Official mbed Real Time Operating System based on the RTX implementation of the CMSIS-RTOS API open standard.
Dependents: denki-yohou_b TestY201 Network-RTOS NTPClient_HelloWorld ... more
Rtos
Data Structures | |
class | Mail< T, queue_sz > |
The Mail class allow to control, send, receive, or wait for mail. More... | |
class | MemoryPool< T, pool_sz > |
Define and manage fixed-size memory pools of objects of a given type. More... | |
class | Mutex |
The Mutex class is used to synchronise the execution of threads. More... | |
class | Queue< T, queue_sz > |
The Queue class allow to control, send, receive, or wait for messages. More... | |
class | RtosTimer |
The RtosTimer class allow creating and and controlling of timer functions in the system. More... | |
class | Semaphore |
The Semaphore class is used to manage and protect access to a set of shared resources. More... | |
class | Thread |
The Thread class allow defining, creating, and controlling thread functions in the system. More... | |
Enumerations | |
enum | State { Inactive, Ready, Running, WaitingDelay, WaitingInterval, WaitingOr, WaitingAnd, WaitingSemaphore, WaitingMailbox, WaitingMutex, Deleted } |
State of the Thread. More... | |
Functions | |
T * | alloc (uint32_t millisec=0) |
Allocate a memory block of type T. | |
T * | calloc (uint32_t millisec=0) |
Allocate a memory block of type T and set memory block to zero. | |
osStatus | put (T *mptr) |
Put a mail in the queue. | |
osEvent | get (uint32_t millisec=osWaitForever) |
Get a mail from a queue. | |
osStatus | free (T *mptr) |
Free a memory block from a mail. | |
T * | alloc (void) |
Allocate a memory block of type T from a memory pool. | |
T * | calloc (void) |
Allocate a memory block of type T from a memory pool and set memory block to zero. | |
osStatus | free (T *block) |
Return an allocated memory block back to a specific memory pool. | |
osStatus | lock (uint32_t millisec=osWaitForever) |
Wait until a Mutex becomes available. | |
bool | trylock () |
Try to lock the mutex, and return immediately. | |
osStatus | unlock () |
Unlock the mutex that has previously been locked by the same thread. | |
osStatus | put (T *data, uint32_t millisec=0) |
Put a message in a Queue. | |
osEvent | get (uint32_t millisec=osWaitForever) |
Get a message or Wait for a message from a Queue. | |
osStatus | start (uint32_t millisec) |
Start the timer. | |
int32_t | wait (uint32_t millisec=osWaitForever) |
Wait until a Semaphore resource becomes available. | |
osStatus | release (void) |
Release a Semaphore resource that was obtain with Semaphore::wait. | |
MBED_DEPRECATED_SINCE ("mbed-os-5.1","Thread-spawning constructors hide errors. ""Replaced by thread.start(task).") Thread(mbed | |
Create a new thread, and start it executing the specified function. | |
template<typename T > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.1","Thread-spawning constructors hide errors. ""Replaced by thread.start(callback(task, argument)).") Thread(T *argument | |
Create a new thread, and start it executing the specified function. | |
template<typename T , typename M > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.1","The start function does not support cv-qualifiers. ""Replaced by thread.start(callback(obj, method)).") osStatus start(T *obj | |
Starts a thread executing the specified function. | |
osStatus | join () |
Wait for thread to terminate. | |
osStatus | terminate () |
Terminate execution of a thread and remove it from Active Threads. | |
osStatus | set_priority (osPriority priority) |
Set priority of an active thread. | |
osPriority | get_priority () |
Get priority of an active thread. | |
int32_t | signal_set (int32_t signals) |
Set the specified Signal Flags of an active thread. | |
int32_t | signal_clr (int32_t signals) |
Clears the specified Signal Flags of an active thread. | |
State | get_state () |
State of this Thread. | |
uint32_t | stack_size () |
Get the total stack memory size for this Thread. | |
uint32_t | free_stack () |
Get the currently unused stack memory for this Thread. | |
uint32_t | used_stack () |
Get the currently used stack memory for this Thread. | |
uint32_t | max_stack () |
Get the maximum stack memory usage to date for this Thread. | |
static osEvent | signal_wait (int32_t signals, uint32_t millisec=osWaitForever) |
Wait for one or more Signal Flags to become signaled for the current RUNNING thread. | |
static osStatus | wait (uint32_t millisec) |
Wait for a specified time period in millisec: | |
static osStatus | yield () |
Pass control to next thread that is in state READY. | |
static osThreadId | gettid () |
Get the thread id of the current running thread. | |
static void | attach_idle_hook (void(*fptr)(void)) |
Attach a function to be called by the RTOS idle task. | |
static void | attach_terminate_hook (void(*fptr)(osThreadId id)) |
Attach a function to be called when a task is killed. |
Enumeration Type Documentation
enum State [inherited] |
State of the Thread.
