A simple Hbridge driver library (tested with the L293D). It uses a PWM pin on the enable and two DIOs on the control pins. Versatile.
Dependents: RSALB_hbridge_helloworld RSALB_lobster uva_nc
HBridge.h
00001 /** 00002 * @author Giles Barton-Owen 00003 * 00004 * @section LICENSE 00005 * 00006 * Copyright (c) 2012 mbed 00007 * 00008 * Permission is hereby granted, free of charge, to any person obtaining a copy 00009 * of this software and associated documentation files (the "Software"), to deal 00010 * in the Software without restriction, including without limitation the rights 00011 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00012 * copies of the Software, and to permit persons to whom the Software is 00013 * furnished to do so, subject to the following conditions: 00014 * 00015 * The above copyright notice and this permission notice shall be included in 00016 * all copies or substantial portions of the Software. 00017 * 00018 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00019 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00020 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00021 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00022 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00023 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00024 * THE SOFTWARE. 00025 * 00026 * @section DESCRIPTION 00027 * A simple library to control an H-Bridge driver using two DIO and a PWM pin 00028 * 00029 */ 00030 00031 00032 #ifndef HBRIDGERAW_H 00033 #define HBRIDGERAW_H 00034 00035 #include "mbed.h" 00036 00037 /** A simple HBridge controller library 00038 */ 00039 class HBridge 00040 { 00041 public: 00042 /** Creates an instance of the HBridge class 00043 * 00044 * @param A The pin the "A" line is connected to (naming taken from the L293D). 00045 * @param B The pin the "B" line is connected to (naming taken from the L293D). 00046 * @param en The pin the enable line is connected to.] 00047 */ 00048 HBridge(PinName A, PinName B, PinName en); 00049 00050 /** Hard stops the motor 00051 */ 00052 void stop(); 00053 00054 /** Starts the motor 00055 */ 00056 void start(); 00057 00058 /** Sets the PWM output of the enable pin and therefore the speed of the motor 00059 * 00060 * @param speed_in The float value of the desired speed between -1 and 1 00061 */ 00062 void speed(float speed_in); 00063 00064 /** Turn off and on the enable pin 00065 * 00066 * @param onoff Enable the motor or not (on(true)) 00067 */ 00068 void power(bool onoff); 00069 00070 /** Set the pwm value of the enable pin 00071 * 00072 * @param power_in The value of the pwm enable pins 00073 */ 00074 void power(float power_in); 00075 00076 /** Soft stop the motor 00077 */ 00078 void soft_stop(); 00079 00080 /** Go forward, starts the motor 00081 */ 00082 void forward(); 00083 00084 /** Go backward, starts the motor 00085 */ 00086 void backward(); 00087 00088 /** Go forward, starts the motor 00089 * 00090 * @param speed_in The float value of the desired speed between 0 and 1 00091 */ 00092 void forward(float speed_in); 00093 00094 /** Go backward, starts the motor 00095 * 00096 * @param speed_in The float value of the desired speed between 0 and 1 00097 */ 00098 void backward(float speed_in); 00099 00100 /** Set A high or low 00101 * 00102 * @param highlow Set the high/low state of the A half-bridge (high(true)) 00103 */ 00104 void A(bool highlow); 00105 00106 /** Set B high or low 00107 * 00108 * @param highlow Set the high/low state of the B half-bridge (high(true)) 00109 */ 00110 void B(bool highlow); 00111 00112 /** Set the direction of travel 00113 * 00114 * @param direction_in Forward = true 00115 */ 00116 void direction(bool direction_in); 00117 00118 00119 private: 00120 PwmOut enable; 00121 DigitalOut Adrive; 00122 DigitalOut Bdrive; 00123 00124 bool power_status; 00125 float speed_value; 00126 bool A_value; 00127 bool B_value; 00128 bool stored_direction; 00129 00130 void set(); 00131 00132 00133 }; 00134 00135 00136 00137 #endif
Generated on Wed Jul 13 2022 02:42:30 by
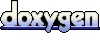