Library for creating menu systems in displays.
Embed:
(wiki syntax)
Show/hide line numbers
Menu.h
00001 #ifndef MENU_H 00002 #define MENU_H 00003 00004 #include <mbed.h> 00005 00006 class Menu; 00007 00008 /** MenuNode represents an entry in a menu. Each menu node is instantiated with 00009 * two callbacks, one for selecting the node and one for entering the node. 00010 * Methods are provided for determining whether the node is selected and 00011 * retrieving the row number set by the containing menu. 00012 */ 00013 class MenuNode { 00014 00015 private: 00016 typedef void (*EnterMenuNode)(MenuNode*); 00017 void (*entered)(MenuNode*); 00018 bool selected; 00019 int row; 00020 friend class Menu; 00021 public: 00022 00023 /** Defines a pointer to a functions that is called when the node is selected. 00024 */ 00025 typedef void (*SelectionChange)(MenuNode*); 00026 00027 /** Constructs a new MenuNode object. The constructor takes two function pointers as 00028 * arguments both of which must take a single argument of a MenuNode pointer. 00029 * 00030 * @code 00031 * 00032 * void enterNode(MenuNode *node) { 00033 * 00034 * } 00035 * 00036 * void selectNode(MenuNode *node) { 00037 * 00038 * } 00039 * 00040 * main() { 00041 * MenuNode *node = new MenuNode(&enterNode, &selectNode, "name"); 00042 * } 00043 * 00044 * @endcode 00045 * 00046 * @param EnterMenuNode A pointer to a callback fuction called when the node is entered. 00047 * @param SelectionChange A pointer to a callback fuction called when the node is selected. 00048 * @param name The name of the MenuNode. 00049 */ 00050 MenuNode(EnterMenuNode, SelectionChange, char*); 00051 00052 /** Gets the name of the node. 00053 * @return A char array containing the node name. 00054 */ 00055 char* getName(); 00056 00057 /** Enters this instance of MenuNode and ultimatley calls the entered callback function. 00058 */ 00059 virtual void enter(); 00060 00061 /** Determines wheter tgis instance is selected. 00062 * @return true if selected. 00063 */ 00064 bool isSelected(); 00065 00066 /** Selects this instance of MenuNode in its containing Menu and called the selected call back function. 00067 */ 00068 void select(); 00069 00070 00071 protected: 00072 char* name; 00073 void (*selection)(MenuNode*); 00074 MenuNode(); 00075 }; 00076 00077 /** Menu represents a container for a set of MenuNodes. Menu is itself 00078 * a menu node meaning that Menu objects can be used as sub menus. 00079 */ 00080 class Menu : public MenuNode { 00081 00082 private: 00083 typedef void (*EnterMenu)(Menu*); 00084 MenuNode **nodes; 00085 void (*entered)(Menu*); 00086 int selectedNode; 00087 int nodeCount; 00088 public: 00089 00090 /** Constructs a new menu objects. 00091 * 00092 * @code 00093 * void enterMenu(Menu *menu) { 00094 * 00095 * } 00096 * 00097 * void selectNode(MenuNode *node) { 00098 * 00099 * } 00100 * 00101 * Menu root(&enterMenu, &selectNode, "root", 5); 00102 * 00103 * @endcode 00104 * 00105 * @param EnterMenu A pointer to a functions to be called when the Menu is entered. 00106 * @param SelectionChange A pointer to a function to be called when the Menu is selected. 00107 * @param char* A char array containing the name of the Menu. 00108 * @param int The number of node that this Menu will contain. 00109 */ 00110 Menu(EnterMenu, SelectionChange, char*, int); 00111 00112 /** Clears down all contained MenuNodes*/ 00113 ~Menu(); 00114 00115 /** Adds the given node to the Menu. MenuNodes are ordered by the order in which they are added. 00116 * Note that a Menu is also a MenuNode meaning that a menu can bee added as a MenuNode to create a sub Menu. 00117 * 00118 * @code 00119 * Menu root(&enterMenu, &selectNode, "root", 5); 00120 * 00121 * void main() { 00122 * Menu *sub1 = new Menu(&enterMenu, &selectNode, "Settings", 5); 00123 * MenuNode *ipnode = new MenuNode(&enterNode, &setip, "IP Address"); 00124 * MenuNode *netmasknode = new MenuNode(&enterNode, &setmask, "Net mask"); 00125 * sub1->addMenuNode(*ipnode); 00126 * sub1->addMenuNode(*netmasknode); 00127 * root.addMenuNode(*sub1); 00128 * root.enter(); 00129 * } 00130 * @endcode 00131 * 00132 * @para MenuNode A reference to the MenuNode to add. 00133 */ 00134 void addMenuNode(MenuNode&); 00135 00136 /** Gets a pointer to the selected MenuNode. 00137 * @return A pointer to the selected MenuNode. 00138 */ 00139 MenuNode & getSelectedNode(); 00140 00141 /** Gets the number of MenuNodes contained by this Menu. 00142 * @return The MenuNode count. 00143 */ 00144 int size(); 00145 00146 /** Enters this implementation of Menu. */ 00147 virtual void enter(); 00148 00149 /** Moves the selection up to the previous MenuNode if there is one and de-selects the current. */ 00150 void up(); 00151 00152 /** Moves the selection down to the next node if there is one and de-selects the current. */ 00153 void down(); 00154 }; 00155 00156 #endif
Generated on Wed Jul 13 2022 20:05:17 by
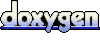