
Simple "hello world" style program for X-NUCLEO-IKS01A1 MEMS Inertial
Dependencies: BLE_API X_NUCLEO_IDB0XA1 X_NUCLEO_IKS01A1 mbed
Fork of HelloWorld_IKS01A1 by
stm32f4xx_usart.c
00001 /** 00002 ****************************************************************************** 00003 * @file stm32f4xx_usart.c 00004 * @author MCD Application Team 00005 * @version V1.0.0 00006 * @date 30-September-2011 00007 * @brief This file provides firmware functions to manage the following 00008 * functionalities of the Universal synchronous asynchronous receiver 00009 * transmitter (USART): 00010 * - Initialization and Configuration 00011 * - Data transfers 00012 * - Multi-Processor Communication 00013 * - LIN mode 00014 * - Half-duplex mode 00015 * - Smartcard mode 00016 * - IrDA mode 00017 * - DMA transfers management 00018 * - Interrupts and flags management 00019 * 00020 * @verbatim 00021 * 00022 * =================================================================== 00023 * How to use this driver 00024 * =================================================================== 00025 * 1. Enable peripheral clock using the follwoing functions 00026 * RCC_APB2PeriphClockCmd(RCC_APB2Periph_USARTx, ENABLE) for USART1 and USART6 00027 * RCC_APB1PeriphClockCmd(RCC_APB1Periph_USARTx, ENABLE) for USART2, USART3, UART4 or UART5. 00028 * 00029 * 2. According to the USART mode, enable the GPIO clocks using 00030 * RCC_AHB1PeriphClockCmd() function. (The I/O can be TX, RX, CTS, 00031 * or/and SCLK). 00032 * 00033 * 3. Peripheral's alternate function: 00034 * - Connect the pin to the desired peripherals' Alternate 00035 * Function (AF) using GPIO_PinAFConfig() function 00036 * - Configure the desired pin in alternate function by: 00037 * GPIO_InitStruct->GPIO_Mode = GPIO_Mode_AF 00038 * - Select the type, pull-up/pull-down and output speed via 00039 * GPIO_PuPd, GPIO_OType and GPIO_Speed members 00040 * - Call GPIO_Init() function 00041 * 00042 * 4. Program the Baud Rate, Word Length , Stop Bit, Parity, Hardware 00043 * flow control and Mode(Receiver/Transmitter) using the USART_Init() 00044 * function. 00045 * 00046 * 5. For synchronous mode, enable the clock and program the polarity, 00047 * phase and last bit using the USART_ClockInit() function. 00048 * 00049 * 5. Enable the NVIC and the corresponding interrupt using the function 00050 * USART_ITConfig() if you need to use interrupt mode. 00051 * 00052 * 6. When using the DMA mode 00053 * - Configure the DMA using DMA_Init() function 00054 * - Active the needed channel Request using USART_DMACmd() function 00055 * 00056 * 7. Enable the USART using the USART_Cmd() function. 00057 * 00058 * 8. Enable the DMA using the DMA_Cmd() function, when using DMA mode. 00059 * 00060 * Refer to Multi-Processor, LIN, half-duplex, Smartcard, IrDA sub-sections 00061 * for more details 00062 * 00063 * In order to reach higher communication baudrates, it is possible to 00064 * enable the oversampling by 8 mode using the function USART_OverSampling8Cmd(). 00065 * This function should be called after enabling the USART clock (RCC_APBxPeriphClockCmd()) 00066 * and before calling the function USART_Init(). 00067 * 00068 * @endverbatim 00069 * 00070 ****************************************************************************** 00071 * @attention 00072 * 00073 * THE PRESENT FIRMWARE WHICH IS FOR GUIDANCE ONLY AIMS AT PROVIDING CUSTOMERS 00074 * WITH CODING INFORMATION REGARDING THEIR PRODUCTS IN ORDER FOR THEM TO SAVE 00075 * TIME. AS A RESULT, STMICROELECTRONICS SHALL NOT BE HELD LIABLE FOR ANY 00076 * DIRECT, INDIRECT OR CONSEQUENTIAL DAMAGES WITH RESPECT TO ANY CLAIMS ARISING 00077 * FROM THE CONTENT OF SUCH FIRMWARE AND/OR THE USE MADE BY CUSTOMERS OF THE 00078 * CODING INFORMATION CONTAINED HEREIN IN CONNECTION WITH THEIR PRODUCTS. 00079 * 00080 * <h2><center>© COPYRIGHT 2011 STMicroelectronics</center></h2> 00081 ****************************************************************************** 00082 */ 00083 00084 /* Includes ------------------------------------------------------------------*/ 00085 #include "stm32f4xx_usart.h" 00086 #include "stm32f4xx_rcc.h" 00087 00088 /** @addtogroup STM32F4xx_StdPeriph_Driver 00089 * @{ 00090 */ 00091 00092 /** @defgroup USART 00093 * @brief USART driver modules 00094 * @{ 00095 */ 00096 00097 /* Private typedef -----------------------------------------------------------*/ 00098 /* Private define ------------------------------------------------------------*/ 00099 00100 /*!< USART CR1 register clear Mask ((~(uint16_t)0xE9F3)) */ 00101 #define CR1_CLEAR_MASK ((uint16_t)(USART_CR1_M | USART_CR1_PCE | \ 00102 USART_CR1_PS | USART_CR1_TE | \ 00103 USART_CR1_RE)) 00104 00105 /*!< USART CR2 register clock bits clear Mask ((~(uint16_t)0xF0FF)) */ 00106 #define CR2_CLOCK_CLEAR_MASK ((uint16_t)(USART_CR2_CLKEN | USART_CR2_CPOL | \ 00107 USART_CR2_CPHA | USART_CR2_LBCL)) 00108 00109 /*!< USART CR3 register clear Mask ((~(uint16_t)0xFCFF)) */ 00110 #define CR3_CLEAR_MASK ((uint16_t)(USART_CR3_RTSE | USART_CR3_CTSE)) 00111 00112 /*!< USART Interrupts mask */ 00113 #define IT_MASK ((uint16_t)0x001F) 00114 00115 /* Private macro -------------------------------------------------------------*/ 00116 /* Private variables ---------------------------------------------------------*/ 00117 /* Private function prototypes -----------------------------------------------*/ 00118 /* Private functions ---------------------------------------------------------*/ 00119 00120 /** @defgroup USART_Private_Functions 00121 * @{ 00122 */ 00123 00124 /** @defgroup USART_Group1 Initialization and Configuration functions 00125 * @brief Initialization and Configuration functions 00126 * 00127 @verbatim 00128 =============================================================================== 00129 Initialization and Configuration functions 00130 =============================================================================== 00131 00132 This subsection provides a set of functions allowing to initialize the USART 00133 in asynchronous and in synchronous modes. 00134 - For the asynchronous mode only these parameters can be configured: 00135 - Baud Rate 00136 - Word Length 00137 - Stop Bit 00138 - Parity: If the parity is enabled, then the MSB bit of the data written 00139 in the data register is transmitted but is changed by the parity bit. 00140 Depending on the frame length defined by the M bit (8-bits or 9-bits), 00141 the possible USART frame formats are as listed in the following table: 00142 +-------------------------------------------------------------+ 00143 | M bit | PCE bit | USART frame | 00144 |---------------------|---------------------------------------| 00145 | 0 | 0 | | SB | 8 bit data | STB | | 00146 |---------|-----------|---------------------------------------| 00147 | 0 | 1 | | SB | 7 bit data | PB | STB | | 00148 |---------|-----------|---------------------------------------| 00149 | 1 | 0 | | SB | 9 bit data | STB | | 00150 |---------|-----------|---------------------------------------| 00151 | 1 | 1 | | SB | 8 bit data | PB | STB | | 00152 +-------------------------------------------------------------+ 00153 - Hardware flow control 00154 - Receiver/transmitter modes 00155 00156 The USART_Init() function follows the USART asynchronous configuration procedure 00157 (details for the procedure are available in reference manual (RM0090)). 