
cc
Fork of AS5048 by
Embed:
(wiki syntax)
Show/hide line numbers
as5048spi.cpp
00001 #include "as5048spi.h" 00002 00003 00004 As5048Spi::As5048Spi(PinName mosi, PinName miso, PinName sclk, int ndevices) : 00005 _nDevices(ndevices), 00006 _spi(mosi, miso, sclk) 00007 { 00008 00009 //chip select à 1 00010 00011 // AS5048 needs 16-bits for is commands 00012 // Mode = 1: 00013 // clock polarity = 0 --> clock pulse is high 00014 // clock phase = 1 --> sample on falling edge of clock pulse 00015 _spi.format(16, 1); 00016 00017 // Set clock frequency to 1 MHz (max is 10Mhz) 00018 _spi.frequency(1000000); 00019 00020 _readBuffer = new int[ndevices]; 00021 } 00022 00023 As5048Spi::~As5048Spi() 00024 { 00025 delete [] _readBuffer; 00026 } 00027 00028 int As5048Spi::degrees(int sensor_result) 00029 { 00030 return mask(sensor_result) * 36000 / 0x4000; 00031 } 00032 00033 00034 int As5048Spi::radian(int sensor_result) 00035 { 00036 return mask(sensor_result) * 62832 / 0x4000; 00037 } 00038 00039 bool As5048Spi::error(int device) 00040 { 00041 if( device == -1 ) { 00042 for(int i = 0; i < _nDevices; ++i) { 00043 if( _readBuffer[i] & 0x4000 ) { 00044 return true; 00045 } 00046 } 00047 } else if( device < _nDevices ) { 00048 return (_readBuffer[device] & 0x4000) == 0x4000; 00049 } 00050 return false; 00051 } 00052 00053 00054 void As5048Spi::frequency(int hz) 00055 { 00056 _spi.frequency(hz); 00057 } 00058 00059 int As5048Spi::mask(int sensor_result) 00060 { 00061 return sensor_result & 0x3FFF; // return lowest 14-bits 00062 } 00063 00064 00065 void As5048Spi::mask(int* sensor_results, int n) 00066 { 00067 for(int i = 0; i < n; ++i) { 00068 sensor_results[i] &= 0x3FFF; 00069 } 00070 } 00071 00072 00073 bool As5048Spi::parity_check(int sensor_result) 00074 { 00075 // Use the LSb of result to keep track of parity (0 = even, 1 = odd) 00076 int result = sensor_result; 00077 00078 for(int i = 1; i <= 15; ++i) { 00079 sensor_result >>= 1; 00080 result ^= sensor_result; 00081 } 00082 // Parity should be even 00083 return (result & 0x0001) == 0; 00084 } 00085 00086 const int* As5048Spi::read(As5048Command command) 00087 { 00088 _read(command); // Send command to device(s) 00089 return _read(AS_CMD_NOP); // Read-out device(s) 00090 } 00091 00092 const int* As5048Spi::read_sequential(As5048Command command) 00093 { 00094 return _read(command); 00095 } 00096 00097 const int* As5048Spi::read_angle() 00098 { 00099 _read(AS_CMD_ANGLE); // Send command to device(s) 00100 return _read(AS_CMD_NOP); // Read-out device(s) 00101 } 00102 00103 const int* As5048Spi::read_angle_sequential() 00104 { 00105 return _read(AS_CMD_ANGLE); 00106 } 00107 00108 00109 int* As5048Spi::_read(As5048Command command) 00110 { 00111 if(_nDevices == 1) 00112 { 00113 // Give command to start reading the angle 00114 //chip select à 0 00115 00116 wait_us(1); // Wait at least 350ns after chip select 00117 _readBuffer[0] = _spi.write(command); 00118 //chip select à 1 00119 00120 wait_us(1); // Wait at least 350ns after chip select 00121 } else 00122 { 00123 // Enable the sensor on the chain 00124 //chip select à 0 00125 00126 wait_us(1); // Wait at least 350ns after chip select 00127 for(int i = 0; i < _nDevices; ++i) 00128 { 00129 _readBuffer[i] = _spi.write(command); 00130 } 00131 //chip select a 1 00132 00133 wait_us(1); // Wait at least 350ns after chip select 00134 } 00135 return _readBuffer; 00136 } 00137 00138 00139 00140 00141 00142
Generated on Wed Aug 10 2022 11:15:14 by
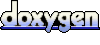