Utility functions for working with data streams
checksum.cpp File Reference
Utility Function - Data integrity helpers. More...
Go to the source code of this file.
Functions | |
bool | validateChecksum (uint8_t const *pkt, uint32_t const length) |
Helpers for working data streams. | |
void | calculateChecksum (uint8_t *pkt, uint32_t const length) |
Calculate and store the checksum into the last position of a data stream. |
Detailed Description
Utility Function - Data integrity helpers.
- Version:
- 1.0
Copyright (c) 2013
Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with the License. You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions and limitations under the License.
Definition in file checksum.cpp.
Function Documentation
void calculateChecksum | ( | uint8_t * | pkt, |
uint32_t const | length | ||
) |
Calculate and store the checksum into the last position of a data stream.
- Parameters:
-
pkt - A pointer to the data length - The amount of data that the checksum calculates
Definition at line 37 of file checksum.cpp.
bool validateChecksum | ( | uint8_t const * | pkt, |
uint32_t const | length | ||
) |
Helpers for working data streams.
Example:
#include "mbed.h" #include "checksum.h" DigitalOut myled(LED1); template <int T> struct data{ uint8_t buf[T]; uint8_t checksum; }; data<12> all_ones = {{1,1,1,1,1,1,1,1,1,1,1,1}}; int main() { calculateChecksum( all_ones.buf, sizeof(all_ones) ); for(int i=0; i<sizeof(all_ones); i++) { printf("%02d: %d\n", i, *(all_ones.buf+i)); } printf("checksum test: %s\n", (result)?"passed":"failed"); while(1) { myled = 1; wait(0.2); myled = 0; wait(0.2); } }
Calculate the checksum of a data stream Assumes the checksum is in the last position, 8-bits and 0 - the sum of data
- Parameters:
-
pkt - A pointer to the data length - The amount of data that the checksum calculates
- Returns:
- true if the checksum is correct and false otherwise
Definition at line 24 of file checksum.cpp.
Generated on Wed Jul 13 2022 03:02:51 by
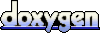