KeyboardManager: a class to manage the polling of a switch-matrix keyboard
KeyMap Class Reference
A class used to store a set of key mappings. More...
#include <KeyMapper.h>
Public Member Functions | |
KeyMap () | |
Creates an empty keymap. | |
KeyMap (const std::string &str) | |
Creates a keymap based on a string. | |
KeyMap (KeyEvent::EventType event, const std::string &str) | |
Creates a keymap based on a string for a certain key event. | |
KeyMap & | operator() (const std::string &str) |
Adds key mappings based on the given string. | |
KeyMap & | operator() (KeyEvent::EventType event, const std::string &str) |
Adds key mappings based on the given string for a given event. | |
KeyMap & | operator() (int key, char ch) |
Adds a key mapping for a key. | |
KeyMap & | operator() (KeyEvent::EventType event, int key, char ch) |
Adds a key mapping for a key event & code combo. | |
char | map (KeyEvent::EventType event, int key) const |
Gets the mapped character for a key press event. |
Detailed Description
A class used to store a set of key mappings.
It associates a key event and a key code to a mapped character. It also allows to specify a mapping for any unmatched key event of a key. The KeyMap can be set at construction time with a string and an optional key event. Additional mappings can be specified by using the () operator. This way, both individual mappings and string mappings are possible. Eg. keymap(KeyEvent::LongKeyPress, 1, '@')(1, '*') maps the key 1 to '*' for all key presses but to '@' when a long key press occurs.
Definition at line 21 of file KeyMapper.h.
Constructor & Destructor Documentation
KeyMap | ( | ) |
Creates an empty keymap.
Definition at line 26 of file KeyMapper.h.
KeyMap | ( | const std::string & | str ) |
Creates a keymap based on a string.
Each character is mapped to the key at that position (key 1 maps to str[1]).
Definition at line 32 of file KeyMapper.h.
KeyMap | ( | KeyEvent::EventType | event, |
const std::string & | str | ||
) |
Creates a keymap based on a string for a certain key event.
Each character is mapped to the key at that position (key 1 maps to str[1]).
Definition at line 39 of file KeyMapper.h.
Member Function Documentation
char map | ( | KeyEvent::EventType | event, |
int | key | ||
) | const |
Gets the mapped character for a key press event.
Definition at line 27 of file KeyMapper.cpp.
KeyMap& operator() | ( | KeyEvent::EventType | event, |
const std::string & | str | ||
) |
Adds key mappings based on the given string for a given event.
Definition at line 54 of file KeyMapper.h.
KeyMap& operator() | ( | KeyEvent::EventType | event, |
int | key, | ||
char | ch | ||
) |
Adds a key mapping for a key event & code combo.
Definition at line 70 of file KeyMapper.h.
KeyMap& operator() | ( | int | key, |
char | ch | ||
) |
Adds a key mapping for a key.
Definition at line 62 of file KeyMapper.h.
KeyMap& operator() | ( | const std::string & | str ) |
Adds key mappings based on the given string.
Definition at line 46 of file KeyMapper.h.
Generated on Thu Jul 14 2022 19:25:04 by
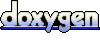