This library provides simple interface for the table football goal counter based on a IR LED or laser diode and phototransistor.
Dependents: goal_counter_project
GoalCounter Class Reference
GoalCounter class. More...
#include <GoalCounter.h>
Public Member Functions | |
GoalCounter (PinName pin, Timer *t) | |
Constructor. | |
~GoalCounter () | |
Destructor. | |
void | tstart () |
Reads value of the timer, this function is called from the interrupt pin fall edge callback. | |
void | tstop () |
Reads value from the timer and measures interval between end and beginning Called from the interrupt pin rise edge callback. | |
uint8_t | get_score () |
Returns score. | |
float | get_balltime (uint8_t score) |
Returns time of a ball pass for a given score. | |
float | get_balltime () |
Returns time of a ball pass for the current score. | |
float | get_ballspeed (uint8_t score) |
Returns speed of a ball pass for a given score. | |
float | get_ballspeed () |
Returns speed of a ball pass for the current score. | |
Data Fields | |
volatile uint8_t | is_goal |
Variable is 1 (true) when the goal is detected. |
Detailed Description
GoalCounter class.
Simple implementation of goal counter for table football using laser diode and phototransistor. Output of the phototransistor is connected to the digital input pin of MCU. When the laser beam is interrupted by ball passing the the cage Timer starts counting and measuring width of the pulse and if the duration of pulse is not longer than two seconds, goal is detected and _score variable is incremented.
Pulse duration is also used for the calculation of ball passing speed. However, measurement is not precise.
Example:
#include "mbed.h" #include "GoalCounter.h" Timer t; GoalCounter gc(D2, &t); int main() { while(1) { if (gc.is_goal) goal(); // call some function } }
Definition at line 36 of file GoalCounter.h.
Constructor & Destructor Documentation
GoalCounter | ( | PinName | pin, |
Timer * | t | ||
) |
Constructor.
- Parameters:
-
pin Input pin where is the outpout of phototransistor connected timer reference to the Timer instance
Definition at line 3 of file GoalCounter.cpp.
~GoalCounter | ( | ) |
Member Function Documentation
float get_ballspeed | ( | uint8_t | score ) |
Returns speed of a ball pass for a given score.
- Parameters:
-
score of the game
- Returns:
- speed of a ball pass in kph
Definition at line 61 of file GoalCounter.cpp.
float get_ballspeed | ( | ) |
Returns speed of a ball pass for the current score.
- Returns:
- speed of a ball pass in kph
Definition at line 70 of file GoalCounter.cpp.
float get_balltime | ( | ) |
Returns time of a ball pass for the current score.
- Returns:
- time of a ball pass in seconds
Definition at line 56 of file GoalCounter.cpp.
float get_balltime | ( | uint8_t | score ) |
Returns time of a ball pass for a given score.
- Parameters:
-
score of the game
- Returns:
- time of a ball pass in seconds
Definition at line 49 of file GoalCounter.cpp.
uint8_t get_score | ( | ) |
void tstart | ( | ) |
Reads value of the timer, this function is called from the interrupt pin fall edge callback.
Definition at line 27 of file GoalCounter.cpp.
void tstop | ( | ) |
Reads value from the timer and measures interval between end and beginning Called from the interrupt pin rise edge callback.
Definition at line 31 of file GoalCounter.cpp.
Field Documentation
volatile uint8_t is_goal |
Variable is 1 (true) when the goal is detected.
Definition at line 92 of file GoalCounter.h.
Generated on Sun Jul 17 2022 06:48:43 by
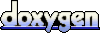