A fork with some I2C optimizations that speed up the display.
Fork of SSD1308_128x64_I2C by
SSD1308 Class Reference
Class to control an SSD1308 based oled Display. More...
#include <SSD1308.h>
Public Member Functions | |
SSD1308 (I2C &i2c, uint8_t address=SSD1308_DEF_SA) | |
Constructor. | |
void | initialize () |
High level Init, most settings remain at Power-On reset value. | |
void | clearDisplay () |
clear the display | |
void | fillDisplay (uint8_t pattern=0x00, uint8_t start_page=0, uint8_t end_page=MAX_PAGE, uint8_t start_col=0, uint8_t end_col=MAX_COL) |
fill the display | |
void | writeBitmap (uint8_t *data, uint8_t start_page=0, uint8_t end_page=MAX_PAGE, uint8_t start_col=0, uint8_t end_col=MAX_COL) |
write a bitmap to the display | |
void | writeProgressBar (uint8_t page, uint8_t col, int percentage) |
write a level meter to the display, Width is (PRG_MAX_SCALE + 2) pixels | |
void | writeLevelBar (uint8_t page, uint8_t col, int percentage) |
write a level meter to the display, Width is (PRG_MAX_SCALE + 2) pixels | |
void | writeChar (char chr) |
Write single character to the display using the 8x8 fontable. | |
void | writeBigChar (uint8_t row, uint8_t col, char chr) |
Write large character (16x24 font) | |
void | writeString (uint8_t row, uint8_t col, const char *txt) |
Write a string to the display using the 8x8 font. | |
void | setHorizontalAddressingMode () |
Set Horizontal Addressing Mode (cursor incr left-to-right, top-to-bottom) | |
void | setVerticalAddressingMode () |
Set Vertical Addressing Mode (cursor incr top-to-bottom, left-to-right) | |
void | setPageAddressingMode () |
Set Page Addressing Mode (cursor incr left-to-right) | |
void | setMemoryAddressingMode (uint8_t mode) |
Set Addressing Mode. | |
void | setColumnStartForPageAddressingMode (uint8_t column) |
Set Column Start (for Page Addressing Mode only) | |
void | setPageStartForPageAddressingMode (uint8_t page) |
Set Page Start (for Page Addressing Mode only) | |
void | setColumnAddress (uint8_t start, uint8_t end) |
void | setPageAddress (uint8_t start, uint8_t end) |
void | setDisplayStartLine (uint8_t line) |
Set Display StartLine, takes one byte, 0x00-0x3F. | |
void | setContrastControl (uint8_t contrast) |
Set Contrast. | |
void | setEntireDisplayOn () |
Shows All Pixels On. | |
void | setEntireDisplayRAM () |
Shows Pixels as RAM content. | |
void | setEntireDisplay (bool on) |
Shows Pixels On or as RAM content. | |
void | setInternalIref () |
Sets Internal Iref. | |
void | setExternalIref () |
Sets External Iref (default) | |
void | setDisplayOn () |
Enable Display. | |
void | setDisplayOff () |
Disable Display. | |
void | setDisplayPower (bool on) |
Enable or Disable Display. | |
void | setDisplayNormal () |
Show White pixels on Black background. | |
void | setDisplayInverse () |
Show Black pixels on White background. | |
void | setDisplayBlink (bool on) |
Blink display by fading in and out over a set number of frames. | |
void | setDisplayFade (bool on) |
Fade out display in set number of frames. | |
void | setDisplayFlip (bool left, bool down) |
Display Flip (Left/Right, Up/Down) | |
void | setContinuousHorizontalScroll (bool left, uint8_t start_page, uint8_t end_page, uint8_t interval) |
Horizontal scroll by one column per interval. | |
void | setContinuousVerticalAndHorizontalScroll (bool left, uint8_t start_page, uint8_t end_page, uint8_t offset, uint8_t interval) |
Horizontal and Vertical scroll by one column per interval. | |
void | setDisplayScroll (bool on) |
Activate or Deactivate Horizontal and Vertical scroll. | |
void | setVerticalScrollArea (uint8_t topRowsFixed, uint8_t scrollRows) |
Set Vertical scroll area. |
Detailed Description
Class to control an SSD1308 based oled Display.
Example:
#include "mbed.h" #include "mbed_logo.h" #include "SSD1308.h" //Pin Defines for I2C Bus #define D_SDA p28 #define D_SCL p27 I2C i2c(D_SDA, D_SCL); // Host PC Communication channels Serial pc(USBTX, USBRX); // tx, rx // Instantiate OLED SSD1308 oled = SSD1308(i2c, SSD1308_SA0); int main() { pc.printf("OLED test start\r"); oled.writeString(0, 0, "Hello World !"); // oled.printf("Hello World !"); oled.fillDisplay(0xAA); oled.setDisplayOff(); wait(1); oled.setDisplayOn(); oled.clearDisplay(); oled.setDisplayInverse(); wait(0.5); oled.setDisplayNormal(); oled.writeBitmap((uint8_t*) mbed_logo); pc.printf("OLED test done\r\n"); }
Definition at line 219 of file SSD1308.h.
