library for C++ CANOpen implementation. mbed independant, but is easy to attach into with mbed.
Dependents: ppCANOpen_Example DISCO-F746NG_rtos_test
ServiceProvider.h
00001 /** 00002 ****************************************************************************** 00003 * @file 00004 * @author Paul Paterson 00005 * @version 00006 * @date 2015-12-22 00007 * @brief CANOpen implementation library 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT(c) 2015 Paul Paterson 00012 * 00013 * All rights reserved. 00014 00015 This program is free software: you can redistribute it and/or modify 00016 it under the terms of the GNU General Public License as published by 00017 the Free Software Foundation, either version 3 of the License, or 00018 (at your option) any later version. 00019 00020 This program is distributed in the hope that it will be useful, 00021 but WITHOUT ANY WARRANTY; without even the implied warranty of 00022 MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00023 GNU General Public License for more details. 00024 00025 You should have received a copy of the GNU General Public License 00026 along with this program. If not, see <http://www.gnu.org/licenses/>. 00027 */ 00028 00029 #ifndef PPCAN_SERVICE_PROVIDER_H 00030 #define PPCAN_SERVICE_PROVIDER_H 00031 00032 #include "CanOpenMessage.h" 00033 #include <queue> 00034 00035 /*========================================================================= 00036 * Forward declarations 00037 *========================================================================= 00038 */ 00039 00040 struct CanOpenHandle; 00041 00042 namespace ppCANOpen 00043 { 00044 00045 /* Avoid circular reference */ 00046 class Node; 00047 00048 /** Node Class to implement feature of a CANOpen NMT node 00049 */ 00050 class ServiceProvider 00051 { 00052 public: 00053 00054 ServiceProvider (void); 00055 ~ServiceProvider (void); 00056 00057 /* ======================================================================== 00058 * Public Methods 00059 * ======================================================================== 00060 */ 00061 00062 /* Main application -----------------------------------------------------*/ 00063 /** Main loop */ 00064 void Run (void); 00065 00066 /** Iterate through Nodes and update */ 00067 void UpdateNodes(void); 00068 00069 /** C helper function to pass to API */ 00070 static void UpdateNodes_CWrapper(void *pServiceObject); 00071 00072 /* Node Management ------------------------------------------------------*/ 00073 /** Register a node to get messages and get update calls */ 00074 int AddNode (Node * node); 00075 00076 // TODO: remove node (swap pointers to fill in nicely 00077 00078 00079 /* CanMessage Transmission ----------------------------------------------*/ 00080 /** Add a message to the message queue outbox */ 00081 void PostMessage (int nodeId, CanOpenMessage * msg); 00082 00083 /** quick way to create and send an NMT Control message 00084 * @note Make posting a message easier! 00085 */ 00086 void PostNmtControl (char targetNodeId, NmtCommandSpecifier cs); 00087 00088 /* Elapsed Time ---------------------------------------------------------*/ 00089 /** A way for the the Nodes to get elapsed time when they want it */ 00090 uint32_t GetTime(void); 00091 00092 private: 00093 /* ======================================================================== 00094 * Private Constants 00095 * ======================================================================== 00096 */ 00097 00098 /** Define size of arrays in one place */ 00099 static const int SERVICE_MAX_NODES = 8; 00100 00101 /* ======================================================================== 00102 * Private Methods 00103 * ======================================================================== 00104 */ 00105 00106 /* CanMessage Reading ---------------------------------------------------*/ 00107 /** Function to be called on every CAN read interrupt */ 00108 void ReadIT(void); 00109 00110 /** C helper function used to pass ReadIT() to API */ 00111 static void ReadIT_CWrapper(void *pServiceObject); 00112 00113 /** Helper function that utilizes backup message inbox when the main one 00114 * is locked by the application. 00115 */ 00116 void ReadIT_clearBuffer(void); 00117 00118 /* Elapsed Time ---------------------------------------------------------*/ 00119 /** Synchronize elapsed time*/ 00120 void HandleTimeMessage(CanOpenMessage *canOpenMsg); 00121 00122 00123 /* ======================================================================== 00124 * Private Member Variables 00125 * ======================================================================== 00126 */ 00127 00128 /* Node Management -------------------------------------------------------*/ 00129 /** array of nodes to cycle through. */ 00130 Node * nodes[SERVICE_MAX_NODES]; 00131 00132 /** Keeps count of current number of nodes. */ 00133 int nodeCount; 00134 00135 /* CanMessage Reading ---------------------------------------------------*/ 00136 /** array of messages in a queue */ 00137 std::queue<CanOpenMessage> inbox; 00138 00139 /** Extra buffer only handled by ISR. Used if inbox is locked */ 00140 std::queue<CanOpenMessage> inboxBuffer; 00141 00142 /** boolean to lock and unlock inbox */ 00143 int bInboxUnlocked; 00144 00145 /** Data to keep track of internal messages. Node ID allows SP to avoid 00146 * dispatching message to the one who sent it 00147 */ 00148 struct InternalMessage { 00149 int nodeId; 00150 CanOpenMessage message; 00151 00152 InternalMessage(uint8_t n, CanOpenMessage m): 00153 nodeId(n), 00154 message(m) 00155 {} 00156 }; 00157 00158 /** array of messages in a queue */ 00159 std::queue<InternalMessage> outbox; 00160 00161 /* Elapsed Time ---------------------------------------------------------*/ 00162 /** Elapsed time offset used for synchronization*/ 00163 int32_t elapsedTimeOffset; 00164 00165 }; 00166 00167 } /* namspace ppCANOpen */ 00168 00169 #endif /* PPCAN_SERVICE_PROVIDER_H */ 00170
Generated on Sun Jul 17 2022 07:51:19 by
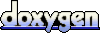