00158 00159 - For the synchronous mode in addition to the asynchronous mode parameters these 00160 parameters should be also configured: 00161 - USART Clock Enabled 00162 - USART polarity 00163 - USART phase 00164 - USART LastBit 00165 00166 These parameters can be configured using the USART_ClockInit() function. 00167 00168 @endverbatim 00169 * @{ 00170 */ 00171 00172 /** 00173 * @brief Deinitializes the USARTx peripheral registers to their default reset values. 00174 * @param USARTx: where x can be 1, 2, 3, 4, 5 or 6 to select the USART or 00175 * UART peripheral. 00176 * @retval None 00177 */ 00178 void USART_DeInit(USART_TypeDef* USARTx) 00179 { 00180 /* Check the parameters */ 00181 assert_param(IS_USART_ALL_PERIPH(USARTx)); 00182 00183 if (USARTx == USART1) 00184 { 00185 RCC_APB2PeriphResetCmd(RCC_APB2Periph_USART1, ENABLE); 00186 RCC_APB2PeriphResetCmd(RCC_APB2Periph_USART1, DISABLE); 00187 } 00188 else if (USARTx == USART2) 00189 { 00190 RCC_APB1PeriphResetCmd(RCC_APB1Periph_USART2, ENABLE); 00191 RCC_APB1PeriphResetCmd(RCC_APB1Periph_USART2, DISABLE); 00192 } 00193 else if (USARTx == USART3) 00194 { 00195 RCC_APB1PeriphResetCmd(RCC_APB1Periph_USART3, ENABLE); 00196 RCC_APB1PeriphResetCmd(RCC_APB1Periph_USART3, DISABLE); 00197 } 00198 else if (USARTx == UART4) 00199 { 00200 RCC_APB1PeriphResetCmd(RCC_APB1Periph_UART4, ENABLE); 00201 RCC_APB1PeriphResetCmd(RCC_APB1Periph_UART4, DISABLE); 00202 } 00203 else if (USARTx == UART5) 00204 { 00205 RCC_APB1PeriphResetCmd(RCC_APB1Periph_UART5, ENABLE); 00206 RCC_APB1PeriphResetCmd(RCC_APB1Periph_UART5, DISABLE); 00207 } 00208 else 00209 { 00210 if (USARTx == USART6) 00211 { 00212 RCC_APB2PeriphResetCmd(RCC_APB2Periph_USART6, ENABLE); 00213 RCC_APB2PeriphResetCmd(RCC_APB2Periph_USART6, DISABLE); 00214 } 00215 } 00216 } 00217 00218 /** 00219 * @brief Initializes the USARTx peripheral according to the specified 00220 * parameters in the USART_InitStruct . 00221 * @param USARTx: where x can be 1, 2, 3, 4, 5 or 6 to select the USART or 00222 * UART peripheral. 00223 * @param USART_InitStruct: pointer to a USART_InitTypeDef structure that contains 00224 * the configuration information for the specified USART peripheral. 00225 * @retval None 00226 */ 00227 void USART_Init(USART_TypeDef* USARTx, USART_InitTypeDef* USART_InitStruct) 00228 { 00229 uint32_t tmpreg = 0x00, apbclock = 0x00; 00230 uint32_t integerdivider = 0x00; 00231 uint32_t fractionaldivider = 0x00; 00232 RCC_ClocksTypeDef RCC_ClocksStatus; 00233 00234 /* Check the parameters */ 00235 assert_param(IS_USART_ALL_PERIPH(USARTx)); 00236 assert_param(IS_USART_BAUDRATE(USART_InitStruct->USART_BaudRate)); 00237 assert_param(IS_USART_WORD_LENGTH(USART_InitStruct->USART_WordLength)); 00238 assert_param(IS_USART_STOPBITS(USART_InitStruct->USART_StopBits)); 00239 assert_param(IS_USART_PARITY(USART_InitStruct->USART_Parity)); 00240 assert_param(IS_USART_MODE(USART_InitStruct->USART_Mode)); 00241 assert_param(IS_USART_HARDWARE_FLOW_CONTROL(USART_InitStruct->USART_HardwareFlowControl)); 00242 00243 /* The hardware flow control is available only for USART1, USART2, USART3 and USART6 */ 00244 if (USART_InitStruct->USART_HardwareFlowControl != USART_HardwareFlowControl_None) 00245 { 00246 assert_param(IS_USART_1236_PERIPH(USARTx)); 00247 } 00248 00249 /*---------------------------- USART CR2 Configuration -----------------------*/ 00250 tmpreg = USARTx->CR2; 00251 00252 /* Clear STOP[13:12] bits */ 00253 tmpreg &= (uint32_t)~((uint32_t)USART_CR2_STOP); 00254 00255 /* Configure the USART Stop Bits, Clock, CPOL, CPHA and LastBit : 00256 Set STOP[13:12] bits according to USART_StopBits value */ 00257 tmpreg |= (uint32_t)USART_InitStruct->USART_StopBits; 00258 00259 /* Write to USART CR2 */ 00260 USARTx->CR2 = (uint16_t)tmpreg; 00261 00262 /*---------------------------- USART CR1 Configuration -----------------------*/ 00263 tmpreg = USARTx->CR1; 00264 00265 /* Clear M, PCE, PS, TE and RE bits */ 00266 tmpreg &= (uint32_t)~((uint32_t)CR1_CLEAR_MASK); 00267 00268 /* Configure the USART Word Length, Parity and mode: 00269 Set the M bits according to USART_WordLength value 00270 Set PCE and PS bits according to USART_Parity value 00271 Set TE and RE bits according to USART_Mode value */ 00272 tmpreg |= (uint32_t)USART_InitStruct->USART_WordLength | USART_InitStruct->USART_Parity | 00273 USART_InitStruct->USART_Mode; 00274 00275 /* Write to USART CR1 */ 00276 USARTx->CR1 = (uint16_t)tmpreg; 00277 00278 /*---------------------------- USART CR3 Configuration -----------------------*/ 00279 tmpreg = USARTx->CR3; 00280 00281 /* Clear CTSE and RTSE bits */ 00282 tmpreg &= (uint32_t)~((uint32_t)CR3_CLEAR_MASK); 00283 00284 /* Configure the USART HFC : 00285 Set CTSE and RTSE bits according to USART_HardwareFlowControl value */ 00286 tmpreg |= USART_InitStruct->USART_HardwareFlowControl; 00287 00288 /* Write to USART CR3 */ 00289 USARTx->CR3 = (uint16_t)tmpreg; 00290 00291 /*---------------------------- USART BRR Configuration -----------------------*/ 00292 /* Configure the USART Baud Rate */ 00293 RCC_GetClocksFreq(&RCC_ClocksStatus); 00294 00295 if ((USARTx == USART1) || (USARTx == USART6)) 00296 { 00297 apbclock = RCC_ClocksStatus.PCLK2_Frequency; 00298 } 00299 else 00300 { 00301 apbclock = RCC_ClocksStatus.PCLK1_Frequency; 00302 } 00303 00304 /* Determine the integer part */ 00305 if ((USARTx->CR1 & USART_CR1_OVER8) != 0) 00306 { 00307 /* Integer part computing in case Oversampling mode is 8 Samples */ 00308 integerdivider = ((25 * apbclock) / (2 * (USART_InitStruct->USART_BaudRate))); 00309 } 00310 else /* if ((USARTx->CR1 & USART_CR1_OVER8) == 0) */ 00311 { 00312 /* Integer part computing in case Oversampling mode is 16 Samples */ 00313 integerdivider = ((25 * apbclock) / (4 * (USART_InitStruct->USART_BaudRate))); 00314 } 00315 tmpreg = (integerdivider / 100) << 4; 00316 00317 /* Determine the fractional part */ 00318 fractionaldivider = integerdivider - (100 * (tmpreg >> 4)); 00319 00320 /* Implement the fractional part in the register */ 00321 if ((USARTx->CR1 & USART_CR1_OVER8) != 0) 00322 { 00323 tmpreg |= ((((fractionaldivider * 8) + 50) / 100)) & ((uint8_t)0x07); 00324 } 00325 else /* if ((USARTx->CR1 & USART_CR1_OVER8) == 0) */ 00326 { 00327 tmpreg |= ((((fractionaldivider * 16) + 50) / 100)) & ((uint8_t)0x0F); 00328 } 00329 00330 /* Write to USART BRR register */ 00331 USARTx->BRR = (uint16_t)tmpreg; 00332 } 00333 00334 /** 00335 * @brief Fills each USART_InitStruct member with its default value. 00336 * @param USART_InitStruct: pointer to a USART_InitTypeDef structure which will 00337 * be initialized. 00338 * @retval None 00339 */ 00340 void USART_StructInit(USART_InitTypeDef* USART_InitStruct) 00341 { 00342 /* USART_InitStruct members default value */ 00343 USART_InitStruct->USART_BaudRate = 9600; 00344 USART_InitStruct->USART_WordLength = USART_WordLength_8b; 00345 USART_InitStruct->USART_StopBits = USART_StopBits_1; 00346 USART_InitStruct->USART_Parity = USART_Parity_No ; 00347 USART_InitStruct->USART_Mode = USART_Mode_Rx | USART_Mode_Tx; 00348 USART_InitStruct->USART_HardwareFlowControl = USART_HardwareFlowControl_None; 00349 } 00350 00351 /** 00352 * @brief Initializes the USARTx peripheral Clock according to the 00353 * specified parameters in the USART_ClockInitStruct . 