Constructor & Destructor Documentation
SSD1308 | ( | I2C & | i2c, |
uint8_t | deviceAddress = SSD1308_DEF_SA |
||
) |
Constructor.
- Parameters:
-
I2C &i2c reference to i2c, uint8_t deviceAddress slaveaddress (8bit to use for the controller (0x78 by default, assumes D/C# (pin 13) grounded) I2C &i2c reference to i2c uint8_t deviceAddress slaveaddress
Definition at line 65 of file SSD1308.cpp.
Member Function Documentation
void clearDisplay | ( | ) |
clear the display
Definition at line 90 of file SSD1308.cpp.
void fillDisplay | ( | uint8_t | pattern = 0x00 , |
uint8_t | start_page = 0 , |
||
uint8_t | end_page = MAX_PAGE , |
||
uint8_t | start_col = 0 , |
||
uint8_t | end_col = MAX_COL |
||
) |
fill the display
- Parameters:
-
uint8_t pattern fillpattern vertical patch or 8 bits uint8_t start_page begin page (0..MAX_PAGE) uint8_t end_page end page (start_page..MAX_PAGE) uint8_t start_col begin column (0..MAX_COL) uint8_t end_col end column (start_col..MAX_COL)
Definition at line 139 of file SSD1308.cpp.
void initialize | ( | ) |
High level Init, most settings remain at Power-On reset value.
Definition at line 75 of file SSD1308.cpp.
void setColumnAddress | ( | uint8_t | start, |
uint8_t | end | ||
) |
- Parameters:
-
uint8_t start startcolumn (valid range 0..MAX_COL) uint8_t end endcolumn (valid range start..MAX_COL)
Definition at line 768 of file SSD1308.cpp.
void setColumnStartForPageAddressingMode | ( | uint8_t | column ) |
Set Column Start (for Page Addressing Mode only)
- Parameters:
-
uint8_t column column start (valid range 0..MAX_COLS) uint8_t column column start (valid range 0..MAX_COL)
Definition at line 789 of file SSD1308.cpp.
void setContinuousHorizontalScroll | ( | bool | left, |
uint8_t | start_page, | ||
uint8_t | end_page, | ||
uint8_t | interval | ||
) |
Horizontal scroll by one column per interval.
- Parameters:
-
bool left select Left/Right scroll uint8_t start_page begin page (0..MAX_PAGE) uint8_t end_page end page (start_page..MAX_PAGE) uint8_t interval scroll interval in frames (see codes above)
Definition at line 953 of file SSD1308.cpp.
void setContinuousVerticalAndHorizontalScroll | ( | bool | left, |
uint8_t | start_page, | ||
uint8_t | end_page, | ||
uint8_t | offset, | ||
uint8_t | interval | ||
) |
Horizontal and Vertical scroll by one column per interval.
- Parameters:
-
bool left select Left/Right scroll uint8_t start_page begin page (0..MAX_PAGE) uint8_t end_page end page (start_page..MAX_PAGE) uint8_t offset vert offset (0x01..0x63) uint8_t interval scroll interval in frames (see codes above)
Definition at line 971 of file SSD1308.cpp.
void setContrastControl | ( | uint8_t | contrast ) |
Set Contrast.
- Parameters:
-
uint8_t contrast (valid range 0x00 (lowest) - 0xFF (highest))
Definition at line 814 of file SSD1308.cpp.
void setDisplayBlink | ( | bool | on ) |
Blink display by fading in and out over a set number of frames.
- Parameters:
-
bool on
Definition at line 857 of file SSD1308.cpp.
void setDisplayFade | ( | bool | on ) |
Fade out display in set number of frames.
- Parameters:
-
bool on
Definition at line 870 of file SSD1308.cpp.
void setDisplayFlip | ( | bool | left, |
bool | down | ||
) |
Display Flip (Left/Right, Up/Down)
- Parameters:
-
bool left flip Left/Right bool down flip Up/Down
Definition at line 883 of file SSD1308.cpp.
void setDisplayInverse | ( | ) |
Show Black pixels on White background.
Definition at line 850 of file SSD1308.cpp.
void setDisplayNormal | ( | ) |
Show White pixels on Black background.
Definition at line 844 of file SSD1308.cpp.
void setDisplayOff | ( | ) |
Disable Display.
Definition at line 827 of file SSD1308.cpp.
void setDisplayOn | ( | ) |
Enable Display.
Definition at line 821 of file SSD1308.cpp.
void setDisplayPower | ( | bool | on ) |
void setDisplayScroll | ( | bool | on ) |
Activate or Deactivate Horizontal and Vertical scroll.
Note: after deactivating scrolling, the RAM data needs to be rewritten
- Parameters:
-
bool on activate scroll
Definition at line 999 of file SSD1308.cpp.
void setDisplayStartLine | ( | uint8_t | line ) |
Set Display StartLine, takes one byte, 0x00-0x3F.
