Utility to manage ASCII communications. Register a header and event and then pass messages into the class and events are generated on a match
CommHandler.cpp
00001 /** 00002 * @file CommHandler.cpp 00003 * @brief Core Utility - Manage ASCII communication between devices 00004 * @author sam grove 00005 * @version 1.0 00006 * @see 00007 * 00008 * Copyright (c) 2013 00009 * 00010 * Licensed under the Apache License, Version 2.0 (the "License"); 00011 * you may not use this file except in compliance with the License. 00012 * You may obtain a copy of the License at 00013 * 00014 * http://www.apache.org/licenses/LICENSE-2.0 00015 * 00016 * Unless required by applicable law or agreed to in writing, software 00017 * distributed under the License is distributed on an "AS IS" BASIS, 00018 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00019 * See the License for the specific language governing permissions and 00020 * limitations under the License. 00021 */ 00022 00023 #include "CommHandler.h" 00024 #include <string.h> 00025 00026 CommHandler::CommHandler( void ) 00027 { 00028 return; 00029 } 00030 00031 void CommHandler::attachMsg( char *string, char *(*function)(char *) ) 00032 { 00033 MsgObj *new_node = new MsgObj [1]; 00034 // make sure the new object was allocated 00035 if (0 == new_node) 00036 { 00037 ERROR("Memory Allocation Failed\n"); 00038 } 00039 // store the user parameters 00040 new_node->string = string; 00041 new_node->handler.attach( function ); 00042 // and insert them into the list 00043 _list.append(new_node); 00044 00045 return; 00046 } 00047 00048 char *CommHandler::serviceMessage( char* buffer ) 00049 { 00050 uint32_t cnt = _list.length(); 00051 node *item; 00052 MsgObj *tmp_data; 00053 00054 for(uint32_t i=1; i<=cnt; ++i) 00055 { 00056 item = _list.pop(i); 00057 tmp_data = (MsgObj *)item->data; 00058 // Test the input against the stored record 00059 if( 0 == memcmp( buffer, tmp_data->string, strlen(tmp_data->string) ) ) 00060 { 00061 return tmp_data->handler( buffer ); 00062 } 00063 } 00064 00065 return 0; 00066 } 00067 00068 char *CommHandler::messageLookup( uint32_t const loc ) 00069 { 00070 node *tmp_item = _list.pop( loc ); 00071 00072 if(0 == tmp_item) 00073 { 00074 return 0; 00075 } 00076 00077 MsgObj *tmp_data = (MsgObj *)tmp_item->data; 00078 00079 return tmp_data->string; 00080 } 00081
Generated on Sat Jul 16 2022 11:55:00 by
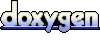