
konashi/SBBLEのテスト
Fork of BLE_LoopbackUART by
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "mbed.h" 00018 #include "BLEDevice.h" 00019 00020 const static char DEVICE_NAME[] = "mbed HRM1017"; 00021 00022 static const uint16_t KONASHI_SERVICE_UUID = 0xFF00; 00023 00024 static const uint16_t KONASHI_PIO_SETTING_UUID = 0x3000; 00025 static const uint16_t KONASHI_PIO_PULLUP_UUID = 0x3001; 00026 static const uint16_t KONASHI_PIO_OUTPUT_UUID = 0x3002; 00027 static const uint16_t KONASHI_PIO_INPUT_NOTIFICATION_UUID = 0x3003; 00028 00029 static const uint16_t KONASHI_PWM_CONFIG_UUID = 0x3004; 00030 static const uint16_t KONASHI_PWM_PARAM_UUID = 0x3005; 00031 static const uint16_t KONASHI_PWM_DUTY_UUID = 0x3006; 00032 00033 static const uint16_t KONASHI_ANALOG_DRIVE_UUID = 0x3007; 00034 static const uint16_t KONASHI_ANALOG_READ0_UUID = 0x3008; 00035 static const uint16_t KONASHI_ANALOG_READ1_UUID = 0x3009; 00036 static const uint16_t KONASHI_ANALOG_READ2_UUID = 0x300A; 00037 00038 static const uint16_t KONASHI_I2C_CONFIG_UUID = 0x300B; 00039 static const uint16_t KONASHI_I2C_START_STOP_UUID = 0x300C; 00040 static const uint16_t KONASHI_I2C_WRITE_UUID = 0x300D; 00041 static const uint16_t KONASHI_I2C_READ_PARAM_UIUD = 0x300E; 00042 static const uint16_t KONASHI_I2C_READ_UUID = 0x300F; 00043 00044 static const uint16_t KONASHI_UART_CONFIG_UUID = 0x3010; 00045 static const uint16_t KONASHI_UART_BAUDRATE_UUID = 0x3011; 00046 static const uint16_t KONASHI_UART_TX_UUID = 0x3012; 00047 static const uint16_t KONASHI_UART_RX_NOTIFICATION_UUID = 0x3013; 00048 00049 static const uint16_t KONASHI_HARDWARE_RESET_UUID = 0x3014; 00050 static const uint16_t KONASHI_HARDWARE_LOW_BAT_NOTIFICATION_UUID = 0x3015; 00051 00052 uint16_t uuid16_list[] = { KONASHI_SERVICE_UUID }; 00053 00054 #define NEED_CONSOLE_OUTPUT 0 /* Set this if you need debug messages on the console; 00055 * it will have an impact on code-size and power consumption. */ 00056 00057 #if NEED_CONSOLE_OUTPUT 00058 Serial pc(USBTX, USBRX); 00059 #define DEBUG(...) { pc.printf(__VA_ARGS__); } 00060 #else 00061 #define DEBUG(...) /* nothing */ 00062 #endif /* #if NEED_CONSOLE_OUTPUT */ 00063 00064 //Serial pc(USBTX, USBRX); 00065 BLEDevice ble; 00066 DigitalOut led1(LED1); 00067 DigitalOut led2(LED2); 00068 00069 BusOut ioOut(P0_0,P0_1,P0_2,P0_3,P0_4,P0_5,P0_6,P0_7); 00070 uint8_t pioSetting[20] = {0,}; 00071 uint8_t pioPullup[20] = {0,}; 00072 uint8_t pioOutput[20] = {0,}; 00073 uint8_t pioInput[20] = {0,}; 00074 00075 uint8_t pwmConfig[20] = {0,}; 00076 uint8_t pwmPeriod[20] = {0,}; 00077 uint8_t pwmDuty[20] = {0,}; 00078 static uint32_t pwm_period[3] = {20000,20000,20000}; 00079 static uint32_t pwm_duty[3] = {0,0,0}; 00080 static uint8_t pwm_config = 0; 00081 PwmOut pwm[3] = {P0_28, P0_29, P0_30}; 00082 00083 uint8_t analogDrive[20] = {0,}; 00084 uint8_t analogRead0[20] = {0,}; 00085 uint8_t analogRead1[20] = {0,}; 00086 uint8_t analogRead2[20] = {0,}; 00087 00088 uint8_t i2cConfig[20] = {0,}; 00089 uint8_t i2cStartStop[20] = {0,}; 00090 uint8_t i2cWrite[20] = {0,}; 00091 uint8_t i2cReadParam[20] = {0,}; 00092 uint8_t i2cRead[20] = {0,}; 00093 00094 uint8_t uartConfig[20] = {0,}; 00095 uint8_t uartBaudrate[20] = {0,}; 00096 uint8_t