Add TFT commands
Fork of DigoleSerialDisp by
Embed:
(wiki syntax)
Show/hide line numbers
DigoleSerialDisp.h
00001 /** Digole Serial Display library, I2C 00002 * 00003 * @Author: Digole Digital Solutions : www.digole.com ported from Arduino to mbed by Michael Shimniok www.bot-thoughts.com 00004 */ 00005 #ifndef DigoleSerialDisp_h 00006 #define DigoleSerialDisp_h 00007 00008 #include "mbed.h" 00009 #include "rtos.h" 00010 #include <inttypes.h> 00011 00012 #define DEC 10 00013 #define HEX 16 00014 #define OCT 8 00015 #define BIN 2 00016 00017 #define delay(x) Thread::wait(x) 00018 00019 // Communication set up command 00020 // Text function command 00021 // Graph function command 00022 00023 #define Serial_UART 0; 00024 #define Serial_I2C 1; 00025 #define Serial_SPI 2; 00026 #define _TEXT_ 0 00027 #define _GRAPH_ 1 00028 00029 /** Digole Serial LCD/OLED Library 00030 * www.digole.com/index.php?productID=535 00031 * 00032 * Includes Arduino Print class member functions 00033 */ 00034 class DigoleSerialDisp { 00035 public: 00036 00037 /** Create a new Digole Serial Display interface 00038 * 00039 * @param sda is the pin for I2C SDA 00040 * @param scl is the pin for I2C SCL 00041 * @param address is the 7-bit address (default is 0x27 for the device) 00042 */ 00043 DigoleSerialDisp(PinName sda, PinName scl, uint8_t address=0x27); 00044 00045 00046 /** Carryover from Arduino library, not needed 00047 */ 00048 void begin(void) { } // nothing to do here 00049 00050 00051 /** Write out a raw character 00052 * @param x is the character to write 00053 * @returns 1 00054 */ 00055 size_t write(const char x); 00056 00057 00058 /** Write out raw data from a buffer 00059 * @param buffer is the char array to write 00060 * @param size is the the number of bytes to write 00061 * @returns size 00062 */ 00063 size_t write(const char *buffer, size_t size); 00064 00065 00066 /** Write out raw string 00067 * @param str is the string to write 00068 * @returns number of bytes written 00069 */ 00070 size_t write(const char *str); 00071 00072 00073 /** Prints a char to the display in a single I2C transmission using "TTb\0" 00074 * 00075 * @param c is the character to print 00076 * @returns 1 00077 */ 00078 size_t print(const char c); 00079 00080 00081 /** Prints a string of data to the display in a single I2C transmission using "TTbbb...\0" 00082 * 00083 * @param s is the null-terminated char array to print 00084 * @returns length of s 00085 */ 00086 size_t print(const char s[]); 00087 00088 00089 /** Print out an unsigned char as a number 00090 * @param u is the integer to print 00091 * @param base is the base to print, either DEC (default), HEX, BIN 00092 * @returns number of chars written 00093 */ 00094 size_t print(unsigned char u, int base = DEC); 00095 00096 00097 /** Print out an integer 00098 * @param i is the integer to print 00099 * @param base is the base to print, either DEC (default), HEX, BIN 00100 * @returns number of chars written 00101 */ 00102 size_t print(int i, int base = DEC); 00103 00104 00105 /** Print out an unsigned integer 00106 * @param u is the integer to print 00107 * @param base is the base to print, either DEC (default), HEX, BIN 00108 * @returns number of chars written 00109 */ 00110 size_t print(unsigned int u, int base = DEC); 00111 00112 00113 /** Print out a long as a number 00114 * @param l is the integer to print 00115 * @param base is the base to print, either DEC (default), HEX, BIN 00116 * @returns number of chars written 00117 */ 00118 size_t print(long l, int base = DEC); 00119 00120 00121 /** Print out an unsigned long 00122 * @param l is the integer to print 00123 * @param base is the base to print, either DEC (default), HEX, BIN 00124 * @returns number of chars written 00125 */ 00126 size_t print(unsigned long l, int base = DEC); 00127 00128 00129 /** Print out a double 00130 * @param f is the integer to print 00131 * @param digits is the number of digits after the decimal 00132 */ 00133 size_t print(double f, int digits = 2); 00134 00135 00136 /** Prints a string of data to the display in a single I2C transmission using "TTbbb...