Official mbed Real Time Operating System based on the RTX implementation of the CMSIS-RTOS API open standard.
Dependents: denki-yohou_b TestY201 Network-RTOS NTPClient_HelloWorld ... more
Thread Class Reference
[Rtos]
The Thread class allow defining, creating, and controlling thread functions in the system. More...
#include <Thread.h>
Public Types | |
enum | State { Inactive, Ready, Running, WaitingDelay, WaitingInterval, WaitingOr, WaitingAnd, WaitingSemaphore, WaitingMailbox, WaitingMutex, Deleted } |
State of the Thread. More... | |
Public Member Functions | |
Thread (osPriority priority=osPriorityNormal, uint32_t stack_size=DEFAULT_STACK_SIZE, unsigned char *stack_pointer=NULL) | |
Allocate a new thread without starting execution. | |
MBED_DEPRECATED_SINCE ("mbed-os-5.1","Thread-spawning constructors hide errors. ""Replaced by thread.start(task).") Thread(mbed | |
Create a new thread, and start it executing the specified function. | |
template<typename T > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.1","Thread-spawning constructors hide errors. ""Replaced by thread.start(callback(task, argument)).") Thread(T *argument | |
Create a new thread, and start it executing the specified function. | |
template<typename T , typename M > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.1","The start function does not support cv-qualifiers. ""Replaced by thread.start(callback(obj, method)).") osStatus start(T *obj | |
Starts a thread executing the specified function. | |
osStatus | join () |
Wait for thread to terminate. | |
osStatus | terminate () |
Terminate execution of a thread and remove it from Active Threads. | |
osStatus | set_priority (osPriority priority) |
Set priority of an active thread. | |
osPriority | get_priority () |
Get priority of an active thread. | |
int32_t | signal_set (int32_t signals) |
Set the specified Signal Flags of an active thread. | |
int32_t | signal_clr (int32_t signals) |
Clears the specified Signal Flags of an active thread. | |
State | get_state () |
State of this Thread. | |
uint32_t | stack_size () |
Get the total stack memory size for this Thread. | |
uint32_t | free_stack () |
Get the currently unused stack memory for this Thread. | |
uint32_t | used_stack () |
Get the currently used stack memory for this Thread. | |
uint32_t | max_stack () |
Get the maximum stack memory usage to date for this Thread. | |
Static Public Member Functions | |
static osEvent | signal_wait (int32_t signals, uint32_t millisec=osWaitForever) |
Wait for one or more Signal Flags to become signaled for the current RUNNING thread. | |
static osStatus | wait (uint32_t millisec) |
Wait for a specified time period in millisec: | |
static osStatus | yield () |
Pass control to next thread that is in state READY. | |
static osThreadId | gettid () |
Get the thread id of the current running thread. | |
static void | attach_idle_hook (void(*fptr)(void)) |
Attach a function to be called by the RTOS idle task. | |
static void | attach_terminate_hook (void(*fptr)(osThreadId id)) |
Attach a function to be called when a task is killed. |
Detailed Description
The Thread class allow defining, creating, and controlling thread functions in the system.
Example:
#include "mbed.h" #include "rtos.h" Thread thread; DigitalOut led1(LED1); volatile bool running = true; // Blink function toggles the led in a long running loop void blink(DigitalOut *led) { while (running) { *led = !*led; Thread::wait(1000); } } // Spawns a thread to run blink for 5 seconds int main() { thread.start(led1, blink); Thread::wait(5000); running = false; thread.join(); }
Definition at line 64 of file Thread.h.
Constructor & Destructor Documentation
Thread | ( | osPriority | priority = osPriorityNormal , |
uint32_t | stack_size = DEFAULT_STACK_SIZE , |
||
unsigned char * | stack_pointer = NULL |
||
) |
Allocate a new thread without starting execution.
- Parameters:
-
priority initial priority of the thread function. (default: osPriorityNormal). stack_size stack size (in bytes) requirements for the thread function. (default: DEFAULT_STACK_SIZE). stack_pointer pointer to the stack area to be used by this thread (default: NULL).
Generated on Tue Jul 12 2022 11:27:30 by
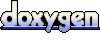