A fork with some I2C optimizations that speed up the display.
Fork of SSD1308_128x64_I2C by
SSD1308.h
00001 /** @file SSD1308 I2C device class header file 00002 * Based on Solomon Systech SSD1308 datasheet, rev. 1, 10/2008 00003 * The SSD1308 is used for example in the Seeed 128x64 OLED Display 00004 * http://www.seeedstudio.com/depot/grove-oled-display-12864-p-781.html?cPath=163_167 00005 */ 00006 // The original code by Andrew Schamp is using (and has been submitted as a part of) Jeff Rowberg's I2Cdevlib library, 00007 // which should (hopefully) always be available at https://github.com/jrowberg/i2cdevlib 00008 // Some parts also mashed up from Graphic Library for driving monochrome displays based on the PCD8544, 00009 // Copyright (c) 2011, Wim De Roeve, who in turn did partial port of code found on 00010 // http://serdisplib.sourceforge.net/ser/pcd8544.html#links and by Petras Saduikis <petras@petras.co.uk> 00011 // 00012 // Changelog: 00013 // 2011-08-25 - Initial release by Andrew Schamp <schamp@gmail.com> 00014 // 2012-06-19 - Ported to mbed and optimised (WH) 00015 // 2013-07-12 - Minor comment fix and placeholder for SSD1306 (WH) 00016 // 2015-01-01 - Switch for optimised I2C calls to test on F401 (WH) 00017 // 00018 /* 00019 ================================================================================ 00020 I2Cdev device library code is placed under the MIT license 00021 Copyright (c) 2011 Andrew Schamp 00022 Copyright (c) 2012,2013 WH (mbed port) 00023 00024 Permission is hereby granted, free of charge, to any person obtaining a copy 00025 of this software and associated documentation files (the "Software"), to deal 00026 in the Software without restriction, including without limitation the rights 00027 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00028 copies of the Software, and to permit persons to whom the Software is 00029 furnished to do so, subject to the following conditions: 00030 00031 The above copyright notice and this permission notice shall be included in 00032 all copies or substantial portions of the Software. 00033 00034 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00035 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00036 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00037 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00038 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00039 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00040 THE SOFTWARE. 00041 ================================================================================ 00042 */ 00043 00044 #ifndef SSD1308_H 00045 #define SSD1308_H 00046 00047 // This is the I2C address (8 bit) 00048 // There are two possible addresses: with D/C# (pin 13) grounded, the address is 0x78, 00049 // with D/C# tied high it is 0x7A. Assume grounded by default. 00050 #define SSD1308_SA0 0x78 00051 #define SSD1308_SA1 0x7A 00052 #define SSD1308_DEF_SA SSD1308_SA0 00053 00054 // Display dimensions 00055 #define ROWS 64 00056 #define COLUMNS 128 00057 #define PAGES (ROWS / 8) 00058 #define MAX_PAGE (PAGES - 1) 00059 #define MAX_ROW (ROWS - 1) 00060 #define MAX_COL (COLUMNS - 1) 00061 00062 // Character dimensions 8x8 font 00063 #define CHARS (COLUMNS / FONT8x8_WIDTH) 00064 00065 // Command and Datamode 00066 #define COMMAND_MODE 0x80 // continuation bit is set! 00067 #define DATA_MODE 0x40 00068 00069 // Commands and Parameter defines 00070 #define SET_LOWER_COLUMN 