Nordic stack and drivers for the mbed BLE API
Dependents: idd_hw5_bleFanProto
Fork of nRF51822 by
nRF51822n.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "mbed.h" 00018 #include "nRF51822n.h" 00019 #include "nrf_soc.h" 00020 00021 #include "btle/btle.h" 00022 #include "nrf_delay.h" 00023 00024 /** 00025 * The singleton which represents the nRF51822 transport for the BLEDevice. 00026 */ 00027 static nRF51822n deviceInstance; 00028 00029 /** 00030 * BLE-API requires an implementation of the following function in order to 00031 * obtain its transport handle. 00032 */ 00033 BLEDeviceInstanceBase * 00034 createBLEDeviceInstance(void) 00035 { 00036 return (&deviceInstance); 00037 } 00038 00039 /**************************************************************************/ 00040 /*! 00041 @brief Constructor 00042 */ 00043 /**************************************************************************/ 00044 nRF51822n::nRF51822n(void) 00045 { 00046 } 00047 00048 /**************************************************************************/ 00049 /*! 00050 @brief Destructor 00051 */ 00052 /**************************************************************************/ 00053 nRF51822n::~nRF51822n(void) 00054 { 00055 } 00056 00057 const char *nRF51822n::getVersion(void) 00058 { 00059 static char versionString[10]; 00060 static bool versionFetched = false; 00061 00062 if (!versionFetched) { 00063 ble_version_t version; 00064 if (sd_ble_version_get(&version) == NRF_SUCCESS) { 00065 snprintf(versionString, sizeof(versionString), "%u.%u", version.version_number, version.subversion_number); 00066 versionFetched = true; 00067 } else { 00068 strncpy(versionString, "unknown", sizeof(versionString)); 00069 } 00070 } 00071 00072 return versionString; 00073 } 00074 00075 /* (Valid values are -40, -20, -16, -12, -8, -4, 0, 4) */ 00076 ble_error_t nRF51822n::setTxPower(int8_t txPower) 00077 { 00078 unsigned rc; 00079 if ((rc = sd_ble_gap_tx_power_set(txPower)) != NRF_SUCCESS) { 00080 switch (rc) { 00081 case NRF_ERROR_BUSY: 00082 return BLE_STACK_BUSY; 00083 case NRF_ERROR_INVALID_PARAM: 00084 default: 00085 return BLE_ERROR_PARAM_OUT_OF_RANGE; 00086 } 00087 } 00088 00089 return BLE_ERROR_NONE; 00090 } 00091 00092 /**************************************************************************/ 00093 /*! 00094 @brief Initialises anything required to start using BLE 00095 00096 @returns ble_error_t 00097 00098 @retval BLE_ERROR_NONE 00099 Everything executed properly 00100 00101 @section EXAMPLE 00102 00103 @code 00104 00105 @endcode 00106 */ 00107 /**************************************************************************/ 00108 ble_error_t nRF51822n::init(void) 00109 { 00110 /* ToDo: Clear memory contents, reset the SD, etc. */ 00111 btle_init(); 00112 00113 reset(); 00114 00115 return BLE_ERROR_NONE; 00116 } 00117 00118 /**************************************************************************/ 00119 /*! 00120 @brief Resets the BLE HW, removing any existing services and 00121 characteristics 00122 00123 @returns ble_error_t 00124 00125 @retval BLE_ERROR_NONE 00126 Everything executed properly 00127 00128 @section EXAMPLE 00129 00130 @code 00131 00132 @endcode 00133 */ 00134 /**************************************************************************/ 00135 ble_error_t nRF51822n::reset(void) 00136 { 00137 nrf_delay_us(500000); 00138 00139 /* Wait for the radio to come back up */ 00140 nrf_delay_us(1000000); 00141 00142 return BLE_ERROR_NONE; 00143 } 00144 00145 void 00146 nRF51822n::waitForEvent(void) 00147 { 00148 sd_app_evt_wait(); 00149 }
Generated on Tue Jul 12 2022 17:01:29 by
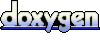