Library for "I2C 8x8 LED matrix board" from Switch Science https://www.switch-science.com/catalog/2071/
Dependents: PCA9622_LED8x8_Demo PCA9622_LED8x8_Hello PCA9622_LED8x8_x6_Demo shake-shake-machine
PCA9622_LED8x8.h
00001 /** 00002 * PCA9622 LED 8x8 library 00003 * 00004 * @author Tedd OKANO 00005 * @version 1.1.1 00006 * @date 26-Feb-2015 00007 * 00008 * Library for "I2C 8x8 LED matrix board" from Switch Science 00009 * https://www.switch-science.com/catalog/2071/ 00010 * 00011 * The I2C LED controller PCA9622 is used on this module 00012 * This LED controller chip enables brightness control by PWM. 00013 * 00014 * For more information about the PCA9622: 00015 * http://www.nxp.com/documents/data_sheet/PCA9622.pdf 00016 */ 00017 00018 #ifndef MBED_PCA9622_LED8x8_H 00019 #define MBED_PCA9622_LED8x8_H 00020 00021 #include "mbed.h" 00022 00023 /** PCA9622_LED8x8 class 00024 * 00025 * PCA9622_LED8x8: A library to control LED matrix using the PCA9622 00026 * 00027 * PCA9622 is an I2C LED controller. 00028 * This library operates LED 8x8 matrix through the PCA9622. 00029 * 00030 * Example: 00031 * @code 00032 * #include "mbed.h" 00033 * #include "PCA9622_LED8x8.h" 00034 * 00035 * PCA9622_LED8x8 matrix( p28, p27 ); // for 40pin type mbed 00036 * //PCA9622_LED8x8 matrix( D14, D15 ); // for Arduino type mbed 00037 * //PCA9622_LED8x8 matrix( dp5, dp27 ); // for mbed LPC1114 00038 * 00039 * float func( float x, float y, float t ); // function to make 8x8 image 00040 * 00041 * int main() 00042 * { 00043 * float image[ 8 ][ 8 ]; // 00044 * int count = 0; 00045 * 00046 * matrix.start(); 00047 * 00048 * while(1) { 00049 * 00050 * // making 8x8 image to "image" array 00051 * for ( int i = 0; i < 8; i++ ) 00052 * for ( int j = 0; j < 8; j++ ) 00053 * image[ i ][ j ] = func( i, j, count * 0.2 ); 00054 * 00055 * matrix.set_data( image ); 00056 * 00057 * count++; 00058 * wait( 0.05 ); 00059 * } 00060 * } 00061 * 00062 * float func( float x, float y, float t ) 00063 * { 00064 * //#define DISPLAY_OFFSET 3.5 00065 * #define DISPLAY_OFFSET 0 00066 * #define SIZE 0.3 00067 * 00068 * float s; 00069 * 00070 * x = (x - DISPLAY_OFFSET) * SIZE; 00071 * y = (y - DISPLAY_OFFSET) * SIZE; 00072 * 00073 * s = cos( powf( x * x + y * y, 0.5 ) - t ); 00074 * return ( powf( s, 4.0 ) ); 00075 * } 00076 * @endcode 00077 */ 00078 00079 class PCA9622_LED8x8 00080 { 00081 public: 00082 enum FrameRate { DEFAULT_FRAMERATE = 50 }; 00083 00084 /** Create a PCA9622_LED8x8 instance for the LED matrix board 00085 * which is connected to specified I2C pins with specified address 00086 * 00087 * @param sda I2C-bus SDA pin 00088 * @param scl I2C-bus SCL pin 00089 * @param slave_adr (option) I2C-bus address (default: 0xA0) 00090 * @param fr (option) frame rate (in "Hz". default: 100) 00091 */ 00092 PCA9622_LED8x8( PinName sda, PinName scl, char slave_adr = 0xA0, float fr = DEFAULT_FRAMERATE ); 00093 00094 /** Create a PCA9622_LED8x8 instance for the LED matrix board 00095 * which is connected to specified I2C pins with specified address 00096 * 00097 * @param i2c_obj I2C object (instance) 00098 * @param slave_adr (option) I2C-bus address (default: 0xA0) 00099 * @param fr (option) frame rate (in "Hz". default: 100) 00100 */ 00101 PCA9622_LED8x8( I2C &i2c_obj, char slave_adr = 0xA0, float fr = DEFAULT_FRAMERATE ); 00102 00103 /** Destructor of PCA9622_LED8x8 00104 */ 00105 ~PCA9622_LED8x8(); 00106 00107 /** Frame rate change 00108 * 00109 * Changes frame rate (scan rate of the matrix) 00110 * 00111 * @param rate Frame rate in "Hz" 00112 */ 00113 void frame_rate( float rate ); 00114 00115 /** Start operation 00116 * 00117 * The LED matrix operation need to be started with this function. 00118 * Scan line drawing by Ticker interrupt will be started. 00119 */ 00120 void start( void ); 00121 00122 /** Stop operation 00123 * 00124 * This stops the operation. 00125 * Ticker interrupt will be stopped 00126 */ 00127 void stop( void ); 00128 00129 /** Set the image to library internal buffer 00130 * 00131 * 8x8 pixel image will be copied into the library internal buffer. 00132 * User don't need to care the timing of this function call because 00133 * the library is using "ping-pong" buffering. 00134 * This function writes data into in-active side of the buffer. 00135 * The image will not be appear immediatly but from next scan start. 00136 * 00137 * Each elements of the array should be brightness information 00138 * in range of 0.0 to 1.0. 00139 * 00140 * The 2 dimensional 8x8 array will be handled as.. 00141 * 00142 * p[0][0] : top-left 00143 * p[0][7] : top-right 00144 * p[7][0] : bottm-left 00145 * p[7][7] : bottom-right 00146 * 00147 * @param p image stored 2-dimensional float array 00148 */ 00149 void set_data( float p[ 8 ][ 8 ] ); 00150 00151 private: 00152 I2C *i2c_p; 00153 I2C &i2c; 00154 char address; // I2C slave address 00155 float framerate; // frame rate in "Hz" 00156 int in_operation; // ture, if scan is on-going 00157 int line_counter; // counting scan line 00158 int frame_counter; // counting frame number 00159 int buffer_switch_request; // flag to switch the ping-pong buffer 00160 int outgoing_buffer; // flag to select the buffer 00161 Ticker t; 00162 char pattern[ 2 ][ 8 ][ 8 ]; // having 2 sets of 8x8 array for pin-pong buffering 00163 00164 void draw_a_line( void ); // ISR called by Ticker 00165 void initialize( void ); // initialize function 00166 }; 00167 00168 #endif // MBED_PCA9622_LED8x8_H
Generated on Wed Jul 13 2022 20:20:15 by
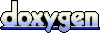