00354 * @param USARTx: where x can be 1, 2, 3 or 6 to select the USART peripheral. 00355 * @param USART_ClockInitStruct: pointer to a USART_ClockInitTypeDef structure that 00356 * contains the configuration information for the specified USART peripheral. 00357 * @note The Smart Card and Synchronous modes are not available for UART4 and UART5. 00358 * @retval None 00359 */ 00360 void USART_ClockInit(USART_TypeDef* USARTx, USART_ClockInitTypeDef* USART_ClockInitStruct) 00361 { 00362 uint32_t tmpreg = 0x00; 00363 /* Check the parameters */ 00364 assert_param(IS_USART_1236_PERIPH(USARTx)); 00365 assert_param(IS_USART_CLOCK(USART_ClockInitStruct->USART_Clock)); 00366 assert_param(IS_USART_CPOL(USART_ClockInitStruct->USART_CPOL)); 00367 assert_param(IS_USART_CPHA(USART_ClockInitStruct->USART_CPHA)); 00368 assert_param(IS_USART_LASTBIT(USART_ClockInitStruct->USART_LastBit)); 00369 00370 /*---------------------------- USART CR2 Configuration -----------------------*/ 00371 tmpreg = USARTx->CR2; 00372 /* Clear CLKEN, CPOL, CPHA and LBCL bits */ 00373 tmpreg &= (uint32_t)~((uint32_t)CR2_CLOCK_CLEAR_MASK); 00374 /* Configure the USART Clock, CPOL, CPHA and LastBit ------------*/ 00375 /* Set CLKEN bit according to USART_Clock value */ 00376 /* Set CPOL bit according to USART_CPOL value */ 00377 /* Set CPHA bit according to USART_CPHA value */ 00378 /* Set LBCL bit according to USART_LastBit value */ 00379 tmpreg |= (uint32_t)USART_ClockInitStruct->USART_Clock | USART_ClockInitStruct->USART_CPOL | 00380 USART_ClockInitStruct->USART_CPHA | USART_ClockInitStruct->USART_LastBit; 00381 /* Write to USART CR2 */ 00382 USARTx->CR2 = (uint16_t)tmpreg; 00383 } 00384 00385 /** 00386 * @brief Fills each USART_ClockInitStruct member with its default value. 00387 * @param USART_ClockInitStruct: pointer to a USART_ClockInitTypeDef structure 00388 * which will be initialized. 00389 * @retval None 00390 */ 00391 void USART_ClockStructInit(USART_ClockInitTypeDef* USART_ClockInitStruct) 00392 { 00393 /* USART_ClockInitStruct members default value */ 00394 USART_ClockInitStruct->USART_Clock = USART_Clock_Disable; 00395 USART_ClockInitStruct->USART_CPOL = USART_CPOL_Low; 00396 USART_ClockInitStruct->USART_CPHA = USART_CPHA_1Edge; 00397 USART_ClockInitStruct->USART_LastBit = USART_LastBit_Disable; 00398 } 00399 00400 /** 00401 * @brief Enables or disables the specified USART peripheral. 00402 * @param USARTx: where x can be 1, 2, 3, 4, 5 or 6 to select the USART or 00403 * UART peripheral. 00404 * @param NewState: new state of the USARTx peripheral. 00405 * This parameter can be: ENABLE or DISABLE. 00406 * @retval None 00407 */ 00408 void USART_Cmd(USART_TypeDef* USARTx, FunctionalState NewState) 00409 { 00410 /* Check the parameters */ 00411 assert_param(IS_USART_ALL_PERIPH(USARTx)); 00412 assert_param(IS_FUNCTIONAL_STATE(NewState)); 00413 00414 if (NewState != DISABLE) 00415 { 00416 /* Enable the selected USART by setting the UE bit in the CR1 register */ 00417 USARTx->CR1 |= USART_CR1_UE; 00418 } 00419 else 00420 { 00421 /* Disable the selected USART by clearing the UE bit in the CR1 register */ 00422 USARTx->CR1 &= (uint16_t)~((uint16_t)USART_CR1_UE); 00423 } 00424 } 00425 00426 /** 00427 * @brief Sets the system clock prescaler. 00428 * @param USARTx: where x can be 1, 2, 3, 4, 5 or 6 to select the USART or 00429 * UART peripheral. 00430 * @param USART_Prescaler: specifies the prescaler clock. 00431 * @note The function is used for IrDA mode with UART4 and UART5. 00432 * @retval None 00433 */ 00434 void USART_SetPrescaler(USART_TypeDef* USARTx, uint8_t USART_Prescaler) 00435 { 00436 /* Check the parameters */ 00437 assert_param(IS_USART_ALL_PERIPH(USARTx)); 00438 00439 /* Clear the USART prescaler */ 00440 USARTx->GTPR &= USART_GTPR_GT; 00441 /* Set the USART prescaler */ 00442 USARTx->GTPR |= USART_Prescaler; 00443 } 00444 00445 /** 00446 * @brief Enables or disables the USART's 8x oversampling mode. 00447 * @note This function has to be called before calling USART_Init() function 00448 * in order to have correct baudrate Divider value. 00449 * @param USARTx: where x can be 1, 2, 3, 4, 5 or 6 to select the USART or 00450 * UART peripheral. 00451 * @param NewState: new state of the USART 8x oversampling mode. 00452 * This parameter can be: ENABLE or DISABLE. 00453 * @retval None 00454 */ 00455 void USART_OverSampling8Cmd(USART_TypeDef* USARTx, FunctionalState NewState) 00456 { 00457 /* Check the parameters */ 00458 assert_param(IS_USART_ALL_PERIPH(USARTx)); 00459 assert_param(IS_FUNCTIONAL_STATE(NewState)); 00460 00461 if (NewState != DISABLE) 00462 { 00463 /* Enable the 8x Oversampling mode by setting the OVER8 bit in the CR1 register */ 00464 USARTx->CR1 |= USART_CR1_OVER8; 00465 } 00466 else 00467 { 00468 /* Disable the 8x Oversampling mode by clearing the OVER8 bit in the CR1 register */ 00469 USARTx->CR1 &= (uint16_t)~((uint16_t)USART_CR1_OVER8); 00470 } 00471 } 00472 00473 /** 00474 * @brief Enables or disables the USART's one bit sampling method. 00475 * @param USARTx: where x can be 1, 2, 3, 4, 5 or 6 to select the USART or 00476 * UART peripheral. 00477 * @param NewState: new state of the USART one bit sampling method. 00478 * This parameter can be: ENABLE or DISABLE. 00479 * @retval None 00480 */ 00481 void USART_OneBitMethodCmd(USART_TypeDef* USARTx, FunctionalState NewState) 00482 { 00483 /* Check the parameters */ 00484 assert_param(IS_USART_ALL_PERIPH(USARTx)); 00485 assert_param(IS_FUNCTIONAL_STATE(NewState)); 00486 00487 if (NewState != DISABLE) 00488 { 00489 /* Enable the one bit method by setting the ONEBITE bit in the CR3 register */ 00490 USARTx->CR3 |= USART_CR3_ONEBIT; 00491 } 00492 else 00493 { 00494 /* Disable the one bit method by clearing the ONEBITE bit in the CR3 register */ 00495 USARTx->CR3 &= (uint16_t)~((uint16_t)USART_CR3_ONEBIT); 00496 } 00497 } 00498 00499 /** 00500 * @} 00501 */ 00502 00503 /** @defgroup USART_Group2 Data transfers functions 00504 * @brief Data transfers functions 00505 * 00506 @verbatim 00507 =============================================================================== 00508 Data transfers functions 00509 =============================================================================== 00510 00511 This subsection provides a set of functions allowing to manage the USART data 00512 transfers. 00513 00514 During an USART reception, data shifts in least significant bit first through 00515 the RX pin. In this mode, the USART_DR register consists of a buffer (RDR) 00516 between the internal bus and the received shift register. 00517 00518 When a transmission is taking place, a write instruction to the USART_DR register 00519 stores the data in the TDR register and which is copied in the shift register 00520 at the end of the current transmission. 00521 00522 The read access of the USART_DR register can be done using the USART_ReceiveData() 00523 function and returns the RDR buffered value. Whereas a write access to the USART_DR 00524 can be done using USART_SendData() function and stores the written data into 00525 TDR buffer. 00526 00527 @endverbatim 00528 * @{ 00529 */ 00530 00531 /** 00532 * @brief Transmits single data through the USARTx peripheral. 