KeyboardManager: a class to manage the polling of a switch-matrix keyboard
Embed:
(wiki syntax)
Show/hide line numbers
KeyMapper.cpp
00001 #include "kbd_mgr/KeyMapper.h" 00002 #include <algorithm> 00003 #include <functional> 00004 00005 namespace kbd_mgr { 00006 00007 void KeyMap::addMapping(KeyEvent::EventType event, int key, char ch) 00008 { 00009 const KeyMapping *mapping = getMapping(event, key); 00010 if (mapping) { 00011 KeyMapping *updatable = const_cast<KeyMapping*>(mapping); 00012 updatable->ch = ch; 00013 } 00014 else { 00015 KeyMapping o(event, key, ch); 00016 this->map_.push_back(o); 00017 } 00018 } 00019 00020 void KeyMap::addMappings(KeyEvent::EventType event, const std::string &str) 00021 { 00022 for(int key = 0; key < str.size(); ++key) { 00023 addMapping(event, key, str[key]); 00024 } 00025 } 00026 00027 char KeyMap::map(KeyEvent::EventType event, int key) const 00028 { 00029 const KeyMapping *mapping = getMapping(event, key); 00030 if (!mapping) { 00031 mapping = getMapping(KeyEvent::NoEvent, key); 00032 } 00033 return (mapping ? mapping->ch : '\0'); 00034 } 00035 00036 const KeyMap::KeyMapping * KeyMap::getMapping(KeyEvent::EventType event, int key) const 00037 { 00038 std::pair<KeyEvent::EventType, int> criteria = std::make_pair(event, key); 00039 Map::const_iterator p = this->map_.begin(); 00040 while (p != this->map_.end() && !p->matches(criteria)) { 00041 ++p; 00042 } 00043 00044 if (p == this->map_.end()) { 00045 return NULL; 00046 } 00047 return &(*p); 00048 } 00049 00050 void KeyMapper::handleKeyPress(const KeyEvent &keypress) 00051 { 00052 char ch = map(keypress.event, keypress.keyCode); 00053 KeyEvent mapped(keypress, ch); 00054 invokeHandler(mapped); 00055 } 00056 00057 } // kbd_mgr
Generated on Thu Jul 14 2022 19:25:04 by
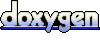