
Simple "hello world" style program for X-NUCLEO-IKS01A1 MEMS Inertial
Dependencies: BLE_API X_NUCLEO_IDB0XA1 X_NUCLEO_IKS01A1 mbed
Fork of HelloWorld_IKS01A1 by
stm32f4xx_tim.c
00001 /** 00002 ****************************************************************************** 00003 * @file stm32f4xx_tim.c 00004 * @author MCD Application Team 00005 * @version V1.0.0 00006 * @date 30-September-2011 00007 * @brief This file provides firmware functions to manage the following 00008 * functionalities of the TIM peripheral: 00009 * - TimeBase management 00010 * - Output Compare management 00011 * - Input Capture management 00012 * - Advanced-control timers (TIM1 and TIM8) specific features 00013 * - Interrupts, DMA and flags management 00014 * - Clocks management 00015 * - Synchronization management 00016 * - Specific interface management 00017 * - Specific remapping management 00018 * 00019 * @verbatim 00020 * 00021 * =================================================================== 00022 * How to use this driver 00023 * =================================================================== 00024 * This driver provides functions to configure and program the TIM 00025 * of all STM32F4xx devices. 00026 * These functions are split in 9 groups: 00027 * 00028 * 1. TIM TimeBase management: this group includes all needed functions 00029 * to configure the TM Timebase unit: 00030 * - Set/Get Prescaler 00031 * - Set/Get Autoreload 00032 * - Counter modes configuration 00033 * - Set Clock division 00034 * - Select the One Pulse mode 00035 * - Update Request Configuration 00036 * - Update Disable Configuration 00037 * - Auto-Preload Configuration 00038 * - Enable/Disable the counter 00039 * 00040 * 2. TIM Output Compare management: this group includes all needed 00041 * functions to configure the Capture/Compare unit used in Output 00042 * compare mode: 00043 * - Configure each channel, independently, in Output Compare mode 00044 * - Select the output compare modes 00045 * - Select the Polarities of each channel 00046 * - Set/Get the Capture/Compare register values 00047 * - Select the Output Compare Fast mode 00048 * - Select the Output Compare Forced mode 00049 * - Output Compare-Preload Configuration 00050 * - Clear Output Compare Reference 00051 * - Select the OCREF Clear signal 00052 * - Enable/Disable the Capture/Compare Channels 00053 * 00054 * 3. TIM Input Capture management: this group includes all needed 00055 * functions to configure the Capture/Compare unit used in 00056 * Input Capture mode: 00057 * - Configure each channel in input capture mode 00058 * - Configure Channel1/2 in PWM Input mode 00059 * - Set the Input Capture Prescaler 00060 * - Get the Capture/Compare values 00061 * 00062 * 4. Advanced-control timers (TIM1 and TIM8) specific features 00063 * - Configures the Break input, dead time, Lock level, the OSSI, 00064 * the OSSR State and the AOE(automatic output enable) 00065 * - Enable/Disable the TIM peripheral Main Outputs 00066 * - Select the Commutation event 00067 * - Set/Reset the Capture Compare Preload Control bit 00068 * 00069 * 5. TIM interrupts, DMA and flags management 00070 * - Enable/Disable interrupt sources 00071 * - Get flags status 00072 * - Clear flags/ Pending bits 00073 * - Enable/Disable DMA requests 00074 * - Configure DMA burst mode 00075 * - Select CaptureCompare DMA request 00076 * 00077 * 6. TIM clocks management: this group includes all needed functions 00078 * to configure the clock controller unit: 00079 * - Select internal/External clock 00080 * - Select the external clock mode: ETR(Mode1/Mode2), TIx or ITRx 00081 * 00082 * 7. TIM synchronization management: this group includes all needed 00083 * functions to configure the Synchronization unit: 00084 * - Select Input Trigger 00085 * - Select Output Trigger 00086 * - Select Master Slave Mode 00087 * - ETR Configuration when used as external trigger 00088 * 00089 * 8. TIM specific interface management, this group includes all 00090 * needed functions to use the specific TIM interface: 00091 * - Encoder Interface Configuration 00092 * - Select Hall Sensor 00093 * 00094 * 9. TIM specific remapping management includes the Remapping 00095 * configuration of specific timers 00096 * 00097 * @endverbatim 00098 * 00099 ****************************************************************************** 00100 * @attention 00101 * 00102 * THE PRESENT FIRMWARE WHICH IS FOR GUIDANCE ONLY AIMS AT PROVIDING CUSTOMERS 00103 * WITH CODING INFORMATION REGARDING THEIR PRODUCTS IN ORDER FOR THEM TO SAVE 00104 * TIME. AS A RESULT, STMICROELECTRONICS SHALL NOT BE HELD LIABLE FOR ANY 00105 * DIRECT, INDIRECT OR CONSEQUENTIAL DAMAGES WITH RESPECT TO ANY CLAIMS ARISING 00106 * FROM THE CONTENT OF SUCH FIRMWARE AND/OR THE USE MADE BY CUSTOMERS OF THE 00107 * CODING INFORMATION CONTAINED HEREIN IN CONNECTION WITH THEIR PRODUCTS. 00108 * 00109 * <h2><center>© COPYRIGHT 2011 STMicroelectronics</center></h2> 00110 ****************************************************************************** 00111 */ 00112 00113 /* Includes ------------------------------------------------------------------*/ 00114 #include "stm32f4xx_tim.h" 00115 #include "stm32f4xx_rcc.h" 00116 00117 /** @addtogroup STM32F4xx_StdPeriph_Driver 00118 * @{ 00119 */ 00120 00121 /** @defgroup TIM 00122 * @brief TIM driver modules 00123 * @{ 00124 */ 00125 00126 /* Private typedef -----------------------------------------------------------*/ 00127 /* Private define ------------------------------------------------------------*/ 00128 00129 /* ---------------------- TIM registers bit mask ------------------------ */ 00130 #define SMCR_ETR_MASK ((uint16_t)0x00FF) 00131 #define CCMR_OFFSET ((uint16_t)0x0018) 00132 #define CCER_CCE_SET ((uint16_t)0x0001) 00133 #define CCER_CCNE_SET ((uint16_t)0x0004) 00134 #define CCMR_OC13M_MASK ((uint16_t)0xFF8F) 00135 #define CCMR_OC24M_MASK ((uint16_t)0x8FFF) 00136 00137 /* Private macro -------------------------------------------------------------*/ 00138 /* Private variables ---------------------------------------------------------*/ 00139 /* Private function prototypes -----------------------------------------------*/ 00140 static void TI1_Config(TIM_TypeDef* TIMx, uint16_t TIM_ICPolarity, uint16_t TIM_ICSelection, 00141 uint16_t TIM_ICFilter); 00142 static void TI2_Config(TIM_TypeDef* TIMx, uint16_t TIM_ICPolarity, uint16_t TIM_ICSelection, 00143 uint16_t TIM_ICFilter); 00144 static void TI3_Config(TIM_TypeDef* TIMx, uint16_t TIM_ICPolarity, uint16_t TIM_ICSelection, 00145 uint16_t TIM_ICFilter); 00146 static void TI4_Config(TIM_TypeDef* TIMx, uint16_t TIM_ICPolarity, uint16_t TIM_ICSelection, 00147 uint16_t TIM_ICFilter); 00148 00149 /* Private functions ---------------------------------------------------------*/ 00150 00151 /** @defgroup TIM_Private_Functions 00152 * @{ 00153 */ 00154 00155 /** @defgroup TIM_Group1 TimeBase management functions 00156 * @brief TimeBase management functions 00157 * 00158 @verbatim 00159 =============================================================================== 00160 TimeBase management functions 00161 =============================================================================== 00162 00163 =================================================================== 00164 TIM Driver: how to use it in Timing(Time base) Mode 00165 =================================================================== 00166 To use the Timer in Timing(Time base) mode, the following steps are mandatory: 00167 00168 1. Enable TIM clock using RCC_APBxPeriphClockCmd(RCC_APBxPeriph_TIMx, ENABLE) function 00169 00170 2. Fill the TIM_TimeBaseInitStruct with the desired parameters. 00171 00172 3. Call TIM_TimeBaseInit(TIMx, &TIM_TimeBaseInitStruct) to configure the Time Base unit 00173 with the corresponding configuration 00174 00175 4. Enable the NVIC if you need to generate the update interrupt. 00176 00177 5. Enable the corresponding interrupt using the function TIM_ITConfig(TIMx, TIM_IT_Update) 00178 00179 6. Call the TIM_Cmd(ENABLE) function to enable the TIM counter. 00180 00181 Note1: All other functions can be used separately to modify, if needed, 00182 a specific feature of the Timer. 00183 00184 @endverbatim 00185 * @{ 00186 */ 00187 00188 /** 00189 * @brief Deinitializes the TIMx peripheral registers to their default reset values. 00190 * @param TIMx: where x can be 1 to 14 to select the TIM peripheral. 00191 * @retval None 00192 00193 */ 00194 void TIM_DeInit(TIM_TypeDef* TIMx) 00195 { 00196 /* Check the parameters */ 00197 assert_param(IS_TIM_ALL_PERIPH(TIMx)); 00198 00199 if (TIMx == TIM1) 00200 { 00201 RCC_APB2PeriphResetCmd(RCC_APB2Periph_TIM1, ENABLE); 00202 RCC_APB2PeriphResetCmd(RCC_APB2Periph_TIM1, DISABLE); 00203 } 00204 else if (TIMx == TIM2) 00205 { 00206 RCC_APB1PeriphResetCmd(RCC_APB1Periph_TIM2, ENABLE); 00207 RCC_APB1PeriphResetCmd(RCC_APB1Periph_TIM2, DISABLE); 00208 } 00209 else if (TIMx == TIM3) 00210 { 00211 RCC_APB1PeriphResetCmd(RCC_APB1Periph_TIM3, ENABLE); 00212 RCC_APB1PeriphResetCmd(RCC_APB1Periph_TIM3, DISABLE); 00213 } 00214 else if (TIMx == TIM4) 00215 { 00216 RCC_APB1PeriphResetCmd(RCC_APB1Periph_TIM4, ENABLE); 00217 RCC_APB1PeriphResetCmd(RCC_APB1Periph_TIM4, DISABLE); 00218 } 00219 else if (TIMx == TIM5) 00220 { 00221 RCC_APB1PeriphResetCmd(RCC_APB1Periph_TIM5, ENABLE); 00222 RCC_APB1PeriphResetCmd(RCC_APB1Periph_TIM5, DISABLE); 00223 } 00224 else if (TIMx == TIM6) 00225 { 00226 RCC_APB1PeriphResetCmd(RCC_APB1Periph_TIM6, ENABLE); 00227 RCC_APB1PeriphResetCmd(RCC_APB1Periph_TIM6, DISABLE); 00228 } 00229 else if (TIMx == TIM7) 00230 { 00231 RCC_APB1PeriphResetCmd(RCC_APB1Periph_TIM7, ENABLE); 00232 RCC_APB1PeriphResetCmd(RCC_APB1Periph_TIM7, DISABLE); 00233 } 00234 else if (TIMx == TIM8) 00235 { 00236 RCC_APB2PeriphResetCmd(RCC_APB2Periph_TIM8, ENABLE); 00237 RCC_APB2PeriphResetCmd(RCC_APB2Periph_TIM8, DISABLE); 00238 } 00239 else if (TIMx == TIM9) 00240 { 00241 RCC_APB2PeriphResetCmd(RCC_APB2Periph_TIM9, ENABLE); 00242 RCC_APB2PeriphResetCmd(RCC_APB2Periph_TIM9, DISABLE); 00243 } 00244 else if (TIMx == TIM10) 00245 { 00246 RCC_APB2PeriphResetCmd(RCC_APB2Periph_TIM10, ENABLE); 00247 RCC_APB2PeriphResetCmd(RCC_APB2Periph_TIM10, DISABLE); 00248 } 00249 else if (TIMx == TIM11) 00250 { 00251 RCC_APB2PeriphResetCmd(RCC_APB2Periph_TIM11, ENABLE); 00252 RCC_APB2PeriphResetCmd(RCC_APB2Periph_TIM11, DISABLE); 00253 } 00254 else if (TIMx == TIM12) 00255 { 00256 RCC_APB1PeriphResetCmd(RCC_APB1Periph_TIM12, ENABLE); 00257 RCC_APB1PeriphResetCmd(RCC_APB1Periph_TIM12, DISABLE); 00258 } 00259 else if (TIMx == TIM13) 00260 { 00261 RCC_APB1PeriphResetCmd(RCC_APB1Periph_TIM13, ENABLE); 00262 RCC_APB1PeriphResetCmd(RCC_APB1Periph_TIM13, DISABLE); 00263 } 00264 else 00265 { 00266 if (TIMx == TIM14) 00267 { 00268 RCC_APB1PeriphResetCmd(RCC_APB1Periph_TIM14, ENABLE); 00269 RCC_APB1PeriphResetCmd(RCC_APB1Periph_TIM14, DISABLE); 00270 } 00271 } 00272 } 00273 00274 /** 00275 * @brief Initializes the TIMx Time Base Unit peripheral according to 00276 * the specified parameters in the TIM_TimeBaseInitStruct. 00277 * @param TIMx: where x can be 1 to 14 to select the TIM peripheral. 00278 * @param TIM_TimeBaseInitStruct: pointer to a TIM_TimeBaseInitTypeDef structure 00279 * that contains the configuration information for the specified TIM peripheral. 00280 * @retval None 00281 */ 00282 void TIM_TimeBaseInit(TIM_TypeDef* TIMx, TIM_TimeBaseInitTypeDef* TIM_TimeBaseInitStruct) 00283 { 00284 uint16_t tmpcr1 = 0; 00285 00286 /* Check the parameters */ 00287 assert_param(IS_TIM_ALL_PERIPH(TIMx)); 00288 assert_param(IS_TIM_COUNTER_MODE(TIM_TimeBaseInitStruct->TIM_CounterMode)); 00289 assert_param(IS_TIM_CKD_DIV(TIM_TimeBaseInitStruct->TIM_ClockDivision)); 00290 00291 tmpcr1 = TIMx->CR1; 00292 00293 if((TIMx == TIM1) || (TIMx == TIM8)|| 00294 (TIMx == TIM2) || (TIMx == TIM3)|| 00295 (TIMx == TIM4) || (TIMx == TIM5)) 00296 { 00297 /* Select the Counter Mode */ 00298 tmpcr1 &= (uint16_t)(~(TIM_CR1_DIR | TIM_CR1_CMS)); 00299 tmpcr1 |= (uint32_t)TIM_TimeBaseInitStruct->TIM_CounterMode; 00300 } 00301 00302 if((TIMx != TIM6) && (TIMx != TIM7)) 00303 { 00304 /* Set the clock division */ 00305 tmpcr1 &= (uint16_t)(~TIM_CR1_CKD); 00306 tmpcr1 |= (uint32_t)TIM_TimeBaseInitStruct->TIM_ClockDivision; 00307 } 00308 00309 TIMx->CR1 = tmpcr1; 00310 00311 /* Set the Autoreload value */ 00312 TIMx->ARR = TIM_TimeBaseInitStruct->TIM_Period ; 00313 00314 /* Set the Prescaler value */ 00315 TIMx->PSC = TIM_TimeBaseInitStruct->TIM_Prescaler; 00316 00317 if ((TIMx == TIM1) || (TIMx == TIM8)) 00318 { 00319 /* Set the Repetition Counter value */ 00320 TIMx->RCR = TIM_TimeBaseInitStruct->TIM_RepetitionCounter; 00321 } 00322 00323 /* Generate an update event to reload the Prescaler 00324 and the repetition counter(only for TIM1 and TIM8) value immediatly */ 00325 TIMx->EGR = TIM_PSCReloadMode_Immediate; 00326 } 00327 00328 /** 00329 * @brief Fills each TIM_TimeBaseInitStruct member with its default value. 00330 * @param TIM_TimeBaseInitStruct : pointer to a TIM_TimeBaseInitTypeDef 00331 * structure which will be initialized. 00332 * @retval None 00333 */ 00334 void TIM_TimeBaseStructInit(TIM_TimeBaseInitTypeDef* TIM_TimeBaseInitStruct) 00335 { 00336 /* Set the default configuration */ 00337 TIM_TimeBaseInitStruct->TIM_Period = 0xFFFFFFFF; 00338 TIM_TimeBaseInitStruct->TIM_Prescaler = 0x0000; 00339 TIM_TimeBaseInitStruct->TIM_ClockDivision = TIM_CKD_DIV1; 00340 TIM_TimeBaseInitStruct->TIM_CounterMode = TIM_CounterMode_Up; 00341 TIM_TimeBaseInitStruct->TIM_RepetitionCounter = 0x0000; 00342 } 00343 00344 /** 00345 * @brief Configures the TIMx Prescaler. 00346 * @param TIMx: where x can be 1 to 14 to select the TIM peripheral. 00347 * @param Prescaler: specifies the Prescaler Register value 00348 * @param TIM_PSCReloadMode: specifies the TIM Prescaler Reload mode 00349 * This parameter can be one of the following values: 00350 * @arg TIM_PSCReloadMode_Update: The Prescaler is loaded at the update event. 00351 * @arg TIM_PSCReloadMode_Immediate: The Prescaler is loaded immediatly. 00352 * @retval None 00353 */ 00354 void TIM_PrescalerConfig(TIM_TypeDef* TIMx, uint16_t Prescaler, uint16_t TIM_PSCReloadMode) 00355 { 00356 /* Check the parameters */ 00357 assert_param(IS_TIM_ALL_PERIPH(TIMx)); 00358 assert_param(IS_TIM_PRESCALER_RELOAD(TIM_PSCReloadMode)); 00359 /* Set the Prescaler value */ 00360 TIMx->PSC = Prescaler; 00361 /* Set or reset the UG Bit */ 00362 TIMx->EGR = TIM_PSCReloadMode; 00363 } 00364 00365 /** 00366 * @brief Specifies the TIMx Counter Mode to be used. 00367 * @param TIMx: where x can be 1, 2, 3, 4, 5 or 8 to select the TIM peripheral. 00368 * @param TIM_CounterMode: specifies the Counter Mode to be used 00369 * This parameter can be one of the following values: 00370 * @arg TIM_CounterMode_Up: TIM Up Counting Mode 00371 * @arg TIM_CounterMode_Down: TIM Down Counting Mode 00372 * @arg TIM_CounterMode_CenterAligned1: TIM Center Aligned Mode1 00373 * @arg TIM_CounterMode_CenterAligned2: TIM Center Aligned Mode2 00374 * @arg TIM_CounterMode_CenterAligned3: TIM Center Aligned Mode3 00375 * @retval None 00376 */ 00377 void TIM_CounterModeConfig(TIM_TypeDef* TIMx, uint16_t TIM_CounterMode) 00378 { 00379 uint16_t tmpcr1 = 0; 00380 00381 /* Check the parameters */ 00382 assert_param(IS_TIM_LIST3_PERIPH(TIMx)); 00383 assert_param(IS_TIM_COUNTER_MODE(TIM_CounterMode)); 00384 00385 tmpcr1 = TIMx->CR1; 00386 00387 /* Reset the CMS and DIR Bits */ 00388 tmpcr1 &= (uint16_t)~(TIM_CR1_DIR | TIM_CR1_CMS); 00389 00390 /* Set the Counter Mode */ 00391 tmpcr1 |= TIM_CounterMode; 00392 00393 /* Write to TIMx CR1 register */ 00394 TIMx->CR1 = tmpcr1; 00395 } 00396 00397 /** 00398 * @brief Sets the TIMx Counter Register value 00399 * @param TIMx: where x can be 1 to 14 to select the TIM peripheral. 00400 * @param Counter: specifies the Counter register new value. 