00533 * @param USARTx: where x can be 1, 2, 3, 4, 5 or 6 to select the USART or 00534 * UART peripheral. 00535 * @param Data: the data to transmit. 00536 * @retval None 00537 */ 00538 void USART_SendData(USART_TypeDef* USARTx, uint16_t Data) 00539 { 00540 /* Check the parameters */ 00541 assert_param(IS_USART_ALL_PERIPH(USARTx)); 00542 assert_param(IS_USART_DATA(Data)); 00543 00544 /* Transmit Data */ 00545 USARTx->DR = (Data & (uint16_t)0x01FF); 00546 } 00547 00548 /** 00549 * @brief Returns the most recent received data by the USARTx peripheral. 00550 * @param USARTx: where x can be 1, 2, 3, 4, 5 or 6 to select the USART or 00551 * UART peripheral. 00552 * @retval The received data. 00553 */ 00554 uint16_t USART_ReceiveData(USART_TypeDef* USARTx) 00555 { 00556 /* Check the parameters */ 00557 assert_param(IS_USART_ALL_PERIPH(USARTx)); 00558 00559 /* Receive Data */ 00560 return (uint16_t)(USARTx->DR & (uint16_t)0x01FF); 00561 } 00562 00563 /** 00564 * @} 00565 */ 00566 00567 /** @defgroup USART_Group3 MultiProcessor Communication functions 00568 * @brief Multi-Processor Communication functions 00569 * 00570 @verbatim 00571 =============================================================================== 00572 Multi-Processor Communication functions 00573 =============================================================================== 00574 00575 This subsection provides a set of functions allowing to manage the USART 00576 multiprocessor communication. 00577 00578 For instance one of the USARTs can be the master, its TX output is connected to 00579 the RX input of the other USART. The others are slaves, their respective TX outputs 00580 are logically ANDed together and connected to the RX input of the master. 00581 00582 USART multiprocessor communication is possible through the following procedure: 00583 1. Program the Baud rate, Word length = 9 bits, Stop bits, Parity, Mode transmitter 00584 or Mode receiver and hardware flow control values using the USART_Init() 00585 function. 00586 2. Configures the USART address using the USART_SetAddress() function. 00587 3. Configures the wake up method (USART_WakeUp_IdleLine or USART_WakeUp_AddressMark) 00588 using USART_WakeUpConfig() function only for the slaves. 00589 4. Enable the USART using the USART_Cmd() function. 00590 5. Enter the USART slaves in mute mode using USART_ReceiverWakeUpCmd() function. 00591 00592 The USART Slave exit from mute mode when receive the wake up condition. 00593 00594 @endverbatim 00595 * @{ 00596 */ 00597 00598 /** 00599 * @brief Sets the address of the USART node. 00600 * @param USARTx: where x can be 1, 2, 3, 4, 5 or 6 to select the USART or 00601 * UART peripheral. 00602 * @param USART_Address: Indicates the address of the USART node. 00603 * @retval None 00604 */ 00605 void USART_SetAddress(USART_TypeDef* USARTx, uint8_t USART_Address) 00606 { 00607 /* Check the parameters */ 00608 assert_param(IS_USART_ALL_PERIPH(USARTx)); 00609 assert_param(IS_USART_ADDRESS(USART_Address)); 00610 00611 /* Clear the USART address */ 00612 USARTx->CR2 &= (uint16_t)~((uint16_t)USART_CR2_ADD); 00613 /* Set the USART address node */ 00614 USARTx->CR2 |= USART_Address; 00615 } 00616 00617 /** 00618 * @brief Determines if the USART is in mute mode or not. 00619 * @param USARTx: where x can be 1, 2, 3, 4, 5 or 6 to select the USART or 00620 * UART peripheral. 00621 * @param NewState: new state of the USART mute mode. 00622 * This parameter can be: ENABLE or DISABLE. 00623 * @retval None 00624 */ 00625 void USART_ReceiverWakeUpCmd(USART_TypeDef* USARTx, FunctionalState NewState) 00626 { 00627 /* Check the parameters */ 00628 assert_param(IS_USART_ALL_PERIPH(USARTx)); 00629 assert_param(IS_FUNCTIONAL_STATE(NewState)); 00630 00631 if (NewState != DISABLE) 00632 { 00633 /* Enable the USART mute mode by setting the RWU bit in the CR1 register */ 00634 USARTx->CR1 |= USART_CR1_RWU; 00635 } 00636 else 00637 { 00638 /* Disable the USART mute mode by clearing the RWU bit in the CR1 register */ 00639 USARTx->CR1 &= (uint16_t)~((uint16_t)USART_CR1_RWU); 00640 } 00641 } 00642 /** 00643 * @brief Selects the USART WakeUp method. 00644 * @param USARTx: where x can be 1, 2, 3, 4, 5 or 6 to select the USART or 00645 * UART peripheral. 00646 * @param USART_WakeUp: specifies the USART wakeup method. 00647 * This parameter can be one of the following values: 00648 * @arg USART_WakeUp_IdleLine: WakeUp by an idle line detection 00649 * @arg USART_WakeUp_AddressMark: WakeUp by an address mark 00650 * @retval None 00651 */ 00652 void USART_WakeUpConfig(USART_TypeDef* USARTx, uint16_t USART_WakeUp) 00653 { 00654 /* Check the parameters */ 00655 assert_param(IS_USART_ALL_PERIPH(USARTx)); 00656 assert_param(IS_USART_WAKEUP(USART_WakeUp)); 00657 00658 USARTx->CR1 &= (uint16_t)~((uint16_t)USART_CR1_WAKE); 00659 USARTx->CR1 |= USART_WakeUp; 00660 } 00661 00662 /** 00663 * @} 00664 */ 00665 00666 /** @defgroup USART_Group4 LIN mode functions 00667 * @brief LIN mode functions 00668 * 00669 @verbatim 00670 =============================================================================== 00671 LIN mode functions 00672 =============================================================================== 00673 00674 This subsection provides a set of functions allowing to manage the USART LIN 00675 Mode communication. 00676 00677 In LIN mode, 8-bit data format with 1 stop bit is required in accordance with 00678 the LIN standard. 00679 00680 Only this LIN Feature is supported by the USART IP: 00681 - LIN Master Synchronous Break send capability and LIN slave break detection 00682 capability : 13-bit break generation and 10/11 bit break detection 00683 00684 00685 USART LIN Master transmitter communication is possible through the following procedure: 00686 1. Program the Baud rate, Word length = 8bits, Stop bits = 1bit, Parity, 00687 Mode transmitter or Mode receiver and hardware flow control values using 00688 the USART_Init() function. 00689 2. Enable the USART using the USART_Cmd() function. 00690 3. Enable the LIN mode using the USART_LINCmd() function. 00691 4. Send the break character using USART_SendBreak() function. 00692 00693 USART LIN Master receiver communication is possible through the following procedure: 00694 1. Program the Baud rate, Word length = 8bits, Stop bits = 1bit, Parity, 00695 Mode transmitter or Mode receiver and hardware flow control values using 00696 the USART_Init() function. 00697 2. Enable the USART using the USART_Cmd() function. 00698 3. Configures the break detection length using the USART_LINBreakDetectLengthConfig() 00699 function. 00700 4. Enable the LIN mode using the USART_LINCmd() function. 00701 00702 00703 @note In LIN mode, the following bits must be kept cleared: 00704 - CLKEN in the USART_CR2 register, 00705 - STOP[1:0], SCEN, HDSEL and IREN in the USART_CR3 register. 00706 00707 @endverbatim 00708 * @{ 00709 */ 00710 00711 /** 00712 * @brief Sets the USART LIN Break detection length. 00713 * @param USARTx: where x can be 1, 2, 3, 4, 5 or 6 to select the USART or 00714 * UART peripheral. 00715 * @param USART_LINBreakDetectLength: specifies the LIN break detection length. 