\0" 00137 * 00138 * @param s is the null-terminated char array to print 00139 * @returns length of s 00140 */ 00141 size_t println(const char s[]); 00142 00143 00144 /** Prints a char the display in a single I2C transmission using "TTb\0" 00145 * 00146 * @param c is the character to print 00147 * @returns 1 00148 */ 00149 size_t println(char c); 00150 00151 00152 /** Prints an unsigned char as a number 00153 * 00154 * @param u is the unsigned char number 00155 * @returns 1 00156 */ 00157 size_t println(unsigned char u, int base = DEC); 00158 00159 00160 /** Print out an integer 00161 * @param i is the integer to print 00162 * @param base is the base to print, either DEC (default), HEX, BIN 00163 * @returns number of chars written 00164 */ 00165 size_t println(int i, int base = DEC); 00166 00167 00168 /** Print out an unsigned char as a number 00169 * @param u is the integer to print 00170 * @param base is the base to print, either DEC (default), HEX, BIN 00171 * @returns number of chars written 00172 */ 00173 size_t println(unsigned int u, int base = DEC); 00174 00175 00176 /** Print out a long as a number 00177 * @param l is the integer to print 00178 * @param base is the base to print, either DEC (default), HEX, BIN 00179 * @returns number of chars written 00180 */ 00181 size_t println(long l, int base = DEC); 00182 00183 00184 /** Print out an unsigned long 00185 * @param l is the integer to print 00186 * @param base is the base to print, either DEC (default), HEX, BIN 00187 * @returns number of chars written 00188 */ 00189 size_t println(unsigned long l, int base = DEC); 00190 00191 00192 /** Print out a double 00193 * @param f is the integer to print 00194 * @param digits is the number of digits after the decimal 00195 * @returns number of chars written 00196 */ 00197 size_t println(double f, int digits = 2); 00198 00199 00200 /** prints, well, nothing in this case, but pretend we printed a newline 00201 * @returns 1 00202 */ 00203 size_t println(void); 00204 00205 00206 /*---------functions for Text and Graphic LCD adapters---------*/ 00207 00208 /** Turns off the cursor */ 00209 void disableCursor(void); 00210 00211 /** Turns on the cursor */ 00212 void enableCursor(void); 00213 00214 /** Displays a string at specified coordinates 00215 * @param x is the x coordinate to display the string 00216 * @param y is the y coordinate to display the string 00217 * @param s is the string to display 00218 */ 00219 void drawStr(uint8_t x, uint8_t y, const char *s); 00220 00221 /** Sets the print position for graphics or text 00222 * @param x is the x coordinate to display the string 00223 * @param y is the y coordinate to display the string 00224 * @param graph if set to _TEXT_ affects subsequent text position, otherwise, affects graphics position 00225 */ 00226 void setPrintPos(uint8_t x, uint8_t y, uint8_t graph = _TEXT_); 00227 00228 /** Clears the display screen */ 00229 void clearScreen(void); 00230 00231 00232 /** Configure your LCD if other than 1602 and the chip is other than KS0066U/F / HD44780 00233 * @param col is the number of columns 00234 * @param row is the number of rows 00235 */ 00236 void setLCDColRow(uint8_t col, uint8_t row); 00237 00238 /** Sets a new I2C address for the display (default is 0x27), the adapter will store the new address in memory 00239 * @param address is the the new address 00240 */ 00241 void setI2CAddress(uint8_t add); 00242 00243 /** Display Config on/off, the factory default set is on, 00244 * so, when the module is powered up, it will display 00245 * current communication mode on LCD, after you 00246 * design finished, you can turn it off 00247 * @param v is the 1 is on, 0 is off 00248 */ 00249 void displayConfig(uint8_t v); 00250 00251 /** Holdover from Arduino library; not needed */ 00252 void preprint(void); 00253 00254 /*----------Functions for Graphic LCD/OLED adapters