Utility to manage ASCII communications. Register a header and event and then pass messages into the class and events are generated on a match
CommHandler Class Reference
Example using the CommHandler class. More...
#include <CommHandler.h>
Public Member Functions | |
CommHandler () | |
Create the CommHandler object. | |
template<class T > | |
void | attachMsg (char *string, T *item, char *(T::*method)(char *)) |
Attach a member function as the match handler. | |
void | attachMsg (char *string, char *(*function)(char *)) |
Attach a global function as the match handler. | |
char * | serviceMessage (char *buffer) |
called in a loop somewhere to processes messages | |
char * | messageLookup (uint32_t const loc) |
Determine what a message in location X is looking to match. |
Detailed Description
Example using the CommHandler class.
#include "mbed.h" #include "CommHandler.h" DigitalOut myled(LED1); CommHandler msgs; char *one(char* msg) { // you can parse msg here LOG("\n"); return msg; } class Wrap { public: Wrap(){} char *two(char *msg) { // you can parse msg here LOG("\n"); return msg; } }obj; char *three(char* msg) { // you can parse msg here LOG("\n"); return msg; } int main() { char *tmp = 0; msgs.attachMsg("One", &one); msgs.attachMsg("Two", &obj, &Wrap::two); msgs.attachMsg("Three", &three); tmp = msgs.messageLookup(0); printf("0:%s\n", tmp); tmp = msgs.messageLookup(1); printf("1:%s\n", tmp); tmp = msgs.messageLookup(2); printf("2:%s\n", tmp); tmp = msgs.messageLookup(3); printf("3:%s\n", tmp); tmp = msgs.messageLookup(4); printf("4:%s\n", tmp); tmp = msgs.serviceMessage("Two-00-66-99-20133"); printf("1: Found: %s\n", tmp); tmp = msgs.serviceMessage("One-99-60-1-339788354"); printf("2: Found: %s\n", tmp); tmp = msgs.serviceMessage("Three-xx-xx-XX-XXXXXXX"); printf("3: Found: %s\n", tmp); error("End of Test\n"); }
API abstraction for managing device to device communication
Definition at line 100 of file CommHandler.h.
Constructor & Destructor Documentation
CommHandler | ( | void | ) |
Create the CommHandler object.
Definition at line 26 of file CommHandler.cpp.
Member Function Documentation
void attachMsg | ( | char * | string, |
T * | item, | ||
char *(T::*)(char *) | method | ||
) |
Attach a member function as the match handler.
- Parameters:
-
string - The string that we're trying to match item - Address of a initialized object member - Address of the initialized object's member function
Definition at line 127 of file CommHandler.h.
void attachMsg | ( | char * | string, |
char *(*)(char *) | function | ||
) |
Attach a global function as the match handler.
- Parameters:
-
string - The string that we're trying to match function - Address of the global function
Definition at line 31 of file CommHandler.cpp.
char * messageLookup | ( | uint32_t const | loc ) |
Determine what a message in location X is looking to match.
- Parameters:
-
loc - The location of the member in the list
- Returns:
- The message that is attached to the list
Definition at line 68 of file CommHandler.cpp.
char * serviceMessage | ( | char * | buffer ) |
called in a loop somewhere to processes messages
- Parameters:
-
buffer - A buffer containing ascii data
- Returns:
- Data from the handler message when a match is found and 0 otherwise
Definition at line 48 of file CommHandler.cpp.
Generated on Sat Jul 16 2022 11:55:00 by
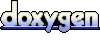