00716 * This parameter can be one of the following values: 00717 * @arg USART_LINBreakDetectLength_10b: 10-bit break detection 00718 * @arg USART_LINBreakDetectLength_11b: 11-bit break detection 00719 * @retval None 00720 */ 00721 void USART_LINBreakDetectLengthConfig(USART_TypeDef* USARTx, uint16_t USART_LINBreakDetectLength) 00722 { 00723 /* Check the parameters */ 00724 assert_param(IS_USART_ALL_PERIPH(USARTx)); 00725 assert_param(IS_USART_LIN_BREAK_DETECT_LENGTH(USART_LINBreakDetectLength)); 00726 00727 USARTx->CR2 &= (uint16_t)~((uint16_t)USART_CR2_LBDL); 00728 USARTx->CR2 |= USART_LINBreakDetectLength; 00729 } 00730 00731 /** 00732 * @brief Enables or disables the USART's LIN mode. 00733 * @param USARTx: where x can be 1, 2, 3, 4, 5 or 6 to select the USART or 00734 * UART peripheral. 00735 * @param NewState: new state of the USART LIN mode. 00736 * This parameter can be: ENABLE or DISABLE. 00737 * @retval None 00738 */ 00739 void USART_LINCmd(USART_TypeDef* USARTx, FunctionalState NewState) 00740 { 00741 /* Check the parameters */ 00742 assert_param(IS_USART_ALL_PERIPH(USARTx)); 00743 assert_param(IS_FUNCTIONAL_STATE(NewState)); 00744 00745 if (NewState != DISABLE) 00746 { 00747 /* Enable the LIN mode by setting the LINEN bit in the CR2 register */ 00748 USARTx->CR2 |= USART_CR2_LINEN; 00749 } 00750 else 00751 { 00752 /* Disable the LIN mode by clearing the LINEN bit in the CR2 register */ 00753 USARTx->CR2 &= (uint16_t)~((uint16_t)USART_CR2_LINEN); 00754 } 00755 } 00756 00757 /** 00758 * @brief Transmits break characters. 00759 * @param USARTx: where x can be 1, 2, 3, 4, 5 or 6 to select the USART or 00760 * UART peripheral. 00761 * @retval None 00762 */ 00763 void USART_SendBreak(USART_TypeDef* USARTx) 00764 { 00765 /* Check the parameters */ 00766 assert_param(IS_USART_ALL_PERIPH(USARTx)); 00767 00768 /* Send break characters */ 00769 USARTx->CR1 |= USART_CR1_SBK; 00770 } 00771 00772 /** 00773 * @} 00774 */ 00775 00776 /** @defgroup USART_Group5 Halfduplex mode function 00777 * @brief Half-duplex mode function 00778 * 00779 @verbatim 00780 =============================================================================== 00781 Half-duplex mode function 00782 =============================================================================== 00783 00784 This subsection provides a set of functions allowing to manage the USART 00785 Half-duplex communication. 00786 00787 The USART can be configured to follow a single-wire half-duplex protocol where 00788 the TX and RX lines are internally connected. 00789 00790 USART Half duplex communication is possible through the following procedure: 00791 1. Program the Baud rate, Word length, Stop bits, Parity, Mode transmitter 00792 or Mode receiver and hardware flow control values using the USART_Init() 00793 function. 00794 2. Configures the USART address using the USART_SetAddress() function. 00795 3. Enable the USART using the USART_Cmd() function. 00796 4. Enable the half duplex mode using USART_HalfDuplexCmd() function. 00797 00798 00799 @note The RX pin is no longer used 00800 @note In Half-duplex mode the following bits must be kept cleared: 00801 - LINEN and CLKEN bits in the USART_CR2 register. 00802 - SCEN and IREN bits in the USART_CR3 register. 00803 00804 @endverbatim 00805 * @{ 00806 */ 00807 00808 /** 00809 * @brief Enables or disables the USART's Half Duplex communication. 00810 * @param USARTx: where x can be 1, 2, 3, 4, 5 or 6 to select the USART or 00811 * UART peripheral. 00812 * @param NewState: new state of the USART Communication. 00813 * This parameter can be: ENABLE or DISABLE. 00814 * @retval None 00815 */ 00816 void USART_HalfDuplexCmd(USART_TypeDef* USARTx, FunctionalState NewState) 00817 { 00818 /* Check the parameters */ 00819 assert_param(IS_USART_ALL_PERIPH(USARTx)); 00820 assert_param(IS_FUNCTIONAL_STATE(NewState)); 00821 00822 if (NewState != DISABLE) 00823 { 00824 /* Enable the Half-Duplex mode by setting the HDSEL bit in the CR3 register */ 00825 USARTx->CR3 |= USART_CR3_HDSEL; 00826 } 00827 else 00828 { 00829 /* Disable the Half-Duplex mode by clearing the HDSEL bit in the CR3 register */ 00830 USARTx->CR3 &= (uint16_t)~((uint16_t)USART_CR3_HDSEL); 00831 } 00832 } 00833 00834 /** 00835 * @} 00836 */ 00837 00838 00839 /** @defgroup USART_Group6 Smartcard mode functions 00840 * @brief Smartcard mode functions 00841 * 00842 @verbatim 00843 =============================================================================== 00844 Smartcard mode functions 00845 =============================================================================== 00846 00847 This subsection provides a set of functions allowing to manage the USART 00848 Smartcard communication. 00849 00850 The Smartcard interface is designed to support asynchronous protocol Smartcards as 00851 defined in the ISO 7816-3 standard. 00852 00853 The USART can provide a clock to the smartcard through the SCLK output. 00854 In smartcard mode, SCLK is not associated to the communication but is simply derived 00855 from the internal peripheral input clock through a 5-bit prescaler. 00856 00857 Smartcard communication is possible through the following procedure: 00858 1. Configures the Smartcard Prescaler using the USART_SetPrescaler() function. 00859 2. Configures the Smartcard Guard Time using the USART_SetGuardTime() function. 00860 3. Program the USART clock using the USART_ClockInit() function as following: 00861 - USART Clock enabled 00862 - USART CPOL Low 00863 - USART CPHA on first edge 00864 - USART Last Bit Clock Enabled 00865 4. Program the Smartcard interface using the USART_Init() function as following: 00866 - Word Length = 9 Bits 00867 - 1.5 Stop Bit 00868 - Even parity 00869 - BaudRate = 12096 baud 00870 - Hardware flow control disabled (RTS and CTS signals) 00871 - Tx and Rx enabled 00872 5. Optionally you can enable the parity error interrupt using the USART_ITConfig() 00873 function 00874 6. Enable the USART using the USART_Cmd() function. 00875 7. Enable the Smartcard NACK using the USART_SmartCardNACKCmd() function. 00876 8. Enable the Smartcard interface using the USART_SmartCardCmd() function. 00877 00878 Please refer to the ISO 7816-3 specification for more details. 00879 00880 00881 @note It is also possible to choose 0.5 stop bit for receiving but it is recommended 00882 to use 1.5 stop bits for both transmitting and receiving to avoid switching 00883 between the two configurations. 00884 @note In smartcard mode, the following bits must be kept cleared: 00885 - LINEN bit in the USART_CR2 register. 00886 - HDSEL and IREN bits in the USART_CR3 register. 00887 @note Smartcard mode is available on USART peripherals only (not available on UART4 00888 and UART5 peripherals). 00889 00890 @endverbatim 00891 * @{ 00892 */ 00893 00894 /** 00895 * @brief Sets the specified USART guard time. 00896 * @param USARTx: where x can be 1, 2, 3 or 6 to select the USART or 00897 * UART peripheral. 00898 * @param USART_GuardTime: specifies the guard time. 00899 * @retval None 00900 */ 00901 void USART_SetGuardTime(USART_TypeDef* USARTx, uint8_t USART_GuardTime) 00902 { 00903 /* Check the parameters */ 00904 assert_param(IS_USART_1236_PERIPH(USARTx)); 00905 00906 /* Clear the USART Guard time */ 00907 USARTx->GTPR &= USART_GTPR_PSC; 00908 /* Set the USART guard time */ 00909 USARTx->GTPR |= (uint16_t)((uint16_t)USART_GuardTime << 0x08); 00910 } 00911 00912 /** 00913 * @brief Enables or disables the USART's Smart Card mode. 00914 * @param USARTx: where x can be 1, 2, 3 or 6 to select the USART or 00915 * UART peripheral. 