v 0.4
Dependents: MCP23S17Test MCP23S17_Basic_IO_Demo HelloWorld Lab3-SnakeGame ... more
MCP23S17.h
00001 /* MCP23S17 - drive the Microchip MCP23S17 16-bit Port Extender using SPI 00002 * Copyright (c) 2010 Romilly Cocking 00003 * Released under the MIT License: http://mbed.org/license/mit 00004 * 00005 * version 0.4 00006 */ 00007 #include "mbed.h" 00008 00009 #ifndef MCP23S17_H 00010 #define MCP23S17_H 00011 00012 #define INTERRUPT_POLARITY_BIT 0x02 00013 #define INTERRUPT_MIRROR_BIT 0x40 00014 00015 // all register addresses assume IOCON.BANK = 0 (POR default) 00016 00017 #define IODIRA 0x00 00018 #define GPINTENA 0x04 00019 #define DEFVALA 0x06 00020 #define INTCONA 0x08 00021 #define IOCON 0x0A 00022 #define GPPUA 0x0C 00023 #define GPIOA 0x12 00024 #define OLATA 0x14 00025 00026 // Control settings 00027 00028 #define IOCON_BANK 0x80 // Banked registers 00029 #define IOCON_BYTE_MODE 0x20 // Disables sequential operation. If bank = 0, operations toggle between A and B registers 00030 #define IOCON_HAEN 0x08 // Hardware address enable 00031 00032 enum Polarity { ACTIVE_LOW , ACTIVE_HIGH }; 00033 enum Port { PORT_A, PORT_B }; 00034 00035 class MCP23S17 { 00036 public: 00037 MCP23S17(SPI& spi, PinName ncs, char writeOpcode); 00038 void direction(Port port, char direction); 00039 void configurePullUps(Port port, char offOrOn); 00040 void interruptEnable(Port port, char interruptsEnabledMask); 00041 void interruptPolarity(Polarity polarity); 00042 void mirrorInterrupts(bool mirror); 00043 void defaultValue(Port port, char valuesToCompare); 00044 void interruptControl(Port port, char interruptContolBits); 00045 char read(Port port); 00046 void write(Port port, char byte); 00047 protected: 00048 SPI& _spi; 00049 DigitalOut _ncs; 00050 void _init(); 00051 void _write(Port port, char address, char data); 00052 void _write(char address, char data); 00053 char _read(Port port, char address); 00054 char _read(char address); 00055 char _readOpcode; 00056 char _writeOpcode; 00057 }; 00058 00059 #endif
Generated on Thu Jul 14 2022 03:21:09 by
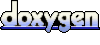