Utility to manage ASCII communications. Register a header and event and then pass messages into the class and events are generated on a match
CommHandler.h
00001 /** 00002 * @file CommHandler.h 00003 * @brief Core Utility - Manage ASCII communication between devices 00004 * @author sam grove 00005 * @version 1.0 00006 * @see 00007 * 00008 * Copyright (c) 2013 00009 * 00010 * Licensed under the Apache License, Version 2.0 (the "License"); 00011 * you may not use this file except in compliance with the License. 00012 * You may obtain a copy of the License at 00013 * 00014 * http://www.apache.org/licenses/LICENSE-2.0 00015 * 00016 * Unless required by applicable law or agreed to in writing, software 00017 * distributed under the License is distributed on an "AS IS" BASIS, 00018 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00019 * See the License for the specific language governing permissions and 00020 * limitations under the License. 00021 */ 00022 00023 #ifndef COMMHANDLER_H 00024 #define COMMHANDLER_H 00025 00026 #include "FP.h" 00027 #include "LinkedList.h" 00028 #include "LogUtil.h" 00029 00030 /** Example using the CommHandler class 00031 * @code 00032 * #include "mbed.h" 00033 * #include "CommHandler.h" 00034 * 00035 * DigitalOut myled(LED1); 00036 * CommHandler msgs; 00037 * 00038 * char *one(char* msg) 00039 * { 00040 * // you can parse msg here 00041 * LOG("\n"); 00042 * return msg; 00043 * } 00044 * 00045 * class Wrap 00046 * { 00047 * public: 00048 * Wrap(){} 00049 * char *two(char *msg) 00050 * { 00051 * // you can parse msg here 00052 * LOG("\n"); 00053 * return msg; 00054 * } 00055 * }obj; 00056 * 00057 * 00058 * char *three(char* msg) 00059 * { 00060 * // you can parse msg here 00061 * LOG("\n"); 00062 * return msg; 00063 * } 00064 * 00065 * int main() 00066 * { 00067 * char *tmp = 0; 00068 * 00069 * msgs.attachMsg("One", &one); 00070 * msgs.attachMsg("Two", &obj, &Wrap::two); 00071 * msgs.attachMsg("Three", &three); 00072 * 00073 * tmp = msgs.messageLookup(0); 00074 * printf("0:%s\n", tmp); 00075 * tmp = msgs.messageLookup(1); 00076 * printf("1:%s\n", tmp); 00077 * tmp = msgs.messageLookup(2); 00078 * printf("2:%s\n", tmp); 00079 * tmp = msgs.messageLookup(3); 00080 * printf("3:%s\n", tmp); 00081 * tmp = msgs.messageLookup(4); 00082 * printf("4:%s\n", tmp); 00083 * 00084 * tmp = msgs.serviceMessage("Two-00-66-99-20133"); 00085 * printf("1: Found: %s\n", tmp); 00086 * tmp = msgs.serviceMessage("One-99-60-1-339788354"); 00087 * printf("2: Found: %s\n", tmp); 00088 * tmp = msgs.serviceMessage("Three-xx-xx-XX-XXXXXXX"); 00089 * printf("3: Found: %s\n", tmp); 00090 * 00091 * error("End of Test\n"); 00092 * } 00093 * @endcode 00094 */ 00095 00096 /** 00097 * @class CommHandler 00098 * @brief API abstraction for managing device to device communication 00099 */ 00100 class CommHandler 00101 { 00102 private: 00103 LinkedList <node>_list; 00104 00105 public: 00106 00107 /** 00108 * @struct MsgObject 00109 * @brief An object to store in the linked list 00110 */ 00111 struct MsgObj 00112 { 00113 char *string; /*!< The header we are going to match */ 00114 FP<char *, char *>handler; /*!< The function to call when a match is found */ 00115 }; 00116 00117 /** Create the CommHandler object 00118 */ 00119 CommHandler(); 00120 00121 /** Attach a member function as the match handler 00122 * @param string - The string that we're trying to match 00123 * @param item - Address of a initialized object 00124 * @param member - Address of the initialized object's member function 00125 */ 00126 template<class T> 00127 void attachMsg( char *string, T *item, char*(T::*method)(char *) ) 00128 { 00129 MsgObj *new_node = new MsgObj [1]; 00130 // make sure the new object was allocated 00131 if (NULL == new_node) 00132 { 00133 ERROR("Memory Allocation Failed\n"); 00134 } 00135 // store the user parameters 00136 new_node->string = string; 00137 new_node->handler.attach( item, method ); 00138 // and insert them into the list 00139 _list.append(new_node); 00140 return; 00141 } 00142 00143 /** Attach a global function as the match handler 00144 * @param string - The string that we're trying to match 00145 * @param function - Address of the global function 00146 */ 00147 void attachMsg( char *string, char *(*function)(char*) ); 00148 00149 /** called in a loop somewhere to processes messages 00150 * @param buffer - A buffer containing ascii data 00151 * @return Data from the handler message when a match is found and 0 otherwise 00152 */ 00153 char *serviceMessage( char* buffer ); 00154 00155 00156 /** Determine what a message in location X is looking to match 00157 * @param loc - The location of the member in the list 00158 * @return The message that is attached to the list 00159 */ 00160 char *messageLookup( uint32_t const loc ); 00161 00162 }; 00163 00164 #endif 00165
Generated on Sat Jul 16 2022 11:55:00 by
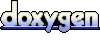