00916 * @param NewState: new state of the Smart Card mode. 00917 * This parameter can be: ENABLE or DISABLE. 00918 * @retval None 00919 */ 00920 void USART_SmartCardCmd(USART_TypeDef* USARTx, FunctionalState NewState) 00921 { 00922 /* Check the parameters */ 00923 assert_param(IS_USART_1236_PERIPH(USARTx)); 00924 assert_param(IS_FUNCTIONAL_STATE(NewState)); 00925 if (NewState != DISABLE) 00926 { 00927 /* Enable the SC mode by setting the SCEN bit in the CR3 register */ 00928 USARTx->CR3 |= USART_CR3_SCEN; 00929 } 00930 else 00931 { 00932 /* Disable the SC mode by clearing the SCEN bit in the CR3 register */ 00933 USARTx->CR3 &= (uint16_t)~((uint16_t)USART_CR3_SCEN); 00934 } 00935 } 00936 00937 /** 00938 * @brief Enables or disables NACK transmission. 00939 * @param USARTx: where x can be 1, 2, 3 or 6 to select the USART or 00940 * UART peripheral. 00941 * @param NewState: new state of the NACK transmission. 00942 * This parameter can be: ENABLE or DISABLE. 00943 * @retval None 00944 */ 00945 void USART_SmartCardNACKCmd(USART_TypeDef* USARTx, FunctionalState NewState) 00946 { 00947 /* Check the parameters */ 00948 assert_param(IS_USART_1236_PERIPH(USARTx)); 00949 assert_param(IS_FUNCTIONAL_STATE(NewState)); 00950 if (NewState != DISABLE) 00951 { 00952 /* Enable the NACK transmission by setting the NACK bit in the CR3 register */ 00953 USARTx->CR3 |= USART_CR3_NACK; 00954 } 00955 else 00956 { 00957 /* Disable the NACK transmission by clearing the NACK bit in the CR3 register */ 00958 USARTx->CR3 &= (uint16_t)~((uint16_t)USART_CR3_NACK); 00959 } 00960 } 00961 00962 /** 00963 * @} 00964 */ 00965 00966 /** @defgroup USART_Group7 IrDA mode functions 00967 * @brief IrDA mode functions 00968 * 00969 @verbatim 00970 =============================================================================== 00971 IrDA mode functions 00972 =============================================================================== 00973 00974 This subsection provides a set of functions allowing to manage the USART 00975 IrDA communication. 00976 00977 IrDA is a half duplex communication protocol. If the Transmitter is busy, any data 00978 on the IrDA receive line will be ignored by the IrDA decoder and if the Receiver 00979 is busy, data on the TX from the USART to IrDA will not be encoded by IrDA. 00980 While receiving data, transmission should be avoided as the data to be transmitted 00981 could be corrupted. 00982 00983 IrDA communication is possible through the following procedure: 00984 1. Program the Baud rate, Word length = 8 bits, Stop bits, Parity, Transmitter/Receiver 00985 modes and hardware flow control values using the USART_Init() function. 00986 2. Enable the USART using the USART_Cmd() function. 00987 3. Configures the IrDA pulse width by configuring the prescaler using 00988 the USART_SetPrescaler() function. 00989 4. Configures the IrDA USART_IrDAMode_LowPower or USART_IrDAMode_Normal mode 00990 using the USART_IrDAConfig() function. 00991 5. Enable the IrDA using the USART_IrDACmd() function. 00992 00993 @note A pulse of width less than two and greater than one PSC period(s) may or may 00994 not be rejected. 00995 @note The receiver set up time should be managed by software. The IrDA physical layer 00996 specification specifies a minimum of 10 ms delay between transmission and 00997 reception (IrDA is a half duplex protocol). 00998 @note In IrDA mode, the following bits must be kept cleared: 00999 - LINEN, STOP and CLKEN bits in the USART_CR2 register. 01000 - SCEN and HDSEL bits in the USART_CR3 register. 01001 01002 @endverbatim 01003 * @{ 01004 */ 01005 01006 /** 01007 * @brief Configures the USART's IrDA interface. 01008 * @param USARTx: where x can be 1, 2, 3, 4, 5 or 6 to select the USART or 01009 * UART peripheral. 01010 * @param USART_IrDAMode: specifies the IrDA mode. 01011 * This parameter can be one of the following values: 01012 * @arg USART_IrDAMode_LowPower 01013 * @arg USART_IrDAMode_Normal 01014 * @retval None 01015 */ 01016 void USART_IrDAConfig(USART_TypeDef* USARTx, uint16_t USART_IrDAMode) 01017 { 01018 /* Check the parameters */ 01019 assert_param(IS_USART_ALL_PERIPH(USARTx)); 01020 assert_param(IS_USART_IRDA_MODE(USART_IrDAMode)); 01021 01022 USARTx->CR3 &= (uint16_t)~((uint16_t)USART_CR3_IRLP); 01023 USARTx->CR3 |= USART_IrDAMode; 01024 } 01025 01026 /** 01027 * @brief Enables or disables the USART's IrDA interface. 01028 * @param USARTx: where x can be 1, 2, 3, 4, 5 or 6 to select the USART or 01029 * UART peripheral. 01030 * @param NewState: new state of the IrDA mode. 01031 * This parameter can be: ENABLE or DISABLE. 01032 * @retval None 01033 */ 01034 void USART_IrDACmd(USART_TypeDef* USARTx, FunctionalState NewState) 01035 { 01036 /* Check the parameters */ 01037 assert_param(IS_USART_ALL_PERIPH(USARTx)); 01038 assert_param(IS_FUNCTIONAL_STATE(NewState)); 01039 01040 if (NewState != DISABLE) 01041 { 01042 /* Enable the IrDA mode by setting the IREN bit in the CR3 register */ 01043 USARTx->CR3 |= USART_CR3_IREN; 01044 } 01045 else 01046 { 01047 /* Disable the IrDA mode by clearing the IREN bit in the CR3 register */ 01048 USARTx->CR3 &= (uint16_t)~((uint16_t)USART_CR3_IREN); 01049 } 01050 } 01051 01052 /** 01053 * @} 01054 */ 01055 01056 /** @defgroup USART_Group8 DMA transfers management functions 01057 * @brief DMA transfers management functions 01058 * 01059 @verbatim 01060 =============================================================================== 01061 DMA transfers management functions 01062 =============================================================================== 01063 01064 @endverbatim 01065 * @{ 01066 */ 01067 01068 /** 01069 * @brief Enables or disables the USART's DMA interface. 01070 * @param USARTx: where x can be 1, 2, 3, 4, 5 or 6 to select the USART or 01071 * UART peripheral. 01072 * @param USART_DMAReq: specifies the DMA request. 01073 * This parameter can be any combination of the following values: 01074 * @arg USART_DMAReq_Tx: USART DMA transmit request 01075 * @arg USART_DMAReq_Rx: USART DMA receive request 01076 * @param NewState: new state of the DMA Request sources. 01077 * This parameter can be: ENABLE or DISABLE. 01078 * @retval None 01079 */ 01080 void USART_DMACmd(USART_TypeDef* USARTx, uint16_t USART_DMAReq, FunctionalState NewState) 01081 { 01082 /* Check the parameters */ 01083 assert_param(IS_USART_ALL_PERIPH(USARTx)); 01084 assert_param(IS_USART_DMAREQ(USART_DMAReq)); 01085 assert_param(IS_FUNCTIONAL_STATE(NewState)); 01086 01087 if (NewState != DISABLE) 01088 { 01089 /* Enable the DMA transfer for selected requests by setting the DMAT and/or 01090 DMAR bits in the USART CR3 register */ 01091 USARTx->CR3 |= USART_DMAReq; 01092 } 01093 else 01094 { 01095 /* Disable the DMA transfer for selected requests by clearing the DMAT and/or 01096 DMAR bits in the USART CR3 register */ 01097 USARTx->CR3 &= (uint16_t)~USART_DMAReq; 01098 } 01099 } 01100 01101 /** 01102 * @} 01103 */ 01104 01105 /** @defgroup USART_Group9 Interrupts and flags management functions 01106 * @brief Interrupts and flags management functions 01107 * 01108 @verbatim 01109 =============================================================================== 01110 Interrupts and flags management functions 01111 =============================================================================== 01112 01113 This subsection provides a set of functions allowing to configure the USART 01114 Interrupts sources, DMA channels requests and check or clear the flags or 01115 pending bits status. 