This library provides simple interface for the table football goal counter based on a IR LED or laser diode and phototransistor.
Dependents: goal_counter_project
GoalCounter.cpp
00001 #include "GoalCounter.h" 00002 00003 GoalCounter::GoalCounter(PinName pin, Timer * t) : _t(t) { 00004 00005 InterruptIn *_interrupt = new InterruptIn(pin); // Initialize instace of InterruptIn 00006 // It has to be done dynamically because of non-copyable class 00007 00008 _interrupt->fall(callback(this, &GoalCounter::tstart)); // Set callbacks to timer 00009 _interrupt->rise(callback(this, &GoalCounter::tstop)); 00010 00011 _score = 0; 00012 _time = 0; 00013 is_goal = 0; 00014 _begin = 0; 00015 _end = 0; 00016 _last_end = 0; 00017 _time_between = 0; 00018 00019 _t->start(); 00020 } 00021 00022 GoalCounter::~GoalCounter() 00023 { 00024 delete[] _interrupt; // free up dynamically allocated memory 00025 } 00026 00027 void GoalCounter::tstart() { 00028 _begin = _t->read(); // read value of the timer 00029 } 00030 00031 void GoalCounter::tstop() { 00032 _end = _t->read(); 00033 _time = _end - _begin; 00034 _time_between = _begin - _last_end; 00035 00036 if ( _time > 0 && _time < 2 && _score < 10 && _time_between > 1) { 00037 _score++; 00038 _balltimes[_score] = _time; 00039 _last_end = _end; 00040 is_goal = 1; 00041 } 00042 } 00043 00044 00045 uint8_t GoalCounter::get_score() { 00046 return _score; 00047 } 00048 00049 float GoalCounter::get_balltime(uint8_t score) { 00050 if (score <= _score && score > 0) 00051 return _balltimes[score]; 00052 else 00053 return -1; 00054 } 00055 00056 float GoalCounter::get_balltime() { 00057 return _balltimes[_score]; 00058 00059 } 00060 00061 float GoalCounter::get_ballspeed(uint8_t score) { 00062 if (score <= _score && score > 0) { 00063 float speed = 0.034f/_balltimes[score]*3.6f; 00064 return speed; 00065 } 00066 else 00067 return -1; 00068 } 00069 00070 float GoalCounter::get_ballspeed() { 00071 float speed = 0.034f/_balltimes[_score]*3.6f; 00072 return speed; 00073 }
Generated on Sun Jul 17 2022 06:48:43 by
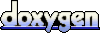