Nordic stack and drivers for the mbed BLE API
Dependents: idd_hw5_bleFanProto
Fork of nRF51822 by
ble_dis.h
00001 /* Copyright (c) 2012 Nordic Semiconductor. All Rights Reserved. 00002 * 00003 * The information contained herein is property of Nordic Semiconductor ASA. 00004 * Terms and conditions of usage are described in detail in NORDIC 00005 * SEMICONDUCTOR STANDARD SOFTWARE LICENSE AGREEMENT. 00006 * 00007 * Licensees are granted free, non-transferable use of the information. NO 00008 * WARRANTY of ANY KIND is provided. This heading must NOT be removed from 00009 * the file. 00010 * 00011 */ 00012 00013 /** @file 00014 * 00015 * @defgroup ble_sdk_srv_dis Device Information Service 00016 * @{ 00017 * @ingroup ble_sdk_srv 00018 * @brief Device Information Service module. 00019 * 00020 * @details This module implements the Device Information Service. 00021 * During initialization it adds the Device Information Service to the BLE stack database. 00022 * It then encodes the supplied information, and adds the curresponding characteristics. 00023 * 00024 * @note Attention! 00025 * To maintain compliance with Nordic Semiconductor ASA Bluetooth profile 00026 * qualification listings, this section of source code must not be modified. 00027 */ 00028 00029 #ifndef BLE_DIS_H__ 00030 #define BLE_DIS_H__ 00031 00032 #include <stdint.h> 00033 #include "ble_srv_common.h " 00034 00035 // Vendor ID Source values 00036 #define BLE_DIS_VENDOR_ID_SRC_BLUETOOTH_SIG 1 /**< Vendor ID assigned by Bluetooth SIG. */ 00037 #define BLE_DIS_VENDOR_ID_SRC_USB_IMPL_FORUM 2 /**< Vendor ID assigned by USB Implementer's Forum. */ 00038 00039 /**@brief System ID parameters */ 00040 typedef struct 00041 { 00042 uint64_t manufacturer_id; /**< Manufacturer ID. Only 5 LSOs shall be used. */ 00043 uint32_t organizationally_unique_id; /**< Organizationally unique ID. Only 3 LSOs shall be used. */ 00044 } ble_dis_sys_id_t; 00045 00046 /**@brief IEEE 11073-20601 Regulatory Certification Data List Structure */ 00047 typedef struct 00048 { 00049 uint8_t * p_list; /**< Pointer the byte array containing the encoded opaque structure based on IEEE 11073-20601 specification. */ 00050 uint8_t list_len; /**< Length of the byte array. */ 00051 } ble_dis_reg_cert_data_list_t; 00052 00053 /**@brief PnP ID parameters */ 00054 typedef struct 00055 { 00056 uint8_t vendor_id_source; /**< Vendor ID Source. see @ref DIS_VENDOR_ID_SRC_VALUES. */ 00057 uint16_t vendor_id; /**< Vendor ID. */ 00058 uint16_t product_id; /**< Product ID. */ 00059 uint16_t product_version; /**< Product Version. */ 00060 } ble_dis_pnp_id_t; 00061 00062 /**@brief Device Information Service init structure. This contains all possible characteristics 00063 * needed for initialization of the service. 00064 */ 00065 typedef struct 00066 { 00067 ble_srv_utf8_str_t manufact_name_str; /**< Manufacturer Name String. */ 00068 ble_srv_utf8_str_t model_num_str; /**< Model Number String. */ 00069 ble_srv_utf8_str_t serial_num_str; /**< Serial Number String. */ 00070 ble_srv_utf8_str_t hw_rev_str; /**< Hardware Revision String. */ 00071 ble_srv_utf8_str_t fw_rev_str; /**< Firmware Revision String. */ 00072 ble_srv_utf8_str_t sw_rev_str; /**< Software Revision String. */ 00073 ble_dis_sys_id_t * p_sys_id; /**< System ID. */ 00074 ble_dis_reg_cert_data_list_t * p_reg_cert_data_list; /**< IEEE 11073-20601 Regulatory Certification Data List. */ 00075 ble_dis_pnp_id_t * p_pnp_id; /**< PnP ID. */ 00076 ble_srv_security_mode_t dis_attr_md; /**< Initial Security Setting for Device Information Characteristics. */ 00077 } ble_dis_init_t; 00078 00079 /**@brief Function for initializing the Device Information Service. 00080 * 00081 * @details This call allows the application to initialize the device information service. 00082 * It adds the DIS service and DIS characteristics to the database, using the initial 00083 * values supplied through the p_dis_init parameter. Characteristics which are not to be 00084 * added, shall be set to NULL in p_dis_init. 00085 * 00086 * @param[in] p_dis_init The structure containing the values of characteristics needed by the 00087 * service. 00088 * 00089 * @return NRF_SUCCESS on successful initialization of service. 00090 */ 00091 uint32_t ble_dis_init(const ble_dis_init_t * p_dis_init); 00092 00093 #endif // BLE_DIS_H__ 00094 00095 /** @} */
Generated on Tue Jul 12 2022 17:01:28 by
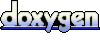