Library for "I2C 8x8 LED matrix board" from Switch Science https://www.switch-science.com/catalog/2071/
Dependents: PCA9622_LED8x8_Demo PCA9622_LED8x8_Hello PCA9622_LED8x8_x6_Demo shake-shake-machine
PCA9622_LED8x8 Class Reference
PCA9622_LED8x8 class. More...
#include <PCA9622_LED8x8.h>
Public Member Functions | |
PCA9622_LED8x8 (PinName sda, PinName scl, char slave_adr=0xA0, float fr=DEFAULT_FRAMERATE) | |
Create a PCA9622_LED8x8 instance for the LED matrix board which is connected to specified I2C pins with specified address. | |
PCA9622_LED8x8 (I2C &i2c_obj, char slave_adr=0xA0, float fr=DEFAULT_FRAMERATE) | |
Create a PCA9622_LED8x8 instance for the LED matrix board which is connected to specified I2C pins with specified address. | |
~PCA9622_LED8x8 () | |
Destructor of PCA9622_LED8x8. | |
void | frame_rate (float rate) |
Frame rate change. | |
void | start (void) |
Start operation. | |
void | stop (void) |
Stop operation. | |
void | set_data (float p[8][8]) |
Set the image to library internal buffer. |
Detailed Description
PCA9622_LED8x8 class.
PCA9622_LED8x8: A library to control LED matrix using the PCA9622
PCA9622 is an I2C LED controller. This library operates LED 8x8 matrix through the PCA9622.
Example:
#include "mbed.h" #include "PCA9622_LED8x8.h" PCA9622_LED8x8 matrix( p28, p27 ); // for 40pin type mbed //PCA9622_LED8x8 matrix( D14, D15 ); // for Arduino type mbed //PCA9622_LED8x8 matrix( dp5, dp27 ); // for mbed LPC1114 float func( float x, float y, float t ); // function to make 8x8 image int main() { float image[ 8 ][ 8 ]; // int count = 0; matrix.start(); while(1) { // making 8x8 image to "image" array for ( int i = 0; i < 8; i++ ) for ( int j = 0; j < 8; j++ ) image[ i ][ j ] = func( i, j, count * 0.2 ); matrix.set_data( image ); count++; wait( 0.05 ); } } float func( float x, float y, float t ) { //#define DISPLAY_OFFSET 3.5 #define DISPLAY_OFFSET 0 #define SIZE 0.3 float s; x = (x - DISPLAY_OFFSET) * SIZE; y = (y - DISPLAY_OFFSET) * SIZE; s = cos( powf( x * x + y * y, 0.5 ) - t ); return ( powf( s, 4.0 ) ); }
Definition at line 79 of file PCA9622_LED8x8.h.
Constructor & Destructor Documentation
PCA9622_LED8x8 | ( | PinName | sda, |
PinName | scl, | ||
char | slave_adr = 0xA0 , |
||
float | fr = DEFAULT_FRAMERATE |
||
) |
Create a PCA9622_LED8x8 instance for the LED matrix board which is connected to specified I2C pins with specified address.
PCA9622 LED 8x8 library.
- Parameters:
-
sda I2C-bus SDA pin scl I2C-bus SCL pin slave_adr (option) I2C-bus address (default: 0xA0) fr (option) frame rate (in "Hz". default: 100)
- Version:
- 1.1.1
- Date:
- 26-Feb-2015
Library for "I2C 8x8 LED matrix board" from Switch Science https://www.switch-science.com/catalog/2071/
The I2C LED controller PCA9622 is used on this module that ebables to control the LEDs with PWM brightness control.
For more information about the PCA9622: http://www.nxp.com/documents/data_sheet/PCA9622.pdf
Definition at line 21 of file PCA9622_LED8x8.cpp.
PCA9622_LED8x8 | ( | I2C & | i2c_obj, |
char | slave_adr = 0xA0 , |
||
float | fr = DEFAULT_FRAMERATE |
||
) |
Create a PCA9622_LED8x8 instance for the LED matrix board which is connected to specified I2C pins with specified address.
- Parameters:
-
i2c_obj I2C object (instance) slave_adr (option) I2C-bus address (default: 0xA0) fr (option) frame rate (in "Hz". default: 100)
Definition at line 36 of file PCA9622_LED8x8.cpp.
~PCA9622_LED8x8 | ( | ) |
Destructor of PCA9622_LED8x8.
Definition at line 51 of file PCA9622_LED8x8.cpp.
Member Function Documentation
void frame_rate | ( | float | rate ) |
Frame rate change.
Changes frame rate (scan rate of the matrix)
- Parameters:
-
rate Frame rate in "Hz"
Definition at line 71 of file PCA9622_LED8x8.cpp.
void set_data | ( | float | p[8][8] ) |
Set the image to library internal buffer.
8x8 pixel image will be copied into the library internal buffer. User don't need to care the timing of this function call because the library is using "ping-pong" buffering. This function writes data into in-active side of the buffer. The image will not be appear immediatly but from next scan start.
Each elements of the array should be brightness information in range of 0.0 to 1.0.
The 2 dimensional 8x8 array will be handled as..
p[0][0] : top-left p[0][7] : top-right p[7][0] : bottm-left p[7][7] : bottom-right
- Parameters:
-
p image stored 2-dimensional float array
Definition at line 129 of file PCA9622_LED8x8.cpp.
void start | ( | void | ) |
Start operation.
The LED matrix operation need to be started with this function. Scan line drawing by Ticker interrupt will be started.
Definition at line 84 of file PCA9622_LED8x8.cpp.
void stop | ( | void | ) |
Stop operation.
This stops the operation. Ticker interrupt will be stopped
Definition at line 90 of file PCA9622_LED8x8.cpp.
Generated on Wed Jul 13 2022 20:20:15 by
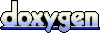