00401 * @retval None 00402 */ 00403 void TIM_SetCounter(TIM_TypeDef* TIMx, uint32_t Counter) 00404 { 00405 /* Check the parameters */ 00406 assert_param(IS_TIM_ALL_PERIPH(TIMx)); 00407 00408 /* Set the Counter Register value */ 00409 TIMx->CNT = Counter; 00410 } 00411 00412 /** 00413 * @brief Sets the TIMx Autoreload Register value 00414 * @param TIMx: where x can be 1 to 14 to select the TIM peripheral. 00415 * @param Autoreload: specifies the Autoreload register new value. 00416 * @retval None 00417 */ 00418 void TIM_SetAutoreload(TIM_TypeDef* TIMx, uint32_t Autoreload) 00419 { 00420 /* Check the parameters */ 00421 assert_param(IS_TIM_ALL_PERIPH(TIMx)); 00422 00423 /* Set the Autoreload Register value */ 00424 TIMx->ARR = Autoreload; 00425 } 00426 00427 /** 00428 * @brief Gets the TIMx Counter value. 00429 * @param TIMx: where x can be 1 to 14 to select the TIM peripheral. 00430 * @retval Counter Register value 00431 */ 00432 uint32_t TIM_GetCounter(TIM_TypeDef* TIMx) 00433 { 00434 /* Check the parameters */ 00435 assert_param(IS_TIM_ALL_PERIPH(TIMx)); 00436 00437 /* Get the Counter Register value */ 00438 return TIMx->CNT; 00439 } 00440 00441 /** 00442 * @brief Gets the TIMx Prescaler value. 00443 * @param TIMx: where x can be 1 to 14 to select the TIM peripheral. 00444 * @retval Prescaler Register value. 00445 */ 00446 uint16_t TIM_GetPrescaler(TIM_TypeDef* TIMx) 00447 { 00448 /* Check the parameters */ 00449 assert_param(IS_TIM_ALL_PERIPH(TIMx)); 00450 00451 /* Get the Prescaler Register value */ 00452 return TIMx->PSC; 00453 } 00454 00455 /** 00456 * @brief Enables or Disables the TIMx Update event. 00457 * @param TIMx: where x can be 1 to 14 to select the TIM peripheral. 00458 * @param NewState: new state of the TIMx UDIS bit 00459 * This parameter can be: ENABLE or DISABLE. 00460 * @retval None 00461 */ 00462 void TIM_UpdateDisableConfig(TIM_TypeDef* TIMx, FunctionalState NewState) 00463 { 00464 /* Check the parameters */ 00465 assert_param(IS_TIM_ALL_PERIPH(TIMx)); 00466 assert_param(IS_FUNCTIONAL_STATE(NewState)); 00467 00468 if (NewState != DISABLE) 00469 { 00470 /* Set the Update Disable Bit */ 00471 TIMx->CR1 |= TIM_CR1_UDIS; 00472 } 00473 else 00474 { 00475 /* Reset the Update Disable Bit */ 00476 TIMx->CR1 &= (uint16_t)~TIM_CR1_UDIS; 00477 } 00478 } 00479 00480 /** 00481 * @brief Configures the TIMx Update Request Interrupt source. 00482 * @param TIMx: where x can be 1 to 14 to select the TIM peripheral. 00483 * @param TIM_UpdateSource: specifies the Update source. 00484 * This parameter can be one of the following values: 00485 * @arg TIM_UpdateSource_Global: Source of update is the counter 00486 * overflow/underflow or the setting of UG bit, or an update 00487 * generation through the slave mode controller. 00488 * @arg TIM_UpdateSource_Regular: Source of update is counter overflow/underflow. 00489 * @retval None 00490 */ 00491 void TIM_UpdateRequestConfig(TIM_TypeDef* TIMx, uint16_t TIM_UpdateSource) 00492 { 00493 /* Check the parameters */ 00494 assert_param(IS_TIM_ALL_PERIPH(TIMx)); 00495 assert_param(IS_TIM_UPDATE_SOURCE(TIM_UpdateSource)); 00496 00497 if (TIM_UpdateSource != TIM_UpdateSource_Global) 00498 { 00499 /* Set the URS Bit */ 00500 TIMx->CR1 |= TIM_CR1_URS; 00501 } 00502 else 00503 { 00504 /* Reset the URS Bit */ 00505 TIMx->CR1 &= (uint16_t)~TIM_CR1_URS; 00506 } 00507 } 00508 00509 /** 00510 * @brief Enables or disables TIMx peripheral Preload register on ARR. 00511 * @param TIMx: where x can be 1 to 14 to select the TIM peripheral. 00512 * @param NewState: new state of the TIMx peripheral Preload register 00513 * This parameter can be: ENABLE or DISABLE. 00514 * @retval None 00515 */ 00516 void TIM_ARRPreloadConfig(TIM_TypeDef* TIMx, FunctionalState NewState) 00517 { 00518 /* Check the parameters */ 00519 assert_param(IS_TIM_ALL_PERIPH(TIMx)); 00520 assert_param(IS_FUNCTIONAL_STATE(NewState)); 00521 00522 if (NewState != DISABLE) 00523 { 00524 /* Set the ARR Preload Bit */ 00525 TIMx->CR1 |= TIM_CR1_ARPE; 00526 } 00527 else 00528 { 00529 /* Reset the ARR Preload Bit */ 00530 TIMx->CR1 &= (uint16_t)~TIM_CR1_ARPE; 00531 } 00532 } 00533 00534 /** 00535 * @brief Selects the TIMx's One Pulse Mode. 00536 * @param TIMx: where x can be 1 to 14 to select the TIM peripheral. 00537 * @param TIM_OPMode: specifies the OPM Mode to be used. 00538 * This parameter can be one of the following values: 00539 * @arg TIM_OPMode_Single 00540 * @arg TIM_OPMode_Repetitive 00541 * @retval None 00542 */ 00543 void TIM_SelectOnePulseMode(TIM_TypeDef* TIMx, uint16_t TIM_OPMode) 00544 { 00545 /* Check the parameters */ 00546 assert_param(IS_TIM_ALL_PERIPH(TIMx)); 00547 assert_param(IS_TIM_OPM_MODE(TIM_OPMode)); 00548 00549 /* Reset the OPM Bit */ 00550 TIMx->CR1 &= (uint16_t)~TIM_CR1_OPM; 00551 00552 /* Configure the OPM Mode */ 00553 TIMx->CR1 |= TIM_OPMode; 00554 } 00555 00556 /** 00557 * @brief Sets the TIMx Clock Division value. 00558 * @param TIMx: where x can be 1 to 14 except 6 and 7, to select the TIM peripheral. 00559 * @param TIM_CKD: specifies the clock division value. 00560 * This parameter can be one of the following value: 00561 * @arg TIM_CKD_DIV1: TDTS = Tck_tim 00562 * @arg TIM_CKD_DIV2: TDTS = 2*Tck_tim 00563 * @arg TIM_CKD_DIV4: TDTS = 4*Tck_tim 00564 * @retval None 00565 */ 00566 void TIM_SetClockDivision(TIM_TypeDef* TIMx, uint16_t TIM_CKD) 00567 { 00568 /* Check the parameters */ 00569 assert_param(IS_TIM_LIST1_PERIPH(TIMx)); 00570 assert_param(IS_TIM_CKD_DIV(TIM_CKD)); 00571 00572 /* Reset the CKD Bits */ 00573 TIMx->CR1 &= (uint16_t)(~TIM_CR1_CKD); 00574 00575 /* Set the CKD value */ 00576 TIMx->CR1 |= TIM_CKD; 00577 } 00578 00579 /** 00580 * @brief Enables or disables the specified TIM peripheral. 00581 * @param TIMx: where x can be 1 to 14 to select the TIMx peripheral. 00582 * @param NewState: new state of the TIMx peripheral. 00583 * This parameter can be: ENABLE or DISABLE. 00584 * @retval None 00585 */ 00586 void TIM_Cmd(TIM_TypeDef* TIMx, FunctionalState NewState) 00587 { 00588 /* Check the parameters */ 00589 assert_param(IS_TIM_ALL_PERIPH(TIMx)); 00590 assert_param(IS_FUNCTIONAL_STATE(NewState)); 00591 00592 if (NewState != DISABLE) 00593 { 00594 /* Enable the TIM Counter */ 00595 TIMx->CR1 |= TIM_CR1_CEN; 00596 } 00597 else 00598 { 00599 /* Disable the TIM Counter */ 00600 TIMx->CR1 &= (uint16_t)~TIM_CR1_CEN; 00601 } 00602 } 00603 /** 00604 * @} 00605 */ 00606 00607 /** @defgroup TIM_Group2 Output Compare management functions 00608 * @brief Output Compare management functions 00609 * 00610 @verbatim 00611 =============================================================================== 00612 Output Compare management functions 00613 =============================================================================== 00614 00615 =================================================================== 00616 TIM Driver: how to use it in Output Compare Mode 00617 =================================================================== 00618 To use the Timer in Output Compare mode, the following steps are mandatory: 00619 00620 1. Enable TIM clock using RCC_APBxPeriphClockCmd(RCC_APBxPeriph_TIMx, ENABLE) function 00621 00622 2. Configure the TIM pins by configuring the corresponding GPIO pins 00623 00624 2. Configure the Time base unit as described in the first part of this driver, 00625 if needed, else the Timer will run with the default configuration: 00626 - Autoreload value = 0xFFFF 00627 - Prescaler value = 0x0000 00628 - Counter mode = Up counting 00629 - Clock Division = TIM_CKD_DIV1 00630 00631 3. Fill the TIM_OCInitStruct with the desired parameters including: 00632 - The TIM Output Compare mode: TIM_OCMode 00633 - TIM Output State: TIM_OutputState 00634 - TIM Pulse value: TIM_Pulse 00635 - TIM Output Compare Polarity : TIM_OCPolarity 00636 00637 4. Call TIM_OCxInit(TIMx, &TIM_OCInitStruct) to configure the desired channel with the 00638 corresponding configuration 00639 00640 5. Call the TIM_Cmd(ENABLE) function to enable the TIM counter. 00641 00642 Note1: All other functions can be used separately to modify, if needed, 00643 a specific feature of the Timer. 00644 00645 Note2: In case of PWM mode, this function is mandatory: 00646 TIM_OCxPreloadConfig(TIMx, TIM_OCPreload_ENABLE); 00647 00648 Note3: If the corresponding interrupt or DMA request are needed, the user should: 00649 1. Enable the NVIC (or the DMA) to use the TIM interrupts (or DMA requests). 00650 2. Enable the corresponding interrupt (or DMA request) using the function 00651 TIM_ITConfig(TIMx, TIM_IT_CCx) (or TIM_DMA_Cmd(TIMx, TIM_DMA_CCx)) 00652 00653 @endverbatim 00654 * @{ 00655 */ 00656 00657 /** 00658 * @brief Initializes the TIMx Channel1 according to the specified parameters in 00659 * the TIM_OCInitStruct. 00660 * @param TIMx: where x can be 1 to 14 except 6 and 7, to select the TIM peripheral. 00661 * @param TIM_OCInitStruct: pointer to a TIM_OCInitTypeDef structure that contains 00662 * the configuration information for the specified TIM peripheral. 00663 * @retval None 00664 */ 00665 void TIM_OC1Init(TIM_TypeDef* TIMx, TIM_OCInitTypeDef* TIM_OCInitStruct) 00666 { 00667 uint16_t tmpccmrx = 0, tmpccer = 0, tmpcr2 = 0; 00668 00669 /* Check the parameters */ 00670 assert_param(IS_TIM_LIST1_PERIPH(TIMx)); 00671 assert_param(IS_TIM_OC_MODE(TIM_OCInitStruct->TIM_OCMode)); 00672 assert_param(IS_TIM_OUTPUT_STATE(TIM_OCInitStruct->TIM_OutputState)); 00673 assert_param(IS_TIM_OC_POLARITY(TIM_OCInitStruct->TIM_OCPolarity)); 00674 00675 /* Disable the Channel 1: Reset the CC1E Bit */ 00676 TIMx->CCER &= (uint16_t)~TIM_CCER_CC1E; 00677 00678 /* Get the TIMx CCER register value */ 00679 tmpccer = TIMx->CCER; 00680 /* Get the TIMx CR2 register value */ 00681 tmpcr2 = TIMx->CR2; 00682 00683 /* Get the TIMx CCMR1 register value */ 00684 tmpccmrx = TIMx->CCMR1; 00685 00686 /* Reset the Output Compare Mode Bits */ 00687 tmpccmrx &= (uint16_t)~TIM_CCMR1_OC1M; 00688 tmpccmrx &= (uint16_t)~TIM_CCMR1_CC1S; 00689 /* Select the Output Compare Mode */ 00690 tmpccmrx |= TIM_OCInitStruct->TIM_OCMode; 00691 00692 /* Reset the Output Polarity level */ 00693 tmpccer &= (uint16_t)~TIM_CCER_CC1P; 00694 /* Set the Output Compare Polarity */ 00695 tmpccer |= TIM_OCInitStruct->TIM_OCPolarity; 00696 00697 /* Set the Output State */ 00698 tmpccer |= TIM_OCInitStruct->TIM_OutputState; 00699 00700 if((TIMx == TIM1) || (TIMx == TIM8)) 00701 { 00702 assert_param(IS_TIM_OUTPUTN_STATE(TIM_OCInitStruct->TIM_OutputNState)); 00703 assert_param(IS_TIM_OCN_POLARITY(TIM_OCInitStruct->TIM_OCNPolarity)); 00704 assert_param(IS_TIM_OCNIDLE_STATE(TIM_OCInitStruct->TIM_OCNIdleState)); 00705 assert_param(IS_TIM_OCIDLE_STATE(TIM_OCInitStruct->TIM_OCIdleState)); 00706 00707 /* Reset the Output N Polarity level */ 00708 tmpccer &= (uint16_t)~TIM_CCER_CC1NP; 00709 /* Set the Output N Polarity */ 00710 tmpccer |= TIM_OCInitStruct->TIM_OCNPolarity; 00711 /* Reset the Output N State */ 00712 tmpccer &= (uint16_t)~TIM_CCER_CC1NE; 00713 00714 /* Set the Output N State */ 00715 tmpccer |= TIM_OCInitStruct->TIM_OutputNState; 00716 /* Reset the Output Compare and Output Compare N IDLE State */ 00717 tmpcr2 &= (uint16_t)~TIM_CR2_OIS1; 00718 tmpcr2 &= (uint16_t)~TIM_CR2_OIS1N; 00719 /* Set the Output Idle state */ 00720 tmpcr2 |= TIM_OCInitStruct->TIM_OCIdleState; 00721 /* Set the Output N Idle state */ 00722 tmpcr2 |= TIM_OCInitStruct->TIM_OCNIdleState; 00723 } 00724 /* Write to TIMx CR2 */ 00725 TIMx->CR2 = tmpcr2; 00726 00727 /* Write to TIMx CCMR1 */ 00728 TIMx->CCMR1 = tmpccmrx; 00729 00730 /* Set the Capture Compare Register value */ 00731 TIMx->CCR1 = TIM_OCInitStruct->TIM_Pulse; 00732 00733 /* Write to TIMx CCER */ 00734 TIMx->CCER = tmpccer; 00735 } 00736 00737 /** 00738 * @brief Initializes the TIMx Channel2 according to the specified parameters 00739 * in the TIM_OCInitStruct. 00740 * @param TIMx: where x can be 1, 2, 3, 4, 5, 8, 9 or 12 to select the TIM 00741 * peripheral. 00742 * @param TIM_OCInitStruct: pointer to a TIM_OCInitTypeDef structure that contains 00743 * the configuration information for the specified TIM peripheral. 00744 * @retval None 00745 */ 00746 void TIM_OC2Init(TIM_TypeDef* TIMx, TIM_OCInitTypeDef* TIM_OCInitStruct) 00747 { 00748 uint16_t tmpccmrx = 0, tmpccer = 0, tmpcr2 = 0; 00749 00750 /* Check the parameters */ 00751 assert_param(IS_TIM_LIST2_PERIPH(TIMx)); 00752 assert_param(IS_TIM_OC_MODE(TIM_OCInitStruct->TIM_OCMode)); 00753 assert_param(IS_TIM_OUTPUT_STATE(TIM_OCInitStruct->TIM_OutputState)); 00754 assert_param(IS_TIM_OC_POLARITY(TIM_OCInitStruct->TIM_OCPolarity)); 00755 00756 /* Disable the Channel 2: Reset the CC2E Bit */ 00757 TIMx->CCER &= (uint16_t)~TIM_CCER_CC2E; 00758 00759 /* Get the TIMx CCER register value */ 00760 tmpccer = TIMx->CCER; 00761 /* Get the TIMx CR2 register value */ 00762 tmpcr2 = TIMx->CR2; 00763 00764 /* Get the TIMx CCMR1 register value */ 00765 tmpccmrx = TIMx->CCMR1; 00766 00767 /* Reset the Output Compare mode and Capture/Compare selection Bits */ 00768 tmpccmrx &= (uint16_t)~TIM_CCMR1_OC2M; 00769 tmpccmrx &= (uint16_t)~TIM_CCMR1_CC2S; 00770 00771 /* Select the Output Compare Mode */ 00772 tmpccmrx |= (uint16_t)(TIM_OCInitStruct->TIM_OCMode << 8); 00773 00774 /* Reset the Output Polarity level */ 00775 tmpccer &= (uint16_t)~TIM_CCER_CC2P; 00776 /* Set the Output Compare Polarity */ 00777 tmpccer |= (uint16_t)(TIM_OCInitStruct->TIM_OCPolarity << 4); 00778 00779 /* Set the Output State */ 00780 tmpccer |= (uint16_t)(TIM_OCInitStruct->TIM_OutputState << 4); 00781 00782 if((TIMx == TIM1) || (TIMx == TIM8)) 00783 { 00784 assert_param(IS_TIM_OUTPUTN_STATE(TIM_OCInitStruct->TIM_OutputNState)); 00785 assert_param(IS_TIM_OCN_POLARITY(TIM_OCInitStruct->TIM_OCNPolarity)); 00786 assert_param(IS_TIM_OCNIDLE_STATE(TIM_OCInitStruct->TIM_OCNIdleState)); 00787 assert_param(IS_TIM_OCIDLE_STATE(TIM_OCInitStruct->TIM_OCIdleState)); 00788 00789 /* Reset the Output N Polarity level */ 00790 tmpccer &= (uint16_t)~TIM_CCER_CC2NP; 00791 /* Set the Output N Polarity */ 00792 tmpccer |= (uint16_t)(TIM_OCInitStruct->TIM_OCNPolarity << 4); 00793 /* Reset the Output N State */ 00794 tmpccer &= (uint16_t)~TIM_CCER_CC2NE; 00795 00796 /* Set the Output N State */ 00797 tmpccer |= (uint16_t)(TIM_OCInitStruct->TIM_OutputNState << 4); 00798 /* Reset the Output Compare and Output Compare N IDLE State */ 00799 tmpcr2 &= (uint16_t)~TIM_CR2_OIS2; 00800 tmpcr2 &= (uint16_t)~TIM_CR2_OIS2N; 00801 /* Set the Output Idle state */ 00802 tmpcr2 |= (uint16_t)(TIM_OCInitStruct->TIM_OCIdleState << 2); 00803 /* Set the Output N Idle state */ 00804 tmpcr2 |= (uint16_t)(TIM_OCInitStruct->TIM_OCNIdleState << 2); 00805 } 00806 /* Write to TIMx CR2 */ 00807 TIMx->CR2 = tmpcr2; 00808 00809 /* Write to TIMx CCMR1 */ 00810 TIMx->CCMR1 = tmpccmrx; 00811 00812 /* Set the Capture Compare Register value */ 00813 TIMx->CCR2 = TIM_OCInitStruct->TIM_Pulse; 00814 00815 /* Write to TIMx CCER */ 00816 TIMx->CCER = tmpccer; 00817 } 00818 00819 /** 00820 * @brief Initializes the TIMx Channel3 according to the specified parameters 00821 * in the TIM_OCInitStruct. 00822 * @param TIMx: where x can be 1, 2, 3, 4, 5 or 8 to select the TIM peripheral. 00823 * @param TIM_OCInitStruct: pointer to a TIM_OCInitTypeDef structure that contains 00824 * the configuration information for the specified TIM peripheral. 