01116 The user should identify which mode will be used in his application to manage 01117 the communication: Polling mode, Interrupt mode or DMA mode. 01118 01119 Polling Mode 01120 ============= 01121 In Polling Mode, the SPI communication can be managed by 10 flags: 01122 1. USART_FLAG_TXE : to indicate the status of the transmit buffer register 01123 2. USART_FLAG_RXNE : to indicate the status of the receive buffer register 01124 3. USART_FLAG_TC : to indicate the status of the transmit operation 01125 4. USART_FLAG_IDLE : to indicate the status of the Idle Line 01126 5. USART_FLAG_CTS : to indicate the status of the nCTS input 01127 6. USART_FLAG_LBD : to indicate the status of the LIN break detection 01128 7. USART_FLAG_NE : to indicate if a noise error occur 01129 8. USART_FLAG_FE : to indicate if a frame error occur 01130 9. USART_FLAG_PE : to indicate if a parity error occur 01131 10. USART_FLAG_ORE : to indicate if an Overrun error occur 01132 01133 In this Mode it is advised to use the following functions: 01134 - FlagStatus USART_GetFlagStatus(USART_TypeDef* USARTx, uint16_t USART_FLAG); 01135 - void USART_ClearFlag(USART_TypeDef* USARTx, uint16_t USART_FLAG); 01136 01137 Interrupt Mode 01138 =============== 01139 In Interrupt Mode, the USART communication can be managed by 8 interrupt sources 01140 and 10 pending bits: 01141 01142 Pending Bits: 01143 ------------- 01144 1. USART_IT_TXE : to indicate the status of the transmit buffer register 01145 2. USART_IT_RXNE : to indicate the status of the receive buffer register 01146 3. USART_IT_TC : to indicate the status of the transmit operation 01147 4. USART_IT_IDLE : to indicate the status of the Idle Line 01148 5. USART_IT_CTS : to indicate the status of the nCTS input 01149 6. USART_IT_LBD : to indicate the status of the LIN break detection 01150 7. USART_IT_NE : to indicate if a noise error occur 01151 8. USART_IT_FE : to indicate if a frame error occur 01152 9. USART_IT_PE : to indicate if a parity error occur 01153 10. USART_IT_ORE : to indicate if an Overrun error occur 01154 01155 Interrupt Source: 01156 ----------------- 01157 1. USART_IT_TXE : specifies the interrupt source for the Tx buffer empty 01158 interrupt. 01159 2. USART_IT_RXNE : specifies the interrupt source for the Rx buffer not 01160 empty interrupt. 01161 3. USART_IT_TC : specifies the interrupt source for the Transmit complete 01162 interrupt. 01163 4. USART_IT_IDLE : specifies the interrupt source for the Idle Line interrupt. 01164 5. USART_IT_CTS : specifies the interrupt source for the CTS interrupt. 01165 6. USART_IT_LBD : specifies the interrupt source for the LIN break detection 01166 interrupt. 01167 7. USART_IT_PE : specifies the interrupt source for the parity error interrupt. 01168 8. USART_IT_ERR : specifies the interrupt source for the errors interrupt. 01169 01170 @note Some parameters are coded in order to use them as interrupt source or as pending bits. 01171 01172 In this Mode it is advised to use the following functions: 01173 - void USART_ITConfig(USART_TypeDef* USARTx, uint16_t USART_IT, FunctionalState NewState); 01174 - ITStatus USART_GetITStatus(USART_TypeDef* USARTx, uint16_t USART_IT); 01175 - void USART_ClearITPendingBit(USART_TypeDef* USARTx, uint16_t USART_IT); 01176 01177 DMA Mode 01178 ======== 01179 In DMA Mode, the USART communication can be managed by 2 DMA Channel requests: 01180 1. USART_DMAReq_Tx: specifies the Tx buffer DMA transfer request 01181 2. USART_DMAReq_Rx: specifies the Rx buffer DMA transfer request 01182 01183 In this Mode it is advised to use the following function: 01184 - void USART_DMACmd(USART_TypeDef* USARTx, uint16_t USART_DMAReq, FunctionalState NewState); 01185 01186 @endverbatim 01187 * @{ 01188 */ 01189 01190 /** 01191 * @brief Enables or disables the specified USART interrupts. 01192 * @param USARTx: where x can be 1, 2, 3, 4, 5 or 6 to select the USART or 01193 * UART peripheral. 01194 * @param USART_IT: specifies the USART interrupt sources to be enabled or disabled. 01195 * This parameter can be one of the following values: 01196 * @arg USART_IT_CTS: CTS change interrupt 01197 * @arg USART_IT_LBD: LIN Break detection interrupt 01198 * @arg USART_IT_TXE: Transmit Data Register empty interrupt 01199 * @arg USART_IT_TC: Transmission complete interrupt 01200 * @arg USART_IT_RXNE: Receive Data register not empty interrupt 01201 * @arg USART_IT_IDLE: Idle line detection interrupt 01202 * @arg USART_IT_PE: Parity Error interrupt 01203 * @arg USART_IT_ERR: Error interrupt(Frame error, noise error, overrun error) 01204 * @param NewState: new state of the specified USARTx interrupts. 01205 * This parameter can be: ENABLE or DISABLE. 01206 * @retval None 01207 */ 01208 void USART_ITConfig(USART_TypeDef* USARTx, uint16_t USART_IT, FunctionalState NewState) 01209 { 01210 uint32_t usartreg = 0x00, itpos = 0x00, itmask = 0x00; 01211 uint32_t usartxbase = 0x00; 01212 /* Check the parameters */ 01213 assert_param(IS_USART_ALL_PERIPH(USARTx)); 01214 assert_param(IS_USART_CONFIG_IT(USART_IT)); 01215 assert_param(IS_FUNCTIONAL_STATE(NewState)); 01216 01217 /* The CTS interrupt is not available for UART4 and UART5 */ 01218 if (USART_IT == USART_IT_CTS) 01219 { 01220 assert_param(IS_USART_1236_PERIPH(USARTx)); 01221 } 01222 01223 usartxbase = (uint32_t)USARTx; 01224 01225 /* Get the USART register index */ 01226 usartreg = (((uint8_t)USART_IT) >> 0x05); 01227 01228 /* Get the interrupt position */ 01229 itpos = USART_IT & IT_MASK; 01230 itmask = (((uint32_t)0x01) << itpos); 01231 01232 if (usartreg == 0x01) /* The IT is in CR1 register */ 01233 { 01234 usartxbase += 0x0C; 01235 } 01236 else if (usartreg == 0x02) /* The IT is in CR2 register */ 01237 { 01238 usartxbase += 0x10; 01239 } 01240 else /* The IT is in CR3 register */ 01241 { 01242 usartxbase += 0x14; 01243 } 01244 if (NewState != DISABLE) 01245 { 01246 *(__IO uint32_t*)usartxbase |= itmask; 01247 } 01248 else 01249 { 01250 *(__IO uint32_t*)usartxbase &= ~itmask; 01251 } 01252 } 01253 01254 /** 01255 * @brief Checks whether the specified USART flag is set or not. 01256 * @param USARTx: where x can be 1, 2, 3, 4, 5 or 6 to select the USART or 01257 * UART peripheral. 01258 * @param USART_FLAG: specifies the flag to check. 01259 * This parameter can be one of the following values: 01260 * @arg USART_FLAG_CTS: CTS Change flag (not available for UART4 and UART5) 01261 * @arg USART_FLAG_LBD: LIN Break detection flag 01262 * @arg USART_FLAG_TXE: Transmit data register empty flag 01263 * @arg USART_FLAG_TC: Transmission Complete flag 01264 * @arg USART_FLAG_RXNE: Receive data register not empty flag 01265 * @arg USART_FLAG_IDLE: Idle Line detection flag 01266 * @arg USART_FLAG_ORE: OverRun Error flag 01267 * @arg USART_FLAG_NE: Noise Error flag 01268 * @arg USART_FLAG_FE: Framing Error flag 01269 * @arg USART_FLAG_PE: Parity Error flag 01270 * @retval The new state of USART_FLAG (SET or RESET). 