This library provides simple interface for the table football goal counter based on a IR LED or laser diode and phototransistor.
Dependents: goal_counter_project
GoalCounter.h
00001 #ifndef GOALCOUNTER_H 00002 #define GOALCOUNTER_H 00003 00004 #include "mbed.h" 00005 00006 /** GoalCounter class. 00007 * Simple implementation of goal counter for table football using laser diode 00008 * and phototransistor. Output of the phototransistor is connected to the digital 00009 * input pin of MCU. When the laser beam is interrupted by ball passing the the cage 00010 * Timer starts counting and measuring width of the pulse and if the duration of pulse 00011 * is not longer than two seconds, goal is detected and _score variable is incremented. 00012 * 00013 * Pulse duration is also used for the calculation of ball passing speed. However, 00014 * measurement is not precise. 00015 * 00016 * Example: 00017 * @code 00018 * #include "mbed.h" 00019 * #include "GoalCounter.h" 00020 * 00021 * Timer t; 00022 * GoalCounter gc(D2, &t); 00023 * 00024 * int main() { 00025 * while(1) { 00026 * if (gc.is_goal) 00027 * goal(); // call some function 00028 * } 00029 * 00030 * } 00031 * 00032 * @endcode 00033 * 00034 **/ 00035 00036 class GoalCounter { 00037 public: 00038 /** 00039 * Constructor 00040 * 00041 * @param pin Input pin where is the outpout of phototransistor connected 00042 * @param timer reference to the Timer instance 00043 * 00044 */ 00045 GoalCounter(PinName pin, Timer * t); 00046 /** 00047 * Destructor 00048 * 00049 * Destroys instance 00050 */ 00051 ~GoalCounter(); 00052 /** 00053 * Reads value of the timer, 00054 * this function is called from the interrupt pin fall edge callback 00055 */ 00056 void tstart(); 00057 /** 00058 * Reads value from the timer and measures interval between end and beginning 00059 * Called from the interrupt pin rise edge callback 00060 */ 00061 void tstop(); 00062 /** 00063 * Returns score 00064 * @return score score of the game 00065 */ 00066 uint8_t get_score(); 00067 /** 00068 * Returns time of a ball pass for a given score 00069 * @param score of the game 00070 * @return time of a ball pass in seconds 00071 */ 00072 float get_balltime(uint8_t score); 00073 /** 00074 * Returns time of a ball pass for the current score 00075 * @return time of a ball pass in seconds 00076 */ 00077 float get_balltime(); 00078 /** 00079 * Returns speed of a ball pass for a given score 00080 * @param score of the game 00081 * @return speed of a ball pass in kph 00082 */ 00083 float get_ballspeed(uint8_t score); 00084 /** 00085 * Returns speed of a ball pass for the current score 00086 * @return speed of a ball pass in kph 00087 */ 00088 float get_ballspeed(); 00089 /** 00090 * Variable is 1 (true) when the goal is detected 00091 */ 00092 volatile uint8_t is_goal; 00093 00094 private: 00095 InterruptIn * _interrupt; 00096 volatile uint8_t _score; 00097 Timer * _t; 00098 float _balltimes[11]; // sorry for the FP arithmetics, i was too lazy to rewrite it 00099 float _time; 00100 float _begin; 00101 float _end; 00102 float _last_end; 00103 float _time_between; 00104 }; 00105 00106 #endif
Generated on Sun Jul 17 2022 06:48:43 by
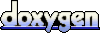