- Enumerator:
Function Documentation
T* alloc | ( | uint32_t | millisec = 0 ) |
[inherited] |
T* alloc | ( | void | ) | [inherited] |
Allocate a memory block of type T from a memory pool.
- Returns:
- address of the allocated memory block or NULL in case of no memory available.
Definition at line 56 of file MemoryPool.h.
void attach_idle_hook | ( | void(*)(void) | fptr ) | [static, inherited] |
Attach a function to be called by the RTOS idle task.
- Parameters:
-
fptr pointer to the function to be called
Definition at line 345 of file Thread.cpp.
void attach_terminate_hook | ( | void(*)(osThreadId id) | fptr ) | [static, inherited] |
Attach a function to be called when a task is killed.
- Parameters:
-
fptr pointer to the function to be called
Definition at line 349 of file Thread.cpp.
T* calloc | ( | uint32_t | millisec = 0 ) |
[inherited] |
T* calloc | ( | void | ) | [inherited] |
Allocate a memory block of type T from a memory pool and set memory block to zero.
- Returns:
- address of the allocated memory block or NULL in case of no memory available.
Definition at line 63 of file MemoryPool.h.
osStatus free | ( | T * | block ) | [inherited] |
Return an allocated memory block back to a specific memory pool.
- Parameters:
-
address of the allocated memory block that is returned to the memory pool.
- Returns:
- status code that indicates the execution status of the function.
Definition at line 71 of file MemoryPool.h.
osStatus free | ( | T * | mptr ) | [inherited] |
uint32_t free_stack | ( | ) | [inherited] |
Get the currently unused stack memory for this Thread.
- Returns:
- the currently unused stack memory in bytes
Definition at line 232 of file Thread.cpp.
osEvent get | ( | uint32_t | millisec = osWaitForever ) |
[inherited] |
osEvent get | ( | uint32_t | millisec = osWaitForever ) |
[inherited] |
osPriority get_priority | ( | ) | [inherited] |
Get priority of an active thread.
- Returns:
- current priority value of the thread function.
Definition at line 155 of file Thread.cpp.
Thread::State get_state | ( | ) | [inherited] |
osThreadId gettid | ( | ) | [static, inherited] |
Get the thread id of the current running thread.
- Returns:
- thread ID for reference by other functions or NULL in case of error.
Definition at line 341 of file Thread.cpp.
osStatus join | ( | ) | [inherited] |
Wait for thread to terminate.
- Returns:
- status code that indicates the execution status of the function.
- Note:
- not callable from interrupt
Definition at line 127 of file Thread.cpp.
osStatus lock | ( | uint32_t | millisec = osWaitForever ) |
[inherited] |
uint32_t max_stack | ( | ) | [inherited] |
Get the maximum stack memory usage to date for this Thread.
- Returns:
- the maximum stack memory usage to date in bytes
Definition at line 294 of file Thread.cpp.
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.1" | , |
"Thread-spawning constructors hide errors. ""Replaced by thread.start(task)." | |||
) | [inherited] |
Create a new thread, and start it executing the specified function.
- Parameters:
-
task function to be executed by this thread. argument pointer that is passed to the thread function as start argument. (default: NULL). priority initial priority of the thread function. (default: osPriorityNormal). stack_size stack size (in bytes) requirements for the thread function. (default: DEFAULT_STACK_SIZE). stack_pointer pointer to the stack area to be used by this thread (default: NULL).
Thread thread(priority, stack_size, stack_pointer); osStatus status = thread.start(task); if (status != osOK) { error("oh no!"); }
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.1" | , |
"The start function does not support cv-qualifiers. ""Replaced by thread.start(callback(obj, method))." | |||
) | [inherited] |
Starts a thread executing the specified function.
- Parameters:
-
obj argument to task method function to be executed by this thread.
- Returns:
- status code that indicates the execution status of the function.
