PCA9547: an I2C bus multiplexer control library. PCA9547 is an I2C multiplexer which enables to select 1:8 multiplexed I2C bus. The multiplexer is useful for deviding I2C bus to avoiding slave address conflict and separating capacitive loads. For more information about PCA9547: http://www.nxp.com/documents/data_sheet/PCA9547.pdf
Dependents: pca9547_Hello m3Dpi
PCA9547.h
00001 /** 00002 * PCA9547 library 00003 * 00004 * @author Tedd OKANO 00005 * @version 0.2 00006 * @date Feb-2015 00007 * 00008 * PCA9547: an I2C bus multiplexer control library 00009 * 00010 * PCA9547 is an I2C multiplexer which enables to select 1:8 multiplexed I2C bus. 00011 * The multiplexer is useful for deviding I2C bus to avoiding slave address conflict and separating capacitive loads. 00012 * 00013 * For more information about PCA9547: 00014 * http://www.nxp.com/documents/data_sheet/PCA9547.pdf 00015 * 00016 */ 00017 00018 #ifndef MBED_PCA9547_H 00019 #define MBED_PCA9547_H 00020 00021 #include "mbed.h" 00022 00023 /** PCA9547 class 00024 * 00025 * PCA9547: an I2C bus multiplexer control library 00026 * 00027 * PCA9547 is an I2C multiplexer which enables to select 1:8 multiplexed I2C bus. 00028 * The multiplexer is useful for deviding I2C bus to avoiding slave address conflict and separating capacitive loads. 00029 * 00030 * For more informatioj about PCA9547: 00031 * http://www.nxp.com/documents/data_sheet/PCA9547.pdf 00032 * 00033 * Example: 00034 * @code 00035 * #include "mbed.h" 00036 * #include "LM75B.h" 00037 * #include "PCA9547.h" 00038 * 00039 * PCA9547 mux( p28, p27, 0xE0 ); 00040 * 00041 * int main() 00042 * { 00043 * mux.select( 0 ); 00044 * 00045 * LM75B tmp0( p28, p27 ); // making instance after a branch of I2C bus (which is connecting the LM75B) enabled 00046 * 00047 * while(1) { 00048 * printf( "%.3f\r\n", tmp0.read() ); 00049 * wait( 1.0 ); 00050 * } 00051 * } 00052 * @endcode 00053 */ 00054 00055 class PCA9547 00056 { 00057 public: 00058 00059 /** Create a PCA9547 instance connected to specified I2C pins with specified address 00060 * 00061 * @param sda I2C-bus SDA pin 00062 * @param scl I2C-bus SCL pin 00063 * @param i2c_address I2C-bus address (default: 0xE0) 00064 */ 00065 PCA9547( PinName sda, PinName scl, char i2c_address = 0xE0 ); 00066 00067 /** Create a PCA9546A instance connected to specified I2C pins with specified address 00068 * 00069 * @param &i2c_ I2C object (instance) 00070 * @param i2c_address I2C-bus address (default: 0xE0) 00071 */ 00072 PCA9547( I2C &i2c_, char i2c_address = 0xE0 ); 00073 00074 /** Destructor of PCA9547 00075 */ 00076 ~PCA9547(); 00077 00078 /** Channel select 00079 * 00080 * Enable and select a channel 00081 * 00082 * @param channel channel number 00083 */ 00084 void select( char channel ); 00085 00086 /** Disabling all channels 00087 * 00088 * Disable all channels 00089 */ 00090 void disable( void ); 00091 00092 private: 00093 I2C *i2c_p; 00094 I2C &i2c; 00095 char i2c_addr; 00096 }; 00097 00098 #endif // MBED_PCA9547_H
Generated on Tue Jul 12 2022 19:04:32 by
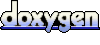