01271 */ 01272 FlagStatus USART_GetFlagStatus(USART_TypeDef* USARTx, uint16_t USART_FLAG) 01273 { 01274 FlagStatus bitstatus = RESET; 01275 /* Check the parameters */ 01276 assert_param(IS_USART_ALL_PERIPH(USARTx)); 01277 assert_param(IS_USART_FLAG(USART_FLAG)); 01278 01279 /* The CTS flag is not available for UART4 and UART5 */ 01280 if (USART_FLAG == USART_FLAG_CTS) 01281 { 01282 assert_param(IS_USART_1236_PERIPH(USARTx)); 01283 } 01284 01285 if ((USARTx->SR & USART_FLAG) != (uint16_t)RESET) 01286 { 01287 bitstatus = SET; 01288 } 01289 else 01290 { 01291 bitstatus = RESET; 01292 } 01293 return bitstatus; 01294 } 01295 01296 /** 01297 * @brief Clears the USARTx's pending flags. 01298 * @param USARTx: where x can be 1, 2, 3, 4, 5 or 6 to select the USART or 01299 * UART peripheral. 01300 * @param USART_FLAG: specifies the flag to clear. 01301 * This parameter can be any combination of the following values: 01302 * @arg USART_FLAG_CTS: CTS Change flag (not available for UART4 and UART5). 01303 * @arg USART_FLAG_LBD: LIN Break detection flag. 01304 * @arg USART_FLAG_TC: Transmission Complete flag. 01305 * @arg USART_FLAG_RXNE: Receive data register not empty flag. 01306 * 01307 * @note PE (Parity error), FE (Framing error), NE (Noise error), ORE (OverRun 01308 * error) and IDLE (Idle line detected) flags are cleared by software 01309 * sequence: a read operation to USART_SR register (USART_GetFlagStatus()) 01310 * followed by a read operation to USART_DR register (USART_ReceiveData()). 01311 * @note RXNE flag can be also cleared by a read to the USART_DR register 01312 * (USART_ReceiveData()). 01313 * @note TC flag can be also cleared by software sequence: a read operation to 01314 * USART_SR register (USART_GetFlagStatus()) followed by a write operation 01315 * to USART_DR register (USART_SendData()). 01316 * @note TXE flag is cleared only by a write to the USART_DR register 01317 * (USART_SendData()). 01318 * 01319 * @retval None 01320 */ 01321 void USART_ClearFlag(USART_TypeDef* USARTx, uint16_t USART_FLAG) 01322 { 01323 /* Check the parameters */ 01324 assert_param(IS_USART_ALL_PERIPH(USARTx)); 01325 assert_param(IS_USART_CLEAR_FLAG(USART_FLAG)); 01326 01327 /* The CTS flag is not available for UART4 and UART5 */ 01328 if ((USART_FLAG & USART_FLAG_CTS) == USART_FLAG_CTS) 01329 { 01330 assert_param(IS_USART_1236_PERIPH(USARTx)); 01331 } 01332 01333 USARTx->SR = (uint16_t)~USART_FLAG; 01334 } 01335 01336 /** 01337 * @brief Checks whether the specified USART interrupt has occurred or not. 01338 * @param USARTx: where x can be 1, 2, 3, 4, 5 or 6 to select the USART or 01339 * UART peripheral. 01340 * @param USART_IT: specifies the USART interrupt source to check. 01341 * This parameter can be one of the following values: 01342 * @arg USART_IT_CTS: CTS change interrupt (not available for UART4 and UART5) 01343 * @arg USART_IT_LBD: LIN Break detection interrupt 01344 * @arg USART_IT_TXE: Transmit Data Register empty interrupt 01345 * @arg USART_IT_TC: Transmission complete interrupt 01346 * @arg USART_IT_RXNE: Receive Data register not empty interrupt 01347 * @arg USART_IT_IDLE: Idle line detection interrupt 01348 * @arg USART_IT_ORE_RX : OverRun Error interrupt if the RXNEIE bit is set 01349 * @arg USART_IT_ORE_ER : OverRun Error interrupt if the EIE bit is set 01350 * @arg USART_IT_NE: Noise Error interrupt 01351 * @arg USART_IT_FE: Framing Error interrupt 01352 * @arg USART_IT_PE: Parity Error interrupt 01353 * @retval The new state of USART_IT (SET or RESET). 01354 */ 01355 ITStatus USART_GetITStatus(USART_TypeDef* USARTx, uint16_t USART_IT) 01356 { 01357 uint32_t bitpos = 0x00, itmask = 0x00, usartreg = 0x00; 01358 ITStatus bitstatus = RESET; 01359 /* Check the parameters */ 01360 assert_param(IS_USART_ALL_PERIPH(USARTx)); 01361 assert_param(IS_USART_GET_IT(USART_IT)); 01362 01363 /* The CTS interrupt is not available for UART4 and UART5 */ 01364 if (USART_IT == USART_IT_CTS) 01365 { 01366 assert_param(IS_USART_1236_PERIPH(USARTx)); 01367 } 01368 01369 /* Get the USART register index */ 01370 usartreg = (((uint8_t)USART_IT) >> 0x05); 01371 /* Get the interrupt position */ 01372 itmask = USART_IT & IT_MASK; 01373 itmask = (uint32_t)0x01 << itmask; 01374 01375 if (usartreg == 0x01) /* The IT is in CR1 register */ 01376 { 01377 itmask &= USARTx->CR1; 01378 } 01379 else if (usartreg == 0x02) /* The IT is in CR2 register */ 01380 { 01381 itmask &= USARTx->CR2; 01382 } 01383 else /* The IT is in CR3 register */ 01384 { 01385 itmask &= USARTx->CR3; 01386 } 01387 01388 bitpos = USART_IT >> 0x08; 01389 bitpos = (uint32_t)0x01 << bitpos; 01390 bitpos &= USARTx->SR; 01391 if ((itmask != (uint16_t)RESET)&&(bitpos != (uint16_t)RESET)) 01392 { 01393 bitstatus = SET; 01394 } 01395 else 01396 { 01397 bitstatus = RESET; 01398 } 01399 01400 return bitstatus; 01401 } 01402 01403 /** 01404 * @brief Clears the USARTx's interrupt pending bits. 01405 * @param USARTx: where x can be 1, 2, 3, 4, 5 or 6 to select the USART or 01406 * UART peripheral. 01407 * @param USART_IT: specifies the interrupt pending bit to clear. 01408 * This parameter can be one of the following values: 01409 * @arg USART_IT_CTS: CTS change interrupt (not available for UART4 and UART5) 01410 * @arg USART_IT_LBD: LIN Break detection interrupt 01411 * @arg USART_IT_TC: Transmission complete interrupt. 01412 * @arg USART_IT_RXNE: Receive Data register not empty interrupt. 01413 * 01414 * @note PE (Parity error), FE (Framing error), NE (Noise error), ORE (OverRun 01415 * error) and IDLE (Idle line detected) pending bits are cleared by 01416 * software sequence: a read operation to USART_SR register 01417 * (USART_GetITStatus()) followed by a read operation to USART_DR register 01418 * (USART_ReceiveData()). 01419 * @note RXNE pending bit can be also cleared by a read to the USART_DR register 01420 * (USART_ReceiveData()). 01421 * @note TC pending bit can be also cleared by software sequence: a read 01422 * operation to USART_SR register (USART_GetITStatus()) followed by a write 01423 * operation to USART_DR register (USART_SendData()). 01424 * @note TXE pending bit is cleared only by a write to the USART_DR register 01425 * (USART_SendData()). 01426 * 01427 * @retval None 01428 */ 01429 void USART_ClearITPendingBit(USART_TypeDef* USARTx, uint16_t USART_IT) 01430 { 01431 uint16_t bitpos = 0x00, itmask = 0x00; 01432 /* Check the parameters */ 01433 assert_param(IS_USART_ALL_PERIPH(USARTx)); 01434 assert_param(IS_USART_CLEAR_IT(USART_IT)); 01435 01436 /* The CTS interrupt is not available for UART4 and UART5 */ 01437 if (USART_IT == USART_IT_CTS) 01438 { 01439 assert_param(IS_USART_1236_PERIPH(USARTx)); 01440 } 01441 01442 bitpos = USART_IT >> 0x08; 01443 itmask = ((uint16_t)0x01 << (uint16_t)bitpos); 01444 USARTx->SR = (uint16_t)~itmask; 01445 } 01446 01447 /** 01448 * @} 01449 */ 01450 01451 /** 01452 * @} 01453 */ 01454 01455 /** 01456 * @} 01457 */ 01458 01459 /** 01460 * @} 01461 */ 01462 01463 /******************* (C) COPYRIGHT 2011 STMicroelectronics *****END OF FILE****/ 01464
Generated on Tue Jul 12 2022 19:53:13 by
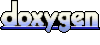