Nordic stack and drivers for the mbed BLE API
Dependents: idd_hw5_bleFanProto
Fork of nRF51822 by
ble_hrs.h
00001 /* Copyright (c) 2012 Nordic Semiconductor. All Rights Reserved. 00002 * 00003 * The information contained herein is property of Nordic Semiconductor ASA. 00004 * Terms and conditions of usage are described in detail in NORDIC 00005 * SEMICONDUCTOR STANDARD SOFTWARE LICENSE AGREEMENT. 00006 * 00007 * Licensees are granted free, non-transferable use of the information. NO 00008 * WARRANTY of ANY KIND is provided. This heading must NOT be removed from 00009 * the file. 00010 * 00011 */ 00012 00013 /** @file 00014 * 00015 * @defgroup ble_sdk_srv_hrs Heart Rate Service 00016 * @{ 00017 * @ingroup ble_sdk_srv 00018 * @brief Heart Rate Service module. 00019 * 00020 * @details This module implements the Heart Rate Service with the Heart Rate Measurement, 00021 * Body Sensor Location and Heart Rate Control Point characteristics. 00022 * During initialization it adds the Heart Rate Service and Heart Rate Measurement 00023 * characteristic to the BLE stack database. Optionally it also adds the 00024 * Body Sensor Location and Heart Rate Control Point characteristics. 00025 * 00026 * If enabled, notification of the Heart Rate Measurement characteristic is performed 00027 * when the application calls ble_hrs_heart_rate_measurement_send(). 00028 * 00029 * The Heart Rate Service also provides a set of functions for manipulating the 00030 * various fields in the Heart Rate Measurement characteristic, as well as setting 00031 * the Body Sensor Location characteristic value. 00032 * 00033 * If an event handler is supplied by the application, the Heart Rate Service will 00034 * generate Heart Rate Service events to the application. 00035 * 00036 * @note The application must propagate BLE stack events to the Heart Rate Service module by calling 00037 * ble_hrs_on_ble_evt() from the from the @ref ble_stack_handler callback. 00038 * 00039 * @note Attention! 00040 * To maintain compliance with Nordic Semiconductor ASA Bluetooth profile 00041 * qualification listings, this section of source code must not be modified. 00042 */ 00043 00044 #ifndef BLE_HRS_H__ 00045 #define BLE_HRS_H__ 00046 00047 #include <stdint.h> 00048 #include <stdbool.h> 00049 #include "ble.h" 00050 #include "ble_srv_common.h " 00051 00052 // Body Sensor Location values 00053 #define BLE_HRS_BODY_SENSOR_LOCATION_OTHER 0 00054 #define BLE_HRS_BODY_SENSOR_LOCATION_CHEST 1 00055 #define BLE_HRS_BODY_SENSOR_LOCATION_WRIST 2 00056 #define BLE_HRS_BODY_SENSOR_LOCATION_FINGER 3 00057 #define BLE_HRS_BODY_SENSOR_LOCATION_HAND 4 00058 #define BLE_HRS_BODY_SENSOR_LOCATION_EAR_LOBE 5 00059 #define BLE_HRS_BODY_SENSOR_LOCATION_FOOT 6 00060 00061 #define BLE_HRS_MAX_BUFFERED_RR_INTERVALS 20 /**< Size of RR Interval buffer inside service. */ 00062 00063 /**@brief Heart Rate Service event type. */ 00064 typedef enum 00065 { 00066 BLE_HRS_EVT_NOTIFICATION_ENABLED, /**< Heart Rate value notification enabled event. */ 00067 BLE_HRS_EVT_NOTIFICATION_DISABLED /**< Heart Rate value notification disabled event. */ 00068 } ble_hrs_evt_type_t; 00069 00070 /**@brief Heart Rate Service event. */ 00071 typedef struct 00072 { 00073 ble_hrs_evt_type_t evt_type; /**< Type of event. */ 00074 } ble_hrs_evt_t; 00075 00076 // Forward declaration of the ble_hrs_t type. 00077 typedef struct ble_hrs_s ble_hrs_t; 00078 00079 /**@brief Heart Rate Service event handler type. */ 00080 typedef void (*ble_hrs_evt_handler_t) (ble_hrs_t * p_hrs, ble_hrs_evt_t * p_evt); 00081 00082 /**@brief Heart Rate Service init structure. This contains all options and data needed for 00083 * initialization of the service. */ 00084 typedef struct 00085 { 00086 ble_hrs_evt_handler_t evt_handler; /**< Event handler to be called for handling events in the Heart Rate Service. */ 00087 bool is_sensor_contact_supported; /**< Determines if sensor contact detection is to be supported. */ 00088 uint8_t * p_body_sensor_location; /**< If not NULL, initial value of the Body Sensor Location characteristic. */ 00089 ble_srv_cccd_security_mode_t hrs_hrm_attr_md; /**< Initial security level for heart rate service measurement attribute */ 00090 ble_srv_security_mode_t hrs_bsl_attr_md; /**< Initial security level for body sensor location attribute */ 00091 } ble_hrs_init_t; 00092 00093 /**@brief Heart Rate Service structure. This contains various status information for the service. */ 00094 typedef struct ble_hrs_s 00095 { 00096 ble_hrs_evt_handler_t evt_handler; /**< Event handler to be called for handling events in the Heart Rate Service. */ 00097 bool is_expended_energy_supported; /**< TRUE if Expended Energy measurement is supported. */ 00098 bool is_sensor_contact_supported; /**< TRUE if sensor contact detection is supported. */ 00099 uint16_t service_handle; /**< Handle of Heart Rate Service (as provided by the BLE stack). */ 00100 ble_gatts_char_handles_t hrm_handles; /**< Handles related to the Heart Rate Measurement