CMSIS DSP library
Dependents: performance_timer Surfboard_ gps2rtty Capstone ... more
arm_sin_f32.c
00001 /* ---------------------------------------------------------------------- 00002 * Copyright (C) 2010-2014 ARM Limited. All rights reserved. 00003 * 00004 * $Date: 21. September 2015 00005 * $Revision: V.1.4.5 a 00006 * 00007 * Project: CMSIS DSP Library 00008 * Title: arm_sin_f32.c 00009 * 00010 * Description: Fast sine calculation for floating-point values. 00011 * 00012 * Target Processor: Cortex-M4/Cortex-M3/Cortex-M0 00013 * 00014 * Redistribution and use in source and binary forms, with or without 00015 * modification, are permitted provided that the following conditions 00016 * are met: 00017 * - Redistributions of source code must retain the above copyright 00018 * notice, this list of conditions and the following disclaimer. 00019 * - Redistributions in binary form must reproduce the above copyright 00020 * notice, this list of conditions and the following disclaimer in 00021 * the documentation and/or other materials provided with the 00022 * distribution. 00023 * - Neither the name of ARM LIMITED nor the names of its contributors 00024 * may be used to endorse or promote products derived from this 00025 * software without specific prior written permission. 00026 * 00027 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS 00028 * "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00029 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS 00030 * FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE 00031 * COPYRIGHT OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, 00032 * INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, 00033 * BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00034 * LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00035 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT 00036 * LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN 00037 * ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 00038 * POSSIBILITY OF SUCH DAMAGE. 00039 * -------------------------------------------------------------------- */ 00040 00041 #include "arm_math.h" 00042 #include "arm_common_tables.h" 00043 #include <math.h> 00044 00045 /** 00046 * @ingroup groupFastMath 00047 */ 00048 00049 /** 00050 * @defgroup sin Sine 00051 * 00052 * Computes the trigonometric sine function using a combination of table lookup 00053 * and linear interpolation. There are separate functions for 00054 * Q15, Q31, and floating-point data types. 00055 * The input to the floating-point version is in radians while the 00056 * fixed-point Q15 and Q31 have a scaled input with the range 00057 * [0 +0.9999] mapping to [0 2*pi). The fixed-point range is chosen so that a 00058 * value of 2*pi wraps around to 0. 00059 * 00060 * The implementation is based on table lookup using 256 values together with linear interpolation. 00061 * The steps used are: 00062 * -# Calculation of the nearest integer table index 00063 * -# Compute the fractional portion (fract) of the table index. 00064 * -# The final result equals <code>(1.0f-fract)*a + fract*b;</code> 00065 * 00066 * where 00067 * <pre> 00068 * b=Table[index+0]; 00069 * c=Table[index+1]; 00070 * </pre> 00071 */ 00072 00073 /** 00074 * @addtogroup sin 00075 * @{ 00076 */ 00077 00078 /** 00079 * @brief Fast approximation to the trigonometric sine function for floating-point data. 00080 * @param[in] x input value in radians. 00081 * @return sin(x). 00082 */ 00083 00084 float32_t arm_sin_f32( 00085 float32_t x) 00086 { 00087 float32_t sinVal, fract, in; /* Temporary variables for input, output */ 00088 uint16_t index; /* Index variable */ 00089 float32_t a, b; /* Two nearest output values */ 00090 int32_t n; 00091 float32_t findex; 00092 00093 /* input x is in radians */ 00094 /* Scale the input to [0 1] range from [0 2*PI] , divide input by 2*pi */ 00095 in = x * 0.159154943092f; 00096 00097 /* Calculation of floor value of input */ 00098 n = (int32_t) in; 00099 00100 /* Make negative values towards -infinity */ 00101 if(x < 0.0f) 00102 { 00103 n--; 00104 } 00105 00106 /* Map input value to [0 1] */ 00107 in = in - (float32_t) n; 00108 00109 /* Calculation of index of the table */ 00110 findex = (float32_t) FAST_MATH_TABLE_SIZE * in; 00111 if (findex >= 512.0f) { 00112 findex -= 512.0f; 00113 } 00114 00115 index = ((uint16_t)findex) & 0x1ff; 00116 00117 /* fractional value calculation */ 00118 fract = findex - (float32_t) index; 00119 00120 /* Read two nearest values of input value from the sin table */ 00121 a = sinTable_f32[index]; 00122 b = sinTable_f32[index+1]; 00123 00124 /* Linear interpolation process */ 00125 sinVal = (1.0f-fract)*a + fract*b; 00126 00127 /* Return the output value */ 00128 return (sinVal); 00129 } 00130 00131 /** 00132 * @} end of sin group 00133 */
Generated on Tue Jul 12 2022 11:59:19 by
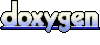