
STM32F7 Ethernet interface for nucleo STM32F767
lwip_api_lib.c File Reference
Sequential API External module. More...
Go to the source code of this file.
Functions | |
static err_t | netconn_close_shutdown (struct netconn *conn, u8_t how) |
Close or shutdown a TCP netconn (doesn't delete it). | |
static err_t | netconn_apimsg (tcpip_callback_fn fn, struct api_msg *apimsg) |
Call the lower part of a netconn_* function This function is then running in the thread context of tcpip_thread and has exclusive access to lwIP core code. | |
struct netconn * | netconn_new_with_proto_and_callback (enum netconn_type t, u8_t proto, netconn_callback callback) |
Create a new netconn (of a specific type) that has a callback function. | |
err_t | netconn_delete (struct netconn *conn) |
Close a netconn 'connection' and free its resources. | |
err_t | netconn_getaddr (struct netconn *conn, ip_addr_t *addr, u16_t *port, u8_t local) |
Get the local or remote IP address and port of a netconn. | |
err_t | netconn_bind (struct netconn *conn, const ip_addr_t *addr, u16_t port) |
Bind a netconn to a specific local IP address and port. | |
err_t | netconn_connect (struct netconn *conn, const ip_addr_t *addr, u16_t port) |
Connect a netconn to a specific remote IP address and port. | |
err_t | netconn_disconnect (struct netconn *conn) |
Disconnect a netconn from its current peer (only valid for UDP netconns). | |
err_t | netconn_listen_with_backlog (struct netconn *conn, u8_t backlog) |
Set a TCP netconn into listen mode. | |
err_t | netconn_accept (struct netconn *conn, struct netconn **new_conn) |
Accept a new connection on a TCP listening netconn. | |
static err_t | netconn_recv_data (struct netconn *conn, void **new_buf) |
Receive data: actual implementation that doesn't care whether pbuf or netbuf is received. | |
err_t | netconn_recv_tcp_pbuf (struct netconn *conn, struct pbuf **new_buf) |
Receive data (in form of a pbuf) from a TCP netconn. | |
err_t | netconn_recv (struct netconn *conn, struct netbuf **new_buf) |
Receive data (in form of a netbuf containing a packet buffer) from a netconn. | |
err_t | netconn_sendto (struct netconn *conn, struct netbuf *buf, const ip_addr_t *addr, u16_t port) |
Send data (in form of a netbuf) to a specific remote IP address and port. | |
err_t | netconn_send (struct netconn *conn, struct netbuf *buf) |
Send data over a UDP or RAW netconn (that is already connected). | |
err_t | netconn_write_partly (struct netconn *conn, const void *dataptr, size_t size, u8_t apiflags, size_t *bytes_written) |
Send data over a TCP netconn. | |
err_t | netconn_close (struct netconn *conn) |
Close a TCP netconn (doesn't delete it). | |
err_t | netconn_shutdown (struct netconn *conn, u8_t shut_rx, u8_t shut_tx) |
Shut down one or both sides of a TCP netconn (doesn't delete it). | |
err_t | netconn_join_leave_group (struct netconn *conn, const ip_addr_t *multiaddr, const ip_addr_t *netif_addr, enum netconn_igmp join_or_leave) |
Join multicast groups for UDP netconns. | |
err_t | netconn_gethostbyname_addrtype (const char *name, ip_addr_t *addr, u8_t dns_addrtype) err_t netconn_gethostbyname(const char *name |
Execute a DNS query, only one IP address is returned. |
Detailed Description
Sequential API External module.
Definition in file lwip_api_lib.c.
Function Documentation
static err_t netconn_apimsg | ( | tcpip_callback_fn | fn, |
struct api_msg * | apimsg | ||
) | [static] |
Call the lower part of a netconn_* function This function is then running in the thread context of tcpip_thread and has exclusive access to lwIP core code.
- Parameters:
-
fn function to call apimsg a struct containing the function to call and its parameters
- Returns:
- ERR_OK if the function was called, another err_t if not
Definition at line 92 of file lwip_api_lib.c.
Get the local or remote IP address and port of a netconn.
For RAW netconns, this returns the protocol instead of a port!
- Parameters:
-
conn the netconn to query addr a pointer to which to save the IP address port a pointer to which to save the port (or protocol for RAW) local 1 to get the local IP address, 0 to get the remote one
- Returns:
- ERR_CONN for invalid connections ERR_OK if the information was retrieved
Definition at line 212 of file lwip_api_lib.c.
struct netconn* netconn_new_with_proto_and_callback | ( | enum netconn_type | t, |
u8_t | proto, | ||
netconn_callback | callback | ||
) | [read] |
Create a new netconn (of a specific type) that has a callback function.
The corresponding pcb is also created.
- Parameters:
-
t the type of 'connection' to create (
- See also:
- enum netconn_type)
- Parameters:
-
proto the IP protocol for RAW IP pcbs callback a function to call on status changes (RX available, TX'ed)
- Returns:
- a newly allocated struct netconn or NULL on memory error
Definition at line 123 of file lwip_api_lib.c.
Generated on Tue Jul 12 2022 14:49:26 by
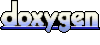