uartTx[20] = {0,}; 00097 uint8_t uartRx[20] = {0,}; 00098 00099 uint8_t hardwareRest[20] = {0,}; 00100 uint8_t hardwareLowBat[20] = {0,}; 00101 00102 GattCharacteristic pioSettingCharacteristic (KONASHI_PIO_SETTING_UUID, pioSetting, 1, 1, 00103 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ | 00104 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_WRITE | 00105 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_WRITE_WITHOUT_RESPONSE); 00106 GattCharacteristic pioPullupCharacteristic (KONASHI_PIO_PULLUP_UUID, pioPullup, 1, 1, 00107 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ | 00108 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_WRITE | 00109 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_WRITE_WITHOUT_RESPONSE); 00110 GattCharacteristic pioOutputCharacteristic (KONASHI_PIO_OUTPUT_UUID, pioOutput, 1, 1, 00111 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_WRITE | 00112 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_WRITE_WITHOUT_RESPONSE); 00113 GattCharacteristic pioInputCharacteristic (KONASHI_PIO_INPUT_NOTIFICATION_UUID, pioInput, 1, 1, 00114 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ | 00115 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_NOTIFY); 00116 00117 GattCharacteristic pwmConfigCharacteristic (KONASHI_PWM_CONFIG_UUID, pwmConfig, 1, 1, 00118 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ | 00119 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_WRITE | 00120 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_WRITE_WITHOUT_RESPONSE); 00121 GattCharacteristic pwmPeriodCharacteristic (KONASHI_PWM_PARAM_UUID, pwmPeriod, 5, 5, 00122 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ | 00123 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_WRITE | 00124 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_WRITE_WITHOUT_RESPONSE); 00125 GattCharacteristic pwmDutyCharacteristic (KONASHI_PWM_DUTY_UUID, pwmDuty, 5, 5, 00126 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ | 00127 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_WRITE | 00128 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_WRITE_WITHOUT_RESPONSE); 00129 00130 GattCharacteristic analogDriveCharacteristic (KONASHI_ANALOG_DRIVE_UUID, analogDrive, 1, 1, 00131 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ | 00132 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_WRITE | 00133 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_WRITE_WITHOUT_RESPONSE); 00134 GattCharacteristic analogRead0Characteristic (KONASHI_ANALOG_READ0_UUID, analogRead0, 2, 2, 00135 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ); 00136 GattCharacteristic analogRead1Characteristic (KONASHI_ANALOG_READ1_UUID, analogRead1, 2, 2, 00137 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ); 00138 GattCharacteristic analogRead2Characteristic (KONASHI_ANALOG_READ2_UUID, analogRead2, 2, 2, 00139 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ); 00140 00141 GattCharacteristic i2cConfigCharacteristic (KONASHI_I2C_CONFIG_UUID, i2cConfig, 1, 1, 00142 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ | 00143 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_WRITE | 00144 