only---------*/ 00255 //the functions in this section compatible with u8glib 00256 void drawBitmap(uint8_t x, uint8_t y, uint8_t w, uint8_t h, const uint8_t *bitmap); 00257 void drawBitmap256(uint8_t x, uint8_t y, uint8_t w, uint8_t h, const uint8_t *bitmap); 00258 void setRot90(void); 00259 void setRot180(void); 00260 void setRot270(void); 00261 void undoRotation(void); 00262 void setRotation(uint8_t); 00263 void setContrast(uint8_t); 00264 void drawBox(uint8_t x, uint8_t y, uint8_t w, uint8_t h); 00265 void drawCircle(uint8_t x, uint8_t y, uint8_t r, uint8_t = 0); 00266 void drawDisc(uint8_t x, uint8_t y, uint8_t r); 00267 void drawFrame(uint8_t x, uint8_t y, uint8_t w, uint8_t h); 00268 void drawPixel(uint8_t x, uint8_t y, uint8_t = 1); 00269 void drawLine(uint8_t x, uint8_t y, uint8_t x1, uint8_t y1); 00270 void drawLineTo(uint8_t x, uint8_t y); 00271 void drawHLine(uint8_t x, uint8_t y, uint8_t w); 00272 void drawVLine(uint8_t x, uint8_t y, uint8_t h); 00273 //------------------------------- 00274 //special functions for our adapters 00275 00276 /** Sets the font 00277 * 00278 * @parameter font - available fonts: 6,10,18,51,120,123, user font 200-203 00279 */ 00280 void setFont(uint8_t font); 00281 00282 /** go to next text line, depending on the font size */ 00283 void nextTextLine(void); 00284 00285 /** set color for graphic function */ 00286 void setColor(uint8_t); 00287 00288 /** set bg color for graphic function */ 00289 void setBgColor(uint8_t); 00290 00291 /** Turn on back light */ 00292 void backLightOn(void); 00293 00294 /** Turn off back light */ 00295 void backLightOff(void); 00296 00297 /** send command to LCD drectly 00298 * @param d - command 00299 */ 00300 void directCommand(uint8_t d); 00301 00302 /** send data to LCD drectly 00303 * @param d is the data 00304 */ 00305 void directData(uint8_t d); 00306 00307 /** Move rectangle area on screen to another place 00308 * @param x0, y1 is the top left of the area to move 00309 * @param x1, y1 is the bottom right of the area to move 00310 * @param xoffset, yoffset is the the distance to move 00311 */ 00312 void moveArea(uint8_t x0, uint8_t y0, uint8_t x1, uint8_t y1, char xoffset, char yoffset); 00313 00314 /** Display startup screen */ 00315 int displayStartScreen(uint8_t m); 00316 00317 /** Set display mode */ 00318 void setMode(uint8_t m); 00319 00320 /** set text position back to previous, only one back allowed */ 00321 void setTextPosBack(void); 00322 00323 void setTextPosOffset(char xoffset, char yoffset); 00324 void setTextPosAbs(uint8_t x, uint8_t y); 00325 void setLinePattern(uint8_t pattern); 00326 /** Only for universal serial adapter */ 00327 void setLCDChip(uint8_t chip); 00328 00329 void setDrawWindow(uint16_t x, uint16_t y, uint16_t w, uint16_t h); 00330 void resetDrawWindow(); 00331 void cleanDrawWindow(); 00332 00333 00334 /** Set Start Screen, 1st B is the lower byte of data length. 00335 * Convert images to C array here: <a href="http://www.digole.com/tools/PicturetoC_Hex_converter.php">http://www.digole.com/tools/PicturetoC_Hex_converter.php</a> 00336 * @param lon is the length of data 00337 * @param data is the binary data 00338 */ 00339 void uploadStartScreen(int lon, const unsigned char *data); //upload start screen 00340 00341 /** Upload a user font 00342 * @param lon is the length of data 00343 * @param data is the user font data 00344 * @param sect is the section of memory you want to upload to 00345 */ 00346 void uploadUserFont(int lon, const unsigned char *data, uint8_t sect); //upload user font 00347 00348 /** Send a Byte to output head on board 00349 * @param x is the byte to output 00350 */ 00351 void digitalOutput(uint8_t x); 00352 00353 private: 00354 I2C _device; 00355 uint8_t _address; 00356 uint8_t _Comdelay; 00357 00358 size_t printNumber(unsigned long n, uint8_t base); 00359 size_t printFloat(double number, uint8_t digits); 00360 }; 00361 00362 #endif
Generated on Wed Jul 13 2022 04:51:52 by
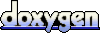