00825 * @retval None 00826 */ 00827 void TIM_OC3Init(TIM_TypeDef* TIMx, TIM_OCInitTypeDef* TIM_OCInitStruct) 00828 { 00829 uint16_t tmpccmrx = 0, tmpccer = 0, tmpcr2 = 0; 00830 00831 /* Check the parameters */ 00832 assert_param(IS_TIM_LIST3_PERIPH(TIMx)); 00833 assert_param(IS_TIM_OC_MODE(TIM_OCInitStruct->TIM_OCMode)); 00834 assert_param(IS_TIM_OUTPUT_STATE(TIM_OCInitStruct->TIM_OutputState)); 00835 assert_param(IS_TIM_OC_POLARITY(TIM_OCInitStruct->TIM_OCPolarity)); 00836 00837 /* Disable the Channel 3: Reset the CC2E Bit */ 00838 TIMx->CCER &= (uint16_t)~TIM_CCER_CC3E; 00839 00840 /* Get the TIMx CCER register value */ 00841 tmpccer = TIMx->CCER; 00842 /* Get the TIMx CR2 register value */ 00843 tmpcr2 = TIMx->CR2; 00844 00845 /* Get the TIMx CCMR2 register value */ 00846 tmpccmrx = TIMx->CCMR2; 00847 00848 /* Reset the Output Compare mode and Capture/Compare selection Bits */ 00849 tmpccmrx &= (uint16_t)~TIM_CCMR2_OC3M; 00850 tmpccmrx &= (uint16_t)~TIM_CCMR2_CC3S; 00851 /* Select the Output Compare Mode */ 00852 tmpccmrx |= TIM_OCInitStruct->TIM_OCMode; 00853 00854 /* Reset the Output Polarity level */ 00855 tmpccer &= (uint16_t)~TIM_CCER_CC3P; 00856 /* Set the Output Compare Polarity */ 00857 tmpccer |= (uint16_t)(TIM_OCInitStruct->TIM_OCPolarity << 8); 00858 00859 /* Set the Output State */ 00860 tmpccer |= (uint16_t)(TIM_OCInitStruct->TIM_OutputState << 8); 00861 00862 if((TIMx == TIM1) || (TIMx == TIM8)) 00863 { 00864 assert_param(IS_TIM_OUTPUTN_STATE(TIM_OCInitStruct->TIM_OutputNState)); 00865 assert_param(IS_TIM_OCN_POLARITY(TIM_OCInitStruct->TIM_OCNPolarity)); 00866 assert_param(IS_TIM_OCNIDLE_STATE(TIM_OCInitStruct->TIM_OCNIdleState)); 00867 assert_param(IS_TIM_OCIDLE_STATE(TIM_OCInitStruct->TIM_OCIdleState)); 00868 00869 /* Reset the Output N Polarity level */ 00870 tmpccer &= (uint16_t)~TIM_CCER_CC3NP; 00871 /* Set the Output N Polarity */ 00872 tmpccer |= (uint16_t)(TIM_OCInitStruct->TIM_OCNPolarity << 8); 00873 /* Reset the Output N State */ 00874 tmpccer &= (uint16_t)~TIM_CCER_CC3NE; 00875 00876 /* Set the Output N State */ 00877 tmpccer |= (uint16_t)(TIM_OCInitStruct->TIM_OutputNState << 8); 00878 /* Reset the Output Compare and Output Compare N IDLE State */ 00879 tmpcr2 &= (uint16_t)~TIM_CR2_OIS3; 00880 tmpcr2 &= (uint16_t)~TIM_CR2_OIS3N; 00881 /* Set the Output Idle state */ 00882 tmpcr2 |= (uint16_t)(TIM_OCInitStruct->TIM_OCIdleState << 4); 00883 /* Set the Output N Idle state */ 00884 tmpcr2 |= (uint16_t)(TIM_OCInitStruct->TIM_OCNIdleState << 4); 00885 } 00886 /* Write to TIMx CR2 */ 00887 TIMx->CR2 = tmpcr2; 00888 00889 /* Write to TIMx CCMR2 */ 00890 TIMx->CCMR2 = tmpccmrx; 00891 00892 /* Set the Capture Compare Register value */ 00893 TIMx->CCR3 = TIM_OCInitStruct->TIM_Pulse; 00894 00895 /* Write to TIMx CCER */ 00896 TIMx->CCER = tmpccer; 00897 } 00898 00899 /** 00900 * @brief Initializes the TIMx Channel4 according to the specified parameters 00901 * in the TIM_OCInitStruct. 00902 * @param TIMx: where x can be 1, 2, 3, 4, 5 or 8 to select the TIM peripheral. 00903 * @param TIM_OCInitStruct: pointer to a TIM_OCInitTypeDef structure that contains 00904 * the configuration information for the specified TIM peripheral. 00905 * @retval None 00906 */ 00907 void TIM_OC4Init(TIM_TypeDef* TIMx, TIM_OCInitTypeDef* TIM_OCInitStruct) 00908 { 00909 uint16_t tmpccmrx = 0, tmpccer = 0, tmpcr2 = 0; 00910 00911 /* Check the parameters */ 00912 assert_param(IS_TIM_LIST3_PERIPH(TIMx)); 00913 assert_param(IS_TIM_OC_MODE(TIM_OCInitStruct->TIM_OCMode)); 00914 assert_param(IS_TIM_OUTPUT_STATE(TIM_OCInitStruct->TIM_OutputState)); 00915 assert_param(IS_TIM_OC_POLARITY(TIM_OCInitStruct->TIM_OCPolarity)); 00916 00917 /* Disable the Channel 4: Reset the CC4E Bit */ 00918 TIMx->CCER &= (uint16_t)~TIM_CCER_CC4E; 00919 00920 /* Get the TIMx CCER register value */ 00921 tmpccer = TIMx->CCER; 00922 /* Get the TIMx CR2 register value */ 00923 tmpcr2 = TIMx->CR2; 00924 00925 /* Get the TIMx CCMR2 register value */ 00926 tmpccmrx = TIMx->CCMR2; 00927 00928 /* Reset the Output Compare mode and Capture/Compare selection Bits */ 00929 tmpccmrx &= (uint16_t)~TIM_CCMR2_OC4M; 00930 tmpccmrx &= (uint16_t)~TIM_CCMR2_CC4S; 00931 00932 /* Select the Output Compare Mode */ 00933 tmpccmrx |= (uint16_t)(TIM_OCInitStruct->TIM_OCMode << 8); 00934 00935 /* Reset the Output Polarity level */ 00936 tmpccer &= (uint16_t)~TIM_CCER_CC4P; 00937 /* Set the Output Compare Polarity */ 00938 tmpccer |= (uint16_t)(TIM_OCInitStruct->TIM_OCPolarity << 12); 00939 00940 /* Set the Output State */ 00941 tmpccer |= (uint16_t)(TIM_OCInitStruct->TIM_OutputState << 12); 00942 00943 if((TIMx == TIM1) || (TIMx == TIM8)) 00944 { 00945 assert_param(IS_TIM_OCIDLE_STATE(TIM_OCInitStruct->TIM_OCIdleState)); 00946 /* Reset the Output Compare IDLE State */ 00947 tmpcr2 &=(uint16_t) ~TIM_CR2_OIS4; 00948 /* Set the Output Idle state */ 00949 tmpcr2 |= (uint16_t)(TIM_OCInitStruct->TIM_OCIdleState << 6); 00950 } 00951 /* Write to TIMx CR2 */ 00952 TIMx->CR2 = tmpcr2; 00953 00954 /* Write to TIMx CCMR2 */ 00955 TIMx->CCMR2 = tmpccmrx; 00956 00957 /* Set the Capture Compare Register value */ 00958 TIMx->CCR4 = TIM_OCInitStruct->TIM_Pulse; 00959 00960 /* Write to TIMx CCER */ 00961 TIMx->CCER = tmpccer; 00962 } 00963 00964 /** 00965 * @brief Fills each TIM_OCInitStruct member with its default value. 00966 * @param TIM_OCInitStruct: pointer to a TIM_OCInitTypeDef structure which will 00967 * be initialized. 00968 * @retval None 00969 */ 00970 void TIM_OCStructInit(TIM_OCInitTypeDef* TIM_OCInitStruct) 00971 { 00972 /* Set the default configuration */ 00973 TIM_OCInitStruct->TIM_OCMode = TIM_OCMode_Timing; 00974 TIM_OCInitStruct->TIM_OutputState = TIM_OutputState_Disable; 00975 TIM_OCInitStruct->TIM_OutputNState = TIM_OutputNState_Disable; 00976 TIM_OCInitStruct->TIM_Pulse = 0x00000000; 00977 TIM_OCInitStruct->TIM_OCPolarity = TIM_OCPolarity_High; 00978 TIM_OCInitStruct->TIM_OCNPolarity = TIM_OCPolarity_High; 00979 TIM_OCInitStruct->TIM_OCIdleState = TIM_OCIdleState_Reset; 00980 TIM_OCInitStruct->TIM_OCNIdleState = TIM_OCNIdleState_Reset; 00981 } 00982 00983 /** 00984 * @brief Selects the TIM Output Compare Mode. 00985 * @note This function disables the selected channel before changing the Output 00986 * Compare Mode. If needed, user has to enable this channel using 00987 * TIM_CCxCmd() and TIM_CCxNCmd() functions. 00988 * @param TIMx: where x can be 1 to 14 except 6 and 7, to select the TIM peripheral. 00989 * @param TIM_Channel: specifies the TIM Channel 00990 * This parameter can be one of the following values: 00991 * @arg TIM_Channel_1: TIM Channel 1 00992 * @arg TIM_Channel_2: TIM Channel 2 00993 * @arg TIM_Channel_3: TIM Channel 3 00994 * @arg TIM_Channel_4: TIM Channel 4 00995 * @param TIM_OCMode: specifies the TIM Output Compare Mode. 00996 * This parameter can be one of the following values: 00997 * @arg TIM_OCMode_Timing 00998 * @arg TIM_OCMode_Active 00999 * @arg TIM_OCMode_Toggle 01000 * @arg TIM_OCMode_PWM1 01001 * @arg TIM_OCMode_PWM2 01002 * @arg TIM_ForcedAction_Active 01003 * @arg TIM_ForcedAction_InActive 01004 * @retval None 01005 */ 01006 void TIM_SelectOCxM(TIM_TypeDef* TIMx, uint16_t TIM_Channel, uint16_t TIM_OCMode) 01007 { 01008 uint32_t tmp = 0; 01009 uint16_t tmp1 = 0; 01010 01011 /* Check the parameters */ 01012 assert_param(IS_TIM_LIST1_PERIPH(TIMx)); 01013 assert_param(IS_TIM_CHANNEL(TIM_Channel)); 01014 assert_param(IS_TIM_OCM(TIM_OCMode)); 01015 01016 tmp = (uint32_t) TIMx; 01017 tmp += CCMR_OFFSET; 01018 01019 tmp1 = CCER_CCE_SET << (uint16_t)TIM_Channel; 01020 01021 /* Disable the Channel: Reset the CCxE Bit */ 01022 TIMx->CCER &= (uint16_t) ~tmp1; 01023 01024 if((TIM_Channel == TIM_Channel_1) ||(TIM_Channel == TIM_Channel_3)) 01025 { 01026 tmp += (TIM_Channel>>1); 01027 01028 /* Reset the OCxM bits in the CCMRx register */ 01029 *(__IO uint32_t *) tmp &= CCMR_OC13M_MASK; 01030 01031 /* Configure the OCxM bits in the CCMRx register */ 01032 *(__IO uint32_t *) tmp |= TIM_OCMode; 01033 } 01034 else 01035 { 01036 tmp += (uint16_t)(TIM_Channel - (uint16_t)4)>> (uint16_t)1; 01037 01038 /* Reset the OCxM bits in the CCMRx register */ 01039 *(__IO uint32_t *) tmp &= CCMR_OC24M_MASK; 01040 01041 /* Configure the OCxM bits in the CCMRx register */ 01042 *(__IO uint32_t *) tmp |= (uint16_t)(TIM_OCMode << 8); 01043 } 01044 } 01045 01046 /** 01047 * @brief Sets the TIMx Capture Compare1 Register value 01048 * @param TIMx: where x can be 1 to 14 except 6 and 7, to select the TIM peripheral. 01049 * @param Compare1: specifies the Capture Compare1 register new value. 01050 * @retval None 01051 */ 01052 void TIM_SetCompare1(TIM_TypeDef* TIMx, uint32_t Compare1) 01053 { 01054 /* Check the parameters */ 01055 assert_param(IS_TIM_LIST1_PERIPH(TIMx)); 01056 01057 /* Set the Capture Compare1 Register value */ 01058 TIMx->CCR1 = Compare1; 01059 } 01060 01061 /** 01062 * @brief Sets the TIMx Capture Compare2 Register value 01063 * @param TIMx: where x can be 1, 2, 3, 4, 5, 8, 9 or 12 to select the TIM 01064 * peripheral. 01065 * @param Compare2: specifies the Capture Compare2 register new value. 01066 * @retval None 01067 */ 01068 void TIM_SetCompare2(TIM_TypeDef* TIMx, uint32_t Compare2) 01069 { 01070 /* Check the parameters */ 01071 assert_param(IS_TIM_LIST2_PERIPH(TIMx)); 01072 01073 /* Set the Capture Compare2 Register value */ 01074 TIMx->CCR2 = Compare2; 01075 } 01076 01077 /** 01078 * @brief Sets the TIMx Capture Compare3 Register value 01079 * @param TIMx: where x can be 1, 2, 3, 4, 5 or 8 to select the TIM peripheral. 01080 * @param Compare3: specifies the Capture Compare3 register new value. 01081 * @retval None 01082 */ 01083 void TIM_SetCompare3(TIM_TypeDef* TIMx, uint32_t Compare3) 01084 { 01085 /* Check the parameters */ 01086 assert_param(IS_TIM_LIST3_PERIPH(TIMx)); 01087 01088 /* Set the Capture Compare3 Register value */ 01089 TIMx->CCR3 = Compare3; 01090 } 01091 01092 /** 01093 * @brief Sets the TIMx Capture Compare4 Register value 01094 * @param TIMx: where x can be 1, 2, 3, 4, 5 or 8 to select the TIM peripheral. 01095 * @param Compare4: specifies the Capture Compare4 register new value. 01096 * @retval None 01097 */ 01098 void TIM_SetCompare4(TIM_TypeDef* TIMx, uint32_t Compare4) 01099 { 01100 /* Check the parameters */ 01101 assert_param(IS_TIM_LIST3_PERIPH(TIMx)); 01102 01103 /* Set the Capture Compare4 Register value */ 01104 TIMx->CCR4 = Compare4; 01105 } 01106 01107 /** 01108 * @brief Forces the TIMx output 1 waveform to active or inactive level. 01109 * @param TIMx: where x can be 1 to 14 except 6 and 7, to select the TIM peripheral. 01110 * @param TIM_ForcedAction: specifies the forced Action to be set to the output waveform. 01111 * This parameter can be one of the following values: 01112 * @arg TIM_ForcedAction_Active: Force active level on OC1REF 01113 * @arg TIM_ForcedAction_InActive: Force inactive level on OC1REF. 01114 * @retval None 01115 */ 01116 void TIM_ForcedOC1Config(TIM_TypeDef* TIMx, uint16_t TIM_ForcedAction) 01117 { 01118 uint16_t tmpccmr1 = 0; 01119 01120 /* Check the parameters */ 01121 assert_param(IS_TIM_LIST1_PERIPH(TIMx)); 01122 assert_param(IS_TIM_FORCED_ACTION(TIM_ForcedAction)); 01123 tmpccmr1 = TIMx->CCMR1; 01124 01125 /* Reset the OC1M Bits */ 01126 tmpccmr1 &= (uint16_t)~TIM_CCMR1_OC1M; 01127 01128 /* Configure The Forced output Mode */ 01129 tmpccmr1 |= TIM_ForcedAction; 01130 01131 /* Write to TIMx CCMR1 register */ 01132 TIMx->CCMR1 = tmpccmr1; 01133 } 01134 01135 /** 01136 * @brief Forces the TIMx output 2 waveform to active or inactive level. 01137 * @param TIMx: where x can be 1, 2, 3, 4, 5, 8, 9 or 12 to select the TIM 01138 * peripheral. 01139 * @param TIM_ForcedAction: specifies the forced Action to be set to the output waveform. 01140 * This parameter can be one of the following values: 01141 * @arg TIM_ForcedAction_Active: Force active level on OC2REF 01142 * @arg TIM_ForcedAction_InActive: Force inactive level on OC2REF. 01143 * @retval None 01144 */ 01145 void TIM_ForcedOC2Config(TIM_TypeDef* TIMx, uint16_t TIM_ForcedAction) 01146 { 01147 uint16_t tmpccmr1 = 0; 01148 01149 /* Check the parameters */ 01150 assert_param(IS_TIM_LIST2_PERIPH(TIMx)); 01151 assert_param(IS_TIM_FORCED_ACTION(TIM_ForcedAction)); 01152 tmpccmr1 = TIMx->CCMR1; 01153 01154 /* Reset the OC2M Bits */ 01155 tmpccmr1 &= (uint16_t)~TIM_CCMR1_OC2M; 01156 01157 /* Configure The Forced output Mode */ 01158 tmpccmr1 |= (uint16_t)(TIM_ForcedAction << 8); 01159 01160 /* Write to TIMx CCMR1 register */ 01161 TIMx->CCMR1 = tmpccmr1; 01162 } 01163 01164 /** 01165 * @brief Forces the TIMx output 3 waveform to active or inactive level. 01166 * @param TIMx: where x can be 1, 2, 3, 4, 5 or 8 to select the TIM peripheral. 01167 * @param TIM_ForcedAction: specifies the forced Action to be set to the output waveform. 01168 * This parameter can be one of the following values: 01169 * @arg TIM_ForcedAction_Active: Force active level on OC3REF 01170 * @arg TIM_ForcedAction_InActive: Force inactive level on OC3REF. 01171 * @retval None 01172 */ 01173 void TIM_ForcedOC3Config(TIM_TypeDef* TIMx, uint16_t TIM_ForcedAction) 01174 { 01175 uint16_t tmpccmr2 = 0; 01176 01177 /* Check the parameters */ 01178 assert_param(IS_TIM_LIST3_PERIPH(TIMx)); 01179 assert_param(IS_TIM_FORCED_ACTION(TIM_ForcedAction)); 01180 01181 tmpccmr2 = TIMx->CCMR2; 01182 01183 /* Reset the OC1M Bits */ 01184 tmpccmr2 &= (uint16_t)~TIM_CCMR2_OC3M; 01185 01186 /* Configure The Forced output Mode */ 01187 tmpccmr2 |= TIM_ForcedAction; 01188 01189 /* Write to TIMx CCMR2 register */ 01190 TIMx->CCMR2 = tmpccmr2; 01191 } 01192 01193 /** 01194 * @brief Forces the TIMx output 4 waveform to active or inactive level. 01195 * @param TIMx: where x can be 1, 2, 3, 4, 5 or 8 to select the TIM peripheral. 01196 * @param TIM_ForcedAction: specifies the forced Action to be set to the output waveform. 01197 * This parameter can be one of the following values: 01198 * @arg TIM_ForcedAction_Active: Force active level on OC4REF 01199 * @arg TIM_ForcedAction_InActive: Force inactive level on OC4REF. 01200 * @retval None 01201 */ 01202 void TIM_ForcedOC4Config(TIM_TypeDef* TIMx, uint16_t TIM_ForcedAction) 01203 { 01204 uint16_t tmpccmr2 = 0; 01205 01206 /* Check the parameters */ 01207 assert_param(IS_TIM_LIST3_PERIPH(TIMx)); 01208 assert_param(IS_TIM_FORCED_ACTION(TIM_ForcedAction)); 01209 tmpccmr2 = TIMx->CCMR2; 01210 01211 /* Reset the OC2M Bits */ 01212 tmpccmr2 &= (uint16_t)~TIM_CCMR2_OC4M; 01213 01214 /* Configure The Forced output Mode */ 01215 tmpccmr2 |= (uint16_t)(TIM_ForcedAction << 8); 01216 01217 /* Write to TIMx CCMR2 register */ 01218 TIMx->CCMR2 = tmpccmr2; 01219 } 01220 01221 /** 01222 * @brief Enables or disables the TIMx peripheral Preload register on CCR1. 01223 * @param TIMx: where x can be 1 to 14 except 6 and 7, to select the TIM peripheral. 01224 * @param TIM_OCPreload: new state of the TIMx peripheral Preload register 01225 * This parameter can be one of the following values: 01226 * @arg TIM_OCPreload_Enable 01227 * @arg TIM_OCPreload_Disable 01228 * @retval None 01229 */ 01230 void TIM_OC1PreloadConfig(TIM_TypeDef* TIMx, uint16_t TIM_OCPreload) 01231 { 01232 uint16_t tmpccmr1 = 0; 01233 01234 /* Check the parameters */ 01235 assert_param(IS_TIM_LIST1_PERIPH(TIMx)); 01236 assert_param(IS_TIM_OCPRELOAD_STATE(TIM_OCPreload)); 01237 01238 tmpccmr1 = TIMx->CCMR1; 01239 01240 /* Reset the OC1PE Bit */ 01241 tmpccmr1 &= (uint16_t)(~TIM_CCMR1_OC1PE); 01242 01243 /* Enable or Disable the Output Compare Preload feature */ 01244 tmpccmr1 |= TIM_OCPreload; 01245 01246 /* Write to TIMx CCMR1 register */ 01247 TIMx->CCMR1 = tmpccmr1; 01248 } 01249 01250 /** 01251 * @brief Enables or disables the TIMx peripheral Preload register on CCR2. 01252 * @param TIMx: where x can be 1, 2, 3, 4, 5, 8, 9 or 12 to select the TIM 01253 * peripheral. 01254 * @param TIM_OCPreload: new state of the TIMx peripheral Preload register 01255 * This parameter can be one of the following values: 01256 * @arg TIM_OCPreload_Enable 01257 * @arg TIM_OCPreload_Disable 01258 * @retval None 01259 */ 01260 void TIM_OC2PreloadConfig(TIM_TypeDef* TIMx, uint16_t TIM_OCPreload) 01261 { 01262 uint16_t tmpccmr1 = 0; 01263 01264 /* Check the parameters */ 01265 assert_param(IS_TIM_LIST2_PERIPH(TIMx)); 01266 assert_param(IS_TIM_OCPRELOAD_STATE(TIM_OCPreload)); 01267 01268 tmpccmr1 = TIMx->CCMR1; 01269 01270 /* Reset the OC2PE Bit */ 01271 tmpccmr1 &= (uint16_t)(~TIM_CCMR1_OC2PE); 01272 01273 /* Enable or Disable the Output Compare Preload feature */ 01274 tmpccmr1 |= (uint16_t)(TIM_OCPreload << 8); 01275 01276 /* Write to TIMx CCMR1 register */ 01277 TIMx->CCMR1 = tmpccmr1; 01278 } 01279 01280 /** 01281 * @brief Enables or disables the TIMx peripheral Preload register on CCR3. 01282 * @param TIMx: where x can be 1, 2, 3, 4, 5 or 8 to select the TIM peripheral. 