0x00 // | with lower nibble (Page mode only) 00071 #define SET_HIGHER_COLUMN 0x10 // | with higher nibble (Page mode only) 00072 00073 #define HORIZONTAL_ADDRESSING_MODE 0x00 00074 #define VERTICAL_ADDRESSING_MODE 0x01 00075 #define PAGE_ADDRESSING_MODE 0x02 00076 #define SET_MEMORY_ADDRESSING_MODE 0x20 // takes one byte as given above 00077 00078 #define SET_COLUMN_ADDRESS 0x21 // takes two bytes, start address and end address of display data RAM 00079 #define SET_PAGE_ADDRESS 0x22 // takes two bytes, start address and end address of display data RAM 00080 00081 // Command maybe unsupported by SSD1308 00082 #define FADE_INTERVAL_8_FRAMES 0x00 00083 #define FADE_INTERVAL_16_FRAMES 0x01 00084 #define FADE_INTERVAL_24_FRAMES 0x02 00085 #define FADE_INTERVAL_32_FRAMES 0x03 00086 #define FADE_INTERVAL_64_FRAMES 0x07 00087 #define FADE_INTERVAL_128_FRAMES 0x0F 00088 #define FADE_BLINK_DISABLE 0x00 00089 #define FADE_OUT_ENABLE 0x20 00090 #define BLINK_ENABLE 0x30 00091 #define SET_FADE_BLINK 0x23 // takes one byte 00092 // bit5-4 = 0, fade/blink mode 00093 // bit3-0 = Time interval in frames 00094 00095 #define SET_DISPLAY_START_LINE 0x40 // | with a row number 0-63 to set start row. (Reset = 0) 00096 00097 #define SET_CONTRAST 0x81 // takes one byte, 0x00 - 0xFF 00098 00099 #define SET_SEGMENT_REMAP_0 0xA0 // column address 0 is mapped to SEG0 (Reset) 00100 #define SET_SEGMENT_REMAP_127 0xA1 // column address 127 is mapped to SEG0 00101 00102 #define SET_DISPLAY_GDDRAM 0xA4 // restores display to contents of RAM 00103 #define SET_ENTIRE_DISPLAY_ON 0xA5 // turns all pixels on, does not affect RAM 00104 00105 #define SET_NORMAL_DISPLAY 0xA6 // a databit of 1 indicates pixel 'ON' 00106 #define SET_INVERSE_DISPLAY 0xA7 // a databit of 1 indicates pixel 'OFF' 00107 00108 #define SET_MULTIPLEX_RATIO 0xA8 // takes one byte, from 16xMUX to 64xMUX (MUX Ratio = byte+1; Default 64) 00109 00110 #define EXTERNAL_IREF 0x10 00111 #define INTERNAL_IREF 0x00 00112 #define SET_IREF_SELECTION 0xAD // sets internal or external Iref 00113 00114 #define SET_DISPLAY_POWER_OFF 0xAE 00115 #define SET_DISPLAY_POWER_ON 0xAF 00116 00117 #define PAGE0 0x00 00118 #define PAGE1 0x01 00119 #define PAGE2 0x02 00120 #define PAGE3 0x03 00121 #define PAGE4 0x04 00122 #define PAGE5 0x05 00123 #define PAGE6 0x06 00124 #define PAGE7 0x07 00125 #define SET_PAGE_START_ADDRESS 0xB0 // | with a page number to get start address (Page mode only) 00126 00127 #define SET_COMMON_REMAP_0 0xC0 // row address 0 is mapped to COM0 (Reset) 00128 #define SET_COMMON_REMAP_63 0xC8 // row address 63 is mapped to COM0 00129 00130 #define SET_DISPLAY_OFFSET 0xD3 // takes one byte from 0-63 for vertical shift, Reset = 0 00131 00132 #define SET_DISPLAY_CLOCK 0xD5 // takes one byte 00133 // bit7-4 = Osc Freq DCLK (Reset = 1000b) 00134 // bit3-0 = Divide ration (Reset = oooob, Ratio = 1) 00135 00136 #define SET_PRECHARGE_TIME 0xD9 // takes one byte 00137 // bit7-4 = Phase2, upto 15 DCLKs (Reset = 0010b) 00138 // bit3-0 = Phase1, upto 15 DCLKs (Reset = 0010b) 00139 00140 00141 #define COMMON_BASE 0x02 // 00142 #define COMMON_SEQUENTIAL 0x00 // Sequential common pins config 00143 #define COMMON_ALTERNATIVE 0x10 // Odd/Even common pins config (Reset) 00144 #define COMMON_LEFTRIGHT_NORMAL 0x00 // LeftRight Normal (Reset) 00145 #define COMMON_LEFTRIGHT_FLIP 0x20 // LeftRight Flip 00146 #define SET_COMMON_CONF 0xDA // takes one byte