
CANfestival - an open source CANopen framework
Embed:
(wiki syntax)
Show/hide line numbers
nmtSlave.c
Go to the documentation of this file.
00001 /* 00002 This file is part of CanFestival, a library implementing CanOpen 00003 Stack. 00004 00005 Copyright (C): Edouard TISSERANT and Francis DUPIN 00006 00007 See COPYING file for copyrights details. 00008 00009 This library is free software; you can redistribute it and/or 00010 modify it under the terms of the GNU Lesser General Public 00011 License as published by the Free Software Foundation; either 00012 version 2.1 of the License, or (at your option) any later version. 00013 00014 This library is distributed in the hope that it will be useful, 00015 but WITHOUT ANY WARRANTY; without even the implied warranty of 00016 MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU 00017 Lesser General Public License for more details. 00018 00019 You should have received a copy of the GNU Lesser General Public 00020 License along with this library; if not, write to the Free Software 00021 Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 00022 USA 00023 */ 00024 /*! 00025 ** @file nmtSlave.c 00026 ** @author Edouard TISSERANT and Francis DUPIN 00027 ** @date Tue Jun 5 08:50:53 2007 00028 ** 00029 ** @brief 00030 ** 00031 ** 00032 */ 00033 #include "nmtSlave.h" 00034 #include "states.h" 00035 #include "canfestival.h" 00036 #include "sysdep.h" 00037 00038 /*! 00039 ** put the slave in the state wanted by the master 00040 ** 00041 ** @param d 00042 ** @param m 00043 **/ 00044 void proceedNMTstateChange(CO_Data* d, Message *m) 00045 { 00046 if( d->nodeState == Pre_operational || 00047 d->nodeState == Operational || 00048 d->nodeState == Stopped ) { 00049 00050 MSG_WAR(0x3400, "NMT received. for node : ", (*m).data[1]); 00051 00052 /* Check if this NMT-message is for this node */ 00053 /* byte 1 = 0 : all the nodes are concerned (broadcast) */ 00054 00055 if( ( (*m).data[1] == 0 ) || ( (*m).data[1] == *d->bDeviceNodeId ) ){ 00056 00057 switch( (*m).data[0]){ /* command specifier (cs) */ 00058 case NMT_Start_Node: 00059 if ( (d->nodeState == Pre_operational) || (d->nodeState == Stopped) ) 00060 setState(d,Operational); 00061 break; 00062 00063 case NMT_Stop_Node: 00064 if ( d->nodeState == Pre_operational || 00065 d->nodeState == Operational ) 00066 setState(d,Stopped); 00067 break; 00068 00069 case NMT_Enter_PreOperational: 00070 if ( d->nodeState == Operational || 00071 d->nodeState == Stopped ) 00072 setState(d,Pre_operational); 00073 break; 00074 00075 case NMT_Reset_Node: 00076 if(d->NMT_Slave_Node_Reset_Callback != NULL) 00077 d->NMT_Slave_Node_Reset_Callback(d); 00078 setState(d,Initialisation); 00079 break; 00080 00081 case NMT_Reset_Comunication: 00082 { 00083 UNS8 currentNodeId = getNodeId(d); 00084 00085 if(d->NMT_Slave_Communications_Reset_Callback != NULL) 00086 d->NMT_Slave_Communications_Reset_Callback(d); 00087 #ifdef CO_ENABLE_LSS 00088 // LSS changes NodeId here in case lss_transfer.nodeID doesn't 00089 // match current getNodeId() 00090 if(currentNodeId!=d->lss_transfer.nodeID) 00091 currentNodeId = d->lss_transfer.nodeID; 00092 #endif 00093 00094 // clear old NodeId to make SetNodeId reinitializing 00095 // SDO, EMCY and other COB Ids 00096 *d->bDeviceNodeId = 0xFF; 00097 00098 setNodeId(d, currentNodeId); 00099 } 00100 setState(d,Initialisation); 00101 break; 00102 00103 }/* end switch */ 00104 00105 }/* end if( ( (*m).data[1] == 0 ) || ( (*m).data[1] == 00106 bDeviceNodeId ) ) */ 00107 } 00108 } 00109 00110 00111 /*! 00112 ** 00113 ** 00114 ** @param d 00115 ** 00116 ** @return 00117 **/ 00118 UNS8 slaveSendBootUp(CO_Data* d) 00119 { 00120 Message m; 00121 00122 #ifdef CO_ENABLE_LSS 00123 if(*d->bDeviceNodeId==0xFF)return 0; 00124 #endif 00125 00126 MSG_WAR(0x3407, "Send a Boot-Up msg ", 0); 00127 00128 /* message configuration */ 00129 { 00130 UNS16 tmp = NODE_GUARD << 7 | *d->bDeviceNodeId; 00131 m.cob_id = UNS16_LE(tmp); 00132 } 00133 m.rtr = NOT_A_REQUEST; 00134 m.len = 1; 00135 m.data[0] = 0x00; 00136 00137 return canSend(d->canHandle,&m); 00138 } 00139
Generated on Tue Jul 12 2022 17:11:51 by
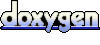