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_WRITE_WITHOUT_RESPONSE); 00145 GattCharacteristic i2cStartStopCharacteristic (KONASHI_I2C_START_STOP_UUID, i2cStartStop, 1, 1, 00146 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ | 00147 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_WRITE | 00148 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_WRITE_WITHOUT_RESPONSE); 00149 GattCharacteristic i2cWriteCharacteristic (KONASHI_I2C_WRITE_UUID, i2cWrite, 3, 20, 00150 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_WRITE | 00151 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_WRITE_WITHOUT_RESPONSE); 00152 GattCharacteristic i2cReadParamCharacteristic (KONASHI_I2C_READ_PARAM_UIUD, i2cReadParam, 2, 2, 00153 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_WRITE | 00154 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_WRITE_WITHOUT_RESPONSE); 00155 GattCharacteristic i2cReadCharacteristic (KONASHI_I2C_READ_UUID, i2cRead, 0, 20, 00156 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ); 00157 00158 GattCharacteristic uartConfigCharacteristic (KONASHI_UART_CONFIG_UUID, uartConfig, 1, 1, 00159 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ | 00160 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_WRITE | 00161 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_WRITE_WITHOUT_RESPONSE); 00162 GattCharacteristic uartBaudrateCharacteristic (KONASHI_UART_BAUDRATE_UUID, uartBaudrate, 1, 1, 00163 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ | 00164 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_WRITE | 00165 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_WRITE_WITHOUT_RESPONSE); 00166 GattCharacteristic uartTxCharacteristic (KONASHI_UART_TX_UUID, uartTx, 1, 1, 00167 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ | 00168 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_WRITE | 00169 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_WRITE_WITHOUT_RESPONSE); 00170 GattCharacteristic uartRxCharacteristic (KONASHI_UART_RX_NOTIFICATION_UUID, uartRx, 0, 20, 00171 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ | 00172 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_NOTIFY); 00173 00174 GattCharacteristic hardwareRestCharacteristic (KONASHI_HARDWARE_RESET_UUID, hardwareRest, 1, 1, 00175 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_WRITE | 00176 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_WRITE_WITHOUT_RESPONSE); 00177 GattCharacteristic hardwareLowBatCharacteristic (KONASHI_HARDWARE_LOW_BAT_NOTIFICATION_UUID, hardwareLowBat, 1, 1, 00178 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ | 00179 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_NOTIFY); 00180 00181 GattCharacteristic *konashiChars[] = {// &pioSettingCharacteristic, 00182 // &pioPullupCharacteristic, 00183 &pioOutputCharacteristic, 00184 &pioInputCharacteristic, 00185 &pwmConfigCharacteristic, 00186 &pwmPeriodCharacteristic, 00187 &pwmDutyCharacteristic, 00188 /* &analogDriveCharacteristic, 00189 &analogRead0Characteristic, 00190 &analogRead1Characteristic, 00191 &analogRead2Characteristic, 00192 &i2cConfigCharacteristic, 00193 &i2cStartStopCharacteristic, 00194 &i2cWriteCharacteristic, 00195 &i2cReadParamCharacteristic, 00196 &i2cReadCharacteristic, 00197 &uartConfigCharacteristic, 00198 &uartBaudrateCharacteristic, 00199 &uartTxCharacteristic, 00200 &uartRxCharacteristic, 00201 &hardwareRestCharacteristic, 00202 &hardwareLowBatCharacteristic,*/ }; 00203 GattService konashiService(KONASHI_SERVICE_UUID, konashiChars, sizeof(konashiChars) / sizeof(GattCharacteristic *)); 00204 00205 GattCharacteristic *konashiChars1[] = {&pioOutputCharacteristic, &uartTxCharacteristic, &uartRxCharacteristic}; 00206 GattService konashiService1(KONASHI_SERVICE_UUID, konashiChars1, sizeof(konashiChars1) / sizeof(GattCharacteristic *)); 00207 00208 00209 // SYSTEM 00210 static char systemId = 'A'; 00211 GattCharacteristic systemID(GattCharacteristic::UUID_SYSTEM_ID_CHAR, (uint8_t *)systemId, sizeof(systemId), sizeof(systemId), 00212 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ); 00213 // MODEL 00214 static char model[31] = "mbed HRM1017"; 00215 GattCharacteristic modelID(GattCharacteristic::UUID_MODEL_NUMBER_STRING_CHAR, (uint8_t *)model, strlen(model), strlen(model), 00216 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ); 00217 // Firmware 00218 static char fwversion[31] = "1.0"; 00219 GattCharacteristic fwChars(GattCharacteristic::UUID_FIRMWARE_REVISION_STRING_CHAR, (uint8_t *)fwversion, strlen(fwversion), strlen(fwversion), 00220 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ); 00221 // Software 00222 static char swversion[31] = "1.0"; 00223 GattCharacteristic swChars(GattCharacteristic::UUID_SOFTWARE_REVISION_STRING_CHAR, (uint8_t *)swversion, strlen(swversion), strlen(swversion), 00224 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ); 00225 // Hardware 00226 static char hwversion[31] = "1.0"; 00227 GattCharacteristic hwChars(GattCharacteristic::UUID_HARDWARE_REVISION_STRING_CHAR, (uint8_t *)hwversion, strlen(hwversion), strlen(hwversion), 00228 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ); 00229 // Manufacturer 00230 static char vendor[31] = "Test Company Inc."; 00231 GattCharacteristic vendorChars(GattCharacteristic::UUID_MANUFACTURER_NAME_STRING_CHAR, (uint8_t *)vendor, strlen(vendor), strlen(vendor), 00232 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ); 00233 // Serial number 00234 static char serial[31] = "1234567890"; 00235 GattCharacteristic serialChars(GattCharacteristic::UUID_SERIAL_NUMBER_STRING_CHAR, (uint8_t *)serial, strlen(serial), strlen(serial), 00236 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ); 00237 00238 //GattCharacteristic *informationChars[] = {&systemID, &modelID, &serialChars, &fwChars, &hwChars, &swChars, &vendorChars, }; 00239 GattCharacteristic *informationChars[] = {&modelID, &fwChars, &hwChars, &swChars, &vendorChars, }; 00240 GattService informationService(GattService::UUID_DEVICE_INFORMATION_SERVICE, informationChars, sizeof(informationChars) / sizeof(GattCharacteristic *)); 00241 00242 00243 static uint8_t batteryLevel = 100; 00244 GattCharacteristic batteryPercentage(GattCharacteristic::UUID_BATTERY_LEVEL_CHAR, (uint8_t *)batteryLevel, sizeof(batteryLevel), sizeof(batteryLevel), 00245 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_NOTIFY); 00246 GattCharacteristic *batteryChars[] = {&batteryPercentage }; 00247 GattService batteryService(GattService::UUID_BATTERY_SERVICE, batteryChars, sizeof(batteryChars) / sizeof(GattCharacteristic *)); 00248 00249 void connectionCallback(uint16_t charHandle) 00250 //void connectionCallback(void) 00251 { 00252 DEBUG("Connected!