i2c RGBW led driver push/pull or opendrain output
Fork of PCA9633 by
PCA9633 Class Reference
#include <PCA9633.h>
Public Member Functions | |
PCA9633 (I2C *i2c, char addr=PCA9633::I2C_ADDR, bool invert=false, bool openDrain=false) | |
Create PCA9633 instance + config method. | |
void | config (bool invert, bool openDrain) |
Configure. | |
void | softReset (void) |
Soft Reset ALL PCA9633 on I2C bus. | |
void | pwm (char bright, char pos=PCA9633::ALL) |
Set PWM value for 1 or all LED. | |
void | dim (char val) |
Set general dimmer (if ledout = single | group) Disable BLINK. | |
void | blink (char duty, float period) |
Blinking led (if ledout = single | group) Disable DIMMER. | |
void | ledout (char state=PCA9633::GROUP, char pos=PCA9633::ALL) |
Set output state. |
Detailed Description
PCA9633 class.
PCA9633 4-bit Fm+ I2C-bus LED driver. This driver ignore !OE pin functionality. This driver disable i2c LED All Call address. This driver disable i2c SUB addresing.
Example:
#include "mbed.h" #include "PCA9633.h" I2C smbus(PB_7,PB_6); PCA9633 leds(&smbus); int main() { leds.config(true, true); //The output config was openDrain and inverted leds.pwm(32,0); //LED0 12.5% leds.pwm(64,1); //LED1 25% leds.pwm(128,2); //LED2 50% leds.pwm(255,3); //LED3 100% leds.ledout(PCA9633::GROUP) //All led was dim/blink and single configurable led.blink(64,1.5) //Leds blink each 1.5s with 25% duty cycle while(1) { } }
Definition at line 33 of file PCA9633.h.
Constructor & Destructor Documentation
PCA9633 | ( | I2C * | i2c, |
char | addr = PCA9633::I2C_ADDR , |
||
bool | invert = false , |
||
bool | openDrain = false |
||
) |
Create PCA9633 instance + config method.
- Parameters:
-
I2C initialized I2C bus to use addr I2C address default 0x62 invert invert output state output was opendrain or push/pull
Definition at line 27 of file PCA9633.cpp.
Member Function Documentation
void blink | ( | char | duty, |
float | period | ||
) |
Blinking led (if ledout = single | group) Disable DIMMER.
- Parameters:
-
duty <0,255> duty cycle period <0.041,10.73> is seconds
Definition at line 77 of file PCA9633.cpp.
void config | ( | bool | invert, |
bool | openDrain | ||
) |
Configure.
- Parameters:
-
invert invert output state output was opendrain or push/pull
Definition at line 40 of file PCA9633.cpp.
void dim | ( | char | val ) |
Set general dimmer (if ledout = single | group) Disable BLINK.
- Parameters:
-
val slowest pwm superimposed on led pwm
Definition at line 65 of file PCA9633.cpp.
void ledout | ( | char | state = PCA9633::GROUP , |
char | pos = PCA9633::ALL |
||
) |
Set output state.
- Parameters:
-
state off, full(on, nopwm), single(pwm, no dim|blink), group(pwm+dim|blink) pos select output<0,3> (all if >3)
Definition at line 91 of file PCA9633.cpp.
void pwm | ( | char | bright, |
char | pos = PCA9633::ALL |
||
) |
Set PWM value for 1 or all LED.
- Parameters:
-
bright <0,255> duty cycle pos select output<0,3> (all if >3)
Definition at line 55 of file PCA9633.cpp.
void softReset | ( | void | ) |
Soft Reset ALL PCA9633 on I2C bus.
Definition at line 50 of file PCA9633.cpp.
Generated on Thu Jul 21 2022 07:29:28 by
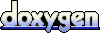