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.1" | , |
"Thread-spawning constructors hide errors. ""Replaced by thread.start(callback(task, argument))." | |||
) | [inherited] |
Create a new thread, and start it executing the specified function.
- Parameters:
-
obj argument to task. method function to be executed by this thread. argument pointer that is passed to the thread function as start argument. (default: NULL). priority initial priority of the thread function. (default: osPriorityNormal). stack_size stack size (in bytes) requirements for the thread function. (default: DEFAULT_STACK_SIZE). stack_pointer pointer to the stack area to be used by this thread (default: NULL).
Thread thread(priority, stack_size, stack_pointer); osStatus status = thread.start(callback(task, argument)); if (status != osOK) { error("oh no!"); }
osStatus put | ( | T * | mptr ) | [inherited] |
osStatus put | ( | T * | data, |
uint32_t | millisec = 0 |
||
) | [inherited] |
osStatus release | ( | void | ) | [inherited] |
Release a Semaphore resource that was obtain with Semaphore::wait.
- Returns:
- status code that indicates the execution status of the function.
Definition at line 40 of file Semaphore.cpp.
osStatus set_priority | ( | osPriority | priority ) | [inherited] |
Set priority of an active thread.
- Parameters:
-
priority new priority value for the thread function.
- Returns:
- status code that indicates the execution status of the function.
Definition at line 145 of file Thread.cpp.
int32_t signal_clr | ( | int32_t | signals ) | [inherited] |
Clears the specified Signal Flags of an active thread.
- Parameters:
-
signals specifies the signal flags of the thread that should be cleared.
- Returns:
- resultant signal flags of the specified thread or 0x80000000 in case of incorrect parameters.
Definition at line 171 of file Thread.cpp.
int32_t signal_set | ( | int32_t | signals ) | [inherited] |
Set the specified Signal Flags of an active thread.
- Parameters:
-
signals specifies the signal flags of the thread that should be set.
- Returns:
- previous signal flags of the specified thread or 0x80000000 in case of incorrect parameters.
Definition at line 165 of file Thread.cpp.
osEvent signal_wait | ( | int32_t | signals, |
uint32_t | millisec = osWaitForever |
||
) | [static, inherited] |
Wait for one or more Signal Flags to become signaled for the current RUNNING thread.
- Parameters:
-
signals wait until all specified signal flags set or 0 for any single signal flag. millisec timeout value or 0 in case of no time-out. (default: osWaitForever).
- Returns:
- event flag information or error code.
- Note:
- not callable from interrupt
Definition at line 329 of file Thread.cpp.
uint32_t stack_size | ( | ) | [inherited] |
Get the total stack memory size for this Thread.
- Returns:
- the total stack memory size in bytes
osStatus start | ( | uint32_t | millisec ) | [inherited] |
Start the timer.
- Parameters:
-
millisec time delay value of the timer.
- Returns:
- status code that indicates the execution status of the function.
Definition at line 43 of file RtosTimer.cpp.
osStatus terminate | ( | ) | [inherited] |
Terminate execution of a thread and remove it from Active Threads.
- Returns:
- status code that indicates the execution status of the function.
Definition at line 110 of file Thread.cpp.
bool trylock | ( | ) | [inherited] |
osStatus unlock | ( | ) | [inherited] |
uint32_t used_stack | ( | ) | [inherited] |
Get the currently used stack memory for this Thread.
- Returns:
- the currently used stack memory in bytes
Definition at line 263 of file Thread.cpp.
int32_t wait | ( | uint32_t | millisec = osWaitForever ) |
[inherited] |
Wait until a Semaphore resource becomes available.
- Parameters:
-
millisec timeout value or 0 in case of no time-out. (default: osWaitForever).
- Returns:
- number of available tokens, or -1 in case of incorrect parameters
Definition at line 36 of file Semaphore.cpp.
osStatus wait | ( | uint32_t | millisec ) | [static, inherited] |
Wait for a specified time period in millisec:
- Parameters:
-
millisec time delay value
- Returns:
- status code that indicates the execution status of the function.
- Note:
- not callable from interrupt
Definition at line 333 of file Thread.cpp.
osStatus yield | ( | ) | [static, inherited] |
Pass control to next thread that is in state READY.
- Returns:
- status code that indicates the execution status of the function.
- Note:
- not callable from interrupt
Definition at line 337 of file Thread.cpp.
Generated on Tue Jul 12 2022 11:27:30 by
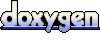