Library for creating menu systems in displays.
Menu Class Reference
Menu represents a container for a set of MenuNodes. More...
#include <Menu.h>
Inherits MenuNode.
Public Types | |
typedef void(* | SelectionChange )(MenuNode *) |
Defines a pointer to a functions that is called when the node is selected. | |
Public Member Functions | |
Menu (EnterMenu, SelectionChange, char *, int) | |
Constructs a new menu objects. | |
~Menu () | |
Clears down all contained MenuNodes. | |
void | addMenuNode (MenuNode &) |
Adds the given node to the Menu. | |
MenuNode & | getSelectedNode () |
Gets a pointer to the selected MenuNode. | |
int | size () |
Gets the number of MenuNodes contained by this Menu. | |
virtual void | enter () |
Enters this implementation of Menu. | |
void | up () |
Moves the selection up to the previous MenuNode if there is one and de-selects the current. | |
void | down () |
Moves the selection down to the next node if there is one and de-selects the current. | |
char * | getName () |
Gets the name of the node. | |
bool | isSelected () |
Determines wheter tgis instance is selected. | |
void | select () |
Selects this instance of MenuNode in its containing Menu and called the selected call back function. |
Detailed Description
Menu represents a container for a set of MenuNodes.
Menu is itself a menu node meaning that Menu objects can be used as sub menus.
Definition at line 80 of file Menu.h.
Member Typedef Documentation
typedef void(* SelectionChange)(MenuNode *) [inherited] |
Constructor & Destructor Documentation
Menu | ( | EnterMenu | enterAction, |
SelectionChange | selectionChangeAct, | ||
char * | newName, | ||
int | size | ||
) |
Constructs a new menu objects.
void enterMenu(Menu *menu) { } void selectNode(MenuNode *node) { } Menu root(&enterMenu, &selectNode, "root", 5);
Member Function Documentation
void addMenuNode | ( | MenuNode & | node ) |
Adds the given node to the Menu.
MenuNodes are ordered by the order in which they are added. Note that a Menu is also a MenuNode meaning that a menu can bee added as a MenuNode to create a sub Menu.
Menu root(&enterMenu, &selectNode, "root", 5); void main() { Menu *sub1 = new Menu(&enterMenu, &selectNode, "Settings", 5); MenuNode *ipnode = new MenuNode(&enterNode, &setip, "IP Address"); MenuNode *netmasknode = new MenuNode(&enterNode, &setmask, "Net mask"); sub1->addMenuNode(*ipnode); sub1->addMenuNode(*netmasknode); root.addMenuNode(*sub1); root.enter(); }
void down | ( | ) |
void enter | ( | ) | [virtual] |
char * getName | ( | ) | [inherited] |
MenuNode & getSelectedNode | ( | ) |
bool isSelected | ( | ) | [inherited] |
void select | ( | ) | [inherited] |
int size | ( | ) |
Generated on Wed Jul 13 2022 20:05:17 by
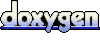