01283 * @param TIM_OCPreload: new state of the TIMx peripheral Preload register 01284 * This parameter can be one of the following values: 01285 * @arg TIM_OCPreload_Enable 01286 * @arg TIM_OCPreload_Disable 01287 * @retval None 01288 */ 01289 void TIM_OC3PreloadConfig(TIM_TypeDef* TIMx, uint16_t TIM_OCPreload) 01290 { 01291 uint16_t tmpccmr2 = 0; 01292 01293 /* Check the parameters */ 01294 assert_param(IS_TIM_LIST3_PERIPH(TIMx)); 01295 assert_param(IS_TIM_OCPRELOAD_STATE(TIM_OCPreload)); 01296 01297 tmpccmr2 = TIMx->CCMR2; 01298 01299 /* Reset the OC3PE Bit */ 01300 tmpccmr2 &= (uint16_t)(~TIM_CCMR2_OC3PE); 01301 01302 /* Enable or Disable the Output Compare Preload feature */ 01303 tmpccmr2 |= TIM_OCPreload; 01304 01305 /* Write to TIMx CCMR2 register */ 01306 TIMx->CCMR2 = tmpccmr2; 01307 } 01308 01309 /** 01310 * @brief Enables or disables the TIMx peripheral Preload register on CCR4. 01311 * @param TIMx: where x can be 1, 2, 3, 4, 5 or 8 to select the TIM peripheral. 01312 * @param TIM_OCPreload: new state of the TIMx peripheral Preload register 01313 * This parameter can be one of the following values: 01314 * @arg TIM_OCPreload_Enable 01315 * @arg TIM_OCPreload_Disable 01316 * @retval None 01317 */ 01318 void TIM_OC4PreloadConfig(TIM_TypeDef* TIMx, uint16_t TIM_OCPreload) 01319 { 01320 uint16_t tmpccmr2 = 0; 01321 01322 /* Check the parameters */ 01323 assert_param(IS_TIM_LIST3_PERIPH(TIMx)); 01324 assert_param(IS_TIM_OCPRELOAD_STATE(TIM_OCPreload)); 01325 01326 tmpccmr2 = TIMx->CCMR2; 01327 01328 /* Reset the OC4PE Bit */ 01329 tmpccmr2 &= (uint16_t)(~TIM_CCMR2_OC4PE); 01330 01331 /* Enable or Disable the Output Compare Preload feature */ 01332 tmpccmr2 |= (uint16_t)(TIM_OCPreload << 8); 01333 01334 /* Write to TIMx CCMR2 register */ 01335 TIMx->CCMR2 = tmpccmr2; 01336 } 01337 01338 /** 01339 * @brief Configures the TIMx Output Compare 1 Fast feature. 01340 * @param TIMx: where x can be 1 to 14 except 6 and 7, to select the TIM peripheral. 01341 * @param TIM_OCFast: new state of the Output Compare Fast Enable Bit. 01342 * This parameter can be one of the following values: 01343 * @arg TIM_OCFast_Enable: TIM output compare fast enable 01344 * @arg TIM_OCFast_Disable: TIM output compare fast disable 01345 * @retval None 01346 */ 01347 void TIM_OC1FastConfig(TIM_TypeDef* TIMx, uint16_t TIM_OCFast) 01348 { 01349 uint16_t tmpccmr1 = 0; 01350 01351 /* Check the parameters */ 01352 assert_param(IS_TIM_LIST1_PERIPH(TIMx)); 01353 assert_param(IS_TIM_OCFAST_STATE(TIM_OCFast)); 01354 01355 /* Get the TIMx CCMR1 register value */ 01356 tmpccmr1 = TIMx->CCMR1; 01357 01358 /* Reset the OC1FE Bit */ 01359 tmpccmr1 &= (uint16_t)~TIM_CCMR1_OC1FE; 01360 01361 /* Enable or Disable the Output Compare Fast Bit */ 01362 tmpccmr1 |= TIM_OCFast; 01363 01364 /* Write to TIMx CCMR1 */ 01365 TIMx->CCMR1 = tmpccmr1; 01366 } 01367 01368 /** 01369 * @brief Configures the TIMx Output Compare 2 Fast feature. 01370 * @param TIMx: where x can be 1, 2, 3, 4, 5, 8, 9 or 12 to select the TIM 01371 * peripheral. 01372 * @param TIM_OCFast: new state of the Output Compare Fast Enable Bit. 01373 * This parameter can be one of the following values: 01374 * @arg TIM_OCFast_Enable: TIM output compare fast enable 01375 * @arg TIM_OCFast_Disable: TIM output compare fast disable 01376 * @retval None 01377 */ 01378 void TIM_OC2FastConfig(TIM_TypeDef* TIMx, uint16_t TIM_OCFast) 01379 { 01380 uint16_t tmpccmr1 = 0; 01381 01382 /* Check the parameters */ 01383 assert_param(IS_TIM_LIST2_PERIPH(TIMx)); 01384 assert_param(IS_TIM_OCFAST_STATE(TIM_OCFast)); 01385 01386 /* Get the TIMx CCMR1 register value */ 01387 tmpccmr1 = TIMx->CCMR1; 01388 01389 /* Reset the OC2FE Bit */ 01390 tmpccmr1 &= (uint16_t)(~TIM_CCMR1_OC2FE); 01391 01392 /* Enable or Disable the Output Compare Fast Bit */ 01393 tmpccmr1 |= (uint16_t)(TIM_OCFast << 8); 01394 01395 /* Write to TIMx CCMR1 */ 01396 TIMx->CCMR1 = tmpccmr1; 01397 } 01398 01399 /** 01400 * @brief Configures the TIMx Output Compare 3 Fast feature. 01401 * @param TIMx: where x can be 1, 2, 3, 4, 5 or 8 to select the TIM peripheral. 01402 * @param TIM_OCFast: new state of the Output Compare Fast Enable Bit. 01403 * This parameter can be one of the following values: 01404 * @arg TIM_OCFast_Enable: TIM output compare fast enable 01405 * @arg TIM_OCFast_Disable: TIM output compare fast disable 01406 * @retval None 01407 */ 01408 void TIM_OC3FastConfig(TIM_TypeDef* TIMx, uint16_t TIM_OCFast) 01409 { 01410 uint16_t tmpccmr2 = 0; 01411 01412 /* Check the parameters */ 01413 assert_param(IS_TIM_LIST3_PERIPH(TIMx)); 01414 assert_param(IS_TIM_OCFAST_STATE(TIM_OCFast)); 01415 01416 /* Get the TIMx CCMR2 register value */ 01417 tmpccmr2 = TIMx->CCMR2; 01418 01419 /* Reset the OC3FE Bit */ 01420 tmpccmr2 &= (uint16_t)~TIM_CCMR2_OC3FE; 01421 01422 /* Enable or Disable the Output Compare Fast Bit */ 01423 tmpccmr2 |= TIM_OCFast; 01424 01425 /* Write to TIMx CCMR2 */ 01426 TIMx->CCMR2 = tmpccmr2; 01427 } 01428 01429 /** 01430 * @brief Configures the TIMx Output Compare 4 Fast feature. 01431 * @param TIMx: where x can be 1, 2, 3, 4, 5 or 8 to select the TIM peripheral. 01432 * @param TIM_OCFast: new state of the Output Compare Fast Enable Bit. 01433 * This parameter can be one of the following values: 01434 * @arg TIM_OCFast_Enable: TIM output compare fast enable 01435 * @arg TIM_OCFast_Disable: TIM output compare fast disable 01436 * @retval None 01437 */ 01438 void TIM_OC4FastConfig(TIM_TypeDef* TIMx, uint16_t TIM_OCFast) 01439 { 01440 uint16_t tmpccmr2 = 0; 01441 01442 /* Check the parameters */ 01443 assert_param(IS_TIM_LIST3_PERIPH(TIMx)); 01444 assert_param(IS_TIM_OCFAST_STATE(TIM_OCFast)); 01445 01446 /* Get the TIMx CCMR2 register value */ 01447 tmpccmr2 = TIMx->CCMR2; 01448 01449 /* Reset the OC4FE Bit */ 01450 tmpccmr2 &= (uint16_t)(~TIM_CCMR2_OC4FE); 01451 01452 /* Enable or Disable the Output Compare Fast Bit */ 01453 tmpccmr2 |= (uint16_t)(TIM_OCFast << 8); 01454 01455 /* Write to TIMx CCMR2 */ 01456 TIMx->CCMR2 = tmpccmr2; 01457 } 01458 01459 /** 01460 * @brief Clears or safeguards the OCREF1 signal on an external event 01461 * @param TIMx: where x can be 1 to 14 except 6 and 7, to select the TIM peripheral. 01462 * @param TIM_OCClear: new state of the Output Compare Clear Enable Bit. 01463 * This parameter can be one of the following values: 01464 * @arg TIM_OCClear_Enable: TIM Output clear enable 01465 * @arg TIM_OCClear_Disable: TIM Output clear disable 01466 * @retval None 01467 */ 01468 void TIM_ClearOC1Ref(TIM_TypeDef* TIMx, uint16_t TIM_OCClear) 01469 { 01470 uint16_t tmpccmr1 = 0; 01471 01472 /* Check the parameters */ 01473 assert_param(IS_TIM_LIST1_PERIPH(TIMx)); 01474 assert_param(IS_TIM_OCCLEAR_STATE(TIM_OCClear)); 01475 01476 tmpccmr1 = TIMx->CCMR1; 01477 01478 /* Reset the OC1CE Bit */ 01479 tmpccmr1 &= (uint16_t)~TIM_CCMR1_OC1CE; 01480 01481 /* Enable or Disable the Output Compare Clear Bit */ 01482 tmpccmr1 |= TIM_OCClear; 01483 01484 /* Write to TIMx CCMR1 register */ 01485 TIMx->CCMR1 = tmpccmr1; 01486 } 01487 01488 /** 01489 * @brief Clears or safeguards the OCREF2 signal on an external event 01490 * @param TIMx: where x can be 1, 2, 3, 4, 5, 8, 9 or 12 to select the TIM 01491 * peripheral. 01492 * @param TIM_OCClear: new state of the Output Compare Clear Enable Bit. 01493 * This parameter can be one of the following values: 01494 * @arg TIM_OCClear_Enable: TIM Output clear enable 01495 * @arg TIM_OCClear_Disable: TIM Output clear disable 01496 * @retval None 01497 */ 01498 void TIM_ClearOC2Ref(TIM_TypeDef* TIMx, uint16_t TIM_OCClear) 01499 { 01500 uint16_t tmpccmr1 = 0; 01501 01502 /* Check the parameters */ 01503 assert_param(IS_TIM_LIST2_PERIPH(TIMx)); 01504 assert_param(IS_TIM_OCCLEAR_STATE(TIM_OCClear)); 01505 01506 tmpccmr1 = TIMx->CCMR1; 01507 01508 /* Reset the OC2CE Bit */ 01509 tmpccmr1 &= (uint16_t)~TIM_CCMR1_OC2CE; 01510 01511 /* Enable or Disable the Output Compare Clear Bit */ 01512 tmpccmr1 |= (uint16_t)(TIM_OCClear << 8); 01513 01514 /* Write to TIMx CCMR1 register */ 01515 TIMx->CCMR1 = tmpccmr1; 01516 } 01517 01518 /** 01519 * @brief Clears or safeguards the OCREF3 signal on an external event 01520 * @param TIMx: where x can be 1, 2, 3, 4, 5 or 8 to select the TIM peripheral. 01521 * @param TIM_OCClear: new state of the Output Compare Clear Enable Bit. 01522 * This parameter can be one of the following values: 01523 * @arg TIM_OCClear_Enable: TIM Output clear enable 01524 * @arg TIM_OCClear_Disable: TIM Output clear disable 01525 * @retval None 01526 */ 01527 void TIM_ClearOC3Ref(TIM_TypeDef* TIMx, uint16_t TIM_OCClear) 01528 { 01529 uint16_t tmpccmr2 = 0; 01530 01531 /* Check the parameters */ 01532 assert_param(IS_TIM_LIST3_PERIPH(TIMx)); 01533 assert_param(IS_TIM_OCCLEAR_STATE(TIM_OCClear)); 01534 01535 tmpccmr2 = TIMx->CCMR2; 01536 01537 /* Reset the OC3CE Bit */ 01538 tmpccmr2 &= (uint16_t)~TIM_CCMR2_OC3CE; 01539 01540 /* Enable or Disable the Output Compare Clear Bit */ 01541 tmpccmr2 |= TIM_OCClear; 01542 01543 /* Write to TIMx CCMR2 register */ 01544 TIMx->CCMR2 = tmpccmr2; 01545 } 01546 01547 /** 01548 * @brief Clears or safeguards the OCREF4 signal on an external event 01549 * @param TIMx: where x can be 1, 2, 3, 4, 5 or 8 to select the TIM peripheral. 01550 * @param TIM_OCClear: new state of the Output Compare Clear Enable Bit. 01551 * This parameter can be one of the following values: 01552 * @arg TIM_OCClear_Enable: TIM Output clear enable 01553 * @arg TIM_OCClear_Disable: TIM Output clear disable 01554 * @retval None 01555 */ 01556 void TIM_ClearOC4Ref(TIM_TypeDef* TIMx, uint16_t TIM_OCClear) 01557 { 01558 uint16_t tmpccmr2 = 0; 01559 01560 /* Check the parameters */ 01561 assert_param(IS_TIM_LIST3_PERIPH(TIMx)); 01562 assert_param(IS_TIM_OCCLEAR_STATE(TIM_OCClear)); 01563 01564 tmpccmr2 = TIMx->CCMR2; 01565 01566 /* Reset the OC4CE Bit */ 01567 tmpccmr2 &= (uint16_t)~TIM_CCMR2_OC4CE; 01568 01569 /* Enable or Disable the Output Compare Clear Bit */ 01570 tmpccmr2 |= (uint16_t)(TIM_OCClear << 8); 01571 01572 /* Write to TIMx CCMR2 register */ 01573 TIMx->CCMR2 = tmpccmr2; 01574 } 01575 01576 /** 01577 * @brief Configures the TIMx channel 1 polarity. 01578 * @param TIMx: where x can be 1 to 14 except 6 and 7, to select the TIM peripheral. 01579 * @param TIM_OCPolarity: specifies the OC1 Polarity 01580 * This parameter can be one of the following values: 01581 * @arg TIM_OCPolarity_High: Output Compare active high 01582 * @arg TIM_OCPolarity_Low: Output Compare active low 01583 * @retval None 01584 */ 01585 void TIM_OC1PolarityConfig(TIM_TypeDef* TIMx, uint16_t TIM_OCPolarity) 01586 { 01587 uint16_t tmpccer = 0; 01588 01589 /* Check the parameters */ 01590 assert_param(IS_TIM_LIST1_PERIPH(TIMx)); 01591 assert_param(IS_TIM_OC_POLARITY(TIM_OCPolarity)); 01592 01593 tmpccer = TIMx->CCER; 01594 01595 /* Set or Reset the CC1P Bit */ 01596 tmpccer &= (uint16_t)(~TIM_CCER_CC1P); 01597 tmpccer |= TIM_OCPolarity; 01598 01599 /* Write to TIMx CCER register */ 01600 TIMx->CCER = tmpccer; 01601 } 01602 01603 /** 01604 * @brief Configures the TIMx Channel 1N polarity. 01605 * @param TIMx: where x can be 1 or 8 to select the TIM peripheral. 01606 * @param TIM_OCNPolarity: specifies the OC1N Polarity 01607 * This parameter can be one of the following values: 01608 * @arg TIM_OCNPolarity_High: Output Compare active high 01609 * @arg TIM_OCNPolarity_Low: Output Compare active low 01610 * @retval None 01611 */ 01612 void TIM_OC1NPolarityConfig(TIM_TypeDef* TIMx, uint16_t TIM_OCNPolarity) 01613 { 01614 uint16_t tmpccer = 0; 01615 /* Check the parameters */ 01616 assert_param(IS_TIM_LIST4_PERIPH(TIMx)); 01617 assert_param(IS_TIM_OCN_POLARITY(TIM_OCNPolarity)); 01618 01619 tmpccer = TIMx->CCER; 01620 01621 /* Set or Reset the CC1NP Bit */ 01622 tmpccer &= (uint16_t)~TIM_CCER_CC1NP; 01623 tmpccer |= TIM_OCNPolarity; 01624 01625 /* Write to TIMx CCER register */ 01626 TIMx->CCER = tmpccer; 01627 } 01628 01629 /** 01630 * @brief Configures the TIMx channel 2 polarity. 01631 * @param TIMx: where x can be 1, 2, 3, 4, 5, 8, 9 or 12 to select the TIM 01632 * peripheral. 01633 * @param TIM_OCPolarity: specifies the OC2 Polarity 01634 * This parameter can be one of the following values: 01635 * @arg TIM_OCPolarity_High: Output Compare active high 01636 * @arg TIM_OCPolarity_Low: Output Compare active low 01637 * @retval None 01638 */ 01639 void TIM_OC2PolarityConfig(TIM_TypeDef* TIMx, uint16_t TIM_OCPolarity) 01640 { 01641 uint16_t tmpccer = 0; 01642 01643 /* Check the parameters */ 01644 assert_param(IS_TIM_LIST2_PERIPH(TIMx)); 01645 assert_param(IS_TIM_OC_POLARITY(TIM_OCPolarity)); 01646 01647 tmpccer = TIMx->CCER; 01648 01649 /* Set or Reset the CC2P Bit */ 01650 tmpccer &= (uint16_t)(~TIM_CCER_CC2P); 01651 tmpccer |= (uint16_t)(TIM_OCPolarity << 4); 01652 01653 /* Write to TIMx CCER register */ 01654 TIMx->CCER = tmpccer; 01655 } 01656 01657 /** 01658 * @brief Configures the TIMx Channel 2N polarity. 01659 * @param TIMx: where x can be 1 or 8 to select the TIM peripheral. 01660 * @param TIM_OCNPolarity: specifies the OC2N Polarity 01661 * This parameter can be one of the following values: 01662 * @arg TIM_OCNPolarity_High: Output Compare active high 01663 * @arg TIM_OCNPolarity_Low: Output Compare active low 01664 * @retval None 01665 */ 01666 void TIM_OC2NPolarityConfig(TIM_TypeDef* TIMx, uint16_t TIM_OCNPolarity) 01667 { 01668 uint16_t tmpccer = 0; 01669 01670 /* Check the parameters */ 01671 assert_param(IS_TIM_LIST4_PERIPH(TIMx)); 01672 assert_param(IS_TIM_OCN_POLARITY(TIM_OCNPolarity)); 01673 01674 tmpccer = TIMx->CCER; 01675 01676 /* Set or Reset the CC2NP Bit */ 01677 tmpccer &= (uint16_t)~TIM_CCER_CC2NP; 01678 tmpccer |= (uint16_t)(TIM_OCNPolarity << 4); 01679 01680 /* Write to TIMx CCER register */ 01681 TIMx->CCER = tmpccer; 01682 } 01683 01684 /** 01685 * @brief Configures the TIMx channel 3 polarity. 01686 * @param TIMx: where x can be 1, 2, 3, 4, 5 or 8 to select the TIM peripheral. 01687 * @param TIM_OCPolarity: specifies the OC3 Polarity 01688 * This parameter can be one of the following values: 01689 * @arg TIM_OCPolarity_High: Output Compare active high 01690 * @arg TIM_OCPolarity_Low: Output Compare active low 01691 * @retval None 01692 */ 01693 void TIM_OC3PolarityConfig(TIM_TypeDef* TIMx, uint16_t TIM_OCPolarity) 01694 { 01695 uint16_t tmpccer = 0; 01696 01697 /* Check the parameters */ 01698 assert_param(IS_TIM_LIST3_PERIPH(TIMx)); 01699 assert_param(IS_TIM_OC_POLARITY(TIM_OCPolarity)); 01700 01701 tmpccer = TIMx->CCER; 01702 01703 /* Set or Reset the CC3P Bit */ 01704 tmpccer &= (uint16_t)~TIM_CCER_CC3P; 01705 tmpccer |= (uint16_t)(TIM_OCPolarity << 8); 01706 01707 /* Write to TIMx CCER register */ 01708 TIMx->CCER = tmpccer; 01709 } 01710 01711 /** 01712 * @brief Configures the TIMx Channel 3N polarity. 01713 * @param TIMx: where x can be 1 or 8 to select the TIM peripheral. 01714 * @param TIM_OCNPolarity: specifies the OC3N Polarity 01715 * This parameter can be one of the following values: 01716 * @arg TIM_OCNPolarity_High: Output Compare active high 01717 * @arg TIM_OCNPolarity_Low: Output Compare active low 01718 * @retval None 01719 */ 01720 void TIM_OC3NPolarityConfig(TIM_TypeDef* TIMx, uint16_t TIM_OCNPolarity) 01721 { 01722 uint16_t tmpccer = 0; 01723 01724 /* Check the parameters */ 01725 assert_param(IS_TIM_LIST4_PERIPH(TIMx)); 01726 assert_param(IS_TIM_OCN_POLARITY(TIM_OCNPolarity)); 01727 01728 tmpccer = TIMx->CCER; 01729 01730 /* Set or Reset the CC3NP Bit */ 01731 tmpccer &= (uint16_t)~TIM_CCER_CC3NP; 01732 tmpccer |= (uint16_t)(TIM_OCNPolarity << 8); 01733 01734 /* Write to TIMx CCER register */ 01735 TIMx->CCER = tmpccer; 01736 } 01737 01738 /** 01739 * @brief Configures the TIMx channel 4 polarity. 01740 * @param TIMx: where x can be 1, 2, 3, 4, 5 or 8 to select the TIM peripheral. 