
STM32F7 Ethernet interface for nucleo STM32F767
TCP only
[Netconn API]
TCP only functions. More...
Functions | |
err_t | netconn_listen_with_backlog (struct netconn *conn, u8_t backlog) |
Set a TCP netconn into listen mode. | |
err_t | netconn_accept (struct netconn *conn, struct netconn **new_conn) |
Accept a new connection on a TCP listening netconn. | |
err_t | netconn_recv_tcp_pbuf (struct netconn *conn, struct pbuf **new_buf) |
Receive data (in form of a pbuf) from a TCP netconn. | |
err_t | netconn_write_partly (struct netconn *conn, const void *dataptr, size_t size, u8_t apiflags, size_t *bytes_written) |
Send data over a TCP netconn. | |
static err_t | netconn_close_shutdown (struct netconn *conn, u8_t how) |
Close or shutdown a TCP netconn (doesn't delete it). | |
err_t | netconn_close (struct netconn *conn) |
Close a TCP netconn (doesn't delete it). | |
err_t | netconn_shutdown (struct netconn *conn, u8_t shut_rx, u8_t shut_tx) |
Shut down one or both sides of a TCP netconn (doesn't delete it). |
Detailed Description
TCP only functions.
Function Documentation
Accept a new connection on a TCP listening netconn.
- Parameters:
-
conn the TCP listen netconn new_conn pointer where the new connection is stored
- Returns:
- ERR_OK if a new connection has been received or an error code otherwise
Definition at line 388 of file lwip_api_lib.c.
Close a TCP netconn (doesn't delete it).
- Parameters:
-
conn the TCP netconn to close
- Returns:
- ERR_OK if the netconn was closed, any other err_t on error
Definition at line 838 of file lwip_api_lib.c.
Close or shutdown a TCP netconn (doesn't delete it).
- Parameters:
-
conn the TCP netconn to close or shutdown how fully close or only shutdown one side?
- Returns:
- ERR_OK if the netconn was closed, any other err_t on error
Definition at line 802 of file lwip_api_lib.c.
Set a TCP netconn into listen mode.
- Parameters:
-
conn the tcp netconn to set to listen mode backlog the listen backlog, only used if TCP_LISTEN_BACKLOG==1
- Returns:
- ERR_OK if the netconn was set to listen (UDP and RAW netconns don't return any error (yet?))
Definition at line 351 of file lwip_api_lib.c.
Receive data (in form of a pbuf) from a TCP netconn.
- Parameters:
-
conn the netconn from which to receive data new_buf pointer where a new pbuf is stored when received data
- Returns:
- ERR_OK if data has been received, an error code otherwise (timeout, memory error or another error) ERR_ARG if conn is not a TCP netconn
Definition at line 603 of file lwip_api_lib.c.
Shut down one or both sides of a TCP netconn (doesn't delete it).
- Parameters:
-
conn the TCP netconn to shut down shut_rx shut down the RX side (no more read possible after this) shut_tx shut down the TX side (no more write possible after this)
- Returns:
- ERR_OK if the netconn was closed, any other err_t on error
Definition at line 854 of file lwip_api_lib.c.
err_t netconn_write_partly | ( | struct netconn * | conn, |
const void * | dataptr, | ||
size_t | size, | ||
u8_t | apiflags, | ||
size_t * | bytes_written | ||
) |
Send data over a TCP netconn.
- Parameters:
-
conn the TCP netconn over which to send data dataptr pointer to the application buffer that contains the data to send size size of the application data to send apiflags combination of following flags : - NETCONN_COPY: data will be copied into memory belonging to the stack
- NETCONN_MORE: for TCP connection, PSH flag will be set on last segment sent
- NETCONN_DONTBLOCK: only write the data if all data can be written at once
bytes_written pointer to a location that receives the number of written bytes
- Returns:
- ERR_OK if data was sent, any other err_t on error
Definition at line 735 of file lwip_api_lib.c.
Generated on Tue Jul 12 2022 14:49:32 by
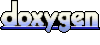