Nordic stack and drivers for the mbed BLE API
Dependents: idd_hw5_bleFanProto
Fork of nRF51822 by
ble_bas_c.h
00001 /* 00002 * Copyright (c) 2012 Nordic Semiconductor. All Rights Reserved. 00003 * 00004 * The information contained herein is confidential property of Nordic Semiconductor. The use, 00005 * copying, transfer or disclosure of such information is prohibited except by express written 00006 * agreement with Nordic Semiconductor. 00007 * 00008 */ 00009 00010 /**@file 00011 * 00012 * @defgroup ble_sdk_srv_bas_c Battery Service Client 00013 * @{ 00014 * @ingroup ble_sdk_srv 00015 * @brief Battery Service Client module. 00016 * 00017 * @details This module contains APIs to read and interact with the Battery Service of a remote 00018 * device. 00019 * 00020 * @note The application must propagate BLE stack events to this module by calling 00021 * ble_hrs_c_on_ble_evt(). 00022 * 00023 */ 00024 00025 #ifndef BLE_BAS_C_H__ 00026 #define BLE_BAS_C_H__ 00027 00028 #include <stdint.h> 00029 #include "ble.h" 00030 00031 /** 00032 * @defgroup bas_c_enums Enumerations 00033 * @{ 00034 */ 00035 00036 /**@brief Battery Service Client event type. */ 00037 typedef enum 00038 { 00039 BLE_BAS_C_EVT_DISCOVERY_COMPLETE, /**< Event indicating that the Battery Service has been discovered at the peer. */ 00040 BLE_BAS_C_EVT_BATT_NOTIFICATION, /**< Event indicating that a notification of the Battery Level characteristic has been received from the peer. */ 00041 BLE_BAS_C_EVT_BATT_READ_RESP /**< Event indicating that a read response on Battery Level characteristic has been received from peer. */ 00042 } ble_bas_c_evt_type_t; 00043 00044 /** @} */ 00045 00046 /** 00047 * @defgroup bas_c_structs Structures 00048 * @{ 00049 */ 00050 00051 /**@brief Battery Service Client Event structure. */ 00052 typedef struct 00053 { 00054 ble_bas_c_evt_type_t evt_type; /**< Event Type. */ 00055 union 00056 { 00057 uint8_t battery_level; /**< Battery level received from peer. This field will be used for the events @ref BLE_BAS_C_EVT_BATT_NOTIFICATION and @ref BLE_BAS_C_EVT_BATT_READ_RESP.*/ 00058 } params; 00059 } ble_bas_c_evt_t; 00060 00061 /** @} */ 00062 00063 /** 00064 * @defgroup bas_c_types Types 00065 * @{ 00066 */ 00067 00068 // Forward declaration of the ble_bas_t type. 00069 typedef struct ble_bas_c_s ble_bas_c_t; 00070 00071 /**@brief Event handler type. 00072 * 00073 * @details This is the type of the event handler that should be provided by the application 00074 * of this module in order to receive events. 00075 */ 00076 typedef void (* ble_bas_c_evt_handler_t) (ble_bas_c_t * p_bas_bas_c, ble_bas_c_evt_t * p_evt); 00077 00078 /** @} */ 00079 00080 /** 00081 * @addtogroup bas_c_structs 00082 * @{ 00083 */ 00084 00085 /**@brief Battery Service Client structure. 00086 00087 */ 00088 typedef struct ble_bas_c_s 00089 { 00090 uint16_t conn_handle; /**< Connection handle as provided by the SoftDevice. */ 00091 uint16_t bl_cccd_handle; /**< Handle of the CCCD of the Battery Level characteristic. */ 00092 uint16_t bl_handle; /**< Handle of the Battery Level characteristic as provided by the SoftDevice. */ 00093 ble_bas_c_evt_handler_t evt_handler; /**< Application event handler to be called when there is an event related to the Battery service. */ 00094 } ble_bas_c_t; 00095 00096 /**@brief Battery Service Client initialization structure. 00097 */ 00098 typedef struct 00099 { 00100 ble_bas_c_evt_handler_t evt_handler; /**< Event handler to be called by the Battery Service Client module whenever there is an event related to the Battery Service. */ 00101 } ble_bas_c_init_t; 00102 00103 /** @} */ 00104 00105 /** 00106 * @defgroup bas_c_functions Functions 00107 * @{ 00108 */ 00109 00110 /**@brief Function for initializing the Battery Service Client module. 00111 * 00112 * @details This function will initialize the module and set up Database Discovery to discover 00113 * the Battery Service. After calling this function, call @ref ble_db_discovery_start 00114 * to start discovery. 00115 * 00116 * @param[out] p_ble_bas_c Pointer to the Battery Service client structure. 00117 * @param[in] p_ble_bas_c_init Pointer to the Battery Service initialization structure containing 00118 * the initialization information. 00119 * 00120 * @retval NRF_SUCCESS Operation success. 00121 * @retval NRF_ERROR_NULL A parameter is NULL. 00122 * Otherwise, an error code returned by @ref ble_db_discovery_register. 00123 */ 00124 uint32_t ble_bas_c_init(ble_bas_c_t * p_ble_bas_c, ble_bas_c_init_t * p_ble_bas_c_init); 00125 00126 00127 /**@brief Function for handling BLE events from the SoftDevice. 00128 * 00129 * @details This function will handle the BLE events received from the SoftDevice. If the BLE 00130 * event is relevant for the Battery Service Client module, then it is used to update 00131 * interval variables and, if necessary, send events to the application. 00132 * 00133 * @note This function must be called by the application. 00134 * 00135 * @param[in] p_ble_bas_c Pointer to the Battery Service client structure. 00136 * @param[in] p_ble_evt Pointer to the BLE event. 00137 */ 00138 void ble_bas_c_on_ble_evt(ble_bas_c_t * p_ble_bas_c, const ble_evt_t * p_ble_evt); 00139 00140 00141 /**@brief Function for enabling notifications on the Battery Level characteristic. 00142 * 00143 * @details This function will enable to notification of the Battery Level characteristic at the 00144 * peer by writing to the CCCD of the Battery Level Characteristic. 00145 * 00146 * @param p_ble_bas_c Pointer to the Battery Service client structure. 00147 * 00148 * @retval NRF_SUCCESS If the SoftDevice has been requested to write to the CCCD of the peer. 00149 * NRF_ERROR_NULL Parameter is NULL. 00150 * Otherwise, an error code returned by the SoftDevice API @ref 00151 * sd_ble_gattc_write. 00152 */ 00153 uint32_t ble_bas_c_bl_notif_enable(ble_bas_c_t * p_ble_bas_c); 00154 00155 00156 /**@brief Function for reading the Battery Level characteristic. 00157 * 00158 * @param p_ble_bas_c Pointer to the Battery Service client structure. 00159 * 00160 * @retval NRF_SUCCESS If the read request was successfully queued to be sent to peer. 00161 */ 00162 uint32_t ble_bas_c_bl_read(ble_bas_c_t * p_ble_bas_c); 00163 00164 00165 /** @} */ // End tag for Function group. 00166 00167 #endif // BLE_BAS_C_H__ 00168 00169 /** @} */ // End tag for the file.
Generated on Tue Jul 12 2022 17:01:28 by
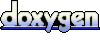