Nordic stack and drivers for the mbed BLE API
Dependents: idd_hw5_bleFanProto
Fork of nRF51822 by
ble_hts.h
00001 /* Copyright (c) 2012 Nordic Semiconductor. All Rights Reserved. 00002 * 00003 * The information contained herein is property of Nordic Semiconductor ASA. 00004 * Terms and conditions of usage are described in detail in NORDIC 00005 * SEMICONDUCTOR STANDARD SOFTWARE LICENSE AGREEMENT. 00006 * 00007 * Licensees are granted free, non-transferable use of the information. NO 00008 * WARRANTY of ANY KIND is provided. This heading must NOT be removed from 00009 * the file. 00010 */ 00011 00012 /** @file 00013 * 00014 * @defgroup ble_sdk_srv_hts Health Thermometer Service 00015 * @{ 00016 * @ingroup ble_sdk_srv 00017 * @brief Health Thermometer Service module. 00018 * 00019 * @details This module implements the Health Thermometer Service. 00020 * 00021 * If an event handler is supplied by the application, the Health Thermometer 00022 * Service will generate Health Thermometer Service events to the application. 00023 * 00024 * @note The application must propagate BLE stack events to the Health Thermometer Service 00025 * module by calling ble_hts_on_ble_evt() from the from the @ref ble_stack_handler function. 00026 * 00027 * @note Attention! 00028 * To maintain compliance with Nordic Semiconductor ASA Bluetooth profile 00029 * qualification listings, this section of source code must not be modified. 00030 */ 00031 00032 #ifndef BLE_HTS_H__ 00033 #define BLE_HTS_H__ 00034 00035 #include <stdint.h> 00036 #include <stdbool.h> 00037 #include "ble.h" 00038 #include "ble_srv_common.h " 00039 #include "ble_date_time.h" 00040 00041 // Temperature Type measurement locations 00042 #define BLE_HTS_TEMP_TYPE_ARMPIT 1 00043 #define BLE_HTS_TEMP_TYPE_BODY 2 00044 #define BLE_HTS_TEMP_TYPE_EAR 3 00045 #define BLE_HTS_TEMP_TYPE_FINGER 4 00046 #define BLE_HTS_TEMP_TYPE_GI_TRACT 5 00047 #define BLE_HTS_TEMP_TYPE_MOUTH 6 00048 #define BLE_HTS_TEMP_TYPE_RECTUM 7 00049 #define BLE_HTS_TEMP_TYPE_TOE 8 00050 #define BLE_HTS_TEMP_TYPE_EAR_DRUM 9 00051 00052 /**@brief Health Thermometer Service event type. */ 00053 typedef enum 00054 { 00055 BLE_HTS_EVT_INDICATION_ENABLED, /**< Health Thermometer value indication enabled event. */ 00056 BLE_HTS_EVT_INDICATION_DISABLED, /**< Health Thermometer value indication disabled event. */ 00057 BLE_HTS_EVT_INDICATION_CONFIRMED /**< Confirmation of a temperature measurement indication has been received. */ 00058 } ble_hts_evt_type_t; 00059 00060 /**@brief Health Thermometer Service event. */ 00061 typedef struct 00062 { 00063 ble_hts_evt_type_t evt_type; /**< Type of event. */ 00064 } ble_hts_evt_t; 00065 00066 // Forward declaration of the ble_hts_t type. 00067 typedef struct ble_hts_s ble_hts_t; 00068 00069 /**@brief Health Thermometer Service event handler type. */ 00070 typedef void (*ble_hts_evt_handler_t) (ble_hts_t * p_hts, ble_hts_evt_t * p_evt); 00071 00072 /**@brief FLOAT format (IEEE-11073 32-bit FLOAT, defined as a 32-bit value with a 24-bit mantissa 00073 * and an 8-bit exponent. */ 00074 typedef struct 00075 { 00076 int8_t exponent; /**< Base 10 exponent */ 00077 int32_t mantissa; /**< Mantissa, should be using only 24 bits */ 00078 } ieee_float32_t; 00079 00080 /**@brief Health Thermometer Service init structure. This contains all options and data 00081 * needed for initialization of the service. */ 00082 typedef struct 00083 { 00084 ble_hts_evt_handler_t evt_handler; /**< Event handler to be called for handling events in the Health Thermometer Service. */ 00085 ble_srv_cccd_security_mode_t hts_meas_attr_md; /**< Initial security level for health thermometer measurement attribute */ 00086 ble_srv_security_mode_t hts_temp_type_attr_md; /**< Initial security level for health thermometer tempearture type attribute */ 00087 uint8_t temp_type_as_characteristic; /**< Set non-zero if temp type given as characteristic */ 00088 uint8_t temp_type; /**< Temperature