\n\r"); 00253 led2 = 0; 00254 } 00255 void disconnectionCallback(uint16_t charHandle) 00256 //void disconnectionCallback(void) 00257 { 00258 DEBUG("Disconnected!\n\r"); 00259 DEBUG("Restarting the advertising process\n\r"); 00260 led2 = 1; 00261 ble.startAdvertising(); 00262 } 00263 static void set_pwm_duty(int ch) 00264 { 00265 // uint32_t period; 00266 uint32_t duty; 00267 unsigned bitmask = 1; 00268 00269 if(ch>=3){ 00270 return; 00271 } 00272 duty=pwm_duty[ch]; 00273 00274 if(pwm_config & (bitmask << ch)) 00275 { 00276 pwm[ch].pulsewidth_us(duty); 00277 } 00278 else 00279 { 00280 pwm[ch].pulsewidth_us(0); 00281 } 00282 } 00283 static void set_pwm_period(int ch) 00284 { 00285 uint32_t period,duty; 00286 00287 if(ch>=3){ 00288 return; 00289 } 00290 period=pwm_period[ch]; 00291 duty=pwm_duty[ch]; 00292 pwm[ch].period_us(period); 00293 pwm[ch].pulsewidth_us(duty); 00294 } 00295 static void set_pwm_config(uint8_t config) 00296 { 00297 pwm_config = config; 00298 for(int i = 0; i < 3 ; i++) 00299 { 00300 set_pwm_duty(i); 00301 } 00302 00303 } 00304 void onDataWritten(uint16_t charHandle) 00305 { 00306 DEBUG("onDataWritten\n\r"); 00307 if (charHandle == pioOutputCharacteristic.getHandle()) { 00308 DEBUG("pioOutputCharacteristic!\n\r"); 00309 uint8_t getPioOut[10]; 00310 uint16_t bytesRead; 00311 ble.readCharacteristicValue(pioOutputCharacteristic.getHandle(), getPioOut, &bytesRead); 00312 DEBUG("DATA: %d %d\n\r",getPioOut[0],pioOutput[0]); 00313 if(getPioOut[0]!=pioOutput[0]) { 00314 pioOutput[0]=getPioOut[0]; 00315 ioOut = getPioOut[0]; 00316 } 00317 /* } else if (charHandle == pioSettingCharacteristic.getHandle()) { 00318 DEBUG("pioSettingCharacteristic!\n\r"); 00319 } else if (charHandle == pioPullupCharacteristic.getHandle()) { 00320 DEBUG("pioPullupCharacteristic!\n\r");*/ 00321 } else if (charHandle == pwmConfigCharacteristic.getHandle()) { 00322 DEBUG("pwmConfigCharacteristic!\n\r"); 00323 uint16_t bytesRead; 00324 ble.readCharacteristicValue(pwmConfigCharacteristic.getHandle(), pwmConfig, &bytesRead); 00325 set_pwm_config(pwmConfig[0]); 00326 } else if (charHandle == pwmPeriodCharacteristic.getHandle()) { 00327 DEBUG("pwmPeriodCharacteristic!\n\r"); 00328 uint32_t l; 00329 uint16_t bytesRead; 00330 ble.readCharacteristicValue(pwmPeriodCharacteristic.getHandle(), pwmPeriod, &bytesRead); 00331 int i=pwmPeriod[0]; 00332 l =((uint32_t)pwmPeriod[1])<<24; 00333 l|=((uint32_t)pwmPeriod[2])<<16; 00334 l|=((uint32_t)pwmPeriod[3])<< 8; 00335 l|=((uint32_t)pwmPeriod[4])<< 0; 00336 if(i<3){ 00337 pwmPeriod[i]=l; 00338 set_pwm_period(i); 00339 } 00340 } else if (charHandle == pwmDutyCharacteristic.getHandle()) { 00341 DEBUG("pwmDutyCharacteristic!\n\r"); 00342 uint32_t l; 00343 uint16_t bytesRead; 00344 ble.readCharacteristicValue(pwmDutyCharacteristic.getHandle(), pwmDuty, &bytesRead); 00345 int i=pwmDuty[0]; 00346 l =((uint32_t)pwmDuty[1])<<24; 00347 l|=((uint32_t)pwmDuty[2])<<16; 00348 l|=((uint32_t)pwmDuty[3])<< 8; 00349 l|=((uint32_t)pwmDuty[4])<< 0; 00350 if(i<3){ 00351 pwm_duty[i]=l; 00352 set_pwm_duty(i); 00353 } 00354 /* } else if (charHandle == analogDriveCharacteristic.getHandle()) { 00355 DEBUG("analogDriveCharacteristic!