01741 * @param TIM_OCPolarity: specifies the OC4 Polarity 01742 * This parameter can be one of the following values: 01743 * @arg TIM_OCPolarity_High: Output Compare active high 01744 * @arg TIM_OCPolarity_Low: Output Compare active low 01745 * @retval None 01746 */ 01747 void TIM_OC4PolarityConfig(TIM_TypeDef* TIMx, uint16_t TIM_OCPolarity) 01748 { 01749 uint16_t tmpccer = 0; 01750 01751 /* Check the parameters */ 01752 assert_param(IS_TIM_LIST3_PERIPH(TIMx)); 01753 assert_param(IS_TIM_OC_POLARITY(TIM_OCPolarity)); 01754 01755 tmpccer = TIMx->CCER; 01756 01757 /* Set or Reset the CC4P Bit */ 01758 tmpccer &= (uint16_t)~TIM_CCER_CC4P; 01759 tmpccer |= (uint16_t)(TIM_OCPolarity << 12); 01760 01761 /* Write to TIMx CCER register */ 01762 TIMx->CCER = tmpccer; 01763 } 01764 01765 /** 01766 * @brief Enables or disables the TIM Capture Compare Channel x. 01767 * @param TIMx: where x can be 1 to 14 except 6 and 7, to select the TIM peripheral. 01768 * @param TIM_Channel: specifies the TIM Channel 01769 * This parameter can be one of the following values: 01770 * @arg TIM_Channel_1: TIM Channel 1 01771 * @arg TIM_Channel_2: TIM Channel 2 01772 * @arg TIM_Channel_3: TIM Channel 3 01773 * @arg TIM_Channel_4: TIM Channel 4 01774 * @param TIM_CCx: specifies the TIM Channel CCxE bit new state. 01775 * This parameter can be: TIM_CCx_Enable or TIM_CCx_Disable. 01776 * @retval None 01777 */ 01778 void TIM_CCxCmd(TIM_TypeDef* TIMx, uint16_t TIM_Channel, uint16_t TIM_CCx) 01779 { 01780 uint16_t tmp = 0; 01781 01782 /* Check the parameters */ 01783 assert_param(IS_TIM_LIST1_PERIPH(TIMx)); 01784 assert_param(IS_TIM_CHANNEL(TIM_Channel)); 01785 assert_param(IS_TIM_CCX(TIM_CCx)); 01786 01787 tmp = CCER_CCE_SET << TIM_Channel; 01788 01789 /* Reset the CCxE Bit */ 01790 TIMx->CCER &= (uint16_t)~ tmp; 01791 01792 /* Set or reset the CCxE Bit */ 01793 TIMx->CCER |= (uint16_t)(TIM_CCx << TIM_Channel); 01794 } 01795 01796 /** 01797 * @brief Enables or disables the TIM Capture Compare Channel xN. 01798 * @param TIMx: where x can be 1 or 8 to select the TIM peripheral. 01799 * @param TIM_Channel: specifies the TIM Channel 01800 * This parameter can be one of the following values: 01801 * @arg TIM_Channel_1: TIM Channel 1 01802 * @arg TIM_Channel_2: TIM Channel 2 01803 * @arg TIM_Channel_3: TIM Channel 3 01804 * @param TIM_CCxN: specifies the TIM Channel CCxNE bit new state. 01805 * This parameter can be: TIM_CCxN_Enable or TIM_CCxN_Disable. 01806 * @retval None 01807 */ 01808 void TIM_CCxNCmd(TIM_TypeDef* TIMx, uint16_t TIM_Channel, uint16_t TIM_CCxN) 01809 { 01810 uint16_t tmp = 0; 01811 01812 /* Check the parameters */ 01813 assert_param(IS_TIM_LIST4_PERIPH(TIMx)); 01814 assert_param(IS_TIM_COMPLEMENTARY_CHANNEL(TIM_Channel)); 01815 assert_param(IS_TIM_CCXN(TIM_CCxN)); 01816 01817 tmp = CCER_CCNE_SET << TIM_Channel; 01818 01819 /* Reset the CCxNE Bit */ 01820 TIMx->CCER &= (uint16_t) ~tmp; 01821 01822 /* Set or reset the CCxNE Bit */ 01823 TIMx->CCER |= (uint16_t)(TIM_CCxN << TIM_Channel); 01824 } 01825 /** 01826 * @} 01827 */ 01828 01829 /** @defgroup TIM_Group3 Input Capture management functions 01830 * @brief Input Capture management functions 01831 * 01832 @verbatim 01833 =============================================================================== 01834 Input Capture management functions 01835 =============================================================================== 01836 01837 =================================================================== 01838 TIM Driver: how to use it in Input Capture Mode 01839 =================================================================== 01840 To use the Timer in Input Capture mode, the following steps are mandatory: 01841 01842 1. Enable TIM clock using RCC_APBxPeriphClockCmd(RCC_APBxPeriph_TIMx, ENABLE) function 01843 01844 2. Configure the TIM pins by configuring the corresponding GPIO pins 01845 01846 2. Configure the Time base unit as described in the first part of this driver, 01847 if needed, else the Timer will run with the default configuration: 01848 - Autoreload value = 0xFFFF 01849 - Prescaler value = 0x0000 01850 - Counter mode = Up counting 01851 - Clock Division = TIM_CKD_DIV1 01852 01853 3. Fill the TIM_ICInitStruct with the desired parameters including: 01854 - TIM Channel: TIM_Channel 01855 - TIM Input Capture polarity: TIM_ICPolarity 01856 - TIM Input Capture selection: TIM_ICSelection 01857 - TIM Input Capture Prescaler: TIM_ICPrescaler 01858 - TIM Input CApture filter value: TIM_ICFilter 01859 01860 4. Call TIM_ICInit(TIMx, &TIM_ICInitStruct) to configure the desired channel with the 01861 corresponding configuration and to measure only frequency or duty cycle of the input signal, 01862 or, 01863 Call TIM_PWMIConfig(TIMx, &TIM_ICInitStruct) to configure the desired channels with the 01864 corresponding configuration and to measure the frequency and the duty cycle of the input signal 01865 01866 5. Enable the NVIC or the DMA to read the measured frequency. 01867 01868 6. Enable the corresponding interrupt (or DMA request) to read the Captured value, 01869 using the function TIM_ITConfig(TIMx, TIM_IT_CCx) (or TIM_DMA_Cmd(TIMx, TIM_DMA_CCx)) 01870 01871 7. Call the TIM_Cmd(ENABLE) function to enable the TIM counter. 01872 01873 8. Use TIM_GetCapturex(TIMx); to read the captured value. 01874 01875 Note1: All other functions can be used separately to modify, if needed, 01876 a specific feature of the Timer. 01877 01878 @endverbatim 01879 * @{ 01880 */ 01881 01882 /** 01883 * @brief Initializes the TIM peripheral according to the specified parameters 01884 * in the TIM_ICInitStruct. 01885 * @param TIMx: where x can be 1 to 14 except 6 and 7, to select the TIM peripheral. 01886 * @param TIM_ICInitStruct: pointer to a TIM_ICInitTypeDef structure that contains 01887 * the configuration information for the specified TIM peripheral. 01888 * @retval None 01889 */ 01890 void TIM_ICInit(TIM_TypeDef* TIMx, TIM_ICInitTypeDef* TIM_ICInitStruct) 01891 { 01892 /* Check the parameters */ 01893 assert_param(IS_TIM_LIST1_PERIPH(TIMx)); 01894 assert_param(IS_TIM_IC_POLARITY(TIM_ICInitStruct->TIM_ICPolarity)); 01895 assert_param(IS_TIM_IC_SELECTION(TIM_ICInitStruct->TIM_ICSelection)); 01896 assert_param(IS_TIM_IC_PRESCALER(TIM_ICInitStruct->TIM_ICPrescaler)); 01897 assert_param(IS_TIM_IC_FILTER(TIM_ICInitStruct->TIM_ICFilter)); 01898 01899 if (TIM_ICInitStruct->TIM_Channel == TIM_Channel_1) 01900 { 01901 /* TI1 Configuration */ 01902 TI1_Config(TIMx, TIM_ICInitStruct->TIM_ICPolarity, 01903 TIM_ICInitStruct->TIM_ICSelection, 01904 TIM_ICInitStruct->TIM_ICFilter); 01905 /* Set the Input Capture Prescaler value */ 01906 TIM_SetIC1Prescaler(TIMx, TIM_ICInitStruct->TIM_ICPrescaler); 01907 } 01908 else if (TIM_ICInitStruct->TIM_Channel == TIM_Channel_2) 01909 { 01910 /* TI2 Configuration */ 01911 assert_param(IS_TIM_LIST2_PERIPH(TIMx)); 01912 TI2_Config(TIMx, TIM_ICInitStruct->TIM_ICPolarity, 01913 TIM_ICInitStruct->TIM_ICSelection, 01914 TIM_ICInitStruct->TIM_ICFilter); 01915 /* Set the Input Capture Prescaler value */ 01916 TIM_SetIC2Prescaler(TIMx, TIM_ICInitStruct->TIM_ICPrescaler); 01917 } 01918 else if (TIM_ICInitStruct->TIM_Channel == TIM_Channel_3) 01919 { 01920 /* TI3 Configuration */ 01921 assert_param(IS_TIM_LIST3_PERIPH(TIMx)); 01922 TI3_Config(TIMx, TIM_ICInitStruct->TIM_ICPolarity, 01923 TIM_ICInitStruct->TIM_ICSelection, 01924 TIM_ICInitStruct->TIM_ICFilter); 01925 /* Set the Input Capture Prescaler value */ 01926 TIM_SetIC3Prescaler(TIMx, TIM_ICInitStruct->TIM_ICPrescaler); 01927 } 01928 else 01929 { 01930 /* TI4 Configuration */ 01931 assert_param(IS_TIM_LIST3_PERIPH(TIMx)); 01932 TI4_Config(TIMx, TIM_ICInitStruct->TIM_ICPolarity, 01933 TIM_ICInitStruct->TIM_ICSelection, 01934 TIM_ICInitStruct->TIM_ICFilter); 01935 /* Set the Input Capture Prescaler value */ 01936 TIM_SetIC4Prescaler(TIMx, TIM_ICInitStruct->TIM_ICPrescaler); 01937 } 01938 } 01939 01940 /** 01941 * @brief Fills each TIM_ICInitStruct member with its default value. 01942 * @param TIM_ICInitStruct: pointer to a TIM_ICInitTypeDef structure which will 01943 * be initialized. 01944 * @retval None 01945 */ 01946 void TIM_ICStructInit(TIM_ICInitTypeDef* TIM_ICInitStruct) 01947 { 01948 /* Set the default configuration */ 01949 TIM_ICInitStruct->TIM_Channel = TIM_Channel_1; 01950 TIM_ICInitStruct->TIM_ICPolarity = TIM_ICPolarity_Rising; 01951 TIM_ICInitStruct->TIM_ICSelection = TIM_ICSelection_DirectTI; 01952 TIM_ICInitStruct->TIM_ICPrescaler = TIM_ICPSC_DIV1; 01953 TIM_ICInitStruct->TIM_ICFilter = 0x00; 01954 } 01955 01956 /** 01957 * @brief Configures the TIM peripheral according to the specified parameters 01958 * in the TIM_ICInitStruct to measure an external PWM signal. 01959 * @param TIMx: where x can be 1, 2, 3, 4, 5,8, 9 or 12 to select the TIM 01960 * peripheral. 01961 * @param TIM_ICInitStruct: pointer to a TIM_ICInitTypeDef structure that contains 01962 * the configuration information for the specified TIM peripheral. 01963 * @retval None 01964 */ 01965 void TIM_PWMIConfig(TIM_TypeDef* TIMx, TIM_ICInitTypeDef* TIM_ICInitStruct) 01966 { 01967 uint16_t icoppositepolarity = TIM_ICPolarity_Rising; 01968 uint16_t icoppositeselection = TIM_ICSelection_DirectTI; 01969 01970 /* Check the parameters */ 01971 assert_param(IS_TIM_LIST2_PERIPH(TIMx)); 01972 01973 /* Select the Opposite Input Polarity */ 01974 if (TIM_ICInitStruct->TIM_ICPolarity == TIM_ICPolarity_Rising) 01975 { 01976 icoppositepolarity = TIM_ICPolarity_Falling; 01977 } 01978 else 01979 { 01980 icoppositepolarity = TIM_ICPolarity_Rising; 01981 } 01982 /* Select the Opposite Input */ 01983 if (TIM_ICInitStruct->TIM_ICSelection == TIM_ICSelection_DirectTI) 01984 { 01985 icoppositeselection = TIM_ICSelection_IndirectTI; 01986 } 01987 else 01988 { 01989 icoppositeselection = TIM_ICSelection_DirectTI; 01990 } 01991 if (TIM_ICInitStruct->TIM_Channel == TIM_Channel_1) 01992 { 01993 /* TI1 Configuration */ 01994 TI1_Config(TIMx, TIM_ICInitStruct->TIM_ICPolarity, TIM_ICInitStruct->TIM_ICSelection, 01995 TIM_ICInitStruct->TIM_ICFilter); 01996 /* Set the Input Capture Prescaler value */ 01997 TIM_SetIC1Prescaler(TIMx, TIM_ICInitStruct->TIM_ICPrescaler); 01998 /* TI2 Configuration */ 01999 TI2_Config(TIMx, icoppositepolarity, icoppositeselection, TIM_ICInitStruct->TIM_ICFilter); 02000 /* Set the Input Capture Prescaler value */ 02001 TIM_SetIC2Prescaler(TIMx, TIM_ICInitStruct->TIM_ICPrescaler); 02002 } 02003 else 02004 { 02005 /* TI2 Configuration */ 02006 TI2_Config(TIMx, TIM_ICInitStruct->TIM_ICPolarity, TIM_ICInitStruct->TIM_ICSelection, 02007 TIM_ICInitStruct->TIM_ICFilter); 02008 /* Set the Input Capture Prescaler value */ 02009 TIM_SetIC2Prescaler(TIMx, TIM_ICInitStruct->TIM_ICPrescaler); 02010 /* TI1 Configuration */ 02011 TI1_Config(TIMx, icoppositepolarity, icoppositeselection, TIM_ICInitStruct->TIM_ICFilter); 02012 /* Set the Input Capture Prescaler value */ 02013 TIM_SetIC1Prescaler(TIMx, TIM_ICInitStruct->TIM_ICPrescaler); 02014 } 02015 } 02016 02017 /** 02018 * @brief Gets the TIMx Input Capture 1 value. 02019 * @param TIMx: where x can be 1 to 14 except 6 and 7, to select the TIM peripheral. 02020 * @retval Capture Compare 1 Register value. 02021 */ 02022 uint32_t TIM_GetCapture1(TIM_TypeDef* TIMx) 02023 { 02024 /* Check the parameters */ 02025 assert_param(IS_TIM_LIST1_PERIPH(TIMx)); 02026 02027 /* Get the Capture 1 Register value */ 02028 return TIMx->CCR1; 02029 } 02030 02031 /** 02032 * @brief Gets the TIMx Input Capture 2 value. 02033 * @param TIMx: where x can be 1, 2, 3, 4, 5, 8, 9 or 12 to select the TIM 02034 * peripheral. 02035 * @retval Capture Compare 2 Register value. 02036 */ 02037 uint32_t TIM_GetCapture2(TIM_TypeDef* TIMx) 02038 { 02039 /* Check the parameters */ 02040 assert_param(IS_TIM_LIST2_PERIPH(TIMx)); 02041 02042 /* Get the Capture 2 Register value */ 02043 return TIMx->CCR2; 02044 } 02045 02046 /** 02047 * @brief Gets the TIMx Input Capture 3 value. 02048 * @param TIMx: where x can be 1, 2, 3, 4, 5 or 8 to select the TIM peripheral. 02049 * @retval Capture Compare 3 Register value. 02050 */ 02051 uint32_t TIM_GetCapture3(TIM_TypeDef* TIMx) 02052 { 02053 /* Check the parameters */ 02054 assert_param(IS_TIM_LIST3_PERIPH(TIMx)); 02055 02056 /* Get the Capture 3 Register value */ 02057 return TIMx->CCR3; 02058 } 02059 02060 /** 02061 * @brief Gets the TIMx Input Capture 4 value. 02062 * @param TIMx: where x can be 1, 2, 3, 4, 5 or 8 to select the TIM peripheral. 02063 * @retval Capture Compare 4 Register value. 02064 */ 02065 uint32_t TIM_GetCapture4(TIM_TypeDef* TIMx) 02066 { 02067 /* Check the parameters */ 02068 assert_param(IS_TIM_LIST3_PERIPH(TIMx)); 02069 02070 /* Get the Capture 4 Register value */ 02071 return TIMx->CCR4; 02072 } 02073 02074 /** 02075 * @brief Sets the TIMx Input Capture 1 prescaler. 02076 * @param TIMx: where x can be 1 to 14 except 6 and 7, to select the TIM peripheral. 02077 * @param TIM_ICPSC: specifies the Input Capture1 prescaler new value. 02078 * This parameter can be one of the following values: 02079 * @arg TIM_ICPSC_DIV1: no prescaler 02080 * @arg TIM_ICPSC_DIV2: capture is done once every 2 events 02081 * @arg TIM_ICPSC_DIV4: capture is done once every 4 events 02082 * @arg TIM_ICPSC_DIV8: capture is done once every 8 events 02083 * @retval None 02084 */ 02085 void TIM_SetIC1Prescaler(TIM_TypeDef* TIMx, uint16_t TIM_ICPSC) 02086 { 02087 /* Check the parameters */ 02088 assert_param(IS_TIM_LIST1_PERIPH(TIMx)); 02089 assert_param(IS_TIM_IC_PRESCALER(TIM_ICPSC)); 02090 02091 /* Reset the IC1PSC Bits */ 02092 TIMx->CCMR1 &= (uint16_t)~TIM_CCMR1_IC1PSC; 02093 02094 /* Set the IC1PSC value */ 02095 TIMx->CCMR1 |= TIM_ICPSC; 02096 } 02097 02098 /** 02099 * @brief Sets the TIMx Input Capture 2 prescaler. 02100 * @param TIMx: where x can be 1, 2, 3, 4, 5, 8, 9 or 12 to select the TIM 02101 * peripheral. 02102 * @param TIM_ICPSC: specifies the Input Capture2 prescaler new value. 02103 * This parameter can be one of the following values: 02104 * @arg TIM_ICPSC_DIV1: no prescaler 02105 * @arg TIM_ICPSC_DIV2: capture is done once every 2 events 02106 * @arg TIM_ICPSC_DIV4: capture is done once every 4 events 02107 * @arg TIM_ICPSC_DIV8: capture is done once every 8 events 02108 * @retval None 02109 */ 02110 void TIM_SetIC2Prescaler(TIM_TypeDef* TIMx, uint16_t TIM_ICPSC) 02111 { 02112 /* Check the parameters */ 02113 assert_param(IS_TIM_LIST2_PERIPH(TIMx)); 02114 assert_param(IS_TIM_IC_PRESCALER(TIM_ICPSC)); 02115 02116 /* Reset the IC2PSC Bits */ 02117 TIMx->CCMR1 &= (uint16_t)~TIM_CCMR1_IC2PSC; 02118 02119 /* Set the IC2PSC value */ 02120 TIMx->CCMR1 |= (uint16_t)(TIM_ICPSC << 8); 02121 } 02122 02123 /** 02124 * @brief Sets the TIMx Input Capture 3 prescaler. 02125 * @param TIMx: where x can be 1, 2, 3, 4, 5 or 8 to select the TIM peripheral. 02126 * @param TIM_ICPSC: specifies the Input Capture3 prescaler new value. 02127 * This parameter can be one of the following values: 02128 * @arg TIM_ICPSC_DIV1: no prescaler 02129 * @arg TIM_ICPSC_DIV2: capture is done once every 2 events 02130 * @arg TIM_ICPSC_DIV4: capture is done once every 4 events 02131 * @arg TIM_ICPSC_DIV8: capture is done once every 8 events 02132 * @retval None 02133 */ 02134 void TIM_SetIC3Prescaler(TIM_TypeDef* TIMx, uint16_t TIM_ICPSC) 02135 { 02136 /* Check the parameters */ 02137 assert_param(IS_TIM_LIST3_PERIPH(TIMx)); 02138 assert_param(IS_TIM_IC_PRESCALER(TIM_ICPSC)); 02139 02140 /* Reset the IC3PSC Bits */ 02141 TIMx->CCMR2 &= (uint16_t)~TIM_CCMR2_IC3PSC; 02142 02143 /* Set the IC3PSC value */ 02144 TIMx->CCMR2 |= TIM_ICPSC; 02145 } 02146 02147 /** 02148 * @brief Sets the TIMx Input Capture 4 prescaler. 02149 * @param TIMx: where x can be 1, 2, 3, 4, 5 or 8 to select the TIM peripheral. 02150 * @param TIM_ICPSC: specifies the Input Capture4 prescaler new value. 