- Parameters:
-
uint8_t line startline (valid range 0..MAX_ROWS)
Definition at line 777 of file SSD1308.cpp.
void setEntireDisplay | ( | bool | on ) |
Shows Pixels On or as RAM content.
- Parameters:
-
bool on (true is All on, false is RAM content)
Definition at line 937 of file SSD1308.cpp.
void setEntireDisplayOn | ( | ) |
Shows All Pixels On.
Definition at line 924 of file SSD1308.cpp.
void setEntireDisplayRAM | ( | ) |
Shows Pixels as RAM content.
Definition at line 930 of file SSD1308.cpp.
void setExternalIref | ( | ) |
Sets External Iref (default)
Definition at line 915 of file SSD1308.cpp.
void setHorizontalAddressingMode | ( | ) |
Set Horizontal Addressing Mode (cursor incr left-to-right, top-to-bottom)
Definition at line 729 of file SSD1308.cpp.
void setInternalIref | ( | ) |
Sets Internal Iref.
Definition at line 906 of file SSD1308.cpp.
void setMemoryAddressingMode | ( | uint8_t | mode ) |
void setPageAddress | ( | uint8_t | start, |
uint8_t | end | ||
) |
- Parameters:
-
uint8_t start startpage (valid range 0..MAX_PAGE) uint8_t end endpage (valid range start..MAX_PAGE)
Definition at line 759 of file SSD1308.cpp.
void setPageAddressingMode | ( | ) |
Set Page Addressing Mode (cursor incr left-to-right)
Definition at line 743 of file SSD1308.cpp.
void setPageStartForPageAddressingMode | ( | uint8_t | page ) |
Set Page Start (for Page Addressing Mode only)
- Parameters:
-
uint8_t page page start (valid range PAGE0 - PAGE7)
Definition at line 802 of file SSD1308.cpp.
void setVerticalAddressingMode | ( | ) |
Set Vertical Addressing Mode (cursor incr top-to-bottom, left-to-right)
Definition at line 736 of file SSD1308.cpp.
void setVerticalScrollArea | ( | uint8_t | topRowsFixed, |
uint8_t | scrollRows | ||
) |
Set Vertical scroll area.
- Parameters:
-
uint8_t topRowsFixed fixed rows (0..MAX_ROW) uint8_t scrollRowsoffset scroll rows (topRowsFixed..MAX_ROW) uint8_t topRowsFixed fixed rows (0..MAX_ROW) uint8_t scrollRowsoffset scroll rows (topRowsFixed..ROWS)
Definition at line 986 of file SSD1308.cpp.
void writeBigChar | ( | uint8_t | row, |
uint8_t | col, | ||
char | chr | ||
) |
Write large character (16x24 font)
- Parameters:
-
uint8_t row row number (0...MAX_ROW) uint8_t col column number (0...MAX_COL) char chr Used for displaying numbers 0 - 9 and '+', '-', '.'
Definition at line 484 of file SSD1308.cpp.
void writeBitmap | ( | uint8_t * | data, |
uint8_t | start_page = 0 , |
||
uint8_t | end_page = MAX_PAGE , |
||
uint8_t | start_col = 0 , |
||
uint8_t | end_col = MAX_COL |
||
) |
write a bitmap to the display
- Parameters:
-
uint8_t* data pointer to bitmap uint8_t start_page begin page (0..MAX_PAGE) uint8_t end_page end page (start_page..MAX_PAGE) uint8_t start_col begin column (0..MAX_COL) uint8_t end_col end column (start_col..MAX_COL)
Definition at line 195 of file SSD1308.cpp.
void writeChar | ( | char | chr ) |
Write single character to the display using the 8x8 fontable.
Start at current cursor location
- Parameters:
-
char chr character to write
Definition at line 420 of file SSD1308.cpp.
void writeLevelBar | ( | uint8_t | page, |
uint8_t | col, | ||
int | percentage | ||
) |
write a level meter to the display, Width is (PRG_MAX_SCALE + 2) pixels
- Parameters:
-
uint8_t page begin page (0..MAX_PAGE) uint8_t col begin column (0..MAX_COL) int percentage value (0..100)
Definition at line 339 of file SSD1308.cpp.
void writeProgressBar | ( | uint8_t | page, |
uint8_t | col, | ||
int | percentage | ||
) |
write a level meter to the display, Width is (PRG_MAX_SCALE + 2) pixels
- Parameters:
-
uint8_t page begin page (0..MAX_PAGE) uint8_t col begin column (0..MAX_COL) int percentage value (0..100)
Definition at line 255 of file SSD1308.cpp.
void writeString | ( | uint8_t | row, |
uint8_t | col, | ||
const char * | text | ||
) |
Write a string to the display using the 8x8 font.
Start at selected cursor location, text will wrap around until it is done
- Parameters:
-
uint8_t row row number (0...ROWS/FONT_HEIGHT) uint8_t col column number (0...COLUMNS/FONT_WIDTH) const char * text pointer to text
Definition at line 444 of file SSD1308.cpp.
Generated on Sat Jul 23 2022 16:11:42 by
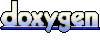