A PWM/duty cycle measurement library using Timer2
Dependents: PWMAverage_test pwm_duty_measurement
PWMAverage Class Reference
A class for measuring PWM/Duty cycle of a signal connected to pins 30 and 29. More...
#include <PWMAverage.h>
Public Member Functions | |
PWMAverage (PinName cap0, PinName cap1) | |
Create a PWMAverage object. | |
void | reset () |
Reset the counters and values. | |
void | start () |
Start the timer. | |
void | stop () |
Stop the timer. | |
float | read () |
Read the duty cycle measured. | |
double | avg_up () |
Read the average length of time the signal was high per cycle in seconds. | |
float | avg_UP () |
Read the average length of time the signal was high per cycle in seconds. | |
double | avg_down () |
Read the average length of time the signal was low per cycle in seconds. | |
double | period () |
Read the average period in seconds. | |
int | count () |
Read the number of cycles counted over. |
Detailed Description
A class for measuring PWM/Duty cycle of a signal connected to pins 30 and 29.
<<<<<<< local Example: =======
>>>>>>> other
// Measure and print the average duty cycle of a signal connected to p29 and p30 (together) while the button (p16) is pulled high #include "mbed.h" #include "PWMAverage.h" DigitalOut myled(LED1); PWMAverage pa(p29,p30); DigitalIn button (p16); Timer tmr; int main() { button.mode(PullDown); while(1) { pa.reset(); while (!button) {} pa.start(); tmr.start(); myled=1; while (button) {} pa.stop(); tmr.stop(); myled=0; printf("Average dudy cycle over %d us was %.4f\n\r",tmr.read_us(),pa.read()); } }
Definition at line 79 of file PWMAverage.h.
Constructor & Destructor Documentation
PWMAverage | ( | PinName | cap0, |
PinName | cap1 | ||
) |
Create a PWMAverage object.
- Parameters:
-
cap0 Pin connected to signal (ignored in program) cap1 Pin connected to signal (ignored in program)
Definition at line 32 of file PWMAverage.cpp.
Member Function Documentation
double avg_down | ( | ) |
Read the average length of time the signal was low per cycle in seconds.
- Returns:
- The Length of time the signal was low per cycle in seconds
Definition at line 85 of file PWMAverage.cpp.
double avg_up | ( | ) |
Read the average length of time the signal was high per cycle in seconds.
- Returns:
- The Length of time the signal was high per cycle in seconds
Definition at line 72 of file PWMAverage.cpp.
float avg_UP | ( | ) |
Read the average length of time the signal was high per cycle in seconds.
- Returns:
- The Length of time the signal was high per cycle in seconds
Definition at line 79 of file PWMAverage.cpp.
int count | ( | ) |
Read the number of cycles counted over.
- Returns:
- The number of cycles
Definition at line 97 of file PWMAverage.cpp.
double period | ( | ) |
Read the average period in seconds.
- Returns:
- The Period
Definition at line 91 of file PWMAverage.cpp.
float read | ( | ) |
void reset | ( | ) |
Reset the counters and values.
Definition at line 43 of file PWMAverage.cpp.
void start | ( | ) |
Start the timer.
Definition at line 52 of file PWMAverage.cpp.
void stop | ( | ) |
Stop the timer.
Definition at line 60 of file PWMAverage.cpp.
Generated on Tue Jul 12 2022 20:22:51 by
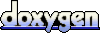