modified by ohneta
Dependents: HelloESP8266Interface_mine
Fork of NetworkSocketAPI by
NetworkInterface.h
00001 /* NetworkInterface Base Class 00002 * Copyright (c) 2015 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef NETWORKINTERFACE_H 00018 #define NETWORKINTERFACE_H 00019 00020 #include "stdint.h" 00021 #include "SocketInterface.h" 00022 #include <map> 00023 00024 /** NetworkInterface class. 00025 This is a common interface that is shared between all hardware that connect 00026 to a network over IP. 00027 */ 00028 class NetworkInterface 00029 { 00030 public: 00031 00032 /** Initialize the network interface with DHCP. 00033 @returns 0 on success, a negative number on failure 00034 */ 00035 virtual int32_t init(void) = 0; 00036 00037 /** Initialize the network interface with a static IP address. 00038 @param ip The static IP address to use 00039 @param mask The IP address mask 00040 @param gateway The gateway to use 00041 @returns 0 on success, a negative number on failure 00042 */ 00043 virtual int32_t init(const char *ip, const char *mask, const char *gateway) = 0; 00044 00045 00046 /** Start the interface, using DHCP if needed. 00047 @param timeout_ms Time in miliseconds to wait while attempting to connect before timing out 00048 @returns 0 on success, a negative number on failure 00049 */ 00050 virtual int32_t connect(uint32_t timeout_ms = 15000) = 0; 00051 00052 /** Stop the interface, bringing down dhcp if necessary. 00053 @returns 0 on success, a negative number on failure 00054 */ 00055 virtual int32_t disconnect(void) = 0; 00056 00057 /** Get the current IP address. 00058 @returns a pointer to a string containing the IP address. 00059 */ 00060 virtual char *getIPAddress(void) = 0; 00061 00062 /** Get the current gateway address. 00063 @returns a pointer to a string containing the gateway address. 00064 */ 00065 virtual char *getGateway(void) const = 0; 00066 00067 00068 /** Get the current network mask. 00069 @returns a pointer to a string containing the network mask. 00070 */ 00071 virtual char *getNetworkMask(void) const = 0; 00072 00073 /** Get the devices MAC address. 00074 @returns a pointer to a string containing the mac address. 00075 */ 00076 virtual char *getMACAddress(void) const = 0; 00077 00078 /** Get the current status of the interface connection. 00079 @returns true if connected, a negative number on failure 00080 */ 00081 virtual int32_t isConnected(void) = 0; 00082 00083 /** Allocate space for a socket. 00084 @param The type of socket you want to open, SOCK_TCP or SOCK_UDP 00085 @returns a pointer to the allocated socket. 00086 */ 00087 virtual SocketInterface* allocateSocket(socket_protocol_t socketProtocol) = 0; 00088 00089 /** Deallocate space for a socket. 00090 @param An allocated SocketInterface 00091 @returns 0 if object is destroyed, -1 otherwise 00092 */ 00093 virtual int deallocateSocket(SocketInterface* socket) = 0; 00094 00095 protected: 00096 /** Map used to keep track of all SocketInterface instances */ 00097 std::map<uint32_t, SocketInterface*> sockets; 00098 00099 /** Counter used to create unique handles for new sockets. 00100 Should be incremented whenever a new socket is created. 00101 */ 00102 uint32_t uuidCounter; 00103 }; 00104 00105 #endif
Generated on Tue Jul 12 2022 18:45:36 by
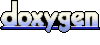