modified by ohneta
Dependents: HelloESP8266Interface_mine
Fork of NetworkSocketAPI by
WiFiInterface Class Reference
WiFiInterface class. More...
#include <WiFiInterface.h>
Inherits NetworkInterface.
Public Member Functions | |
virtual int32_t | connect (const char *ap, const char *pass_phrase=0, wifi_security_t security=WI_NONE, uint32_t timeout_ms=15000)=0 |
Start the interface using ap name, phrase and security attributes. | |
virtual int32_t | init (void)=0 |
Initialize the network interface with DHCP. | |
virtual int32_t | init (const char *ip, const char *mask, const char *gateway)=0 |
Initialize the network interface with a static IP address. | |
virtual int32_t | connect (uint32_t timeout_ms=15000)=0 |
Start the interface, using DHCP if needed. | |
virtual int32_t | disconnect (void)=0 |
Stop the interface, bringing down dhcp if necessary. | |
virtual char * | getIPAddress (void)=0 |
Get the current IP address. | |
virtual char * | getGateway (void) const =0 |
Get the current gateway address. | |
virtual char * | getNetworkMask (void) const =0 |
Get the current network mask. | |
virtual char * | getMACAddress (void) const =0 |
Get the devices MAC address. | |
virtual int32_t | isConnected (void)=0 |
Get the current status of the interface connection. | |
virtual SocketInterface * | allocateSocket (socket_protocol_t socketProtocol)=0 |
Allocate space for a socket. | |
virtual int | deallocateSocket (SocketInterface *socket)=0 |
Deallocate space for a socket. | |
Protected Attributes | |
std::map< uint32_t, SocketInterface * > | sockets |
Map used to keep track of all SocketInterface instances. | |
uint32_t | uuidCounter |
Counter used to create unique handles for new sockets. |
Detailed Description
WiFiInterface class.
This is a common interface to handle how WiFi connects to an access point
Definition at line 34 of file WiFiInterface.h.
Member Function Documentation
virtual SocketInterface* allocateSocket | ( | socket_protocol_t | socketProtocol ) | [pure virtual, inherited] |
Allocate space for a socket.
- Parameters:
-
The type of socket you want to open, SOCK_TCP or SOCK_UDP
- Returns:
- a pointer to the allocated socket.
virtual int32_t connect | ( | const char * | ap, |
const char * | pass_phrase = 0 , |
||
wifi_security_t | security = WI_NONE , |
||
uint32_t | timeout_ms = 15000 |
||
) | [pure virtual] |
Start the interface using ap name, phrase and security attributes.
- Parameters:
-
ap Name of the network the radio should connect to pass_pharase The security phrase needed for connecting to the network security Type of encryption the network requires for connection timeout_ms Time in miliseconds to wait while attempting to connect before timing out
- Returns:
- 0 on success, a negative number on failure
virtual int32_t connect | ( | uint32_t | timeout_ms = 15000 ) |
[pure virtual, inherited] |
Start the interface, using DHCP if needed.
- Parameters:
-
timeout_ms Time in miliseconds to wait while attempting to connect before timing out
- Returns:
- 0 on success, a negative number on failure
virtual int deallocateSocket | ( | SocketInterface * | socket ) | [pure virtual, inherited] |
Deallocate space for a socket.
- Parameters:
-
An allocated SocketInterface
- Returns:
- 0 if object is destroyed, -1 otherwise
virtual int32_t disconnect | ( | void | ) | [pure virtual, inherited] |
Stop the interface, bringing down dhcp if necessary.
- Returns:
- 0 on success, a negative number on failure
virtual char* getGateway | ( | void | ) | const [pure virtual, inherited] |
Get the current gateway address.
- Returns:
- a pointer to a string containing the gateway address.
virtual char* getIPAddress | ( | void | ) | [pure virtual, inherited] |
Get the current IP address.
- Returns:
- a pointer to a string containing the IP address.
virtual char* getMACAddress | ( | void | ) | const [pure virtual, inherited] |
Get the devices MAC address.
- Returns:
- a pointer to a string containing the mac address.
virtual char* getNetworkMask | ( | void | ) | const [pure virtual, inherited] |
Get the current network mask.
- Returns:
- a pointer to a string containing the network mask.
virtual int32_t init | ( | const char * | ip, |
const char * | mask, | ||
const char * | gateway | ||
) | [pure virtual, inherited] |
Initialize the network interface with a static IP address.
- Parameters:
-
ip The static IP address to use mask The IP address mask gateway The gateway to use
- Returns:
- 0 on success, a negative number on failure
virtual int32_t init | ( | void | ) | [pure virtual, inherited] |
Initialize the network interface with DHCP.
- Returns:
- 0 on success, a negative number on failure
virtual int32_t isConnected | ( | void | ) | [pure virtual, inherited] |
Get the current status of the interface connection.
- Returns:
- true if connected, a negative number on failure
Field Documentation
std::map<uint32_t, SocketInterface*> sockets [protected, inherited] |
Map used to keep track of all SocketInterface instances.
Definition at line 97 of file NetworkInterface.h.
uint32_t uuidCounter [protected, inherited] |
Counter used to create unique handles for new sockets.
Should be incremented whenever a new socket is created.
Definition at line 102 of file NetworkInterface.h.
Generated on Tue Jul 12 2022 18:45:36 by
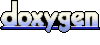