KeyboardManager: a class to manage the polling of a switch-matrix keyboard
Embed:
(wiki syntax)
Show/hide line numbers
KeyMapper.h
00001 #ifndef KEY_MAPPER_H_ 00002 #define KEY_MAPPER_H_ 00003 00004 #include "kbd_mgr/KeyPressEventServer.h" 00005 00006 #include <vector> 00007 #include <string> 00008 #include <utility> 00009 00010 namespace kbd_mgr { 00011 00012 /** 00013 * @brief A class used to store a set of key mappings. 00014 * It associates a key event and a key code to a mapped character. 00015 * It also allows to specify a mapping for any unmatched key event of a key. 00016 * The KeyMap can be set at construction time with a string and an optional key event. 00017 * Additional mappings can be specified by using the () operator. This way, both individual mappings and string mappings are possible. 00018 * Eg. keymap(KeyEvent::LongKeyPress, 1, '@')(1, '*') 00019 * maps the key 1 to '*' for all key presses but to '@' when a long key press occurs. 00020 */ 00021 class KeyMap { 00022 public: 00023 /** 00024 * @brief Creates an empty keymap. 00025 */ 00026 KeyMap() : map_() { } 00027 00028 /** 00029 * @brief Creates a keymap based on a string. 00030 * Each character is mapped to the key at that position (key 1 maps to str[1]). 00031 */ 00032 KeyMap(const std::string &str) : map_() { 00033 addMappings(KeyEvent::NoEvent, str); 00034 } 00035 /** 00036 * @brief Creates a keymap based on a string for a certain key event. 00037 * Each character is mapped to the key at that position (key 1 maps to str[1]). 00038 */ 00039 KeyMap(KeyEvent::EventType event, const std::string &str) : map_() { 00040 addMappings(event, str); 00041 } 00042 00043 /** 00044 * @brief Adds key mappings based on the given string. 00045 */ 00046 KeyMap& operator()(const std::string &str) { 00047 addMappings(KeyEvent::NoEvent, str); 00048 return *this; 00049 } 00050 00051 /** 00052 * @brief Adds key mappings based on the given string for a given event. 00053 */ 00054 KeyMap& operator()(KeyEvent::EventType event, const std::string &str) { 00055 addMappings(event, str); 00056 return *this; 00057 } 00058 00059 /** 00060 * @brief Adds a key mapping for a key. 00061 */ 00062 KeyMap& operator()(int key, char ch) { 00063 addMapping(KeyEvent::NoEvent, key, ch); 00064 return *this; 00065 } 00066 00067 /** 00068 * @brief Adds a key mapping for a key event & code combo. 00069 */ 00070 KeyMap& operator()(KeyEvent::EventType event, int key, char ch) { 00071 addMapping(event, key, ch); 00072 return *this; 00073 } 00074 00075 /** 00076 * @brief Gets the mapped character for a key press event. 00077 */ 00078 char map(KeyEvent::EventType event, int key) const; 00079 00080 private: 00081 struct KeyMapping { 00082 KeyEvent::EventType event; 00083 int key; 00084 char ch; 00085 00086 KeyMapping(KeyEvent::EventType event, int key, char ch) : event(event), key(key), ch(ch) { } 00087 00088 bool matches(const std::pair<KeyEvent::EventType, int> &arg) const { 00089 return this->event == arg.first && this->key == arg.second; 00090 } 00091 }; 00092 00093 void addMapping(KeyEvent::EventType event, int key, char ch); 00094 void addMappings(KeyEvent::EventType event, const std::string &str); 00095 const KeyMapping * getMapping(KeyEvent::EventType event, int key) const; 00096 00097 typedef std::vector<KeyMapping> Map; 00098 Map map_; 00099 }; 00100 00101 /** 00102 * @brief A key press event handler that adds a mapped key char to key events. 00103 * Mappings can be specified for any key press event or for some specific key press event. 00104 */ 00105 class KeyMapper : public KeyPressEventServer, public KeyPressEventHandler { 00106 public: 00107 00108 KeyMapper(const KeyMap &keymap = KeyMap()) : 00109 keymap_(keymap) 00110 { } 00111 00112 const KeyMap & keymap() const { return this->keymap_; } 00113 char map(KeyEvent::EventType event, int key) const { return this->keymap_.map(event, key); } 00114 00115 virtual void handleKeyPress(const KeyEvent &keypress); 00116 00117 private: 00118 KeyMap keymap_; 00119 }; 00120 00121 } // kbd_mgr 00122 00123 #endif // KEY_MAPPER_H_
Generated on Thu Jul 14 2022 19:25:04 by
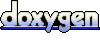