02151 * This parameter can be one of the following values: 02152 * @arg TIM_ICPSC_DIV1: no prescaler 02153 * @arg TIM_ICPSC_DIV2: capture is done once every 2 events 02154 * @arg TIM_ICPSC_DIV4: capture is done once every 4 events 02155 * @arg TIM_ICPSC_DIV8: capture is done once every 8 events 02156 * @retval None 02157 */ 02158 void TIM_SetIC4Prescaler(TIM_TypeDef* TIMx, uint16_t TIM_ICPSC) 02159 { 02160 /* Check the parameters */ 02161 assert_param(IS_TIM_LIST3_PERIPH(TIMx)); 02162 assert_param(IS_TIM_IC_PRESCALER(TIM_ICPSC)); 02163 02164 /* Reset the IC4PSC Bits */ 02165 TIMx->CCMR2 &= (uint16_t)~TIM_CCMR2_IC4PSC; 02166 02167 /* Set the IC4PSC value */ 02168 TIMx->CCMR2 |= (uint16_t)(TIM_ICPSC << 8); 02169 } 02170 /** 02171 * @} 02172 */ 02173 02174 /** @defgroup TIM_Group4 Advanced-control timers (TIM1 and TIM8) specific features 02175 * @brief Advanced-control timers (TIM1 and TIM8) specific features 02176 * 02177 @verbatim 02178 =============================================================================== 02179 Advanced-control timers (TIM1 and TIM8) specific features 02180 =============================================================================== 02181 02182 =================================================================== 02183 TIM Driver: how to use the Break feature 02184 =================================================================== 02185 After configuring the Timer channel(s) in the appropriate Output Compare mode: 02186 02187 1. Fill the TIM_BDTRInitStruct with the desired parameters for the Timer 02188 Break Polarity, dead time, Lock level, the OSSI/OSSR State and the 02189 AOE(automatic output enable). 02190 02191 2. Call TIM_BDTRConfig(TIMx, &TIM_BDTRInitStruct) to configure the Timer 02192 02193 3. Enable the Main Output using TIM_CtrlPWMOutputs(TIM1, ENABLE) 02194 02195 4. Once the break even occurs, the Timer's output signals are put in reset 02196 state or in a known state (according to the configuration made in 02197 TIM_BDTRConfig() function). 02198 02199 @endverbatim 02200 * @{ 02201 */ 02202 02203 /** 02204 * @brief Configures the Break feature, dead time, Lock level, OSSI/OSSR State 02205 * and the AOE(automatic output enable). 02206 * @param TIMx: where x can be 1 or 8 to select the TIM 02207 * @param TIM_BDTRInitStruct: pointer to a TIM_BDTRInitTypeDef structure that 02208 * contains the BDTR Register configuration information for the TIM peripheral. 02209 * @retval None 02210 */ 02211 void TIM_BDTRConfig(TIM_TypeDef* TIMx, TIM_BDTRInitTypeDef *TIM_BDTRInitStruct) 02212 { 02213 /* Check the parameters */ 02214 assert_param(IS_TIM_LIST4_PERIPH(TIMx)); 02215 assert_param(IS_TIM_OSSR_STATE(TIM_BDTRInitStruct->TIM_OSSRState)); 02216 assert_param(IS_TIM_OSSI_STATE(TIM_BDTRInitStruct->TIM_OSSIState)); 02217 assert_param(IS_TIM_LOCK_LEVEL(TIM_BDTRInitStruct->TIM_LOCKLevel)); 02218 assert_param(IS_TIM_BREAK_STATE(TIM_BDTRInitStruct->TIM_Break)); 02219 assert_param(IS_TIM_BREAK_POLARITY(TIM_BDTRInitStruct->TIM_BreakPolarity)); 02220 assert_param(IS_TIM_AUTOMATIC_OUTPUT_STATE(TIM_BDTRInitStruct->TIM_AutomaticOutput)); 02221 02222 /* Set the Lock level, the Break enable Bit and the Polarity, the OSSR State, 02223 the OSSI State, the dead time value and the Automatic Output Enable Bit */ 02224 TIMx->BDTR = (uint32_t)TIM_BDTRInitStruct->TIM_OSSRState | TIM_BDTRInitStruct->TIM_OSSIState | 02225 TIM_BDTRInitStruct->TIM_LOCKLevel | TIM_BDTRInitStruct->TIM_DeadTime | 02226 TIM_BDTRInitStruct->TIM_Break | TIM_BDTRInitStruct->TIM_BreakPolarity | 02227 TIM_BDTRInitStruct->TIM_AutomaticOutput; 02228 } 02229 02230 /** 02231 * @brief Fills each TIM_BDTRInitStruct member with its default value. 02232 * @param TIM_BDTRInitStruct: pointer to a TIM_BDTRInitTypeDef structure which 02233 * will be initialized. 02234 * @retval None 02235 */ 02236 void TIM_BDTRStructInit(TIM_BDTRInitTypeDef* TIM_BDTRInitStruct) 02237 { 02238 /* Set the default configuration */ 02239 TIM_BDTRInitStruct->TIM_OSSRState = TIM_OSSRState_Disable; 02240 TIM_BDTRInitStruct->TIM_OSSIState = TIM_OSSIState_Disable; 02241 TIM_BDTRInitStruct->TIM_LOCKLevel = TIM_LOCKLevel_OFF; 02242 TIM_BDTRInitStruct->TIM_DeadTime = 0x00; 02243 TIM_BDTRInitStruct->TIM_Break = TIM_Break_Disable; 02244 TIM_BDTRInitStruct->TIM_BreakPolarity = TIM_BreakPolarity_Low; 02245 TIM_BDTRInitStruct->TIM_AutomaticOutput = TIM_AutomaticOutput_Disable; 02246 } 02247 02248 /** 02249 * @brief Enables or disables the TIM peripheral Main Outputs. 02250 * @param TIMx: where x can be 1 or 8 to select the TIMx peripheral. 02251 * @param NewState: new state of the TIM peripheral Main Outputs. 02252 * This parameter can be: ENABLE or DISABLE. 02253 * @retval None 02254 */ 02255 void TIM_CtrlPWMOutputs(TIM_TypeDef* TIMx, FunctionalState NewState) 02256 { 02257 /* Check the parameters */ 02258 assert_param(IS_TIM_LIST4_PERIPH(TIMx)); 02259 assert_param(IS_FUNCTIONAL_STATE(NewState)); 02260 02261 if (NewState != DISABLE) 02262 { 02263 /* Enable the TIM Main Output */ 02264 TIMx->BDTR |= TIM_BDTR_MOE; 02265 } 02266 else 02267 { 02268 /* Disable the TIM Main Output */ 02269 TIMx->BDTR &= (uint16_t)~TIM_BDTR_MOE; 02270 } 02271 } 02272 02273 /** 02274 * @brief Selects the TIM peripheral Commutation event. 02275 * @param TIMx: where x can be 1 or 8 to select the TIMx peripheral 02276 * @param NewState: new state of the Commutation event. 02277 * This parameter can be: ENABLE or DISABLE. 02278 * @retval None 02279 */ 02280 void TIM_SelectCOM(TIM_TypeDef* TIMx, FunctionalState NewState) 02281 { 02282 /* Check the parameters */ 02283 assert_param(IS_TIM_LIST4_PERIPH(TIMx)); 02284 assert_param(IS_FUNCTIONAL_STATE(NewState)); 02285 02286 if (NewState != DISABLE) 02287 { 02288 /* Set the COM Bit */ 02289 TIMx->CR2 |= TIM_CR2_CCUS; 02290 } 02291 else 02292 { 02293 /* Reset the COM Bit */ 02294 TIMx->CR2 &= (uint16_t)~TIM_CR2_CCUS; 02295 } 02296 } 02297 02298 /** 02299 * @brief Sets or Resets the TIM peripheral Capture Compare Preload Control bit. 02300 * @param TIMx: where x can be 1 or 8 to select the TIMx peripheral 02301 * @param NewState: new state of the Capture Compare Preload Control bit 02302 * This parameter can be: ENABLE or DISABLE. 02303 * @retval None 02304 */ 02305 void TIM_CCPreloadControl(TIM_TypeDef* TIMx, FunctionalState NewState) 02306 { 02307 /* Check the parameters */ 02308 assert_param(IS_TIM_LIST4_PERIPH(TIMx)); 02309 assert_param(IS_FUNCTIONAL_STATE(NewState)); 02310 if (NewState != DISABLE) 02311 { 02312 /* Set the CCPC Bit */ 02313 TIMx->CR2 |= TIM_CR2_CCPC; 02314 } 02315 else 02316 { 02317 /* Reset the CCPC Bit */ 02318 TIMx->CR2 &= (uint16_t)~TIM_CR2_CCPC; 02319 } 02320 } 02321 /** 02322 * @} 02323 */ 02324 02325 /** @defgroup TIM_Group5 Interrupts DMA and flags management functions 02326 * @brief Interrupts, DMA and flags management functions 02327 * 02328 @verbatim 02329 =============================================================================== 02330 Interrupts, DMA and flags management functions 02331 =============================================================================== 02332 02333 @endverbatim 02334 * @{ 02335 */ 02336 02337 /** 02338 * @brief Enables or disables the specified TIM interrupts. 02339 * @param TIMx: where x can be 1 to 14 to select the TIMx peripheral. 02340 * @param TIM_IT: specifies the TIM interrupts sources to be enabled or disabled. 02341 * This parameter can be any combination of the following values: 02342 * @arg TIM_IT_Update: TIM update Interrupt source 02343 * @arg TIM_IT_CC1: TIM Capture Compare 1 Interrupt source 02344 * @arg TIM_IT_CC2: TIM Capture Compare 2 Interrupt source 02345 * @arg TIM_IT_CC3: TIM Capture Compare 3 Interrupt source 02346 * @arg TIM_IT_CC4: TIM Capture Compare 4 Interrupt source 02347 * @arg TIM_IT_COM: TIM Commutation Interrupt source 02348 * @arg TIM_IT_Trigger: TIM Trigger Interrupt source 02349 * @arg TIM_IT_Break: TIM Break Interrupt source 02350 * 02351 * @note For TIM6 and TIM7 only the parameter TIM_IT_Update can be used 02352 * @note For TIM9 and TIM12 only one of the following parameters can be used: TIM_IT_Update, 02353 * TIM_IT_CC1, TIM_IT_CC2 or TIM_IT_Trigger. 02354 * @note For TIM10, TIM11, TIM13 and TIM14 only one of the following parameters can 02355 * be used: TIM_IT_Update or TIM_IT_CC1 02356 * @note TIM_IT_COM and TIM_IT_Break can be used only with TIM1 and TIM8 02357 * 02358 * @param NewState: new state of the TIM interrupts. 02359 * This parameter can be: ENABLE or DISABLE. 02360 * @retval None 02361 */ 02362 void TIM_ITConfig(TIM_TypeDef* TIMx, uint16_t TIM_IT, FunctionalState NewState) 02363 { 02364 /* Check the parameters */ 02365 assert_param(IS_TIM_ALL_PERIPH(TIMx)); 02366 assert_param(IS_TIM_IT(TIM_IT)); 02367 assert_param(IS_FUNCTIONAL_STATE(NewState)); 02368 02369 if (NewState != DISABLE) 02370 { 02371 /* Enable the Interrupt sources */ 02372 TIMx->DIER |= TIM_IT; 02373 } 02374 else 02375 { 02376 /* Disable the Interrupt sources */ 02377 TIMx->DIER &= (uint16_t)~TIM_IT; 02378 } 02379 } 02380 02381 /** 02382 * @brief Configures the TIMx event to be generate by software. 02383 * @param TIMx: where x can be 1 to 14 to select the TIM peripheral. 02384 * @param TIM_EventSource: specifies the event source. 02385 * This parameter can be one or more of the following values: 02386 * @arg TIM_EventSource_Update: Timer update Event source 02387 * @arg TIM_EventSource_CC1: Timer Capture Compare 1 Event source 02388 * @arg TIM_EventSource_CC2: Timer Capture Compare 2 Event source 02389 * @arg TIM_EventSource_CC3: Timer Capture Compare 3 Event source 02390 * @arg TIM_EventSource_CC4: Timer Capture Compare 4 Event source 02391 * @arg TIM_EventSource_COM: Timer COM event source 02392 * @arg TIM_EventSource_Trigger: Timer Trigger Event source 02393 * @arg TIM_EventSource_Break: Timer Break event source 02394 * 02395 * @note TIM6 and TIM7 can only generate an update event. 02396 * @note TIM_EventSource_COM and TIM_EventSource_Break are used only with TIM1 and TIM8. 02397 * 02398 * @retval None 02399 */ 02400 void TIM_GenerateEvent(TIM_TypeDef* TIMx, uint16_t TIM_EventSource) 02401 { 02402 /* Check the parameters */ 02403 assert_param(IS_TIM_ALL_PERIPH(TIMx)); 02404 assert_param(IS_TIM_EVENT_SOURCE(TIM_EventSource)); 02405 02406 /* Set the event sources */ 02407 TIMx->EGR = TIM_EventSource; 02408 } 02409 02410 /** 02411 * @brief Checks whether the specified TIM flag is set or not. 02412 * @param TIMx: where x can be 1 to 14 to select the TIM peripheral. 02413 * @param TIM_FLAG: specifies the flag to check. 02414 * This parameter can be one of the following values: 02415 * @arg TIM_FLAG_Update: TIM update Flag 02416 * @arg TIM_FLAG_CC1: TIM Capture Compare 1 Flag 02417 * @arg TIM_FLAG_CC2: TIM Capture Compare 2 Flag 02418 * @arg TIM_FLAG_CC3: TIM Capture Compare 3 Flag 02419 * @arg TIM_FLAG_CC4: TIM Capture Compare 4 Flag 02420 * @arg TIM_FLAG_COM: TIM Commutation Flag 02421 * @arg TIM_FLAG_Trigger: TIM Trigger Flag 02422 * @arg TIM_FLAG_Break: TIM Break Flag 02423 * @arg TIM_FLAG_CC1OF: TIM Capture Compare 1 over capture Flag 02424 * @arg TIM_FLAG_CC2OF: TIM Capture Compare 2 over capture Flag 02425 * @arg TIM_FLAG_CC3OF: TIM Capture Compare 3 over capture Flag 02426 * @arg TIM_FLAG_CC4OF: TIM Capture Compare 4 over capture Flag 02427 * 02428 * @note TIM6 and TIM7 can have only one update flag. 02429 * @note TIM_FLAG_COM and TIM_FLAG_Break are used only with TIM1 and TIM8. 02430 * 02431 * @retval The new state of TIM_FLAG (SET or RESET). 02432 */ 02433 FlagStatus TIM_GetFlagStatus(TIM_TypeDef* TIMx, uint16_t TIM_FLAG) 02434 { 02435 ITStatus bitstatus = RESET; 02436 /* Check the parameters */ 02437 assert_param(IS_TIM_ALL_PERIPH(TIMx)); 02438 assert_param(IS_TIM_GET_FLAG(TIM_FLAG)); 02439 02440 02441 if ((TIMx->SR & TIM_FLAG) != (uint16_t)RESET) 02442 { 02443 bitstatus = SET; 02444 } 02445 else 02446 { 02447 bitstatus = RESET; 02448 } 02449 return bitstatus; 02450 } 02451 02452 /** 02453 * @brief Clears the TIMx's pending flags. 02454 * @param TIMx: where x can be 1 to 14 to select the TIM peripheral. 02455 * @param TIM_FLAG: specifies the flag bit to clear. 02456 * This parameter can be any combination of the following values: 02457 * @arg TIM_FLAG_Update: TIM update Flag 02458 * @arg TIM_FLAG_CC1: TIM Capture Compare 1 Flag 02459 * @arg TIM_FLAG_CC2: TIM Capture Compare 2 Flag 02460 * @arg TIM_FLAG_CC3: TIM Capture Compare 3 Flag 02461 * @arg TIM_FLAG_CC4: TIM Capture Compare 4 Flag 02462 * @arg TIM_FLAG_COM: TIM Commutation Flag 02463 * @arg TIM_FLAG_Trigger: TIM Trigger Flag 02464 * @arg TIM_FLAG_Break: TIM Break Flag 02465 * @arg TIM_FLAG_CC1OF: TIM Capture Compare 1 over capture Flag 02466 * @arg TIM_FLAG_CC2OF: TIM Capture Compare 2 over capture Flag 02467 * @arg TIM_FLAG_CC3OF: TIM Capture Compare 3 over capture Flag 02468 * @arg TIM_FLAG_CC4OF: TIM Capture Compare 4 over capture Flag 02469 * 02470 * @note TIM6 and TIM7 can have only one update flag. 02471 * @note TIM_FLAG_COM and TIM_FLAG_Break are used only with TIM1 and TIM8. 02472 * 02473 * @retval None 02474 */ 02475 void TIM_ClearFlag(TIM_TypeDef* TIMx, uint16_t TIM_FLAG) 02476 { 02477 /* Check the parameters */ 02478 assert_param(IS_TIM_ALL_PERIPH(TIMx)); 02479 02480 /* Clear the flags */ 02481 TIMx->SR = (uint16_t)~TIM_FLAG; 02482 } 02483 02484 /** 02485 * @brief Checks whether the TIM interrupt has occurred or not. 02486 * @param TIMx: where x can be 1 to 14 to select the TIM peripheral. 02487 * @param TIM_IT: specifies the TIM interrupt source to check. 02488 * This parameter can be one of the following values: 02489 * @arg TIM_IT_Update: TIM update Interrupt source 02490 * @arg TIM_IT_CC1: TIM Capture Compare 1 Interrupt source 02491 * @arg TIM_IT_CC2: TIM Capture Compare 2 Interrupt source 02492 * @arg TIM_IT_CC3: TIM Capture Compare 3 Interrupt source 02493 * @arg TIM_IT_CC4: TIM Capture Compare 4 Interrupt source 02494 * @arg TIM_IT_COM: TIM Commutation Interrupt source 02495 * @arg TIM_IT_Trigger: TIM Trigger Interrupt source 02496 * @arg TIM_IT_Break: TIM Break Interrupt source 02497 * 02498 * @note TIM6 and TIM7 can generate only an update interrupt. 02499 * @note TIM_IT_COM and TIM_IT_Break are used only with TIM1 and TIM8. 02500 * 02501 * @retval The new state of the TIM_IT(SET or RESET). 02502 */ 02503 ITStatus TIM_GetITStatus(TIM_TypeDef* TIMx, uint16_t TIM_IT) 02504 { 02505 ITStatus bitstatus = RESET; 02506 uint16_t itstatus = 0x0, itenable = 0x0; 02507 /* Check the parameters */ 02508 assert_param(IS_TIM_ALL_PERIPH(TIMx)); 02509 assert_param(IS_TIM_GET_IT(TIM_IT)); 02510 02511 itstatus = TIMx->SR & TIM_IT; 02512 02513 itenable = TIMx->DIER & TIM_IT; 02514 if ((itstatus != (uint16_t)RESET) && (itenable != (uint16_t)RESET)) 02515 { 02516 bitstatus = SET; 02517 } 02518 else 02519 { 02520 bitstatus = RESET; 02521 } 02522 return bitstatus; 02523 } 02524 02525 /** 02526 * @brief Clears the TIMx's interrupt pending bits. 02527 * @param TIMx: where x can be 1 to 14 to select the TIM peripheral. 02528 * @param TIM_IT: specifies the pending bit to clear. 02529 * This parameter can be any combination of the following values: 02530 * @arg TIM_IT_Update: TIM1 update Interrupt source 02531 * @arg TIM_IT_CC1: TIM Capture Compare 1 Interrupt source 02532 * @arg TIM_IT_CC2: TIM Capture Compare 2 Interrupt source 02533 * @arg TIM_IT_CC3: TIM Capture Compare 3 Interrupt source 02534 * @arg TIM_IT_CC4: TIM Capture Compare 4 Interrupt source 02535 * @arg TIM_IT_COM: TIM Commutation Interrupt source 02536 * @arg TIM_IT_Trigger: TIM Trigger Interrupt source 02537 * @arg TIM_IT_Break: TIM Break Interrupt source 02538 * 02539 * @note TIM6 and TIM7 can generate only an update interrupt. 02540 * @note TIM_IT_COM and TIM_IT_Break are used only with TIM1 and TIM8. 02541 * 02542 * @retval None 02543 */ 02544 void TIM_ClearITPendingBit(TIM_TypeDef* TIMx, uint16_t TIM_IT) 02545 { 02546 /* Check the parameters */ 02547 assert_param(IS_TIM_ALL_PERIPH(TIMx)); 02548 02549 /* Clear the IT pending Bit */ 02550 TIMx->SR = (uint16_t)~TIM_IT; 02551 } 02552 02553 /** 02554 * @brief Configures the TIMx's DMA interface. 02555 * @param TIMx: where x can be 1, 2, 3, 4, 5 or 8 to select the TIM peripheral. 02556 * @param TIM_DMABase: DMA Base address. 