type if temperature characteristic is used */ 00089 } ble_hts_init_t; 00090 00091 /**@brief Health Thermometer Service structure. This contains various status information for 00092 * the service. */ 00093 typedef struct ble_hts_s 00094 { 00095 ble_hts_evt_handler_t evt_handler; /**< Event handler to be called for handling events in the Health Thermometer Service. */ 00096 uint16_t service_handle; /**< Handle of Health Thermometer Service (as provided by the BLE stack). */ 00097 ble_gatts_char_handles_t meas_handles; /**< Handles related to the Health Thermometer Measurement characteristic. */ 00098 ble_gatts_char_handles_t temp_type_handles; /**< Handles related to the Health Thermometer Temperature Type characteristic. */ 00099 uint16_t conn_handle; /**< Handle of the current connection (as provided by the BLE stack, is BLE_CONN_HANDLE_INVALID if not in a connection). */ 00100 uint8_t temp_type; /**< Temperature type indicates where the measurement was taken. */ 00101 } ble_hts_t; 00102 00103 /**@brief Health Thermometer Service measurement structure. This contains a Health Thermometer 00104 * measurement. */ 00105 typedef struct ble_hts_meas_s 00106 { 00107 bool temp_in_fahr_units; /**< True if Temperature is in Fahrenheit units, Celcius otherwise. */ 00108 bool time_stamp_present; /**< True if Time Stamp is present. */ 00109 bool temp_type_present; /**< True if Temperature Type is present. */ 00110 ieee_float32_t temp_in_celcius; /**< Temperature Measurement Value (Celcius). */ 00111 ieee_float32_t temp_in_fahr; /**< Temperature Measurement Value (Fahrenheit). */ 00112 ble_date_time_t time_stamp; /**< Time Stamp. */ 00113 uint8_t temp_type; /**< Temperature Type. */ 00114 } ble_hts_meas_t; 00115 00116 /**@brief Function for initializing the Health Thermometer Service. 00117 * 00118 * @param[out] p_hts Health Thermometer Service structure. This structure will have to 00119 * be supplied by the application. It will be initialized by this function, 00120 * and will later be used to identify this particular service instance. 00121 * @param[in] p_hts_init Information needed to initialize the service. 00122 * 00123 * @return NRF_SUCCESS on successful initialization of service, otherwise an error code. 00124 */ 00125 uint32_t ble_hts_init(ble_hts_t * p_hts, const ble_hts_init_t * p_hts_init); 00126 00127 /**@brief Function for handling the Application's BLE Stack events. 00128 * 00129 * @details Handles all events from the BLE stack of interest to the Health Thermometer Service. 00130 * 00131 * @param[in] p_hts Health Thermometer Service structure. 00132 * @param[in] p_ble_evt Event received from the BLE stack. 00133 */ 00134 void ble_hts_on_ble_evt(ble_hts_t * p_hts, ble_evt_t * p_ble_evt); 00135 00136 /**@brief Function for sending health thermometer measurement if indication has been enabled. 00137 * 00138 * @details The application calls this function after having performed a Health Thermometer 00139 * measurement. If indication has been enabled, the measurement data is encoded and 00140 * sent to the client. 00141 * 00142 * @param[in] p_hts Health Thermometer Service structure. 00143 * @param[in] p_hts_meas Pointer to new health thermometer measurement. 00144 * 00145 * @return NRF_SUCCESS on success, otherwise an error code. 00146 */ 00147 uint32_t ble_hts_measurement_send(ble_hts_t * p_hts, ble_hts_meas_t * p_hts_meas); 00148 00149 /**@brief Function for checking if indication of Temperature Measurement is currently enabled. 00150 * 00151 * @param[in] p_hts Health Thermometer Service structure. 00152 * @param[out] p_indication_enabled TRUE if indication is enabled, FALSE otherwise. 00153 * 00154 * @return NRF_SUCCESS on success, otherwise an error code. 00155 */ 00156 uint32_t ble_hts_is_indication_enabled(ble_hts_t * p_hts, bool * p_indication_enabled); 00157 00158 #endif // BLE_HTS_H__ 00159 00160 /** @} */
Generated on Tue Jul 12 2022 17:01:28 by
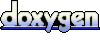