as given above 00147 00148 00149 #define VCOMH_DESELECT_0_65_CODE 0x00 00150 #define VCOMH_DESELECT_0_77_CODE 0x20 00151 #define VCOMH_DESELECT_0_83_CODE 0x30 00152 #define SET_VCOMH_DESELECT_LEVEL 0xDB // takes one byte as given above 00153 00154 #define NOP 0xE3 00155 00156 #define SCROLL_INTERVAL_5_FRAMES 0x00 00157 #define SCROLL_INTERVAL_64_FRAMES 0x01 00158 #define SCROLL_INTERVAL_128_FRAMES 0x02 00159 #define SCROLL_INTERVAL_256_FRAMES 0x03 00160 #define SCROLL_INTERVAL_3_FRAMES 0x04 00161 #define SCROLL_INTERVAL_4_FRAMES 0x05 00162 #define SCROLL_INTERVAL_25_FRAMES 0x06 00163 #define SCROLL_INTERVAL_2_FRAMES 0x07 00164 00165 #define SET_RIGHT_HOR_SCROLL 0x26 // takes 6 bytes: 0x00, PageStart, Scroll_Interval, PageEnd, 0x00, 0xFF 00166 #define SET_LEFT_HOR_SCROLL 0x27 // takes 6 bytes: 0x00, PageStart, Scroll_Interval, PageEnd, 0x00, 0xFF 00167 00168 #define SET_VERT_RIGHT_HOR_SCROLL 0x29 // takes 5 bytes: 0x00, PageStart, Scroll_Interval, PageEnd, VertOffset 00169 #define SET_VERT_LEFT_HOR_SCROLL 0x2A // takes 5 bytes: 0x00, PageStart, Scroll_Interval, PageEnd, VertOffset 00170 00171 #define SET_DEACTIVATE_SCROLL 0x2E 00172 #define SET_ACTIVATE_SCROLL 0x2F 00173 00174 #define SET_VERTICAL_SCROLL_AREA 0xA3 // takes 2 bytes: Rows in Top Area (Reset=0), Rows in Scroll Area (Reset=64) 00175 00176 00177 00178 /** Class to control an SSD1308 based oled Display 00179 * 00180 * Example: 00181 * @code 00182 * #include "mbed.h" 00183 * #include "mbed_logo.h" 00184 * #include "SSD1308.h" 00185 00186 * //Pin Defines for I2C Bus 00187 * #define D_SDA p28 00188 * #define D_SCL p27 00189 * I2C i2c(D_SDA, D_SCL); 00190 * 00191 * // Host PC Communication channels 00192 * Serial pc(USBTX, USBRX); // tx, rx 00193 * 00194 * // Instantiate OLED 00195 * SSD1308 oled = SSD1308(i2c, SSD1308_SA0); 00196 * 00197 * int main() { 00198 * pc.printf("OLED test start\r"); 00199 * oled.writeString(0, 0, "Hello World !"); 00200 * // oled.printf("Hello World !"); 00201 * 00202 * oled.fillDisplay(0xAA); 00203 * oled.setDisplayOff(); 00204 * wait(1); 00205 * oled.setDisplayOn(); 00206 * 00207 * oled.clearDisplay(); 00208 * oled.setDisplayInverse(); 00209 * wait(0.5); 00210 * oled.setDisplayNormal(); 00211 * 00212 * oled.writeBitmap((uint8_t*) mbed_logo); 00213 * 00214 * pc.printf("OLED test done\r\n"); 00215 * } 00216 * 00217 * @endcode 00218 */ 00219 class SSD1308 : public Stream { 00220 public: 00221 00222 /** 00223 *@brief Constructor 00224 *@param I2C &i2c reference to i2c, 00225 *@param uint8_t deviceAddress slaveaddress (8bit to use for the controller (0x78 by default, assumes D/C# (pin 13) grounded) 00226 */ 00227 SSD1308(I2C &i2c, uint8_t address = SSD1308_DEF_SA); 00228 00229 // High Level methods 00230 00231 /** @brief High level Init, most settings remain at Power-On reset value 00232 */ 00233 void initialize(); 00234 00235 /** @brief clear the display 00236 */ 00237 void clearDisplay(); 00238 00239 00240 /** @brief fill the display 00241 * @param uint8_t pattern fillpattern vertical patch or 8 bits 00242 * @param uint8_t start_page begin page (0..MAX_PAGE) 00243 * @param uint8_t end_page end page (start_page..MAX_PAGE) 00244 * @param uint8_t start_col begin column (0..MAX_COL) 00245 * @param uint8_t end_col end column (start_col..MAX_COL) 00246 */ 00247 void fillDisplay(uint8_t pattern = 0x00, 00248 uint8_t start_page=0, uint8_t end_page=MAX_PAGE, 00249 uint8_t start_col=0, uint8_t