A simple Hbridge driver library (tested with the L293D). It uses a PWM pin on the enable and two DIOs on the control pins. Versatile.
Dependents: RSALB_hbridge_helloworld RSALB_lobster uva_nc
HBridge.cpp
00001 #include "HBridge.h" 00002 00003 HBridge::HBridge(PinName A, PinName B, PinName en): enable(en),Adrive(A),Bdrive(B) 00004 { 00005 speed_value = 1; 00006 power_status = false; 00007 A_value = false; 00008 B_value = false; 00009 stored_direction = true; 00010 set(); 00011 } 00012 00013 void HBridge::stop() 00014 { 00015 power_status = true; 00016 A_value = false; 00017 B_value = false; 00018 set(); 00019 } 00020 00021 void HBridge::start() 00022 { 00023 direction(true); 00024 power_status = true; 00025 set(); 00026 } 00027 00028 void HBridge::power(float power_in) 00029 { 00030 if(power_in <= 0) 00031 { 00032 speed_value = 0; 00033 } 00034 else 00035 { 00036 speed_value = power_in; 00037 } 00038 set(); 00039 } 00040 00041 void HBridge::speed(float speed_in) 00042 { 00043 if(speed_in < 0) 00044 { 00045 power(speed_in * -1); 00046 direction(false); 00047 } 00048 else 00049 { 00050 power(speed_in); 00051 direction(true); 00052 } 00053 } 00054 00055 void HBridge::power(bool onoff) 00056 { 00057 power_status = onoff; 00058 set(); 00059 } 00060 00061 void HBridge::soft_stop() 00062 { 00063 power_status = false; 00064 set(); 00065 } 00066 00067 00068 void HBridge::forward() 00069 { 00070 direction(true); 00071 power(true); 00072 } 00073 00074 00075 void HBridge::backward() 00076 { 00077 direction(false); 00078 power(true); 00079 } 00080 00081 00082 void HBridge::forward(float speed_in) 00083 { 00084 speed(speed_in); 00085 forward(); 00086 } 00087 00088 00089 void HBridge::backward(float speed_in) 00090 { 00091 speed(speed_in); 00092 backward(); 00093 } 00094 00095 void HBridge::direction(bool direction_in) 00096 { 00097 stored_direction = direction_in; 00098 A_value = stored_direction; 00099 B_value = !stored_direction; 00100 set(); 00101 } 00102 00103 void HBridge::A(bool highlow) 00104 { 00105 A_value = highlow; 00106 } 00107 00108 void HBridge::B(bool highlow) 00109 { 00110 B_value = highlow; 00111 } 00112 00113 void HBridge::set() 00114 { 00115 Adrive = A_value & power_status; 00116 Bdrive = B_value & power_status; 00117 enable = speed_value * float(power_status); 00118 }
Generated on Wed Jul 13 2022 02:42:30 by
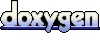