characteristic. */ 00101 ble_gatts_char_handles_t bsl_handles; /**< Handles related to the Body Sensor Location characteristic. */ 00102 ble_gatts_char_handles_t hrcp_handles; /**< Handles related to the Heart Rate Control Point characteristic. */ 00103 uint16_t conn_handle; /**< Handle of the current connection (as provided by the BLE stack, is BLE_CONN_HANDLE_INVALID if not in a connection). */ 00104 bool is_sensor_contact_detected; /**< TRUE if sensor contact has been detected. */ 00105 uint16_t rr_interval[BLE_HRS_MAX_BUFFERED_RR_INTERVALS]; /**< Set of RR Interval measurements since the last Heart Rate Measurement transmission. */ 00106 uint16_t rr_interval_count; /**< Number of RR Interval measurements since the last Heart Rate Measurement transmission. */ 00107 } ble_hrs_t; 00108 00109 /**@brief Function for initializing the Heart Rate Service. 00110 * 00111 * @param[out] p_hrs Heart Rate Service structure. This structure will have to be supplied by 00112 * the application. It will be initialized by this function, and will later 00113 * be used to identify this particular service instance. 00114 * @param[in] p_hrs_init Information needed to initialize the service. 00115 * 00116 * @return NRF_SUCCESS on successful initialization of service, otherwise an error code. 00117 */ 00118 uint32_t ble_hrs_init(ble_hrs_t * p_hrs, const ble_hrs_init_t * p_hrs_init); 00119 00120 /**@brief Function for handling the Application's BLE Stack events. 00121 * 00122 * @details Handles all events from the BLE stack of interest to the Heart Rate Service. 00123 * 00124 * @param[in] p_hrs Heart Rate Service structure. 00125 * @param[in] p_ble_evt Event received from the BLE stack. 00126 */ 00127 void ble_hrs_on_ble_evt(ble_hrs_t * p_hrs, ble_evt_t * p_ble_evt); 00128 00129 /**@brief Function for sending heart rate measurement if notification has been enabled. 00130 * 00131 * @details The application calls this function after having performed a heart rate measurement. 00132 * If notification has been enabled, the heart rate measurement data is encoded and sent to 00133 * the client. 00134 * 00135 * @param[in] p_hrs Heart Rate Service structure. 00136 * @param[in] heart_rate New heart rate measurement. 00137 * @param[in] include_expended_energy Determines if expended energy will be included in the 00138 * heart rate measurement data. 00139 * 00140 * @return NRF_SUCCESS on success, otherwise an error code. 00141 */ 00142 uint32_t ble_hrs_heart_rate_measurement_send(ble_hrs_t * p_hrs, uint16_t heart_rate); 00143 00144 /**@brief Function for adding a RR Interval measurement to the RR Interval buffer. 00145 * 00146 * @details All buffered RR Interval measurements will be included in the next heart rate 00147 * measurement message, up to the maximum number of measurements that will fit into the 00148 * message. If the buffer is full, the oldest measurement in the buffer will be deleted. 00149 * 00150 * @param[in] p_hrs Heart Rate Service structure. 00151 * @param[in] rr_interval New RR Interval measurement (will be buffered until the next 00152 * transmission of Heart Rate Measurement). 00153 */ 00154 void ble_hrs_rr_interval_add(ble_hrs_t * p_hrs, uint16_t rr_interval); 00155 00156 /**@brief Function for checking if RR Interval buffer is full. 00157 * 00158 * @param[in] p_hrs Heart Rate Service structure. 00159 * 00160 * @return true if RR Interval buffer is full, false otherwise. 00161 */ 00162 bool ble_hrs_rr_interval_buffer_is_full(ble_hrs_t * p_hrs); 00163 00164 /**@brief Function for setting the state of the Sensor Contact Supported bit. 00165 * 00166 * @param[in] p_hrs Heart Rate Service structure. 00167 * @param[in] is_sensor_contact_supported New state of the Sensor Contact Supported bit. 00168 * 00169 * @return NRF_SUCCESS on success, otherwise an error code. 00170 */ 00171 uint32_t ble_hrs_sensor_contact_supported_set(ble_hrs_t * p_hrs, bool is_sensor_contact_supported); 00172 00173 /**@brief Function for setting the state of the Sensor Contact Detected bit. 00174 * 00175 * @param[in] p_hrs Heart Rate Service structure. 00176 * @param[in] is_sensor_contact_detected TRUE if sensor contact is detected, FALSE otherwise. 00177 */ 00178 void ble_hrs_sensor_contact_detected_update(ble_hrs_t * p_hrs, bool is_sensor_contact_detected); 00179 00180 /**@brief Function for setting the Body Sensor Location. 00181 * 00182 * @details Sets a new value of the Body Sensor Location characteristic. The new value will be sent 00183 * to the client the next time the client reads the Body Sensor Location characteristic. 00184 * 00185 * @param[in] p_hrs Heart Rate Service structure. 00186 * @param[in] body_sensor_location New Body Sensor Location. 00187 * 00188 * @return NRF_SUCCESS on success, otherwise an error code. 00189 */ 00190 uint32_t ble_hrs_body_sensor_location_set(ble_hrs_t * p_hrs, uint8_t body_sensor_location); 00191 00192 #endif // BLE_HRS_H__ 00193 00194 /** @} */
Generated on Tue Jul 12 2022 17:01:28 by
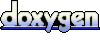