
STM32F7 Ethernet interface for nucleo STM32F767
Common functions
[Netconn API]
For use with TCP and UDP. More...
Enumerations | |
enum | netconn_type { , NETCONN_TCP = 0x10, NETCONN_TCP_IPV6 = NETCONN_TCP | NETCONN_TYPE_IPV6, NETCONN_UDP = 0x20, NETCONN_UDPLITE = 0x21, NETCONN_UDPNOCHKSUM = 0x22, NETCONN_UDP_IPV6 = NETCONN_UDP | NETCONN_TYPE_IPV6, NETCONN_UDPLITE_IPV6 = NETCONN_UDPLITE | NETCONN_TYPE_IPV6, NETCONN_UDPNOCHKSUM_IPV6 = NETCONN_UDPNOCHKSUM | NETCONN_TYPE_IPV6, NETCONN_RAW } |
Protocol family and type of the netconn. More... | |
Functions | |
err_t | netconn_delete (struct netconn *conn) |
Close a netconn 'connection' and free its resources. | |
err_t | netconn_bind (struct netconn *conn, const ip_addr_t *addr, u16_t port) |
Bind a netconn to a specific local IP address and port. | |
err_t | netconn_connect (struct netconn *conn, const ip_addr_t *addr, u16_t port) |
Connect a netconn to a specific remote IP address and port. | |
static err_t | netconn_recv_data (struct netconn *conn, void **new_buf) |
Receive data: actual implementation that doesn't care whether pbuf or netbuf is received. | |
err_t | netconn_recv (struct netconn *conn, struct netbuf **new_buf) |
Receive data (in form of a netbuf containing a packet buffer) from a netconn. | |
err_t | netconn_gethostbyname_addrtype (const char *name, ip_addr_t *addr, u8_t dns_addrtype) err_t netconn_gethostbyname(const char *name |
Execute a DNS query, only one IP address is returned. |
Detailed Description
For use with TCP and UDP.
Enumeration Type Documentation
enum netconn_type |
Protocol family and type of the netconn.
- Enumerator:
Function Documentation
Bind a netconn to a specific local IP address and port.
Binding one netconn twice might not always be checked correctly!
- Parameters:
-
conn the netconn to bind addr the local IP address to bind the netconn to (use IP4_ADDR_ANY/IP6_ADDR_ANY to bind to all addresses) port the local port to bind the netconn to (not used for RAW)
- Returns:
- ERR_OK if bound, any other err_t on failure
Definition at line 250 of file lwip_api_lib.c.
Connect a netconn to a specific remote IP address and port.
- Parameters:
-
conn the netconn to connect addr the remote IP address to connect to port the remote port to connect to (no used for RAW)
- Returns:
- ERR_OK if connected, return value of tcp_/udp_/raw_connect otherwise
Definition at line 294 of file lwip_api_lib.c.
Close a netconn 'connection' and free its resources.
UDP and RAW connection are completely closed, TCP pcbs might still be in a waitstate after this returns.
- Parameters:
-
conn the netconn to delete
- Returns:
- ERR_OK if the connection was deleted
Definition at line 166 of file lwip_api_lib.c.
err_t netconn_gethostbyname_addrtype | ( | const char * | name, |
ip_addr_t * | addr, | ||
u8_t | dns_addrtype | ||
) | const |
Execute a DNS query, only one IP address is returned.
- Parameters:
-
name a string representation of the DNS host name to query addr a preallocated ip_addr_t where to store the resolved IP address dns_addrtype IP address type (IPv4 / IPv6)
- Returns:
- ERR_OK: resolving succeeded ERR_MEM: memory error, try again later ERR_ARG: dns client not initialized or invalid hostname ERR_VAL: dns server response was invalid
Receive data (in form of a netbuf containing a packet buffer) from a netconn.
- Parameters:
-
conn the netconn from which to receive data new_buf pointer where a new netbuf is stored when received data
- Returns:
- ERR_OK if data has been received, an error code otherwise (timeout, memory error or another error)
Definition at line 621 of file lwip_api_lib.c.
Receive data: actual implementation that doesn't care whether pbuf or netbuf is received.
- Parameters:
-
conn the netconn from which to receive data new_buf pointer where a new pbuf/netbuf is stored when received data
- Returns:
- ERR_OK if data has been received, an error code otherwise (timeout, memory error or another error)
Definition at line 476 of file lwip_api_lib.c.
Generated on Tue Jul 12 2022 14:49:32 by
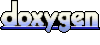