\n\r"); 00356 } else if (charHandle == i2cConfigCharacteristic.getHandle()) { 00357 DEBUG("i2cConfigCharacteristic!\n\r"); 00358 } else if (charHandle == i2cStartStopCharacteristic.getHandle()) { 00359 DEBUG("pioSettingCharacteristic!\n\r"); 00360 } else if (charHandle == i2cWriteCharacteristic.getHandle()) { 00361 DEBUG("i2cStartStopCharacteristic!\n\r"); 00362 } else if (charHandle == i2cReadParamCharacteristic.getHandle()) { 00363 DEBUG("i2cReadParamCharacteristic!\n\r"); 00364 } else if (charHandle == uartConfigCharacteristic.getHandle()) { 00365 DEBUG("uartConfigCharacteristic!\n\r"); 00366 } else if (charHandle == uartBaudrateCharacteristic.getHandle()) { 00367 DEBUG("uartBaudrateCharacteristic!\n\r"); 00368 } else if (charHandle == uartTxCharacteristic.getHandle()) { 00369 DEBUG("uartTxCharacteristic!\n\r"); 00370 uint16_t bytesRead; 00371 ble.readCharacteristicValue(uartTxCharacteristic.getHandle(), uartTx, &bytesRead); 00372 DEBUG("ECHO: %s\n\r", (char *)uartTx); 00373 // pc.putc(uartTx[0]); 00374 ble.updateCharacteristicValue(uartRxCharacteristic.getHandle(), uartTx, bytesRead); 00375 } else if (charHandle == hardwareRestCharacteristic.getHandle()) { 00376 DEBUG("hardwareRestCharacteristic!\n\r");*/ 00377 } 00378 } 00379 00380 void periodicCallback(void) 00381 { 00382 led1 = !led1; /* Do blinky on LED1 while we're waiting for BLE events */ 00383 if (ble.getGapState().connected) { 00384 /* Update the battery measurement */ 00385 batteryLevel--; 00386 if (batteryLevel == 1) { 00387 batteryLevel = 100; 00388 } 00389 // ble.updateCharacteristicValue(batteryPercentage.getHandle(), &batteryLevel, sizeof(batteryLevel)); 00390 // ble.updateCharacteristicValue(hardwareLowBatCharacteristic.getHandle(), &batteryLevel, sizeof(batteryLevel)); 00391 /* if(pc.readable()) { 00392 char data; 00393 data = pc.getc(); 00394 ble.updateCharacteristicValue(uartRxCharacteristic.getHandle(), (uint8_t*)&data, 1); 00395 }*/ 00396 } 00397 } 00398 00399 int main(void) 00400 { 00401 led1 = 1; 00402 led2 = 1; 00403 Ticker ticker; 00404 ticker.attach(periodicCallback, 1); 00405 00406 DEBUG("Initialising the nRF51822\n\r"); 00407 ble.init(); 00408 ble.onConnection(connectionCallback); 00409 ble.onDisconnection(disconnectionCallback); 00410 ble.onDataWritten(onDataWritten); 00411 00412 /* setup advertising */ 00413 ble.accumulateAdvertisingPayload(GapAdvertisingData::BREDR_NOT_SUPPORTED); 00414 ble.accumulateAdvertisingPayload(GapAdvertisingData::COMPLETE_LOCAL_NAME, (uint8_t *)DEVICE_NAME, sizeof(DEVICE_NAME)); 00415 ble.setAdvertisingType(GapAdvertisingParams::ADV_CONNECTABLE_UNDIRECTED); 00416 00417 ble.setAdvertisingInterval(160); /* 100ms; in multiples of 0.625ms. */ 00418 ble.startAdvertising(); 00419 00420 // ble.addService(batteryService); 00421 ble.addService(informationService); 00422 // ble.addService(konashiService1); 00423 ble.addService(konashiService); 00424 00425 while (true) { 00426 ble.waitForEvent(); 00427 } 00428 }
Generated on Tue Jul 12 2022 20:35:46 by
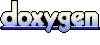