Utility functions for working with data streams
Embed:
(wiki syntax)
Show/hide line numbers
checksum.cpp
Go to the documentation of this file.
00001 /** 00002 * @file checksum.cpp 00003 * @brief Utility Function - Data integrity helpers 00004 * @author sam grove 00005 * @version 1.0 00006 * 00007 * Copyright (c) 2013 00008 * 00009 * Licensed under the Apache License, Version 2.0 (the "License"); 00010 * you may not use this file except in compliance with the License. 00011 * You may obtain a copy of the License at 00012 * 00013 * http://www.apache.org/licenses/LICENSE-2.0 00014 * 00015 * Unless required by applicable law or agreed to in writing, software 00016 * distributed under the License is distributed on an "AS IS" BASIS, 00017 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00018 * See the License for the specific language governing permissions and 00019 * limitations under the License. 00020 */ 00021 00022 #include "checksum.h" 00023 00024 bool validateChecksum( uint8_t const *pkt, uint32_t const length ) 00025 { 00026 uint32_t pos = 0; 00027 uint8_t sum = 0; 00028 while ( pos < (length-1) ) 00029 { 00030 sum += *(pkt+pos++); 00031 } 00032 sum = 0x0 - sum; 00033 // return 0 or 1 based on the checksum test 00034 return (sum == *(pkt+pos)) ? 1 : 0; 00035 } 00036 00037 void calculateChecksum( uint8_t *pkt, uint32_t const length ) 00038 { 00039 uint32_t pos = 0; 00040 uint8_t sum = 0; 00041 while ( pos < (length-1) ) 00042 { 00043 sum += *(pkt+pos++); 00044 } 00045 // put the checksum into the data stream 00046 *(pkt+pos) = 0x0 - sum; 00047 00048 return; 00049 } 00050
Generated on Wed Jul 13 2022 03:02:51 by
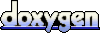