end_col=MAX_COL); 00250 00251 00252 /** @brief write a bitmap to the display 00253 * @param uint8_t* data pointer to bitmap 00254 * @param uint8_t start_page begin page (0..MAX_PAGE) 00255 * @param uint8_t end_page end page (start_page..MAX_PAGE) 00256 * @param uint8_t start_col begin column (0..MAX_COL) 00257 * @param uint8_t end_col end column (start_col..MAX_COL) 00258 */ 00259 void writeBitmap(uint8_t* data, 00260 uint8_t start_page=0, uint8_t end_page=MAX_PAGE, 00261 uint8_t start_col=0, uint8_t end_col=MAX_COL); 00262 00263 /** @brief write a level meter to the display, Width is (PRG_MAX_SCALE + 2) pixels 00264 * @param uint8_t page begin page (0..MAX_PAGE) 00265 * @param uint8_t col begin column (0..MAX_COL) 00266 * @param int percentage value (0..100) 00267 */ 00268 void writeProgressBar(uint8_t page, uint8_t col, int percentage); 00269 00270 00271 /** @brief write a level meter to the display, Width is (PRG_MAX_SCALE + 2) pixels 00272 * @param uint8_t page begin page (0..MAX_PAGE) 00273 * @param uint8_t col begin column (0..MAX_COL) 00274 * @param int percentage value (0..100) 00275 */ 00276 void writeLevelBar(uint8_t page, uint8_t col, int percentage); 00277 00278 //void setXY(uint8_t, uint8_t y); 00279 00280 // Select inverted or normal text 00281 void setInverted(bool inverted) { _inverted = inverted; }; 00282 00283 /** @brief Write single character to the display using the 8x8 fontable 00284 * @brief Start at current cursor location 00285 * @param char chr character to write 00286 */ 00287 void writeChar(char chr); 00288 00289 /** @brief Write large character (16x24 font) 00290 * @param uint8_t row row number (0...MAX_ROW) 00291 * @param uint8_t col column number (0...MAX_COL) 00292 * @param char chr Used for displaying numbers 0 - 9 and '+', '-', '.' 00293 */ 00294 void writeBigChar(uint8_t row, uint8_t col, char chr); 00295 00296 /** @brief Write a string to the display using the 8x8 font 00297 * @brief Start at selected cursor location, text will wrap around until it is done 00298 * @param uint8_t row row number (0...ROWS/FONT_HEIGHT) 00299 * @param uint8_t col column number (0...COLUMNS/FONT_WIDTH) 00300 * @param const char * text pointer to text 00301 */ 00302 void writeString(uint8_t row, uint8_t col, const char* txt); 00303 00304 // Stream implementation - provides printf() interface 00305 // You would otherwise be forced to use writeChar() or writeString() 00306 virtual int _putc(int value) { writeChar(value); return 1; }; 00307 virtual int _getc() { return -1; }; 00308 00309 // Future extension with graphics features 00310 // this must be defined by the subclass 00311 // virtual void drawPixel(int16_t x, int16_t y, uint16_t color) = 0; 00312 00313 // Medium Level methods 00314 00315 /** @brief Set Horizontal Addressing Mode (cursor incr left-to-right, top-to-bottom) 00316 * 00317 */ 00318 void setHorizontalAddressingMode(); 00319 00320 /** @brief Set Vertical Addressing Mode (cursor incr top-to-bottom, left-to-right) 00321 * 00322 */ 00323 void setVerticalAddressingMode(); 00324 00325 /** @brief Set Page Addressing Mode (cursor incr left-to-right) 00326 * 00327 */ 00328 void setPageAddressingMode(); 00329 00330 /** @brief Set Addressing Mode 00331 * @param uint8_t mode 00332 */ 00333 void setMemoryAddressingMode(uint8_t mode); 00334 00335 00336 /** 00337 * @brief Set Column Start (for Page Addressing Mode only) 00338 * @param uint8_t column column