 * @returns 0 on success, negative error code on failure. 01062 * 01063 * @description It changes only the values of BCLK_PREDIV and BCLK_POSTDIV 01064 * Baud_rate = f_clk/((1+BCLK_PREDIV)x2^(1+BCLK_POSTDIV)) 01065 * where f_clk = f_xtal/XOCLKDIV_ratio 01066 * Note that to maintain the internal 3.2MHz time base, 01067 * XOCLKDIV[1:0] (register CFG1, 0x00, bit 4) must be programmed, 01068 * based on the crystal frequency 01069 */ 01070 int adjust_baudrate(float rate); 01071 01072 /** 01073 * @brief Gets baud rate 01074 * 01075 * @returns baud rate. 01076 * 01077 */ 01078 float get_baudrate(); 01079 01080 /** 01081 * @brief Adjust Manchester Bitrate 01082 * 01083 * @param[in] data_rate preferred data rate in (1-50)kbps 01084 * 01085 * @returns 0 on success, negative error code on failure. 01086 */ 01087 int adjust_manchester_bitrate(char rate); 01088 01089 /** 01090 * @brief Get Manchester Bitrate 01091 * 01092 * @returns data rate in (1-50)kbps 01093 */ 01094 char get_manchester_bitrate(); 01095 01096 /** 01097 * @brief Configures the crystal frequency of the chip (Fxtal) 01098 * 01099 * @param[in] freq crystal frequency values between 12.8 MHz and 19.2 MHz 01100 * 01101 * @returns 0 on success, negative error code on failure. 01102 */ 01103 int set_crystal_frequency(float freq); 01104 01105 /** 01106 * @brief Get the crystal frequency of the chip (Fxtal) 01107 * 01108 * @returns crystal frequency values between 12.8 MHz and 19.2 MHz 01109 */ 01110 float get_crystal_frequency(); 01111 01112 /** 01113 * @brief Adjust center/carrier frequency of the chip 01114 * 01115 * @param[in] freq center/carrier frequency value between 250 MHz and 950 MHz 01116 * 01117 * @returns 0 on success, negative error code on failure. 01118 */ 01119 int set_center_frequency(float freq); 01120 01121 /** 01122 * @brief Gets center/carrier frequency of the chip 01123 * 01124 * @returns center/carrier frequency value between 250 MHz and 950 MHz 01125 */ 01126 float get_center_frequency(); 01127 01128 /** 01129 * @brief set the FSK deviation values (Delta_f) 01130 * 01131 * @param[in] deviation deviation value in in kHz baud rate 01132 * 01133 * @returns 0 on success, negative error code on failure. 01134 * 01135 * @description The mark frequency is defined by the space frequency 01136 * plus a frequency deviation. If frequency shaping is 01137 * disabled by setting FSKSHAPE = 0 (register CFG1, bit 2), 01138 * the frequency deviation is defined by DELTAF[6:0] 01139 * (register PLL6, bits 6:0). 01140 * DELTAF[6 : 0] = (Delta_f * 8192)f_xtal 01141 * If frequency shaping is enabled by setting FSKSHAPE = 1 01142 * (register CFG1, bit 2), the frequency deviation is defined 01143 * by DETLAF_SHAPE[3:0] (register PLL7, bits 3:0). 01144 * DELTAF_SHAPE[3 : 0] = (Delta_f * 8192)/(f_xtal * 10) 01145 */ 01146 int adjust_frequency_deviation(float deviation); 01147 01148 /** 01149 * @brief Send data for selected Preset/I2C/SPI mode 01150 * 01151 * @param[in] data data pointer for data to be transferred 01152 * @param[in] length legth of data to be transferred 01153 * 01154 * @returns 0 on success, negative error code on failure. 01155 */ 01156 int send_data(uint8_t *data, uint32_t length); 01157 01158 int set_i2c_data(uint8_t setting); //sdivarci 01159 }; 01160 01161 01162 /** MAX41460 Device Class 01163 * 01164 * Hold configurations for the MAX41460 01165 */ 01166 class MAX41460 : public MAX4146X <max41460_reg_map_t> 01167 { 01168 max41460_reg_map_t regmap; 01169 public: 01170 MAX41460(SPI *spi, DigitalOut *cs) : MAX4146X<max41460_reg_map_t> (®map, spi, cs) {} 01171 01172 MAX41460(SPI *spi) : MAX4146X<max41460_reg_map_t> (®map, spi) {} 01173 01174 MAX41460(DigitalOut *cs) : MAX4146X(cs) {} 01175 01176 }; 01177 01178 /** MAX41461 Device Class 01179 * 01180 * Hold configurations for the MAX41461 01181 */ 01182 class MAX41461 : public MAX4146X <max41461_2_reg_map_t> 01183 { 01184 max41461_2_reg_map_t regmap; 01185 public: 01186 MAX41461(I2C *i2c) : MAX4146X<max41461_2_reg_map_t> (®map, i2c) {} 01187 01188 MAX41461(DigitalOut *cs) : MAX4146X(cs) {} 01189 }; 01190 01191 /** MAX41462 Device Class 01192 * 01193 * Hold configurations for the MAX41462 01194 */ 01195 class MAX41462 : public MAX41461 01196 { 01197 public: 01198 MAX41462(I2C *i2c) : MAX41461(i2c) {} 01199 01200 MAX41462(DigitalOut *cs) : MAX41461(cs) {} 01201 01202 }; 01203 01204 /** MAX41463 Device Class 01205 * 01206 * Hold configurations for the MAX41463 01207 */ 01208 class MAX41463 : public MAX4146X <max41463_4_reg_map_t> 01209 { 01210 max41463_4_reg_map_t regmap; 01211 public: 01212 MAX41463(I2C *i2c) : MAX4146X<max41463_4_reg_map_t> (®map, i2c) {} 01213 01214 MAX41463(DigitalOut *cs) : MAX4146X(cs) {} 01215 01216 }; 01217 01218 /** MAX41464 Device Class 01219 * 01220 * Hold configurations for the MAX41464 01221 */ 01222 class MAX41464 : public MAX41463 01223 { 01224 public: 01225 MAX41464(I2C *i2c) : MAX41463(i2c) {} 01226 01227 MAX41464(DigitalOut *cs) : MAX41463(cs) {} 01228 01229 }; 01230 01231 01232 #endif /* MAX4146x_H_ */
Generated on Tue Jul 12 2022 16:06:46 by
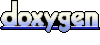