02557 * This parameter can be one of the following values: 02558 * @arg TIM_DMABase_CR1 02559 * @arg TIM_DMABase_CR2 02560 * @arg TIM_DMABase_SMCR 02561 * @arg TIM_DMABase_DIER 02562 * @arg TIM1_DMABase_SR 02563 * @arg TIM_DMABase_EGR 02564 * @arg TIM_DMABase_CCMR1 02565 * @arg TIM_DMABase_CCMR2 02566 * @arg TIM_DMABase_CCER 02567 * @arg TIM_DMABase_CNT 02568 * @arg TIM_DMABase_PSC 02569 * @arg TIM_DMABase_ARR 02570 * @arg TIM_DMABase_RCR 02571 * @arg TIM_DMABase_CCR1 02572 * @arg TIM_DMABase_CCR2 02573 * @arg TIM_DMABase_CCR3 02574 * @arg TIM_DMABase_CCR4 02575 * @arg TIM_DMABase_BDTR 02576 * @arg TIM_DMABase_DCR 02577 * @param TIM_DMABurstLength: DMA Burst length. This parameter can be one value 02578 * between: TIM_DMABurstLength_1Transfer and TIM_DMABurstLength_18Transfers. 02579 * @retval None 02580 */ 02581 void TIM_DMAConfig(TIM_TypeDef* TIMx, uint16_t TIM_DMABase, uint16_t TIM_DMABurstLength) 02582 { 02583 /* Check the parameters */ 02584 assert_param(IS_TIM_LIST3_PERIPH(TIMx)); 02585 assert_param(IS_TIM_DMA_BASE(TIM_DMABase)); 02586 assert_param(IS_TIM_DMA_LENGTH(TIM_DMABurstLength)); 02587 02588 /* Set the DMA Base and the DMA Burst Length */ 02589 TIMx->DCR = TIM_DMABase | TIM_DMABurstLength; 02590 } 02591 02592 /** 02593 * @brief Enables or disables the TIMx's DMA Requests. 02594 * @param TIMx: where x can be 1, 2, 3, 4, 5, 6, 7 or 8 to select the TIM peripheral. 02595 * @param TIM_DMASource: specifies the DMA Request sources. 02596 * This parameter can be any combination of the following values: 02597 * @arg TIM_DMA_Update: TIM update Interrupt source 02598 * @arg TIM_DMA_CC1: TIM Capture Compare 1 DMA source 02599 * @arg TIM_DMA_CC2: TIM Capture Compare 2 DMA source 02600 * @arg TIM_DMA_CC3: TIM Capture Compare 3 DMA source 02601 * @arg TIM_DMA_CC4: TIM Capture Compare 4 DMA source 02602 * @arg TIM_DMA_COM: TIM Commutation DMA source 02603 * @arg TIM_DMA_Trigger: TIM Trigger DMA source 02604 * @param NewState: new state of the DMA Request sources. 02605 * This parameter can be: ENABLE or DISABLE. 02606 * @retval None 02607 */ 02608 void TIM_DMACmd(TIM_TypeDef* TIMx, uint16_t TIM_DMASource, FunctionalState NewState) 02609 { 02610 /* Check the parameters */ 02611 assert_param(IS_TIM_LIST5_PERIPH(TIMx)); 02612 assert_param(IS_TIM_DMA_SOURCE(TIM_DMASource)); 02613 assert_param(IS_FUNCTIONAL_STATE(NewState)); 02614 02615 if (NewState != DISABLE) 02616 { 02617 /* Enable the DMA sources */ 02618 TIMx->DIER |= TIM_DMASource; 02619 } 02620 else 02621 { 02622 /* Disable the DMA sources */ 02623 TIMx->DIER &= (uint16_t)~TIM_DMASource; 02624 } 02625 } 02626 02627 /** 02628 * @brief Selects the TIMx peripheral Capture Compare DMA source. 02629 * @param TIMx: where x can be 1, 2, 3, 4, 5 or 8 to select the TIM peripheral. 02630 * @param NewState: new state of the Capture Compare DMA source 02631 * This parameter can be: ENABLE or DISABLE. 02632 * @retval None 02633 */ 02634 void TIM_SelectCCDMA(TIM_TypeDef* TIMx, FunctionalState NewState) 02635 { 02636 /* Check the parameters */ 02637 assert_param(IS_TIM_LIST3_PERIPH(TIMx)); 02638 assert_param(IS_FUNCTIONAL_STATE(NewState)); 02639 02640 if (NewState != DISABLE) 02641 { 02642 /* Set the CCDS Bit */ 02643 TIMx->CR2 |= TIM_CR2_CCDS; 02644 } 02645 else 02646 { 02647 /* Reset the CCDS Bit */ 02648 TIMx->CR2 &= (uint16_t)~TIM_CR2_CCDS; 02649 } 02650 } 02651 /** 02652 * @} 02653 */ 02654 02655 /** @defgroup TIM_Group6 Clocks management functions 02656 * @brief Clocks management functions 02657 * 02658 @verbatim 02659 =============================================================================== 02660 Clocks management functions 02661 =============================================================================== 02662 02663 @endverbatim 02664 * @{ 02665 */ 02666 02667 /** 02668 * @brief Configures the TIMx internal Clock 02669 * @param TIMx: where x can be 1, 2, 3, 4, 5, 8, 9 or 12 to select the TIM 02670 * peripheral. 02671 * @retval None 02672 */ 02673 void TIM_InternalClockConfig(TIM_TypeDef* TIMx) 02674 { 02675 /* Check the parameters */ 02676 assert_param(IS_TIM_LIST2_PERIPH(TIMx)); 02677 02678 /* Disable slave mode to clock the prescaler directly with the internal clock */ 02679 TIMx->SMCR &= (uint16_t)~TIM_SMCR_SMS; 02680 } 02681 02682 /** 02683 * @brief Configures the TIMx Internal Trigger as External Clock 02684 * @param TIMx: where x can be 1, 2, 3, 4, 5, 8, 9 or 12 to select the TIM 02685 * peripheral. 02686 * @param TIM_InputTriggerSource: Trigger source. 02687 * This parameter can be one of the following values: 02688 * @arg TIM_TS_ITR0: Internal Trigger 0 02689 * @arg TIM_TS_ITR1: Internal Trigger 1 02690 * @arg TIM_TS_ITR2: Internal Trigger 2 02691 * @arg TIM_TS_ITR3: Internal Trigger 3 02692 * @retval None 02693 */ 02694 void TIM_ITRxExternalClockConfig(TIM_TypeDef* TIMx, uint16_t TIM_InputTriggerSource) 02695 { 02696 /* Check the parameters */ 02697 assert_param(IS_TIM_LIST2_PERIPH(TIMx)); 02698 assert_param(IS_TIM_INTERNAL_TRIGGER_SELECTION(TIM_InputTriggerSource)); 02699 02700 /* Select the Internal Trigger */ 02701 TIM_SelectInputTrigger(TIMx, TIM_InputTriggerSource); 02702 02703 /* Select the External clock mode1 */ 02704 TIMx->SMCR |= TIM_SlaveMode_External1; 02705 } 02706 02707 /** 02708 * @brief Configures the TIMx Trigger as External Clock 02709 * @param TIMx: where x can be 1, 2, 3, 4, 5, 8, 9, 10, 11, 12, 13 or 14 02710 * to select the TIM peripheral. 02711 * @param TIM_TIxExternalCLKSource: Trigger source. 02712 * This parameter can be one of the following values: 02713 * @arg TIM_TIxExternalCLK1Source_TI1ED: TI1 Edge Detector 02714 * @arg TIM_TIxExternalCLK1Source_TI1: Filtered Timer Input 1 02715 * @arg TIM_TIxExternalCLK1Source_TI2: Filtered Timer Input 2 02716 * @param TIM_ICPolarity: specifies the TIx Polarity. 02717 * This parameter can be one of the following values: 02718 * @arg TIM_ICPolarity_Rising 02719 * @arg TIM_ICPolarity_Falling 02720 * @param ICFilter: specifies the filter value. 02721 * This parameter must be a value between 0x0 and 0xF. 02722 * @retval None 02723 */ 02724 void TIM_TIxExternalClockConfig(TIM_TypeDef* TIMx, uint16_t TIM_TIxExternalCLKSource, 02725 uint16_t TIM_ICPolarity, uint16_t ICFilter) 02726 { 02727 /* Check the parameters */ 02728 assert_param(IS_TIM_LIST1_PERIPH(TIMx)); 02729 assert_param(IS_TIM_IC_POLARITY(TIM_ICPolarity)); 02730 assert_param(IS_TIM_IC_FILTER(ICFilter)); 02731 02732 /* Configure the Timer Input Clock Source */ 02733 if (TIM_TIxExternalCLKSource == TIM_TIxExternalCLK1Source_TI2) 02734 { 02735 TI2_Config(TIMx, TIM_ICPolarity, TIM_ICSelection_DirectTI, ICFilter); 02736 } 02737 else 02738 { 02739 TI1_Config(TIMx, TIM_ICPolarity, TIM_ICSelection_DirectTI, ICFilter); 02740 } 02741 /* Select the Trigger source */ 02742 TIM_SelectInputTrigger(TIMx, TIM_TIxExternalCLKSource); 02743 /* Select the External clock mode1 */ 02744 TIMx->SMCR |= TIM_SlaveMode_External1; 02745 } 02746 02747 /** 02748 * @brief Configures the External clock Mode1 02749 * @param TIMx: where x can be 1, 2, 3, 4, 5 or 8 to select the TIM peripheral. 02750 * @param TIM_ExtTRGPrescaler: The external Trigger Prescaler. 02751 * This parameter can be one of the following values: 02752 * @arg TIM_ExtTRGPSC_OFF: ETRP Prescaler OFF. 02753 * @arg TIM_ExtTRGPSC_DIV2: ETRP frequency divided by 2. 02754 * @arg TIM_ExtTRGPSC_DIV4: ETRP frequency divided by 4. 02755 * @arg TIM_ExtTRGPSC_DIV8: ETRP frequency divided by 8. 02756 * @param TIM_ExtTRGPolarity: The external Trigger Polarity. 02757 * This parameter can be one of the following values: 02758 * @arg TIM_ExtTRGPolarity_Inverted: active low or falling edge active. 02759 * @arg TIM_ExtTRGPolarity_NonInverted: active high or rising edge active. 02760 * @param ExtTRGFilter: External Trigger Filter. 02761 * This parameter must be a value between 0x00 and 0x0F 02762 * @retval None 02763 */ 02764 void TIM_ETRClockMode1Config(TIM_TypeDef* TIMx, uint16_t TIM_ExtTRGPrescaler, 02765 uint16_t TIM_ExtTRGPolarity, uint16_t ExtTRGFilter) 02766 { 02767 uint16_t tmpsmcr = 0; 02768 02769 /* Check the parameters */ 02770 assert_param(IS_TIM_LIST3_PERIPH(TIMx)); 02771 assert_param(IS_TIM_EXT_PRESCALER(TIM_ExtTRGPrescaler)); 02772 assert_param(IS_TIM_EXT_POLARITY(TIM_ExtTRGPolarity)); 02773 assert_param(IS_TIM_EXT_FILTER(ExtTRGFilter)); 02774 /* Configure the ETR Clock source */ 02775 TIM_ETRConfig(TIMx, TIM_ExtTRGPrescaler, TIM_ExtTRGPolarity, ExtTRGFilter); 02776 02777 /* Get the TIMx SMCR register value */ 02778 tmpsmcr = TIMx->SMCR; 02779 02780 /* Reset the SMS Bits */ 02781 tmpsmcr &= (uint16_t)~TIM_SMCR_SMS; 02782 02783 /* Select the External clock mode1 */ 02784 tmpsmcr |= TIM_SlaveMode_External1; 02785 02786 /* Select the Trigger selection : ETRF */ 02787 tmpsmcr &= (uint16_t)~TIM_SMCR_TS; 02788 tmpsmcr |= TIM_TS_ETRF; 02789 02790 /* Write to TIMx SMCR */ 02791 TIMx->SMCR = tmpsmcr; 02792 } 02793 02794 /** 02795 * @brief Configures the External clock Mode2 02796 * @param TIMx: where x can be 1, 2, 3, 4, 5 or 8 to select the TIM peripheral. 02797 * @param TIM_ExtTRGPrescaler: The external Trigger Prescaler. 02798 * This parameter can be one of the following values: 02799 * @arg TIM_ExtTRGPSC_OFF: ETRP Prescaler OFF. 02800 * @arg TIM_ExtTRGPSC_DIV2: ETRP frequency divided by 2. 02801 * @arg TIM_ExtTRGPSC_DIV4: ETRP frequency divided by 4. 02802 * @arg TIM_ExtTRGPSC_DIV8: ETRP frequency divided by 8. 02803 * @param TIM_ExtTRGPolarity: The external Trigger Polarity. 02804 * This parameter can be one of the following values: 02805 * @arg TIM_ExtTRGPolarity_Inverted: active low or falling edge active. 02806 * @arg TIM_ExtTRGPolarity_NonInverted: active high or rising edge active. 02807 * @param ExtTRGFilter: External Trigger Filter. 02808 * This parameter must be a value between 0x00 and 0x0F 02809 * @retval None 02810 */ 02811 void TIM_ETRClockMode2Config(TIM_TypeDef* TIMx, uint16_t TIM_ExtTRGPrescaler, 02812 uint16_t TIM_ExtTRGPolarity, uint16_t ExtTRGFilter) 02813 { 02814 /* Check the parameters */ 02815 assert_param(IS_TIM_LIST3_PERIPH(TIMx)); 02816 assert_param(IS_TIM_EXT_PRESCALER(TIM_ExtTRGPrescaler)); 02817 assert_param(IS_TIM_EXT_POLARITY(TIM_ExtTRGPolarity)); 02818 assert_param(IS_TIM_EXT_FILTER(ExtTRGFilter)); 02819 02820 /* Configure the ETR Clock source */ 02821 TIM_ETRConfig(TIMx, TIM_ExtTRGPrescaler, TIM_ExtTRGPolarity, ExtTRGFilter); 02822 02823 /* Enable the External clock mode2 */ 02824 TIMx->SMCR |= TIM_SMCR_ECE; 02825 } 02826 /** 02827 * @} 02828 */ 02829 02830 /** @defgroup TIM_Group7 Synchronization management functions 02831 * @brief Synchronization management functions 02832 * 02833 @verbatim 02834 =============================================================================== 02835 Synchronization management functions 02836 =============================================================================== 02837 02838 =================================================================== 02839 TIM Driver: how to use it in synchronization Mode 02840 =================================================================== 02841 Case of two/several Timers 02842 ************************** 02843 1. Configure the Master Timers using the following functions: 02844 - void TIM_SelectOutputTrigger(TIM_TypeDef* TIMx, uint16_t TIM_TRGOSource); 02845 - void TIM_SelectMasterSlaveMode(TIM_TypeDef* TIMx, uint16_t TIM_MasterSlaveMode); 02846 2. Configure the Slave Timers using the following functions: 02847 - void TIM_SelectInputTrigger(TIM_TypeDef* TIMx, uint16_t TIM_InputTriggerSource); 02848 - void TIM_SelectSlaveMode(TIM_TypeDef* TIMx, uint16_t TIM_SlaveMode); 02849 02850 Case of Timers and external trigger(ETR pin) 02851 ******************************************** 02852 1. Configure the External trigger using this function: 02853 - void TIM_ETRConfig(TIM_TypeDef* TIMx, uint16_t TIM_ExtTRGPrescaler, uint16_t TIM_ExtTRGPolarity, 02854 uint16_t ExtTRGFilter); 02855 2. Configure the Slave Timers using the following functions: 02856 - void TIM_SelectInputTrigger(TIM_TypeDef* TIMx, uint16_t TIM_InputTriggerSource); 02857 - void TIM_SelectSlaveMode(TIM_TypeDef* TIMx, uint16_t TIM_SlaveMode); 02858 02859 @endverbatim 02860 * @{ 02861 */ 02862 02863 /** 02864 * @brief Selects the Input Trigger source 02865 * @param TIMx: where x can be 1, 2, 3, 4, 5, 8, 9, 10, 11, 12, 13 or 14 02866 * to select the TIM peripheral. 02867 * @param TIM_InputTriggerSource: The Input Trigger source. 02868 * This parameter can be one of the following values: 02869 * @arg TIM_TS_ITR0: Internal Trigger 0 02870 * @arg TIM_TS_ITR1: Internal Trigger 1 02871 * @arg TIM_TS_ITR2: Internal Trigger 2 02872 * @arg TIM_TS_ITR3: Internal Trigger 3 02873 * @arg TIM_TS_TI1F_ED: TI1 Edge Detector 02874 * @arg TIM_TS_TI1FP1: Filtered Timer Input 1 02875 * @arg TIM_TS_TI2FP2: Filtered Timer Input 2 02876 * @arg TIM_TS_ETRF: External Trigger input 02877 * @retval None 02878 */ 02879 void TIM_SelectInputTrigger(TIM_TypeDef* TIMx, uint16_t TIM_InputTriggerSource) 02880 { 02881 uint16_t tmpsmcr = 0; 02882 02883 /* Check the parameters */ 02884 assert_param(IS_TIM_LIST1_PERIPH(TIMx)); 02885 assert_param(IS_TIM_TRIGGER_SELECTION(TIM_InputTriggerSource)); 02886 02887 /* Get the TIMx SMCR register value */ 02888 tmpsmcr = TIMx->SMCR; 02889 02890 /* Reset the TS Bits */ 02891 tmpsmcr &= (uint16_t)~TIM_SMCR_TS; 02892 02893 /* Set the Input Trigger source */ 02894 tmpsmcr |= TIM_InputTriggerSource; 02895 02896 /* Write to TIMx SMCR */ 02897 TIMx->SMCR = tmpsmcr; 02898 } 02899 02900 /** 02901 * @brief Selects the TIMx Trigger Output Mode. 02902 * @param TIMx: where x can be 1, 2, 3, 4, 5, 6, 7 or 8 to select the TIM peripheral. 02903 * 02904 * @param TIM_TRGOSource: specifies the Trigger Output source. 02905 * This parameter can be one of the following values: 02906 * 02907 * - For all TIMx 02908 * @arg TIM_TRGOSource_Reset: The UG bit in the TIM_EGR register is used as the trigger output(TRGO) 02909 * @arg TIM_TRGOSource_Enable: The Counter Enable CEN is used as the trigger output(TRGO) 02910 * @arg TIM_TRGOSource_Update: The update event is selected as the trigger output(TRGO) 02911 * 02912 * - For all TIMx except TIM6 and TIM7 02913 * @arg TIM_TRGOSource_OC1: The trigger output sends a positive pulse when the CC1IF flag 02914 * is to be set, as soon as a capture or compare match occurs(TRGO) 02915 * @arg TIM_TRGOSource_OC1Ref: OC1REF signal is used as the trigger output(TRGO) 02916 * @arg TIM_TRGOSource_OC2Ref: OC2REF signal is used as the trigger output(TRGO) 02917 * @arg TIM_TRGOSource_OC3Ref: OC3REF signal is used as the trigger output(TRGO) 02918 * @arg TIM_TRGOSource_OC4Ref: OC4REF signal is used as the trigger output(TRGO) 02919 * 02920 * @retval None 02921 */ 02922 void TIM_SelectOutputTrigger(TIM_TypeDef* TIMx, uint16_t TIM_TRGOSource) 02923 { 02924 /* Check the parameters */ 02925 assert_param(IS_TIM_LIST5_PERIPH(TIMx)); 02926 assert_param(IS_TIM_TRGO_SOURCE(TIM_TRGOSource)); 02927 02928 /* Reset the MMS Bits */ 02929 TIMx->CR2 &= (uint16_t)~TIM_CR2_MMS; 02930 /* Select the TRGO source */ 02931 TIMx->CR2 |= TIM_TRGOSource; 02932 } 02933 02934 /** 02935 * @brief Selects the TIMx Slave Mode. 02936 * @param TIMx: where x can be 1, 2, 3, 4, 5, 8, 9 or 12 to select the TIM peripheral. 02937 * @param TIM_SlaveMode: specifies the Timer Slave Mode. 02938 * This parameter can be one of the following values: 02939 * @arg TIM_SlaveMode_Reset: Rising edge of the selected trigger signal(TRGI) reinitialize 02940 * the counter and triggers an update of the registers 02941 * @arg TIM_SlaveMode_Gated: The counter clock is enabled when the trigger signal (TRGI) is high 02942 * @arg TIM_SlaveMode_Trigger: The counter starts at a rising edge of the trigger TRGI 02943 * @arg TIM_SlaveMode_External1: Rising edges of the selected trigger (TRGI) clock the counter 02944 * @retval None 02945 */ 02946 void TIM_SelectSlaveMode(TIM_TypeDef* TIMx, uint16_t TIM_SlaveMode) 02947 { 02948 /* Check the parameters */ 02949 assert_param(IS_TIM_LIST2_PERIPH(TIMx)); 02950 assert_param(IS_TIM_SLAVE_MODE(TIM_SlaveMode)); 02951 02952 /* Reset the SMS Bits */ 02953 TIMx->SMCR &= (uint16_t)~TIM_SMCR_SMS; 02954 02955 /* Select the Slave Mode */ 02956 TIMx->SMCR |= TIM_SlaveMode; 02957 } 02958 02959 /** 02960 * @brief Sets or Resets the TIMx Master/Slave Mode. 02961 * @param TIMx: where x can be 1, 2, 3, 4, 5, 8, 9 or 12 to select the TIM peripheral. 