example project to explain how to write a class library
Embed:
(wiki syntax)
Show/hide line numbers
test_LM75B.h
00001 /** サンプルコード:ライブラリ開発の例 00002 * 00003 * @author Tedd OKANO 00004 * @version 1.1 00005 * @date 11-Nov-2014 00006 * 00007 * ライブラリ開発の例として,コードをステップ・バイ・ステップで解説しています 00008 * 各ステップはリポジトリ履歴で追うことができます 00009 * I2Cインターフェースを持った温度センサのLM75Bをターゲットとしています 00010 * 00011 * LM75Bの詳細は 00012 * http://www.nxp.com/documents/data_sheet/LM75B.pdf 00013 */ 00014 00015 #include "mbed.h" 00016 00017 /** デフォルト・スレーブアドレス */ 00018 #define ADDRESS_LM75B 0x90 00019 00020 /** LM75Bのレジスタ名とアドレス */ 00021 #define LM75B_Conf 0x01 00022 #define LM75B_Temp 0x00 00023 #define LM75B_Tos 0x03 00024 #define LM75B_Thyst 0x02 00025 00026 /** test_LM75Bクラスライブラリ 00027 * 00028 * クラスライブラリは非常にシンプルなインターフェースを提供します 00029 * 00030 * コード例: 00031 * @code 00032 * #include "mbed.h" 00033 * #include "test_LM75B.h" 00034 * 00035 * test_LM75B temp0( p28, p27 ); 00036 * 00037 * I2C i2c( p28, p27 ); 00038 * test_LM75B temp1( i2c ); 00039 * 00040 * 00041 * int main() 00042 * { 00043 * float t0; 00044 * float t1; 00045 * 00046 * i2c.frequency( 400 * 1000 ); 00047 * 00048 * while(1) { 00049 * t0 = temp0; 00050 * t1 = temp1; 00051 * printf( "temp = %7.3f, %7.3f\r\n", t0, t1 ); 00052 * wait( 1 ); 00053 * } 00054 * } 00055 * @endcode 00056 */ 00057 class test_LM75B 00058 { 00059 public: 00060 00061 /** I2Cピンとスレーブアドレスを指定し,インスタンスを作成します 00062 * 00063 * @param sda I2C-bus SDAピン 00064 * @param scl I2C-bus SCLピン 00065 * @param address (オプション) I2C-bus スレーブアドレス (デフォルト: 0x90) 00066 */ 00067 test_LM75B( PinName sda, PinName scl, char address = ADDRESS_LM75B ); 00068 00069 /** I2Cオブジェクトとスレーブアドレスを指定し,インスタンスを作成します 00070 * 00071 * @param i2c_obj I2C オブジェクト (インスタンス) 00072 * @param address (オプション) I2C-bus スレーブアドレス (デフォルト: 0x90) 00073 */ 00074 test_LM75B( I2C &i2c_obj, char address = ADDRESS_LM75B ); 00075 00076 /** デストラクタ 00077 */ 00078 ~test_LM75B(); 00079 00080 /** 初期化 00081 */ 00082 void init( void ); 00083 00084 /** 温度の読み出し 00085 * 00086 * @return 摂氏温度を返します(float型) 00087 */ 00088 float read( void ); 00089 00090 /** 温度の読み出し 00091 * 00092 * @return オブジェクトが読みだした値を返すようにしています 00093 */ 00094 operator float( void ); 00095 00096 private: 00097 I2C *i2c_p; 00098 I2C &i2c; 00099 char adr; 00100 };
Generated on Fri May 17 2024 16:10:41 by
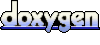