start (valid range 0..MAX_COLS) 00339 */ 00340 void setColumnStartForPageAddressingMode(uint8_t column); 00341 00342 /** 00343 * @brief Set Page Start (for Page Addressing Mode only) 00344 * @param uint8_t page page start (valid range PAGE0 - PAGE7) 00345 */ 00346 void setPageStartForPageAddressingMode(uint8_t page); 00347 00348 00349 00350 /** @param uint8_t start startcolumn (valid range 0..MAX_COL) 00351 * @param uint8_t end endcolumn (valid range start..MAX_COL) 00352 */ 00353 void setColumnAddress (uint8_t start, uint8_t end); 00354 00355 /** @param uint8_t start startpage (valid range 0..MAX_PAGE) 00356 * @param uint8_t end endpage (valid range start..MAX_PAGE) 00357 */ 00358 void setPageAddress (uint8_t start, uint8_t end); 00359 00360 00361 /** 00362 * @brief Set Display StartLine, takes one byte, 0x00-0x3F 00363 * @param uint8_t line startline (valid range 0..MAX_ROWS) 00364 */ 00365 void setDisplayStartLine(uint8_t line); 00366 00367 /** @brief Set Contrast 00368 * @param uint8_t contrast (valid range 0x00 (lowest) - 0xFF (highest)) 00369 */ 00370 void setContrastControl(uint8_t contrast); 00371 00372 00373 /** @brief Shows All Pixels On 00374 */ 00375 void setEntireDisplayOn(); 00376 00377 /** @brief Shows Pixels as RAM content 00378 */ 00379 void setEntireDisplayRAM(); 00380 00381 /** @brief Shows Pixels On or as RAM content 00382 * @param bool on (true is All on, false is RAM content) 00383 */ 00384 void setEntireDisplay(bool on); 00385 00386 00387 // @brief Set Display line MPX Ratio, takes one byte, 0x00-0x3F 00388 // @param uint8_t lines (valid range 0..MAX_ROWS) 00389 void setMultiplexRatio(uint8_t lines); 00390 00391 00392 /** @brief Sets Internal Iref 00393 */ 00394 void setInternalIref(); 00395 00396 /** @brief Sets External Iref (default) 00397 */ 00398 void setExternalIref(); 00399 00400 00401 /** @brief Enable Display 00402 */ 00403 void setDisplayOn(); 00404 00405 /** @brief Disable Display 00406 */ 00407 void setDisplayOff(); 00408 00409 /** @brief Enable or Disable Display 00410 * @param bool on 00411 */ 00412 void setDisplayPower(bool on); 00413 00414 /** @brief Show White pixels on Black background 00415 */ 00416 void setDisplayNormal(); 00417 00418 /** @brief Show Black pixels on White background 00419 */ 00420 void setDisplayInverse(); 00421 00422 /** @brief Blink display by fading in and out over a set number of frames 00423 * @param bool on 00424 */ 00425 void setDisplayBlink(bool on); 00426 00427 /** @brief Fade out display in set number of frames 00428 * @param bool on 00429 */ 00430 void setDisplayFade(bool on); 00431 00432 /** @brief Display Flip (Left/Right, Up/Down) 00433 * @param bool left flip Left/Right 00434 * @param bool down flip Up/Down 00435 */ 00436 void setDisplayFlip(bool left, bool down); 00437 00438 // Set vertical shift by COM from 0 - 63 (0x00 - 0x3F) (Reset = 0x00) 00439 void setDisplayOffset(uint8_t offset); 00440 00441 // Oscillator freq 0x00-0x0F (reset 0x08) 00442 // Divide ratio 0x00-0x0F, value +1 (reset 0x00) 00443 void setDisplayClock(uint8_t divideRatio, uint8_t oscFreq); 00444 00445 // Phase1 0x01-0x0F period of up to 15 DCLK clocks (reset 0x02, 0 is invalid) 00446 // Phase2 0x01-0x0F period of up to 15 DCLK clocks (reset 0x02, 0 is invalid) 00447 void setPrechargePeriod(uint8_t phase1, uint8_t phase2); 00448 00449 // See defines above for levels 00450 void setVcomhDeselectLevel(uint8_t level); 00451 00452 