Library for the use of the atmospheric pressure sensor SCP1000
SCP1000 Class Reference
Example: More...
#include <SCP1000.h>
Public Member Functions | |
SCP1000 (PinName mosi, PinName miso, PinName sclk, PinName cs, PinName trig, PinName drdy) | |
Create an SCP1000 interface. | |
int | init (void) |
Initialize the SCP1000. | |
void | setMode (int mode) |
Set the measurement mode of the SCP1000. | |
void | triggerHard (void) |
Trigger the measurement by TRIG wire in Low Power Mode NOTE: IT CURRENTLY SEEMS NOT WORKING. | |
void | triggerSoft (void) |
Trigger the measurement by register writing in Low Power Mode. | |
bool | IsReady (void) |
Check the data readiness. | |
float | readTemperature (void) |
Read temperature measurement result in degrees Celcius. | |
float | readPressure (void) |
Read atmospheric pressure measurement result in hectopascal. |
Detailed Description
Example:
#include "mbed.h" #include "SCP1000.h" SCP1000 scp(p5, p6, p7, p18, p21, p19); int main() { // Variables float temp, press; // SCP1000 Initialization err = scp.init(); if (err == SCP1000::ERR) { printf("Error!"); return 1; } while(true) { do {} while (scp.IsReady() == false); temp = scp.readTemperature(); press = scp.readPressure(); printf("Temperature: %2.2f degC\r\n", temp); printf("Atmospheric Pressure: %2.2f hPa", press); } }
Definition at line 53 of file SCP1000.h.
Constructor & Destructor Documentation
SCP1000 | ( | PinName | mosi, |
PinName | miso, | ||
PinName | sclk, | ||
PinName | cs, | ||
PinName | trig, | ||
PinName | drdy | ||
) |
Create an SCP1000 interface.
- Parameters:
-
mosi Master-Output-Slave-Input line of SPI miso Master-Input-Slave-Output line of SPI sclk Serial Clock of SPI cs Chip Select signal to SCP1000 trig Measuremnt Trigger (TRIG) to SCP1000 drdy Data Ready (DRDY) signal from SCP1000
Definition at line 22 of file SCP1000.cpp.
Member Function Documentation
int init | ( | void | ) |
Initialize the SCP1000.
Definition at line 86 of file SCP1000.cpp.
bool IsReady | ( | void | ) |
Check the data readiness.
- Returns:
- DRDY pin status: false-Not Ready, true-Ready
Definition at line 175 of file SCP1000.cpp.
float readPressure | ( | void | ) |
Read atmospheric pressure measurement result in hectopascal.
- Returns:
- Atmospheric pressure in hPa
Definition at line 201 of file SCP1000.cpp.
float readTemperature | ( | void | ) |
Read temperature measurement result in degrees Celcius.
- Returns:
- Temperature in degrees Celcius
Definition at line 185 of file SCP1000.cpp.
void setMode | ( | int | mode ) |
Set the measurement mode of the SCP1000.
- Parameters:
-
mode Measurement mode: 0-HIGH_RESOLUTION, 1-HIGH_SPEED, 2-ULTRA_LOW_POWER, 3-LOW_POWER_17BIT, 4-LOW_POWER_15BIT
Definition at line 128 of file SCP1000.cpp.
void triggerHard | ( | void | ) |
Trigger the measurement by TRIG wire in Low Power Mode NOTE: IT CURRENTLY SEEMS NOT WORKING.
PLEASE USE triggerSoft() INSTEAD.
Definition at line 161 of file SCP1000.cpp.
void triggerSoft | ( | void | ) |
Trigger the measurement by register writing in Low Power Mode.
Definition at line 169 of file SCP1000.cpp.
Generated on Wed Jul 27 2022 01:30:32 by
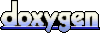