i2c RGBW led driver push/pull or opendrain output
Fork of PCA9633 by
PCA9633.h
00001 #ifndef PCA9633_H 00002 #define PCA9633_H 00003 00004 #include "mbed.h" 00005 /** PCA9633 class. 00006 * PCA9633 4-bit Fm+ I2C-bus LED driver. 00007 * This driver ignore !OE pin functionality. 00008 * This driver disable i2c LED All Call address. 00009 * This driver disable i2c SUB addresing. 00010 * 00011 * Example: 00012 * @code 00013 * #include "mbed.h" 00014 * #include "PCA9633.h" 00015 * I2C smbus(PB_7,PB_6); 00016 * 00017 * PCA9633 leds(&smbus); 00018 * 00019 * int main() { 00020 * leds.config(true, true); //The output config was openDrain and inverted 00021 * leds.pwm(32,0); //LED0 12.5% 00022 * leds.pwm(64,1); //LED1 25% 00023 * leds.pwm(128,2); //LED2 50% 00024 * leds.pwm(255,3); //LED3 100% 00025 * leds.ledout(PCA9633::GROUP) //All led was dim/blink and single configurable 00026 * led.blink(64,1.5) //Leds blink each 1.5s with 25% duty cycle 00027 * 00028 * while(1) { 00029 * } 00030 * } 00031 * @endcode 00032 */ 00033 class PCA9633{ 00034 public: 00035 00036 enum state{ 00037 OFF = 0, //LED driver is off (default power-up state) 00038 FULL = 1, //LED driver is fully on 00039 SINGLE = 2, //LED driver individual brightness can be controlled 00040 //through its PWMx register. 00041 GROUP = 3, //LED driver individual brightness and group 00042 //dimming/blinking can be controlled through its 00043 //PWMx register and the PCA9633_GRPPWM registers. 00044 ALL = 4, //Select all LED for pos 00045 I2C_ADDR = 0x62 //Base I2C address 00046 }; 00047 /** Create PCA9633 instance + config method 00048 * @param I2C initialized I2C bus to use 00049 * @param addr I2C address default 0x62 00050 * @param invert invert output state 00051 * @param output was opendrain or push/pull 00052 */ 00053 PCA9633(I2C *i2c, char addr=PCA9633::I2C_ADDR, bool invert=false, bool openDrain=false); 00054 00055 /** Configure 00056 * @param invert invert output state 00057 * @param output was opendrain or push/pull 00058 */ 00059 void config(bool invert, bool openDrain); 00060 00061 /** Soft Reset ALL PCA9633 on I2C bus 00062 */ 00063 void softReset(void); 00064 00065 00066 /** Set PWM value for 1 or all LED 00067 * @param bright <0,255> duty cycle 00068 * @param pos select output<0,3> (all if >3) 00069 */ 00070 void pwm(char bright, char pos=PCA9633::ALL); 00071 00072 /** Set general dimmer (if ledout = single | group) 00073 * Disable BLINK 00074 * @param val slowest pwm superimposed on led pwm 00075 */ 00076 void dim(char val); 00077 00078 /** Blinking led (if ledout = single | group) 00079 * Disable DIMMER 00080 * @param duty <0,255> duty cycle 00081 * @param period <0.041,10.73> is seconds 00082 */ 00083 void blink(char duty, float period); 00084 00085 /** Set output state 00086 * @param state off, full(on, nopwm), single(pwm, no dim|blink), group(pwm+dim|blink) 00087 * @param pos select output<0,3> (all if >3) 00088 */ 00089 void ledout(char state=PCA9633::GROUP, char pos=PCA9633::ALL); 00090 00091 private: 00092 I2C *_i2c; 00093 char _addr; 00094 }; 00095 00096 #endif
Generated on Thu Jul 21 2022 07:29:28 by
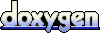