02962 * @param TIM_MasterSlaveMode: specifies the Timer Master Slave Mode. 02963 * This parameter can be one of the following values: 02964 * @arg TIM_MasterSlaveMode_Enable: synchronization between the current timer 02965 * and its slaves (through TRGO) 02966 * @arg TIM_MasterSlaveMode_Disable: No action 02967 * @retval None 02968 */ 02969 void TIM_SelectMasterSlaveMode(TIM_TypeDef* TIMx, uint16_t TIM_MasterSlaveMode) 02970 { 02971 /* Check the parameters */ 02972 assert_param(IS_TIM_LIST2_PERIPH(TIMx)); 02973 assert_param(IS_TIM_MSM_STATE(TIM_MasterSlaveMode)); 02974 02975 /* Reset the MSM Bit */ 02976 TIMx->SMCR &= (uint16_t)~TIM_SMCR_MSM; 02977 02978 /* Set or Reset the MSM Bit */ 02979 TIMx->SMCR |= TIM_MasterSlaveMode; 02980 } 02981 02982 /** 02983 * @brief Configures the TIMx External Trigger (ETR). 02984 * @param TIMx: where x can be 1, 2, 3, 4, 5 or 8 to select the TIM peripheral. 02985 * @param TIM_ExtTRGPrescaler: The external Trigger Prescaler. 02986 * This parameter can be one of the following values: 02987 * @arg TIM_ExtTRGPSC_OFF: ETRP Prescaler OFF. 02988 * @arg TIM_ExtTRGPSC_DIV2: ETRP frequency divided by 2. 02989 * @arg TIM_ExtTRGPSC_DIV4: ETRP frequency divided by 4. 02990 * @arg TIM_ExtTRGPSC_DIV8: ETRP frequency divided by 8. 02991 * @param TIM_ExtTRGPolarity: The external Trigger Polarity. 02992 * This parameter can be one of the following values: 02993 * @arg TIM_ExtTRGPolarity_Inverted: active low or falling edge active. 02994 * @arg TIM_ExtTRGPolarity_NonInverted: active high or rising edge active. 02995 * @param ExtTRGFilter: External Trigger Filter. 02996 * This parameter must be a value between 0x00 and 0x0F 02997 * @retval None 02998 */ 02999 void TIM_ETRConfig(TIM_TypeDef* TIMx, uint16_t TIM_ExtTRGPrescaler, 03000 uint16_t TIM_ExtTRGPolarity, uint16_t ExtTRGFilter) 03001 { 03002 uint16_t tmpsmcr = 0; 03003 03004 /* Check the parameters */ 03005 assert_param(IS_TIM_LIST3_PERIPH(TIMx)); 03006 assert_param(IS_TIM_EXT_PRESCALER(TIM_ExtTRGPrescaler)); 03007 assert_param(IS_TIM_EXT_POLARITY(TIM_ExtTRGPolarity)); 03008 assert_param(IS_TIM_EXT_FILTER(ExtTRGFilter)); 03009 03010 tmpsmcr = TIMx->SMCR; 03011 03012 /* Reset the ETR Bits */ 03013 tmpsmcr &= SMCR_ETR_MASK; 03014 03015 /* Set the Prescaler, the Filter value and the Polarity */ 03016 tmpsmcr |= (uint16_t)(TIM_ExtTRGPrescaler | (uint16_t)(TIM_ExtTRGPolarity | (uint16_t)(ExtTRGFilter << (uint16_t)8))); 03017 03018 /* Write to TIMx SMCR */ 03019 TIMx->SMCR = tmpsmcr; 03020 } 03021 /** 03022 * @} 03023 */ 03024 03025 /** @defgroup TIM_Group8 Specific interface management functions 03026 * @brief Specific interface management functions 03027 * 03028 @verbatim 03029 =============================================================================== 03030 Specific interface management functions 03031 =============================================================================== 03032 03033 @endverbatim 03034 * @{ 03035 */ 03036 03037 /** 03038 * @brief Configures the TIMx Encoder Interface. 03039 * @param TIMx: where x can be 1, 2, 3, 4, 5, 8, 9 or 12 to select the TIM 03040 * peripheral. 03041 * @param TIM_EncoderMode: specifies the TIMx Encoder Mode. 03042 * This parameter can be one of the following values: 03043 * @arg TIM_EncoderMode_TI1: Counter counts on TI1FP1 edge depending on TI2FP2 level. 03044 * @arg TIM_EncoderMode_TI2: Counter counts on TI2FP2 edge depending on TI1FP1 level. 03045 * @arg TIM_EncoderMode_TI12: Counter counts on both TI1FP1 and TI2FP2 edges depending 03046 * on the level of the other input. 03047 * @param TIM_IC1Polarity: specifies the IC1 Polarity 03048 * This parameter can be one of the following values: 03049 * @arg TIM_ICPolarity_Falling: IC Falling edge. 03050 * @arg TIM_ICPolarity_Rising: IC Rising edge. 03051 * @param TIM_IC2Polarity: specifies the IC2 Polarity 03052 * This parameter can be one of the following values: 03053 * @arg TIM_ICPolarity_Falling: IC Falling edge. 03054 * @arg TIM_ICPolarity_Rising: IC Rising edge. 03055 * @retval None 03056 */ 03057 void TIM_EncoderInterfaceConfig(TIM_TypeDef* TIMx, uint16_t TIM_EncoderMode, 03058 uint16_t TIM_IC1Polarity, uint16_t TIM_IC2Polarity) 03059 { 03060 uint16_t tmpsmcr = 0; 03061 uint16_t tmpccmr1 = 0; 03062 uint16_t tmpccer = 0; 03063 03064 /* Check the parameters */ 03065 assert_param(IS_TIM_LIST2_PERIPH(TIMx)); 03066 assert_param(IS_TIM_ENCODER_MODE(TIM_EncoderMode)); 03067 assert_param(IS_TIM_IC_POLARITY(TIM_IC1Polarity)); 03068 assert_param(IS_TIM_IC_POLARITY(TIM_IC2Polarity)); 03069 03070 /* Get the TIMx SMCR register value */ 03071 tmpsmcr = TIMx->SMCR; 03072 03073 /* Get the TIMx CCMR1 register value */ 03074 tmpccmr1 = TIMx->CCMR1; 03075 03076 /* Get the TIMx CCER register value */ 03077 tmpccer = TIMx->CCER; 03078 03079 /* Set the encoder Mode */ 03080 tmpsmcr &= (uint16_t)~TIM_SMCR_SMS; 03081 tmpsmcr |= TIM_EncoderMode; 03082 03083 /* Select the Capture Compare 1 and the Capture Compare 2 as input */ 03084 tmpccmr1 &= ((uint16_t)~TIM_CCMR1_CC1S) & ((uint16_t)~TIM_CCMR1_CC2S); 03085 tmpccmr1 |= TIM_CCMR1_CC1S_0 | TIM_CCMR1_CC2S_0; 03086 03087 /* Set the TI1 and the TI2 Polarities */ 03088 tmpccer &= ((uint16_t)~TIM_CCER_CC1P) & ((uint16_t)~TIM_CCER_CC2P); 03089 tmpccer |= (uint16_t)(TIM_IC1Polarity | (uint16_t)(TIM_IC2Polarity << (uint16_t)4)); 03090 03091 /* Write to TIMx SMCR */ 03092 TIMx->SMCR = tmpsmcr; 03093 03094 /* Write to TIMx CCMR1 */ 03095 TIMx->CCMR1 = tmpccmr1; 03096 03097 /* Write to TIMx CCER */ 03098 TIMx->CCER = tmpccer; 03099 } 03100 03101 /** 03102 * @brief Enables or disables the TIMx's Hall sensor interface. 03103 * @param TIMx: where x can be 1, 2, 3, 4, 5, 8, 9 or 12 to select the TIM 03104 * peripheral. 03105 * @param NewState: new state of the TIMx Hall sensor interface. 03106 * This parameter can be: ENABLE or DISABLE. 03107 * @retval None 03108 */ 03109 void TIM_SelectHallSensor(TIM_TypeDef* TIMx, FunctionalState NewState) 03110 { 03111 /* Check the parameters */ 03112 assert_param(IS_TIM_LIST2_PERIPH(TIMx)); 03113 assert_param(IS_FUNCTIONAL_STATE(NewState)); 03114 03115 if (NewState != DISABLE) 03116 { 03117 /* Set the TI1S Bit */ 03118 TIMx->CR2 |= TIM_CR2_TI1S; 03119 } 03120 else 03121 { 03122 /* Reset the TI1S Bit */ 03123 TIMx->CR2 &= (uint16_t)~TIM_CR2_TI1S; 03124 } 03125 } 03126 /** 03127 * @} 03128 */ 03129 03130 /** @defgroup TIM_Group9 Specific remapping management function 03131 * @brief Specific remapping management function 03132 * 03133 @verbatim 03134 =============================================================================== 03135 Specific remapping management function 03136 =============================================================================== 03137 03138 @endverbatim 03139 * @{ 03140 */ 03141 03142 /** 03143 * @brief Configures the TIM2, TIM5 and TIM11 Remapping input capabilities. 03144 * @param TIMx: where x can be 2, 5 or 11 to select the TIM peripheral. 03145 * @param TIM_Remap: specifies the TIM input remapping source. 03146 * This parameter can be one of the following values: 03147 * @arg TIM2_TIM8_TRGO: TIM2 ITR1 input is connected to TIM8 Trigger output(default) 03148 * @arg TIM2_ETH_PTP: TIM2 ITR1 input is connected to ETH PTP trogger output. 03149 * @arg TIM2_USBFS_SOF: TIM2 ITR1 input is connected to USB FS SOF. 03150 * @arg TIM2_USBHS_SOF: TIM2 ITR1 input is connected to USB HS SOF. 03151 * @arg TIM5_GPIO: TIM5 CH4 input is connected to dedicated Timer pin(default) 03152 * @arg TIM5_LSI: TIM5 CH4 input is connected to LSI clock. 03153 * @arg TIM5_LSE: TIM5 CH4 input is connected to LSE clock. 03154 * @arg TIM5_RTC: TIM5 CH4 input is connected to RTC Output event. 03155 * @arg TIM11_GPIO: TIM11 CH4 input is connected to dedicated Timer pin(default) 03156 * @arg TIM11_HSE: TIM11 CH4 input is connected to HSE_RTC clock 03157 * (HSE divided by a programmable prescaler) 03158 * @retval None 03159 */ 03160 void TIM_RemapConfig(TIM_TypeDef* TIMx, uint16_t TIM_Remap) 03161 { 03162 /* Check the parameters */ 03163 assert_param(IS_TIM_LIST6_PERIPH(TIMx)); 03164 assert_param(IS_TIM_REMAP(TIM_Remap)); 03165 03166 /* Set the Timer remapping configuration */ 03167 TIMx->OR = TIM_Remap; 03168 } 03169 /** 03170 * @} 03171 */ 03172 03173 /** 03174 * @brief Configure the TI1 as Input. 03175 * @param TIMx: where x can be 1, 2, 3, 4, 5, 8, 9, 10, 11, 12, 13 or 14 03176 * to select the TIM peripheral. 03177 * @param TIM_ICPolarity : The Input Polarity. 03178 * This parameter can be one of the following values: 03179 * @arg TIM_ICPolarity_Rising 03180 * @arg TIM_ICPolarity_Falling 03181 * @arg TIM_ICPolarity_BothEdge 03182 * @param TIM_ICSelection: specifies the input to be used. 03183 * This parameter can be one of the following values: 03184 * @arg TIM_ICSelection_DirectTI: TIM Input 1 is selected to be connected to IC1. 03185 * @arg TIM_ICSelection_IndirectTI: TIM Input 1 is selected to be connected to IC2. 03186 * @arg TIM_ICSelection_TRC: TIM Input 1 is selected to be connected to TRC. 03187 * @param TIM_ICFilter: Specifies the Input Capture Filter. 03188 * This parameter must be a value between 0x00 and 0x0F. 03189 * @retval None 03190 */ 03191 static void TI1_Config(TIM_TypeDef* TIMx, uint16_t TIM_ICPolarity, uint16_t TIM_ICSelection, 03192 uint16_t TIM_ICFilter) 03193 { 03194 uint16_t tmpccmr1 = 0, tmpccer = 0; 03195 03196 /* Disable the Channel 1: Reset the CC1E Bit */ 03197 TIMx->CCER &= (uint16_t)~TIM_CCER_CC1E; 03198 tmpccmr1 = TIMx->CCMR1; 03199 tmpccer = TIMx->CCER; 03200 03201 /* Select the Input and set the filter */ 03202 tmpccmr1 &= ((uint16_t)~TIM_CCMR1_CC1S) & ((uint16_t)~TIM_CCMR1_IC1F); 03203 tmpccmr1 |= (uint16_t)(TIM_ICSelection | (uint16_t)(TIM_ICFilter << (uint16_t)4)); 03204 03205 /* Select the Polarity and set the CC1E Bit */ 03206 tmpccer &= (uint16_t)~(TIM_CCER_CC1P | TIM_CCER_CC1NP); 03207 tmpccer |= (uint16_t)(TIM_ICPolarity | (uint16_t)TIM_CCER_CC1E); 03208 03209 /* Write to TIMx CCMR1 and CCER registers */ 03210 TIMx->CCMR1 = tmpccmr1; 03211 TIMx->CCER = tmpccer; 03212 } 03213 03214 /** 03215 * @brief Configure the TI2 as Input. 03216 * @param TIMx: where x can be 1, 2, 3, 4, 5, 8, 9 or 12 to select the TIM 03217 * peripheral. 03218 * @param TIM_ICPolarity : The Input Polarity. 03219 * This parameter can be one of the following values: 03220 * @arg TIM_ICPolarity_Rising 03221 * @arg TIM_ICPolarity_Falling 03222 * @arg TIM_ICPolarity_BothEdge 03223 * @param TIM_ICSelection: specifies the input to be used. 03224 * This parameter can be one of the following values: 03225 * @arg TIM_ICSelection_DirectTI: TIM Input 2 is selected to be connected to IC2. 03226 * @arg TIM_ICSelection_IndirectTI: TIM Input 2 is selected to be connected to IC1. 03227 * @arg TIM_ICSelection_TRC: TIM Input 2 is selected to be connected to TRC. 03228 * @param TIM_ICFilter: Specifies the Input Capture Filter. 03229 * This parameter must be a value between 0x00 and 0x0F. 03230 * @retval None 03231 */ 03232 static void TI2_Config(TIM_TypeDef* TIMx, uint16_t TIM_ICPolarity, uint16_t TIM_ICSelection, 03233 uint16_t TIM_ICFilter) 03234 { 03235 uint16_t tmpccmr1 = 0, tmpccer = 0, tmp = 0; 03236 03237 /* Disable the Channel 2: Reset the CC2E Bit */ 03238 TIMx->CCER &= (uint16_t)~TIM_CCER_CC2E; 03239 tmpccmr1 = TIMx->CCMR1; 03240 tmpccer = TIMx->CCER; 03241 tmp = (uint16_t)(TIM_ICPolarity << 4); 03242 03243 /* Select the Input and set the filter */ 03244 tmpccmr1 &= ((uint16_t)~TIM_CCMR1_CC2S) & ((uint16_t)~TIM_CCMR1_IC2F); 03245 tmpccmr1 |= (uint16_t)(TIM_ICFilter << 12); 03246 tmpccmr1 |= (uint16_t)(TIM_ICSelection << 8); 03247 03248 /* Select the Polarity and set the CC2E Bit */ 03249 tmpccer &= (uint16_t)~(TIM_CCER_CC2P | TIM_CCER_CC2NP); 03250 tmpccer |= (uint16_t)(tmp | (uint16_t)TIM_CCER_CC2E); 03251 03252 /* Write to TIMx CCMR1 and CCER registers */ 03253 TIMx->CCMR1 = tmpccmr1 ; 03254 TIMx->CCER = tmpccer; 03255 } 03256 03257 /** 03258 * @brief Configure the TI3 as Input. 03259 * @param TIMx: where x can be 1, 2, 3, 4, 5 or 8 to select the TIM peripheral. 03260 * @param TIM_ICPolarity : The Input Polarity. 03261 * This parameter can be one of the following values: 03262 * @arg TIM_ICPolarity_Rising 03263 * @arg TIM_ICPolarity_Falling 03264 * @arg TIM_ICPolarity_BothEdge 03265 * @param TIM_ICSelection: specifies the input to be used. 03266 * This parameter can be one of the following values: 03267 * @arg TIM_ICSelection_DirectTI: TIM Input 3 is selected to be connected to IC3. 03268 * @arg TIM_ICSelection_IndirectTI: TIM Input 3 is selected to be connected to IC4. 03269 * @arg TIM_ICSelection_TRC: TIM Input 3 is selected to be connected to TRC. 03270 * @param TIM_ICFilter: Specifies the Input Capture Filter. 03271 * This parameter must be a value between 0x00 and 0x0F. 03272 * @retval None 03273 */ 03274 static void TI3_Config(TIM_TypeDef* TIMx, uint16_t TIM_ICPolarity, uint16_t TIM_ICSelection, 03275 uint16_t TIM_ICFilter) 03276 { 03277 uint16_t tmpccmr2 = 0, tmpccer = 0, tmp = 0; 03278 03279 /* Disable the Channel 3: Reset the CC3E Bit */ 03280 TIMx->CCER &= (uint16_t)~TIM_CCER_CC3E; 03281 tmpccmr2 = TIMx->CCMR2; 03282 tmpccer = TIMx->CCER; 03283 tmp = (uint16_t)(TIM_ICPolarity << 8); 03284 03285 /* Select the Input and set the filter */ 03286 tmpccmr2 &= ((uint16_t)~TIM_CCMR1_CC1S) & ((uint16_t)~TIM_CCMR2_IC3F); 03287 tmpccmr2 |= (uint16_t)(TIM_ICSelection | (uint16_t)(TIM_ICFilter << (uint16_t)4)); 03288 03289 /* Select the Polarity and set the CC3E Bit */ 03290 tmpccer &= (uint16_t)~(TIM_CCER_CC3P | TIM_CCER_CC3NP); 03291 tmpccer |= (uint16_t)(tmp | (uint16_t)TIM_CCER_CC3E); 03292 03293 /* Write to TIMx CCMR2 and CCER registers */ 03294 TIMx->CCMR2 = tmpccmr2; 03295 TIMx->CCER = tmpccer; 03296 } 03297 03298 /** 03299 * @brief Configure the TI4 as Input. 03300 * @param TIMx: where x can be 1, 2, 3, 4, 5 or 8 to select the TIM peripheral. 03301 * @param TIM_ICPolarity : The Input Polarity. 03302 * This parameter can be one of the following values: 03303 * @arg TIM_ICPolarity_Rising 03304 * @arg TIM_ICPolarity_Falling 03305 * @arg TIM_ICPolarity_BothEdge 03306 * @param TIM_ICSelection: specifies the input to be used. 03307 * This parameter can be one of the following values: 03308 * @arg TIM_ICSelection_DirectTI: TIM Input 4 is selected to be connected to IC4. 03309 * @arg TIM_ICSelection_IndirectTI: TIM Input 4 is selected to be connected to IC3. 03310 * @arg TIM_ICSelection_TRC: TIM Input 4 is selected to be connected to TRC. 03311 * @param TIM_ICFilter: Specifies the Input Capture Filter. 03312 * This parameter must be a value between 0x00 and 0x0F. 03313 * @retval None 03314 */ 03315 static void TI4_Config(TIM_TypeDef* TIMx, uint16_t TIM_ICPolarity, uint16_t TIM_ICSelection, 03316 uint16_t TIM_ICFilter) 03317 { 03318 uint16_t tmpccmr2 = 0, tmpccer = 0, tmp = 0; 03319 03320 /* Disable the Channel 4: Reset the CC4E Bit */ 03321 TIMx->CCER &= (uint16_t)~TIM_CCER_CC4E; 03322 tmpccmr2 = TIMx->CCMR2; 03323 tmpccer = TIMx->CCER; 03324 tmp = (uint16_t)(TIM_ICPolarity << 12); 03325 03326 /* Select the Input and set the filter */ 03327 tmpccmr2 &= ((uint16_t)~TIM_CCMR1_CC2S) & ((uint16_t)~TIM_CCMR1_IC2F); 03328 tmpccmr2 |= (uint16_t)(TIM_ICSelection << 8); 03329 tmpccmr2 |= (uint16_t)(TIM_ICFilter << 12); 03330 03331 /* Select the Polarity and set the CC4E Bit */ 03332 tmpccer &= (uint16_t)~(TIM_CCER_CC4P | TIM_CCER_CC4NP); 03333 tmpccer |= (uint16_t)(tmp | (uint16_t)TIM_CCER_CC4E); 03334 03335 /* Write to TIMx CCMR2 and CCER registers */ 03336 TIMx->CCMR2 = tmpccmr2; 03337 TIMx->CCER = tmpccer ; 03338 } 03339 03340 /** 03341 * @} 03342 */ 03343 03344 /** 03345 * @} 03346 */ 03347 03348 /** 03349 * @} 03350 */ 03351 03352 /******************* (C) COPYRIGHT 2011 STMicroelectronics *****END OF FILE****/ 03353
Generated on Tue Jul 12 2022 19:53:13 by
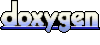