
cc
Fork of AS5048 by
As5048Spi Class Reference
Class for interfacing with the AMS AS5048A magnetic rotary sensor over the SPI-interface. More...
#include <as5048spi.h>
Public Member Functions | |
void | frequency (int frequency=1000000) |
Sets the SPI clock frequency in Hz. Maximum tested frequency is 10MHz. | |
const int * | read (As5048Command command) |
Sends a read command to the sensor. | |
const int * | read_sequential (As5048Command command) |
Sends a read command to the sensor. | |
const int * | read_angle () |
Performs a single angle measurement on all sensors. | |
const int * | read_angle_sequential () |
Performs sequential angle measurements on all sensors. | |
Static Public Member Functions | |
static int | mask (int sensor_result) |
Returns lowest 14-bits. | |
static void | mask (int *sensor_results, int n) |
Applies the mask to the first n bytes in the read buffer (for daisychained sensors). | |
static bool | parity_check (int sensor_result) |
Checks if the return value from the sensor has the right parity. | |
static int | degrees (int sensor_result) |
Returns an angle from 0 to 36000 (degrees times 100). | |
static int | radian (int sensor_result) |
Returns an angle from 0 to 2*PI*100. |
Detailed Description
Class for interfacing with the AMS AS5048A magnetic rotary sensor over the SPI-interface.
Definition at line 25 of file as5048spi.h.
Member Function Documentation
int degrees | ( | int | sensor_result ) | [static] |
Returns an angle from 0 to 36000 (degrees times 100).
- Parameters:
-
sensor_result is one of the values returned by read_angle or read_angle_sequential
Definition at line 28 of file as5048spi.cpp.
void frequency | ( | int | frequency = 1000000 ) |
Sets the SPI clock frequency in Hz. Maximum tested frequency is 10MHz.
Definition at line 54 of file as5048spi.cpp.
void mask | ( | int * | sensor_results, |
int | n | ||
) | [static] |
Applies the mask to the first n bytes in the read buffer (for daisychained sensors).
Definition at line 65 of file as5048spi.cpp.
int mask | ( | int | sensor_result ) | [static] |
Returns lowest 14-bits.
Definition at line 59 of file as5048spi.cpp.
bool parity_check | ( | int | sensor_result ) | [static] |
Checks if the return value from the sensor has the right parity.
- Returns:
- true if ok
Definition at line 73 of file as5048spi.cpp.
int radian | ( | int | sensor_result ) | [static] |
Returns an angle from 0 to 2*PI*100.
- Parameters:
-
sensor_result is one of the values returned by read_angle or read_angle_sequential
Definition at line 34 of file as5048spi.cpp.
const int * read | ( | As5048Command | command ) |
Sends a read command to the sensor.
Definition at line 86 of file as5048spi.cpp.
const int * read_angle | ( | ) |
Performs a single angle measurement on all sensors.
- Returns:
- Array of raw angle data. To get the 14-bit value representing the angle, apply the mask() to the result.
Definition at line 97 of file as5048spi.cpp.
const int * read_angle_sequential | ( | ) |
Performs sequential angle measurements on all sensors.
The first time this method is called the result is not usefull, the measurement data of the call will be returned by the next call to this method.
- Returns:
- Array of raw angle data. To get the 14-bit value representing the angle, apply the mask() to the result.
Definition at line 103 of file as5048spi.cpp.
const int * read_sequential | ( | As5048Command | command ) |
Sends a read command to the sensor.
A call to this function will not directly return the requested value. The requested value will be returned in a next read_sequential call. Use this function to read sensor values with minimum speed impact on SPI-bus and microcontroller.
Definition at line 92 of file as5048spi.cpp.
Generated on Wed Aug 10 2022 11:15:14 by
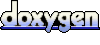