A library which allows the playing of Wav files using the TLV320
Dependents: RSALB_hbridge_helloworld RSALB_lobster WavPlayer_test AudioCODEC_HelloWorld
WavPlayer.h
00001 /** 00002 * @author Giles Barton-Owen 00003 * 00004 * @section LICENSE 00005 * 00006 * Copyright (c) 2012 mbed 00007 * 00008 * Permission is hereby granted, free of charge, to any person obtaining a copy 00009 * of this software and associated documentation files (the "Software"), to deal 00010 * in the Software without restriction, including without limitation the rights 00011 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00012 * copies of the Software, and to permit persons to whom the Software is 00013 * furnished to do so, subject to the following conditions: 00014 * 00015 * The above copyright notice and this permission notice shall be included in 00016 * all copies or substantial portions of the Software. 00017 * 00018 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00019 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00020 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00021 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00022 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00023 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00024 * THE SOFTWARE. 00025 * 00026 * @section DESCRIPTION 00027 * A WAV player library for the TLV320 and the LPC1768's built in I2S peripheral 00028 * 00029 */ 00030 00031 #ifndef WAVPLAYER_H 00032 #define WAVPLAYER_H 00033 00034 #include "mbed.h" 00035 #include "WavPlayerConfig.h" 00036 #include "RingBuffer.h" 00037 #include "TLV320.h" 00038 #include "I2S.h" 00039 00040 /** A class to play WAV files from a file system, tested with USB 00041 * 00042 * Example (note, this has requires the USB MSC library to be imported): 00043 * @code 00044 * 00045 * #include "mbed.h" 00046 * #include "WavPlayer.h" 00047 * #include "MSCFileSystem.h" 00048 * 00049 * MSCFileSystem msc("msc"); // Mount flash drive under the name "msc" 00050 * WavPlayer player; 00051 * 00052 * int main() { 00053 * FILE *fp = fopen("/msc/test.wav", "r"); // Open "out.txt" on the local file system for writing 00054 * player.open(&fp); 00055 * player.play(); 00056 * fclose(fp); 00057 * } 00058 * @endcode 00059 */ 00060 00061 00062 class WavPlayer 00063 { 00064 public: 00065 /** Create a WavPlayer instance 00066 * 00067 */ 00068 WavPlayer(); 00069 00070 /** Create a WavPlayer instance 00071 * 00072 * @param fpp A pointer to a file pointer to read out of 00073 */ 00074 WavPlayer(FILE **fpp); 00075 00076 /** Set the file to read out of 00077 * 00078 * @param fpp A pointer to a file pointer to read out of 00079 */ 00080 void open(FILE **fpp); 00081 00082 /** Extract the header infomation, automatically called by open 00083 */ 00084 int getConfig(); 00085 00086 /** Play the entire file. Blocking 00087 */ 00088 float play(); 00089 00090 /** Play the file for a certain number of seconds. Blocking 00091 * 00092 * @param time The number of seconds to play the file for. 00093 */ 00094 float play(float time); 00095 00096 /** Play the file for a certain number of seconds, from a certain start point. Blocking 00097 * 00098 * @param start The start time 00099 * @param timefor The number of seconds to play the file for. 00100 */ 00101 float play(float start, float timefor); 00102 00103 00104 00105 private: 00106 WavPlayerConfig config; 00107 FILE ** filepp; 00108 RingBuffer rbuf; 00109 00110 I2S i2s; 00111 TLV320 codec; 00112 static void i2sisr(); 00113 void i2sisr_(); 00114 static WavPlayer* instance; 00115 00116 int fseekread(FILE *fp, char* str, int offset, int len); 00117 uint32_t getu32(char str[], int len); 00118 uint16_t getu16(char str[], int len); 00119 int32_t get32(char str[], int len); 00120 int16_t get16(char str[], int len); 00121 uint32_t fsru32(FILE *fp, int offset, int len = 4); 00122 uint16_t fsru16(FILE *fp, int offset, int len = 2); 00123 void clear(char* str, int len); 00124 00125 int findChunk(FILE *fp, char* match, int len, int fileSize, int startOffset = 12); 00126 int findChunk(FILE *fp, char* match, int len, int startOffset = 12); 00127 void fgets_m(char* str, int num, FILE* fp); 00128 00129 float sine_gen(int16_t* buf, int len, float div, float phase); 00130 00131 bool flag_vol; 00132 double volume; 00133 00134 bool stereo; 00135 00136 bool flag_play; 00137 00138 Timer timer; 00139 00140 int isr_underrun; 00141 00142 float read_time; 00143 int read_time_div; 00144 00145 float isr_time; 00146 int isr_time_div; 00147 00148 float current_time; 00149 00150 int slow_count; 00151 00152 //Timer isrtimer; 00153 00154 //DigitalOut ext_flag; 00155 //DigitalOut run_flag; 00156 00157 }; 00158 00159 #endif
Generated on Tue Jul 12 2022 17:42:18 by
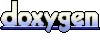