00453 // Command for no-operation 00454 void nop(); 00455 00456 00457 /** @brief Horizontal scroll by one column per interval 00458 * @param bool left select Left/Right scroll 00459 * @param uint8_t start_page begin page (0..MAX_PAGE) 00460 * @param uint8_t end_page end page (start_page..MAX_PAGE) 00461 * @param uint8_t interval scroll interval in frames (see codes above) 00462 */ 00463 void setContinuousHorizontalScroll(bool left, uint8_t start_page, uint8_t end_page, uint8_t interval); 00464 00465 00466 /** @brief Horizontal and Vertical scroll by one column per interval 00467 * @param bool left select Left/Right scroll 00468 * @param uint8_t start_page begin page (0..MAX_PAGE) 00469 * @param uint8_t end_page end page (start_page..MAX_PAGE) 00470 * @param uint8_t offset vert offset (0x01..0x63) 00471 * @param uint8_t interval scroll interval in frames (see codes above) 00472 */ 00473 void setContinuousVerticalAndHorizontalScroll(bool left, uint8_t start_page, uint8_t end_page, 00474 uint8_t offset, uint8_t interval); 00475 00476 /** @brief Activate or Deactivate Horizontal and Vertical scroll 00477 * @brief Note: after deactivating scrolling, the RAM data needs to be rewritten 00478 * @param bool on activate scroll 00479 */ 00480 void setDisplayScroll(bool on); 00481 00482 00483 /** @brief Set Vertical scroll area 00484 * @param uint8_t topRowsFixed fixed rows (0..MAX_ROW) 00485 * @param uint8_t scrollRowsoffset scroll rows (topRowsFixed..MAX_ROW) 00486 */ 00487 void setVerticalScrollArea(uint8_t topRowsFixed, uint8_t scrollRows); 00488 00489 private: 00490 00491 // Low Level methods 00492 00493 /** @brief Write command that has no parameters 00494 */ 00495 void _sendCommand(uint8_t command); 00496 00497 /** @brief Write command that has one parameter 00498 */ 00499 void _sendCommand(uint8_t command, uint8_t param1); 00500 00501 /** @brief Write command that has two parameters 00502 */ 00503 void _sendCommand(uint8_t command, uint8_t param1, uint8_t param2); 00504 // void sendCommands(uint8_t len, uint8_t* buf); 00505 00506 /** @brief Write command that has five parameters 00507 */ 00508 void _sendCommand(uint8_t command, uint8_t param1, uint8_t param2, 00509 uint8_t param3, uint8_t param4, 00510 uint8_t param5); 00511 00512 /** @brief Write command that has six parameters 00513 */ 00514 void _sendCommand(uint8_t command, uint8_t param1, uint8_t param2, 00515 uint8_t param3, uint8_t param4, 00516 uint8_t param5, uint8_t param6); 00517 00518 /** @brief Write databyte to display 00519 * @brief Start at current cursor location 00520 * @param uint8_t data databyte to write 00521 */ 00522 void _sendData(uint8_t data); 00523 00524 /** @brief Write len bytes from buffer data to display, 00525 * @brief Start at current cursor location 00526 * @param uint8_t len number of bytes to write 00527 * @param uint8_t* data pointer to data 00528 */ 00529 void _sendData(uint8_t len, uint8_t* data); 00530 00531 /** @brief Low level Init 00532 * @brief Init the configuration registers in accordance with the datasheet 00533 */ 00534 void _init(); 00535 00536 I2C _i2c; // I2C bus reference 00537 uint8_t _readOpcode; // contains the I2C address of the device 00538 uint8_t _writeOpcode; // contains the I2C address of the device 00539 00540 bool _inverted; // inverted or normal text 00541 }; 00542 00543 #endif
Generated on Sat Jul 23 2022 16:11:42 by
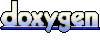